Introduction
One of the fastest-growing technologies for building large, complex applications is Node.JS. Node JS is a powerful, open-source runtime environment that allows developers to build high-performance network applications using JavaScript. It lets you run JavaScript code outside of a browser context, which means you can use it on the server-side. The platform is built on Google’s V8 JavaScript engine, which provides exceptional speed and ensures that applications written in Node JS are fast and highly scalable.
The history of Node JS dates back to 2009 when Ryan Dahl, a software engineer, created the first version of Node JS and released it to the world. The platform quickly gained traction among web developers because of its performance and scalability. Since then, it has grown by leaps and bounds, and today, it is one of the most popular development technologies in use worldwide.
Getting Started with Node JS
The first step towards learning Node JS is to install it on your computer. You can download and install Node JS from the official Node JS website. Node JS comes with its built-in package manager called npm (Node Package Manager). It allows you to install and manage packages in your Node JS projects.
The next step is to create a basic Node JS server, which is relatively simple. First, create a new file in your project’s directory called server.js, and add the following code:
const http = require("http");
http.createServer((request, response) => {
response.writeHead(200, { "Content-Type": "text/plain" });
response.end("Hello, Node JS!");
}).listen(8080);
console.log("Server running at http://localhost:8080/");
In the code above, we have used the http
module, a built-in module that provides HTTP server and client functionality. We then create a server using the http.createServer()
method and specify a callback function to handle incoming HTTP requests. The response.writeHead()
method specifies the response header, and the response.end()
method sends the response body.
To start your Node JS server, run the following command in your terminal:
node server.js
Open your web browser and navigate to http://localhost:8080/
, and you should see the message “Hello, Node JS!” displayed on the screen.
Understanding Node JS Modules and Packages
Node JS uses a concept called modules to organize code into a reusable form. A module in Node JS can be any file or directory in your project that contains Node JS code. Each module has its scope, and its functions and variables are not accessible from outside the module.
You can create a new module by creating a new file with a .js
extension in your project directory. The contents of the file are treated as a module, and the code is executed in its private scope. To make the contents of a module available in another module, you need to export them using the module.exports
object.
Node JS also has a vast ecosystem of packages that you can use to build complex applications. A package is a collection of modules that is distributed using the Node Package Manager (npm). You can download any package from the npm registry and install it in your project using the npm install
command.
Node JS Fundamentals
Asynchronous Programming in Node JS
Asynchronous programming is a programming paradigm where the program’s execution flow is not blocked by long-running I/O operations. The idea behind it is to execute code in parallel, which is particularly useful when working with I/O operations such as reading and writing to files and databases.
Node JS has a built-in module called fs
, which provides an interface for interacting with the file system. You can use it to read and write files asynchronously with the fs.readFile()
and fs.writeFile()
methods.
const fs = require("fs");
fs.readFile("data.txt", "utf-8", (err, data) => {
if (err) throw err;
console.log(data);
});
In the code above, we use the fs.readFile()
method to read data from a file called data.txt
. The second parameter passed to the method is the file encoding, and the third parameter is a callback function that is executed after the file has been read. The callback function receives two arguments: an error object and the file data.
Handling and Manipulating Files
Node JS’s fs
module provides several methods for manipulating files. Some of the most common ones include:
fs.writeFile()
– Used to write data to a file.fs.appendFile()
– Used to append data to a file.fs.rename()
– Used to rename a file.fs.unlink()
– Used to delete a file.
Sending and Receiving Data Using Node JS
Node JS has built-in support for sending and receiving data over HTTP using the http
module. Here’s an example of how to send an HTTP GET request and receive data:
const http = require("http");
http.get("http://jsonplaceholder.typicode.com/posts", (response) => {
let data = "";
response.on("data", (chunk) => {
data += chunk;
});
response.on("end", () => {
console.log(JSON.parse(data));
});
});
In the code above, we use the http.get()
method to send an HTTP GET request to an API that returns a list of posts in JSON format. We then use the response
object to listen for data events and store the received data in a variable. Finally, when the response has ended, we log the received data to the console after parsing it as JSON.
Node JS Express Framework
What is Express?
Express is a popular web framework for Node JS that simplifies the process of building complex web applications. It provides a set of features and tools that allow you to create RESTful APIs, web applications, and web services easily.
Creating a Basic Express Server
To use Express in your Node JS project, you first need to install it using the following command:
npm install express
Once installed, you can create a basic Express server by creating a new file and adding the following code:
const express = require("express");
const app = express();
app.get("/", (req, res) => {
res.send("Hello, Express!");
});
app.listen(8080, () => {
console.log("Server running at http://localhost:8080/");
});
In the code above, we have created a new instance of the express application using the express()
function. We then define a route using the app.get()
method that responds with the message “Hello, Express!” when a user navigates to the root URL.
