React JS is an open-source JavaScript library that has revolutionized the way web developers create and build single-page applications. It is used by some of the biggest companies in the world, including Facebook, Instagram, Netflix, and Airbnb. If you’re passionate about web development and are looking to add new skills to your resume, then learning React JS will be crucial.
This React JS tutorial is perfect for web developers who are just starting with front-end web development and need to learn the basics of React. In this tutorial, we will go through the process of setting up a development environment, the basics of React components, using state, props, event handling, and conditional rendering.
Setting up the Development Environment
Before we can start writing any code, we need to set up our development environment. The following steps will guide you on how to do that:
- Installing Node.js and NPM
The first step to setting up your environment is to install Node.js and NPM (Node Package Manager). Node.js is a JavaScript runtime environment that allows developers to run JavaScript code outside the browser. Meanwhile, NPM is a package manager that is used to install third-party libraries and packages.
To install Node.js, visit https://nodejs.org/en/download/ website and download the version that is compatible with your operating system. Once downloaded, run the installation file and follow the prompts on the screen.
- Installing the React JS library
Once Node.js and NPM are installed, the next step is to install the React JS library. To do that, open your command prompt and run the following command:
npm install -g create-react-app
This command will install the create-react-app CLI tool globally. This tool enables you to create new React projects quickly. After the installation is complete, navigate to the directory where you would like your project to live and run the following command:
create-react-app my-react-app
This command will create a new React project in a directory called my-react-app.
- Creating a new React project using Create React App tool
Finally, navigate to your project directory and start the React development server using the following command:
cd my-react-app && npm start
Congratulations, you now have a working React development environment. Now it’s time to start coding.
The Basics of React JS
React JS uses a component-based architecture, which makes it easy to break up your user interface into reusable pieces of code. Before we dive deep into creating components, let’s first understand what they are.
Understanding React Components
A React component is a reusable piece of HTML code that encapsulates some data and functionality. It is intended to be modular and reusable across different parts of your application. Every React application has at least one React component, which is known as the root component.
There are two ways to define components in React, the functional and class-based approach.
Creating Components Using a Functional and Class-Based Approach
Functional components are JavaScript functions that accept props (short for properties) and return a React element. Props are properties that can be passed down to a component from its parent component. The following code is an example of how to create a basic functional component in React.
function Greetings(props) {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
}
The above component takes a single prop called name and displays “Hello, name!” in the browser. Props are passed as key-value pairs in a JavaScript object. For example, if you wanted to render the Greetings component with the name “John”, you would pass the following prop:
<Greetings name="John" />
Class-based components are similar to functional components, but they are declared using ES6 classes. They offer more features and are more powerful than functional components. Here’s an example of a class-based component in React:
import React, { Component } from 'react';
class Button extends Component {
constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log("Button clicked");
}
render() {
return (
<button onClick={this.handleClick}>
Click Me
</button>
);
}
}
export default Button;
The above class-based component is called Button. It has a constructor that accepts props, a handleClick method that logs a message to the console when the button is clicked, and a render method that returns a button element with an onClick event listener attached to it.
Rendering Components in the DOM
The final step in creating a React component is to render it in the browser. To do this, we use the ReactDOM library, which is a part of React. Here’s an example of how to render a component in the browser:
import React from 'react';
import ReactDOM from 'react-dom';
import Greetings from './Greetings';
ReactDOM.render(
<Greetings name="John" />,
document.getElementById('root')
);
The code above uses the ReactDOM.render() method to render the Greetings component to the element with an ID of root in the index.html file.
State and Props
In React, there are two types of data that we deal with, state and props. Understanding the difference between state
and props is crucial when building React applications.
Understanding State and Props in React
Props are properties passed into a component from its parent component. They are read-only and cannot be modified by the component they are passed to. State, on the other hand, is local data that is used within a component that can be changed by that component.
Passing Data Between Components Using Props
Props are used to pass data from a parent component to a child component. This is achieved by passing data through the child component’s props. Here’s an example of a parent component passing down a prop to a child component:
import React from 'react';
import ChildComponent from './ChildComponent';
class ParentComponent extends React.Component {
render() {
return (
<div>
<ChildComponent myProp="Hello, World!" />
</div>
);
}
}
export default ParentComponent;
The code above creates a ParentComponent that renders a ChildComponent and passes down a prop called myProp as a string.
