Sorting Algorithm | Average Time Complexity | Worst Case Time Complexity | Space Complexity | Stability | Description |
---|---|---|---|---|---|
Bubble Sort | O(n^2) | O(n^2) | O(1) | Stable | Simple and easy to implement. Used on small data sets or as a simple teaching tool. |
Selection Sort | O(n^2) | O(n^2) | O(1) | Unstable | Simple and easy to implement. Used on small data sets or as a simple teaching tool. |
Insertion Sort | O(n^2) | O(n^2) | O(1) | Stable | Simple and efficient for small data sets or partially sorted data. |
Merge Sort | O(n log n) | O(n log n) | O(n) | Stable | Efficient for sorting large amounts of data. Used in external sorting and in parallel computing. |
Quick Sort | O(n log n) | O(n^2) | O(log n) | Unstable | Efficient for sorting large amounts of data. Used in practice for its average case performance, but can have poor worst-case performance. |
Heap Sort | O(n log n) | O(n log n) | O(1) | Unstable | Efficient for sorting large amounts of data. Used in practice when the size of the data set is unknown or unbounded. |
Radix Sort | O(dn) | O(dn) | O(n + k) | Stable | Efficient for sorting data with a fixed number of digits or keys. Used in practice for sorting strings, integers, or IP addresses. |
Counting Sort | O(n + k) | O(n + k) | O(n + k) | Stable | Efficient for sorting integers with a small range. Used in practice for sorting grades, exam scores, and frequencies. |
Bucket Sort | O(n + k) | O(n^2) | O(n + k) | Stable | Efficient for sorting data with a uniform distribution. Used in practice for sorting letters, names, and ranges. |
Shell Sort | O(n log n) | Depends on gap sequence | O(1) | Unstable | Efficient for sorting data with a large number of elements. Used in practice for sorting word lists and arrays. |
Comb Sort | Depends on gap sequence | O(n^2) | O(1) | Unstable | Efficient for sorting data with a large number of elements. Used in practice for sorting word lists and arrays. |
Cocktail Sort | O(n^2) | O(n^2) | O(1) | Stable | Variation of bubble sort. Used in practice for sorting word lists and arrays. |