Handling HTTP Requests with Express
Express makes it easy to handle HTTP requests using its built-in methods. You can use the app.get()
, app.post()
, app.put()
, and app.delete()
methods to handle GET, POST, PUT, and DELETE requests, respectively.
Here’s an example of how to create a route that handles a POST request:
app.post("/users", (req, res) => {
// Handle POST request
...
});
In the code above, we have created a new route that handles a POST request to the “/users” URL. We can access the request body using the req.body
object.
Building a Full-Stack Application with Node JS
Integrating Node JS with Databases
Node JS provides several libraries and modules for connecting to different databases, such as MongoDB and MySQL. You can use these libraries to store and retrieve data from your database.
Here’s an example of how to connect to a MongoDB database using the mongodb
module:
“`
const MongoClient = require(“mongodb”).MongoClient;
const url = “mongodb://localhost
27017/mydatabase”;
MongoClient.connect(url, (err, db) => {
if (err) throw err;
console.log(“Database created!”);
db.close();
});
“`
In the code above, we have used the MongoClient
constructor to create a new database connection. We then specify the URL of the MongoDB database and a callback function to handle the response. The callback function logs a message to the console if the connection is successful.
Creating APIs with Node JS
A RESTful API is an application programming interface (API) that follows the principles of Representational State Transfer (REST). REST API allows client applications to interact with a server using HTTP verbs such as GET, POST, PUT, and DELETE.
With Node JS, you can easily create RESTful APIs using the Express framework and other libraries. Here’s an example of how to create an API that returns a list of products:
const express = require("express");
const app = express();
const products = [
{ id: 1, name: "Product 1", price: 10.99 },
{ id: 2, name: "Product 2", price: 14.99 },
{ id: 3, name: "Product 3", price: 19.99 },
];
app.get("/api/products", (req, res) => {
res.json(products);
});
app.listen(8080, () => {
console.log("Server running at http://localhost:8080/");
});
In the code above, we have created a new route in our Express application that responds to GET requests to the “/api/products” URL. The route returns an array of product objects in JSON format using the res.json()
method.
Connecting Node JS with Front-End Frameworks
Node JS works seamlessly with front-end frameworks such as React, Angular, and Vue. These frameworks allow you to build modern, dynamic user interfaces easily.
When using Node JS with front-end frameworks, you can use npm to manage your dependencies and build tools. Most front-end frameworks come with a command-line interface (CLI) that allows you to generate new projects and components.
Here’s an example of how to create a new React application using Create React App:
npx create-react-app my-app
cd my-app
npm start
In the code above, we have used the npx
command to create a new React application called “my-app”. We then navigate into the project directory and start the development server using the npm start
command.
Node JS Deployment and Scaling
Once you have created your Node JS application, you need to deploy it to a web server to make it available to users. There are several ways you can deploy a Node JS application, including using a cloud provider such as AWS or Google Cloud, or deploying to a dedicated server.
Deploying Node JS Applications to a Web Server
To deploy a Node JS application to a web server, you first need to ensure that Node JS is installed on the server. You can then copy your project files to the server and install any necessary dependencies using npm.
Here’s a simple deployment process for a Node JS application:
- Copy your project files to the server using a tool such as FTP or SCP.
- Install Node JS and npm on the server.
- Install any necessary dependencies using the
npm install
command. - Start the Node JS server using the
node server.js
command. - Use a process manager such as PM2 to ensure that your Node JS application is always running.
Using PM2 to Manage Node JS Processes
PM2 is a process manager for Node JS applications that provides advanced features such as automatic server restarts and load balancing. With PM2, you can easily deploy and manage multiple Node JS applications on a single server.
To install PM2, use the following command:
npm install pm2 -g
Once installed, you can use the pm2 start
command to start your Node JS application and the pm2 list
command to list all running processes.
pm2 start server.js
pm2 list
Scaling Node JS Applications with Load Balancers
If your Node JS application receives a lot of traffic, you can use a load balancer to distribute the traffic across multiple servers. Load balancing helps improve the performance and availability of your application by preventing any single server from becoming overwhelmed.
There are several load balancing solutions available for Node JS, including Nginx, HAProxy, and LoadBalance.io.
Conclusion
Node JS is a powerful and flexible platform for building high-performance network applications using JavaScript. It provides a vast ecosystem of packages and libraries that make it easy to build complex applications quickly.