Updating a Component’s State Using setState()
A component’s state can only be updated using the setState() method provided by React. Here’s an example:
import React from 'react';
class ExampleComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
counter: 0
};
}
handleIncrement = () => {
this.setState({
counter: this.state.counter + 1
});
};
render() {
return (
<div>
<p>{this.state.counter}</p>
<button onClick={this.handleIncrement}>Increment</button>
</div>
);
}
}
export default ExampleComponent;
The code above creates an ExampleComponent with a state property called counter, which is initialized to 0 in the constructor. The component has a handleIncrement method that is executed when the button is clicked, which increments the counter value by 1 using the setState() method.
Handling User Events
React provides an easy way to handle user events within components using event handlers. Here’s an example of how to create an event handler for a button element using React:
import React from 'react';
class Button extends React.Component {
handleClick = () => {
alert('Button clicked');
}
render() {
return (
<button onClick={this.handleClick}>Click Me</button>
);
}
}
export default Button;
The above code creates a button element with an onClick event handler that triggers an alert when clicked.
Conditional Rendering and CSS Styling
React allows you to conditionally render components based on different conditions. Here’s an example:
import React from 'react';
class ExampleComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
isLoggedIn: false,
};
}
render() {
const isLoggedIn = this.state.isLoggedIn;
if (isLoggedIn) {
return <h1>Welcome back!</h1>;
}
return <h1>Please log in</h1>;
}
}
export default ExampleComponent;
The code above creates an ExampleComponent that conditionally renders the text “Welcome back!” or “Please log in” based on the value of isLoggedIn. If isLoggedIn is true, it will render the first statement; otherwise, it will render the second statement.
You can also style your React components using CSS. Here’s an example of how to do that:
import React from 'react';
import './Button.css';
class Button extends React.Component {
render() {
return (
<button className="custom-button">
Click Me
</button>
);
}
}
export default Button;
The code above creates a Button element that uses an external CSS file called Button.css to style the button with a custom class called “custom-button”.
React Router
React Router is a powerful library for managing routes in a React application. It allows you to navigate between different pages of your application without the need for reloading the page. Here’s an example of how to use React Router in a React application:
import React from 'react';
import { BrowserRouter as Router, Switch, Route, Link } from 'react-router-dom';
import HomePage from './HomePage';
import AboutPage from './AboutPage';
import ContactPage from './ContactPage';
const App = () => {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
<Switch>
<Route path="/about">
<AboutPage />
</Route>
<Route path="/contact">
<ContactPage />
</Route>
<Route path="/">
<HomePage />
</Route>
</Switch>
</div>
</Router>
);
};
export default App;
The code above creates a React application with three pages: HomePage, AboutPage, and ContactPage. The app uses React Router to manage the different routes and renders the relevant
component when the route changes. The Link component is used to create navigation links within the application.
Conclusion
React JS is a powerful tool that can help you build complex and dynamic single-page applications. In this tutorial, we covered the basics of React development, including setting up the development environment, creating components using both functional and class-based approaches, using state and props, handling user events, and conditional rendering and styling with CSS.
We also covered the use of React Router to manage navigation within a React application. With this knowledge, you can start building your own React applications and dive deeper into the world of front-end web development. Remember that practice makes perfect, so keep coding and experimenting with React to become a better developer.
Happy coding!