Gnome Sort | O(n^2) | O(n^2) | O(1) | Stable | Efficient for sorting small data sets. Used in practice for sorting word lists and arrays. |
Pancake Sort | O(n^2) | O(n^2) | O(n) | Unstable | Efficient for sorting data with limited number of flips. Used in practice for sorting word lists and arrays. |
Bogo Sort | O(n*n!) | O(∞) | O(1) | Unstable | Inefficient and impractical for large data sets. Used as a benchmark for comparing other sorting algorithms. |
Stooge Sort | O(n^2.71) | O(n^?) | O(n) | Unstable | Recursively sorts a quarter of the array pass by swapping with another quarter. Used in practice for sorting large and small arrays. |
Batcher Sort | O(n log^2 n) | O(n log^2 n) | O(n) | Unstable | Based on mergesort. Used to sort large and small arrays. |
Odd-even Sort | O(n^2) | O(n^2) | O(1) | Stable | Similar to bubble sort. Used for sorting small arrays. |
Bitonic Sort | O(n log^2 n) | O(n log^2 n) | O(n log n) | Unstable | Based on mergesort. Used for data with a power of two size. |
Cycle Sort | O(n^2) | O(n^2) | O(1) | Stable | Efficient for sorting data with repeated elements. Used in practice for sorting words lists and arrays. |
Pigeonhole Sort | O(n + k) | O(n + k) | O(n + k) | Stable | Efficient for sorting data with integer keys within a small range. Used in practice for sorting ages, birthdates, phone numbers, and colors. |
Library Sort | O(n log n) | O(n log n) | O(n log n) | Stable | Based on radix sort. Used for sorting data with variable-length keys. |
Binary Tree Sort | O(n log n) | O(n^2) | O(n) | Stable | Builds a binary search tree. Used in practice for sorting large and small data sets. |
Bead Sort | O(n) | O(n^2) | O(n) | Unstable | Efficient for sorting non-negative integers with a small range. Used in practice for sorting trinkets, jewelry, and toys. |
Smooth Sort | O(n log n) | O(n log n) | O(1) | Unstable | Combines heap sort with Fibonacci heaps. Used in practice for sorting data sets with a skewed distribution. |
