Introduction:
Express JS is a widely used and popular framework for building web applications in Node.js. It provides a robust set of features and tools that enable developers to quickly create scalable, efficient, and robust web applications.
As a widely used framework in the Node.js community, familiarity with Express JS is essential for any developer seeking to build robust web applications using Node.js. The purpose of this article is to provide a detailed tutorial on the fundamentals of Express JS and how it can be used to build web applications.
Part 1: Getting Started with Express JS
Before diving into Express JS, there are certain prerequisites that must be satisfied. You need to have Node.js and npm installed on your system. Here are the basic steps to get started with Express JS:
Installing Express JS:
Open your terminal and run the following command:
npm install express
Initializing an Express app:
Once you have installed Express JS, you can create a new Express app by running the following command:
express myapp
This will create a new directory named myapp, which contains the basic structure of an Express app.
Understanding the Basic Structure of an Express App:
An Express app has a specific structure that serves as the foundation for building web applications. The basic structure of an Express app includes the following:
- The package.json file: This is a file that contains metadata about the app, including its name, version, and various dependencies.
- The app.js file: This is the main file where you define your app configuration, including setting up routes and middleware.
- The views directory: This is where you store your app’s templates that get rendered in response to requests.
- The public directory: This is where you store static files such as images, stylesheets, and client-side JavaScript files.
Exploring Express Objects and Methods:
- The app object is the core object of an Express application. It provides you with methods for defining routes, configuring middleware, and rendering templates.
- The response object (res) represents the HTTP response that an Express app sends back to the client.
- The request object (req) represents the HTTP request that an Express app receives from the client.
Part 2: Routing in Express JS
Routing is the process of mapping URL requests to specific handlers in an application. In Express, routing is done using the app object’s methods.
Creating Basic Routes in Express:
app.get(‘/’, function(req, res) {
res.send(‘Hello World!’);
});
In this code example, we have defined a route for the root of our application. When a user navigates to this URL, the callback function responds with a message that says “Hello World!”
Using Route Parameters and Handling Dynamic Content in Routes:
app.get(‘/users/:id’, function(req, res) {
res.send(‘User ID: ‘ + req.params.id);
});
In this code example, we have defined a route that accepts a parameter for a user ID. This parameter is dynamic, meaning that it can be changed to any value and the route handler will respond accordingly.
Working with Query Parameters in Express Routes:
app.get(‘/search’, function(req, res) {
var query = req.query.q;
res.send(‘Search Query: ‘ + query);
});
In this code example, we have defined a route that accepts a query parameter. This allows users to search for a specific string on our application. The query parameter can be accessed through the req.query object.
Handling HTTP Methods in Routes:
app.post(‘/users’, function(req, res) {
// Code to create a new user
});
In this code example, we have defined a route that accepts a POST request. This is useful for creating new resources in our application, such as new users. The HTTP method used will determine which route handler gets executed.
Part 3: Middleware in Express JS
Middleware is a sequence of functions that are executed in response to a request. Middleware functions can perform various tasks, such as checking for authentication, parsing requests, or handling errors.
Creating and Using Middleware in Express JS:
To create middleware in Express JS, use the following syntax:
function customMiddleware(req, res, next) {
// Code to execute
next();
}
In this code example, we have defined a custom middleware function. This function accepts two parameters – the request object and the response object. The third parameter is the next function, which is used to pass control to the next middleware or routing function.
Handling Error Middleware:
Error middleware is used to handle errors that occur during the execution of middleware or routing functions.
Built-In Middlewares in Express JS:
Express JS provides a set of built-in middlewares, which are automatically installed when you initialize your application. Some of the built-in middleware include:
- express.static: This middleware is used to serve static files, such as images and stylesheets.
- express.urlencoded: This middleware is used for parsing URL-encoded requests.
Part 4: Template Engines in Express JS
Template engines play a crucial role in web development as they enable developers to render dynamic content on web pages. Express JS supports several template engines, including Pug, Handlebars, and EJS.