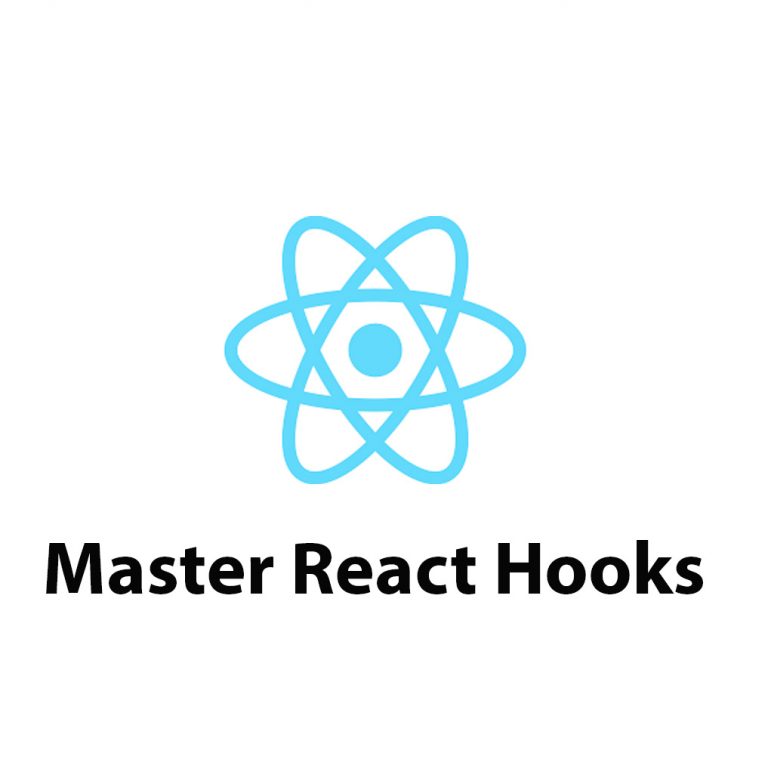
Mastering Hooks In React JS
Introduction React JS is a popular JavaScript library that has gained a lot of popularity since its introduction in 2013. It has been adopted by many web developers who use it to build robust and reliable web applications. With React JS, developers can create user interfaces by building reusable UI components, which can be combined […]
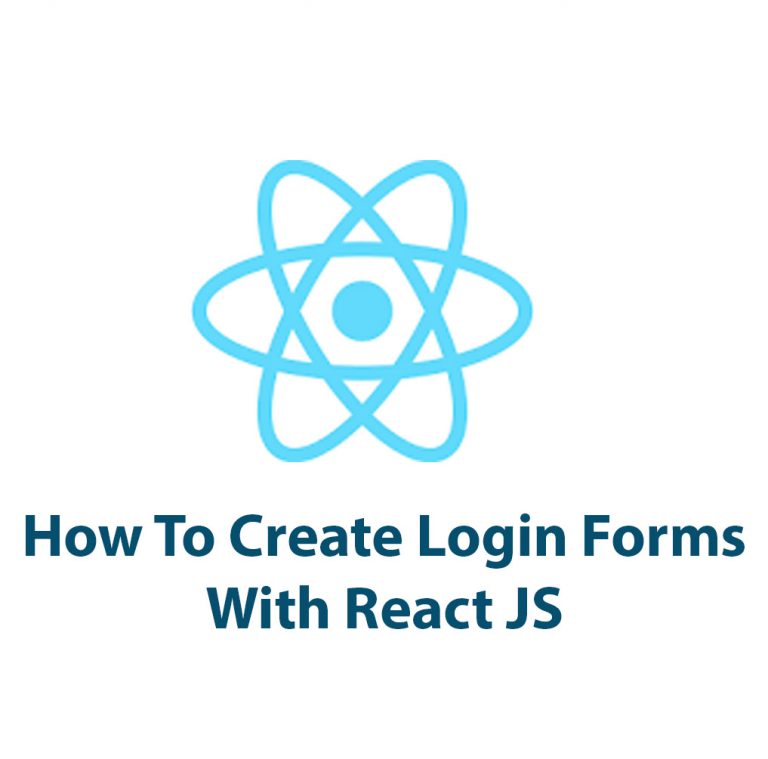
Create A Login Form In React
Creating a login form with React is an essential component in web development that can be accomplished in a few simple steps. In this article, we will walk through the process of creating a login form with React and explore its benefits. Before we begin, let’s take a moment to understand React. React is a […]