Timsort | O(n log n) | O(n log n) | O(n) | Stable | Combines insertion sort and merge sort. Used in practice for sorting arrays, lists, and stable sorts. |
Cube Sort | O(n log n) | O(n log n) | O(n log n) | Unstable | Efficient for sorting numeric data. Used in practice for sorting scientific data. |
Sleep Sort | O(n) | O(n) | O(1) | Unstable | Sorts integers in time proportional to the largest number. Used in practice for fun purposes. |
Introsort | O(n log n) | O(n log n) | O(log n) | Unstable | Based on quick sort with additional steps. Used in practice for sorting data that contains duplicates. |
Flashsort | O(n + k) | O(n^2) | O(k) | Unstable | Efficient for sorting data with a uniform distribution. Used in practice for sorting data from physical measurements. |
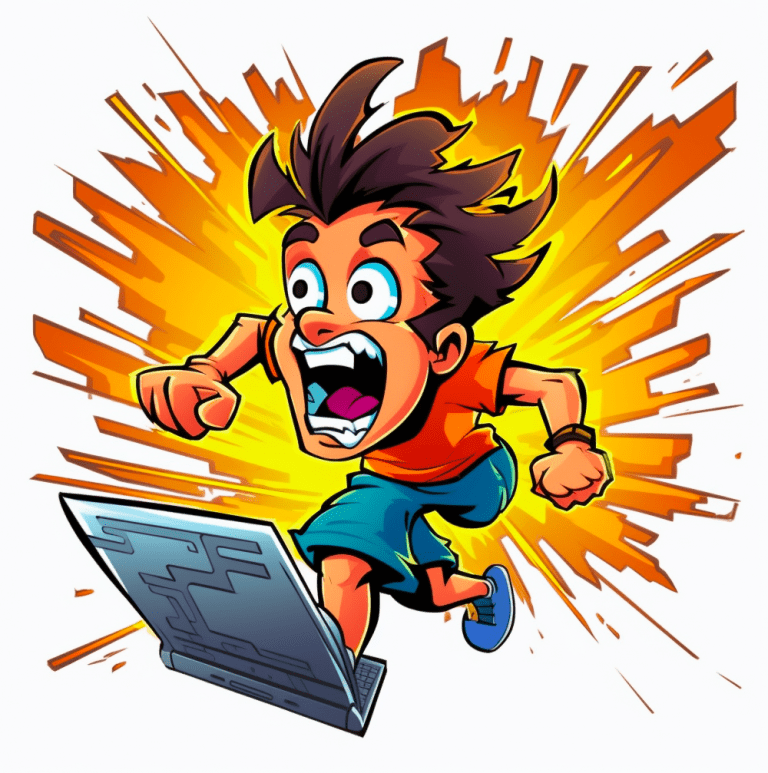
How To Write Quicksort: In Typescript
Introduction: When we talk about sorting algorithms, Quick Sort is one of the most widely used ones. It is a fast, efficient, and in-place algorithm. Typescript on the other hand, is a superset of Javascript which adds static typing, class-based object-oriented programming, and improved syntax to the language. It has gained popularity among developers due […]
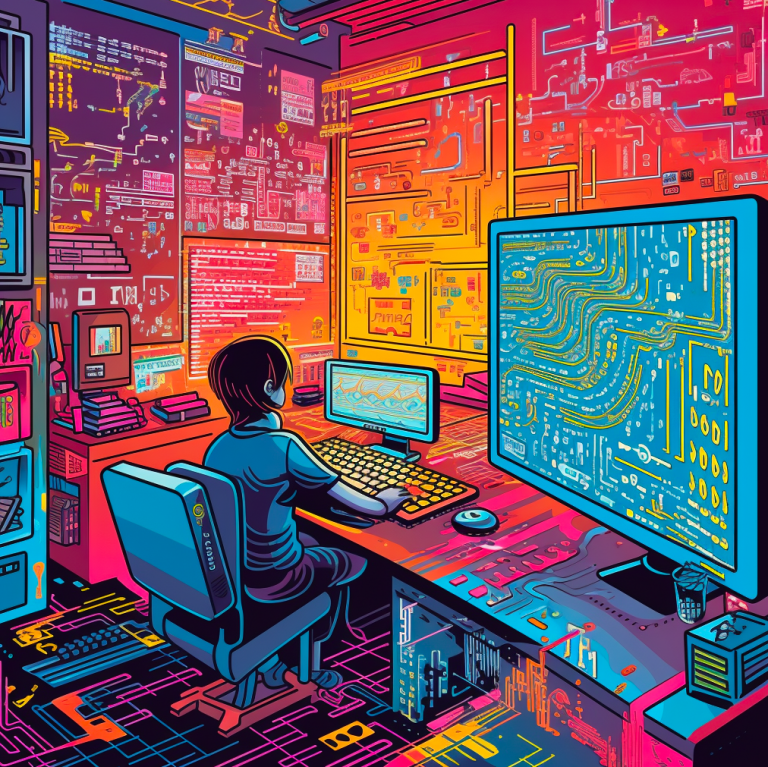
The Different Types Of Algorithms
Algorithms are an integral part of computer science and software development. They are step-by-step procedures for solving problems and are used in various applications such as data processing, artificial intelligence, and cryptography. Different types of algorithms are used to solve specific types of problems. In this article, we will explore the different types of algorithms […]
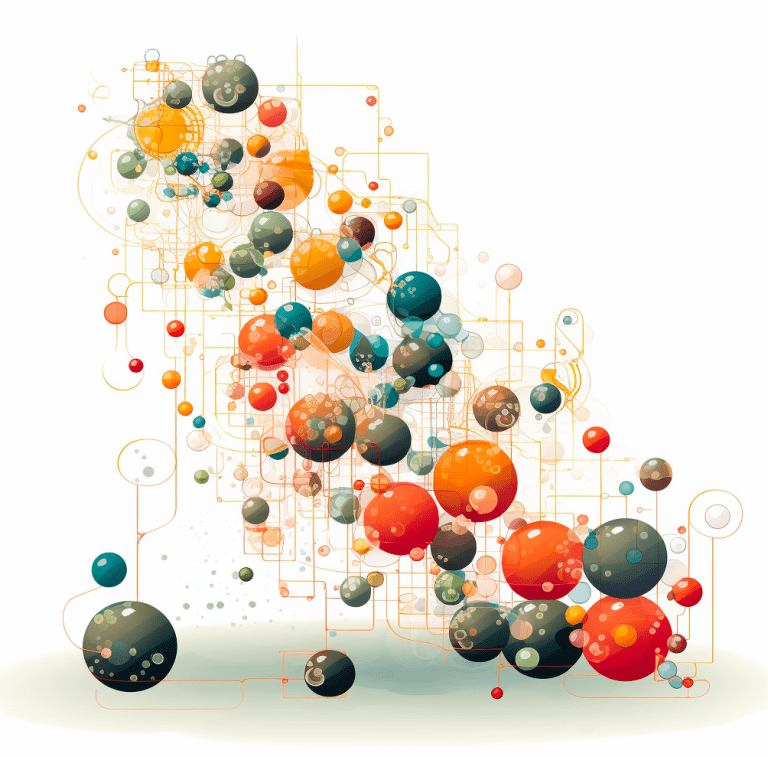
How To Write Bubble Sort: In Typescript
Introduction Bubble sort is a popular sorting algorithm that is commonly used in computer science, and has been around for many years. The algorithm sorts an array of elements by continuously swapping neighboring elements until the array is sorted. In this article, we will be discussing how to implement bubble sort in Typescript, which is […]