In this article, we have covered the basics of Node JS, including how to create a basic server, handle files, and send and receive data. We have also explored the Express framework and how to create RESTful APIs and connect Node JS with databases and front-end frameworks.
Finally, we have looked at how to deploy and scale Node JS applications, including using process managers and load balancers. With this knowledge, you are well-equipped to start building powerful applications using Node JS.
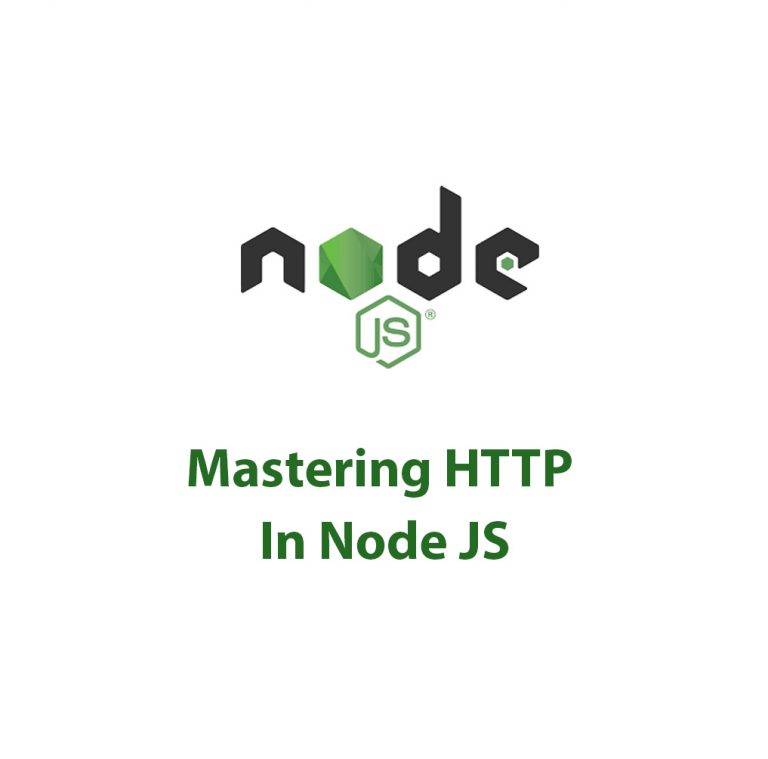
Working With HTTP In Node JS
Introduction As a developer, you may have come across the term HTTP quite a few times. It stands for Hypertext Transfer Protocol and is the backbone of how the internet works. It is the protocol you use when you visit a website, send emails, and watch videos online. In the world of programming, HTTP is […]