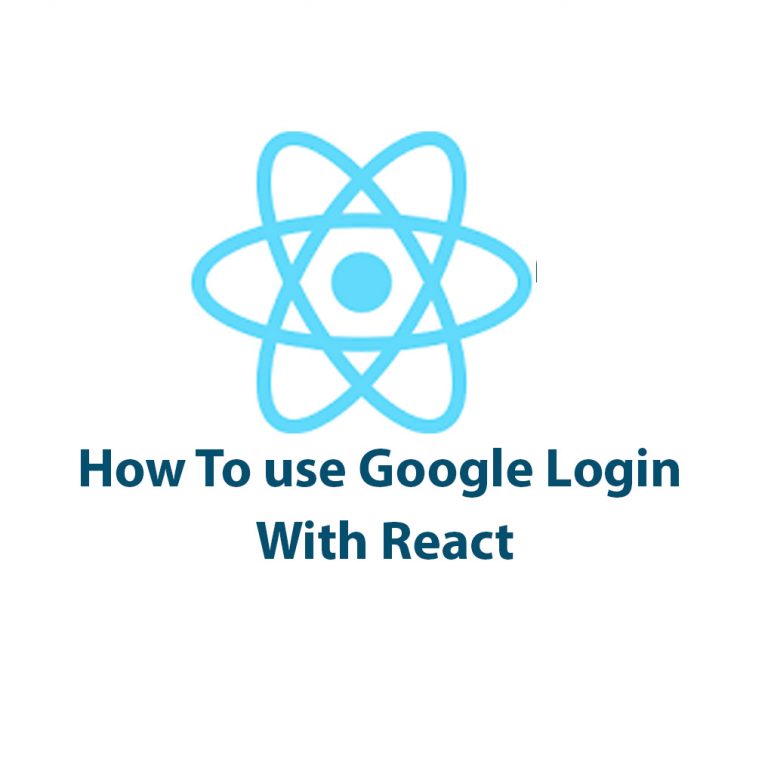
How To Use Google Login With React
As a developer, I cannot stress enough the importance of creating a seamless user experience when it comes to authentication. One of the most popular and convenient ways to allow users to authenticate is by using Google Login. In this article, we’ll explore how to use Google Login with React. Before we delve deep into […]
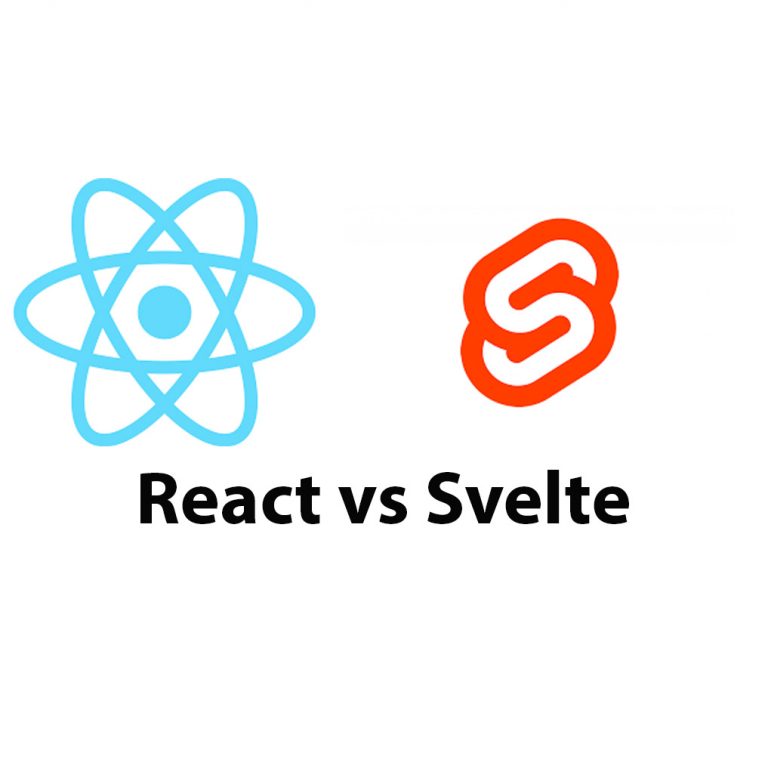
React JS vs Svelte
Introduction In the world of web development, there are many frameworks available for use. Two of the most popular frameworks are React JS and Svelte. React JS is an open-source