Installing Template Engines in Express:
First, you need to install the template engine of your choice using npm. For example, let’s install Pug:
npm install pug
Setting Up and Using Template Engines with Express:
app.set(‘view engine’, ‘pug’);
In this code example, we are setting the view engine to Pug. This tells Express to use Pug for rendering templates.
Using Template Inheritance and Partials:
Template inheritance is a technique used to create reusable templates, allowing you to extend or override templates throughout your application.
Handling Form Data Using Template Engines:
Form data is submitted to the server using an HTTP POST request. We can use middleware such as express.urlencoded to parse the data and make it available to our routes and templates.
Part 5: Database Integration with Express JS
Express JS supports several database systems, including MongoDB, PostgreSQL, and MySQL. Here, we will focus on MongoDB integration.
Connecting to and Querying a MongoDB Database with Express JS:
To connect to MongoDB using Express JS, you need to install the Mongoose library. Then, you can connect to MongoDB using:
const mongoose = require(‘mongoose’);
mongoose.connect(‘mongodb://localhost/dbname’);
After establishing the connection, you can create models and schemas using Mongoose.
Using Mongoose ODM with Express JS:
Mongoose is an Object Document Mapper (ODM) for MongoDB. It provides a simple API for interacting with MongoDB, making it easy to develop data-driven applications.
Part 6: Deployment of an Express JS Application
Once you have built your Express application, it’s time to deploy it. Here are some deployment strategies you can use:
- Server Deployment of an Express Application
- Deployment of Express Apps to Heroku
- Deployment of Express Apps to AWS
Conclusion:
Express JS is a powerful framework for building web applications in Node.js. With its intuitive API, powerful routing, and extensive middleware support, it provides developers with a powerful toolset for building robust, scalable, and efficient web applications. By following the guidelines outlined in this tutorial, you should now have a solid foundation for building your own Node.js web applications using Express JS.
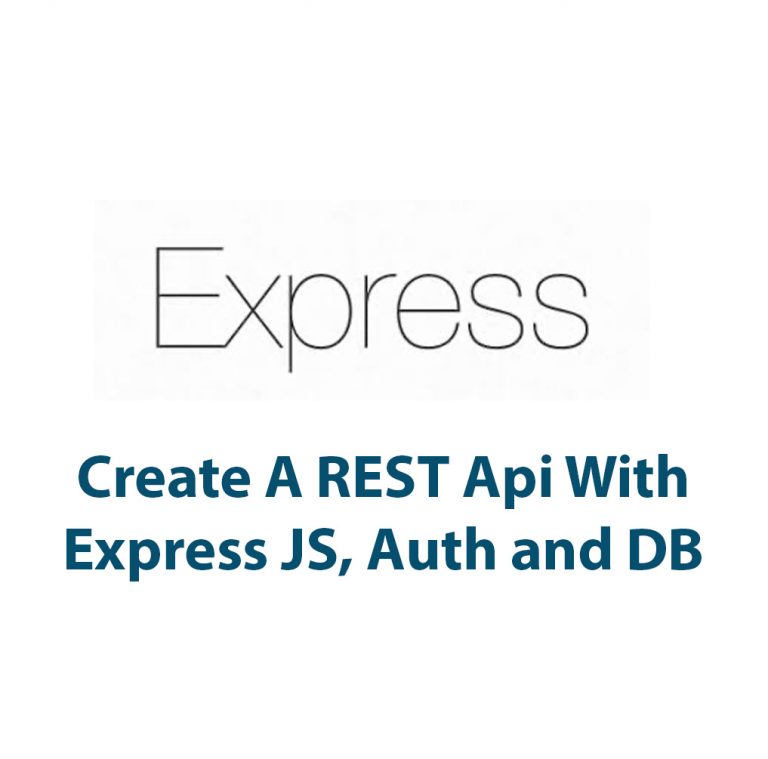
How To Create A REST Api With Express JS
Introduction Hey there! Today I’m going to walk you through the process of creating a REST API with Express JS. REST APIs have become an essential part of modern web and mobile app development, and they allow us to build efficient and scalable backends for our applications. Express JS is a powerful Node.js framework that […]