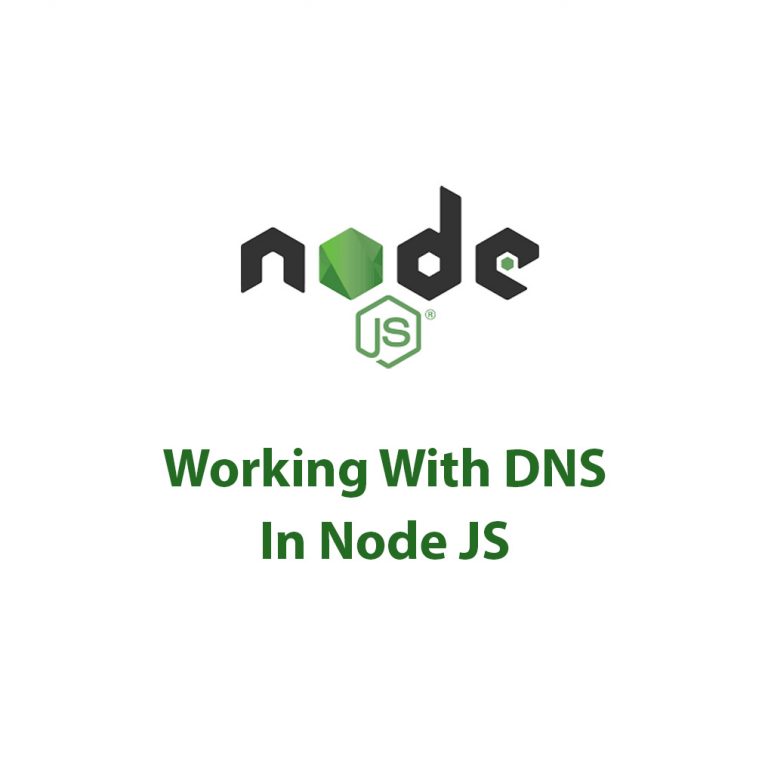
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]
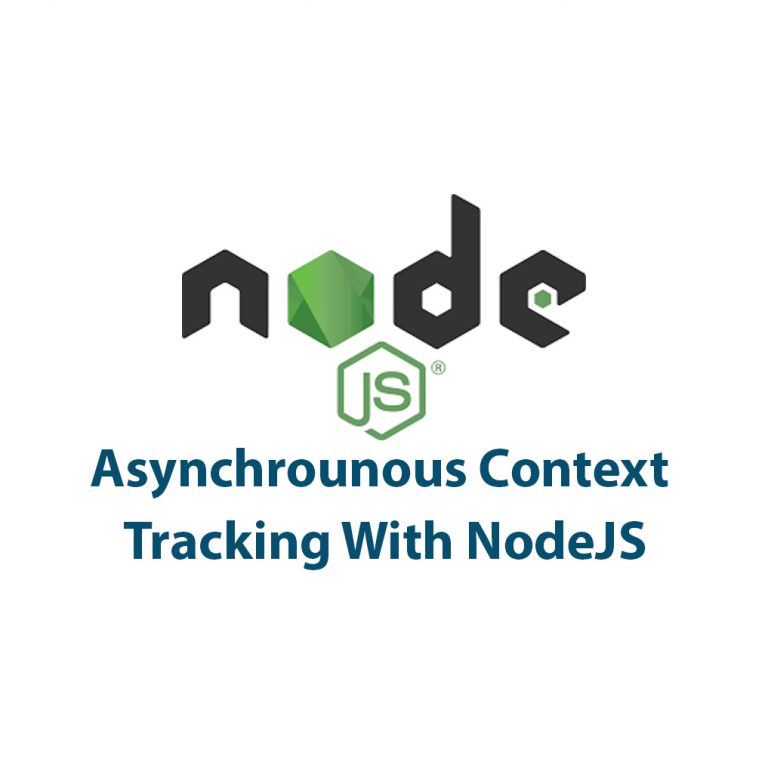
Asynchronous Context Tracking With Node JS
As someone who has spent a lot of time working with Node JS, I have come to understand the importance of Asynchronous Context Tracking in the development of high-performance applications. In this article, I will explore the concept of Asynchronous Context Tracking with Node JS, its advantages, techniques, challenges and best practices. Before we dive […]
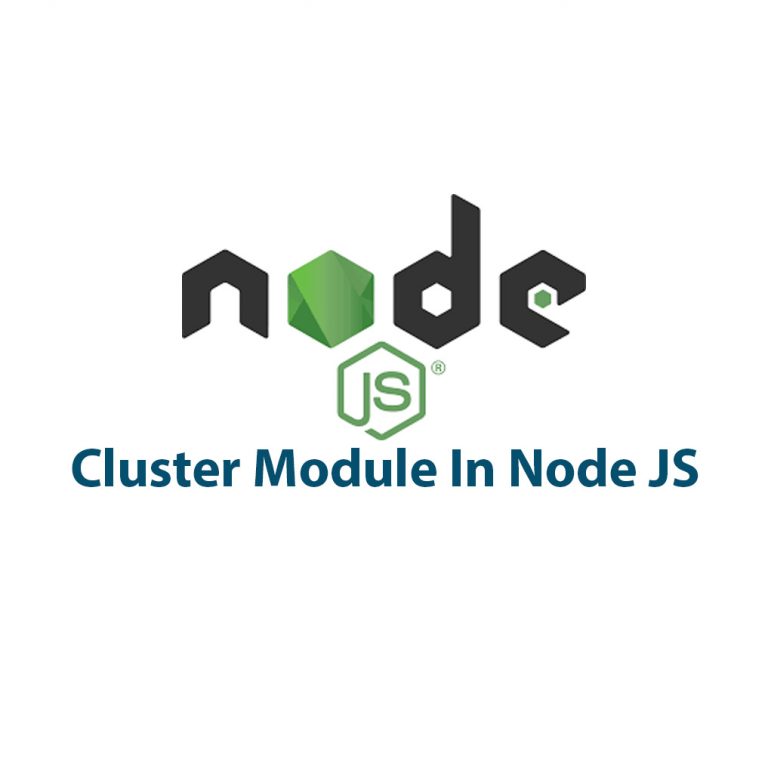
How To Cluster In Node JS
As a developer, I’ve always been interested in exploring different ways to improve the performance of my Node JS applications. One tool that has proven to be immensely beneficial is cluster module. In this article, we’ll dive deep into clustering for Node JS, how it works, how to implement it, and the benefits it provides. […]