JavaScript library developed by Facebook while Svelte is a relatively new framework developed by Rich Harris in 2016. In this article, we will compare React […]
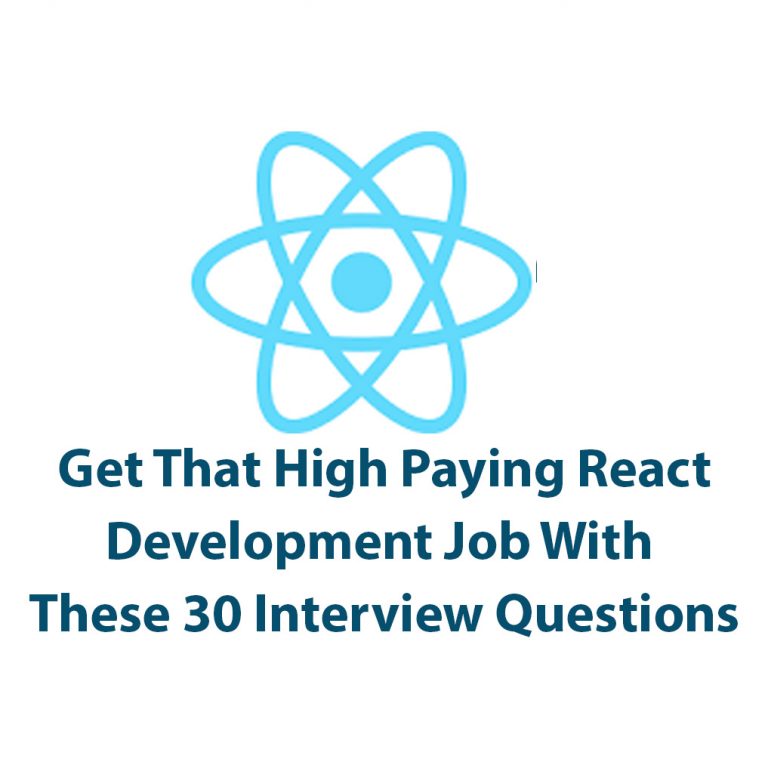
React JS Interview Questions (and answers)
Introduction: React is a powerful JavaScript library with a growing presence in web development today. With its powerful rendering capabilities and cross-functional library, it has become an important skill for developers to learn. In order to prepare for React interviews, it is important to familiarize yourself with React interview questions, which cover a range of […]
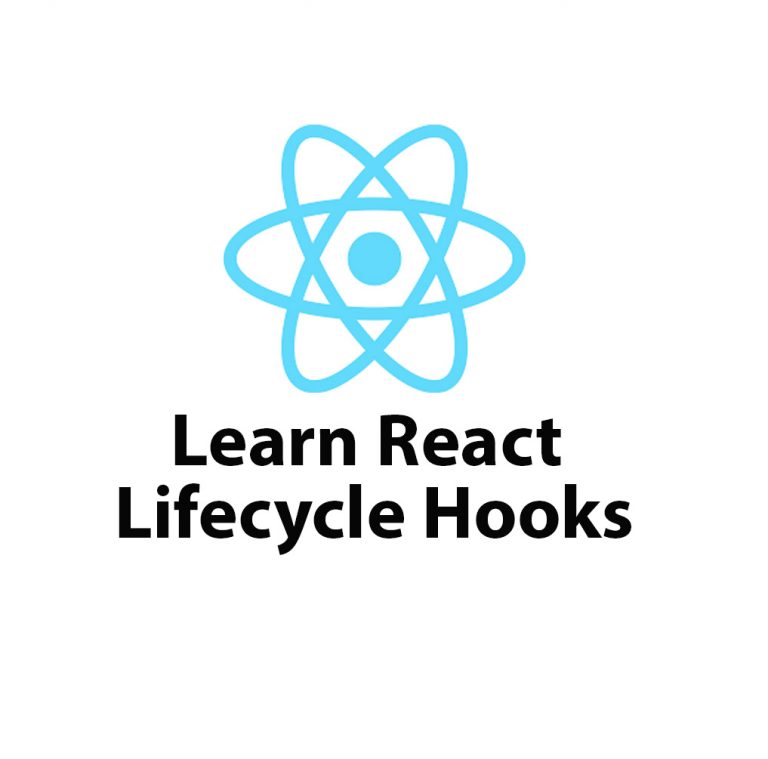
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]