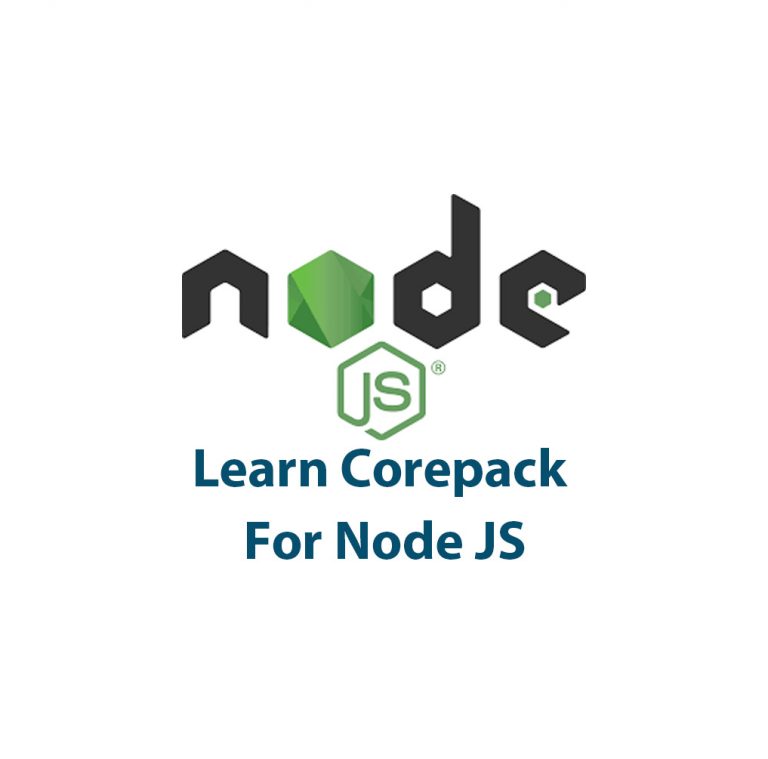
Corepack In Node JS
Have you ever worked on a Node JS project and struggled with managing dependencies? You’re not alone! Managing packages in Node JS can get confusing and messy quickly. That’s where Corepack comes in. In this article, we’ll be diving into Corepack in Node JS and how it can make dependency management a breeze. First of […]
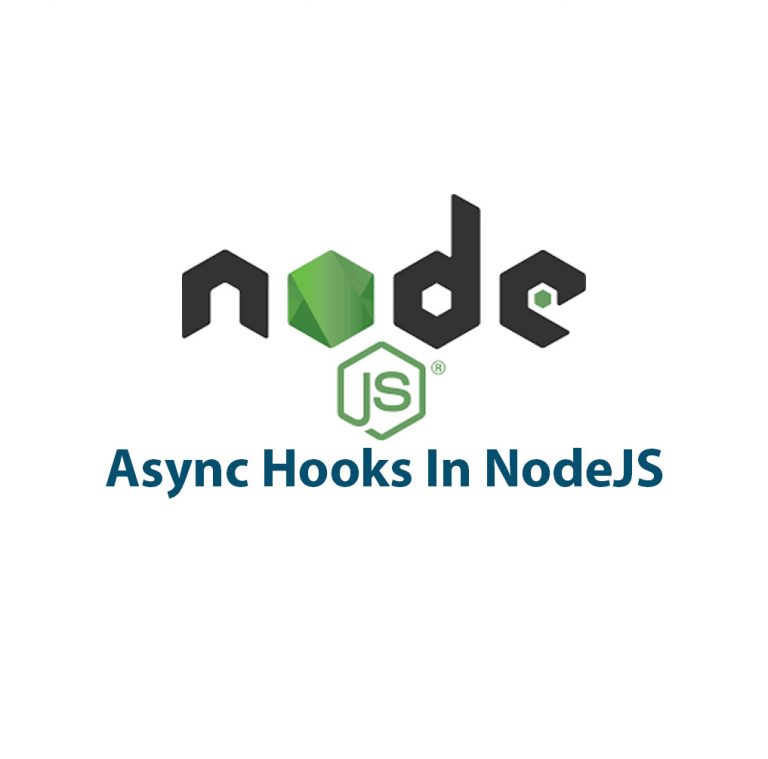
Async Hooks In Node JS
Introduction: If you’re a Node.js developer, you’ve probably heard the term “Async Hooks” thrown around in conversation. But do you know what they are or how they work? In this article, I’ll be diving into the world of Async Hooks, explaining what they are and how to use them effectively. What are Async Hooks? Async […]