Algorithms are an integral part of computer science and software development. They are step-by-step procedures for solving problems and are used in various applications such as data processing, artificial intelligence, and cryptography. Different types of algorithms are used to solve specific types of problems. In this article, we will explore the different types of algorithms and their applications.
Sorting Algorithms
Sorting algorithms are used to rearrange data in a specific order. The most common sorting algorithms are Bubble Sort, Insertion Sort, Selection Sort, Quick Sort, and Merge Sort. Bubble Sort compares adjacent pairs of elements and swaps them if they are in the wrong order. Insertion Sort works by dividing the data into two sections: sorted and unsorted. It then picks an element from the unsorted section and places it in the correct position in the sorted section. Selection Sort selects the smallest element from the unsorted section and places it at the beginning of the sorted section. Quick Sort is a divide-and-conquer algorithm that partitions the data into smaller sub-arrays and sorts them recursively. Merge Sort also employs the divide-and-conquer method by dividing the data into smaller sub-arrays, sorting them, and merging them back into a single array.
Searching Algorithms
Searching algorithms are used to find specific data within a larger set of data. Common searching algorithms include Linear Search, Binary Search, Depth-First Search (DFS), and Breadth-First Search (BFS). Linear Search scans each element of the array until it finds the target element. Binary Search works by dividing the array into two halves and checking whether the target element is in the left or right half. DFS and BFS are used to search for data in graphs. DFS explores as far as possible along each branch before backtracking, while BFS explores all the neighboring nodes of the current node before moving on to the next level.
Graph Algorithms
Graph algorithms are used to find paths and routes in graphs. Popular graph algorithms include Dijkstra’s Algorithm, Prim’s Algorithm, and Kruskal’s Algorithm. Dijkstra’s Algorithm is used to find the shortest path between two nodes in a graph. Prim’s Algorithm is used to find the minimum spanning tree (MST) of a weighted undirected graph. Kruskal’s Algorithm is also used to find the MST of a graph, but it works by sorting the edges of the graph by weight and adding them to the MST in ascending order.
Dynamic Programming Algorithms
Dynamic programming algorithms are used to solve optimization problems by dividing them into smaller sub-problems. Examples include the Knapsack Problem, Traveling Salesman Problem, and Longest Common Subsequence Problem. The Knapsack Problem involves selecting items from a set to maximize the value of the selected items without exceeding a given weight limit. The Traveling Salesman Problem involves finding the shortest possible route that visits every given city exactly once. The Longest Common Subsequence Problem involves finding the longest subsequence that is common to two given sequences.
String Algorithms
String algorithms are used for operations that involve manipulation of strings. The most commonly used string algorithms include the KMP Algorithm, Boyer Moore Algorithm, and Rabin-Karp Algorithm. The KMP Algorithm is used to find the occurrence of a pattern in a string. The Boyer Moore Algorithm is used to find the position of a pattern in a text. The Rabin-Karp Algorithm uses hashing to find the occurrence of a pattern in a string.
Divide and Conquer Algorithms
Divide and Conquer algorithms involve dividing the problem into smaller sub-problems and solving them recursively. Binary Search, Merge Sort, and Quick Sort are examples of Divide and Conquer algorithms.
Greedy Algorithms
Greedy algorithms are used to make the best decision at each step of the algorithm. Examples include Dijkstra’s Algorithm, Kruskal’s Algorithm, and the Job Scheduling Problem. The Job Scheduling Problem involves finding the best schedule for a set of tasks with different processing times and deadlines.
Backtracking Algorithms
Backtracking algorithms are used to find all or some solutions for a problem by exploring all possible states. Examples include the N-Queens Problem, Sudoku Solver, and 0/1 Knapsack Problem.
Brute Force Algorithms
Brute Force algorithms involve trying all possible solutions to a problem. Examples include Subsequence Problem, Subset Problem, and Traveling Salesman Problem.
Randomized Algorithms
Randomized algorithms use a random number generator to solve problems. Examples include Quick Sort, Randomized Selection, and Monte Carlo Algorithm.
Conclusion
In conclusion, different types of algorithms solve specific problems and are widely used in computer science and software development. Understanding these algorithms is crucial for solving complex real-world problems. With this knowledge, developers can build more efficient and effective software systems.
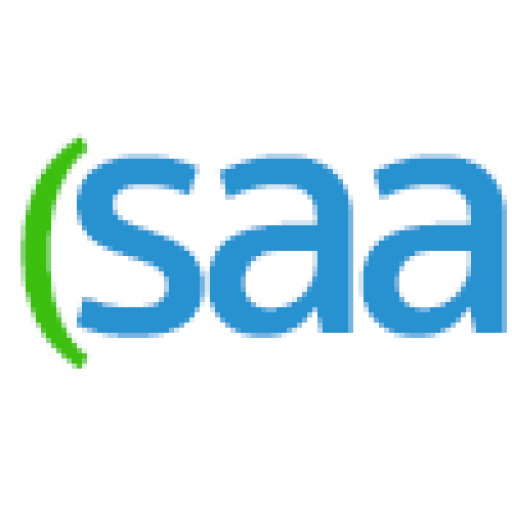
Sorting Algorithm Quick Guide
Sorting Algorithm Average Time Complexity Worst Case Time Complexity Space Complexity Stability Description Bubble Sort O(n^2) O(n^2) O(1) Stable Simple and easy to implement. Used on small data sets or as a simple teaching tool. Selection Sort O(n^2) O(n^2) O(1) Unstable Simple and easy to implement. Used on small data sets or as a simple […]
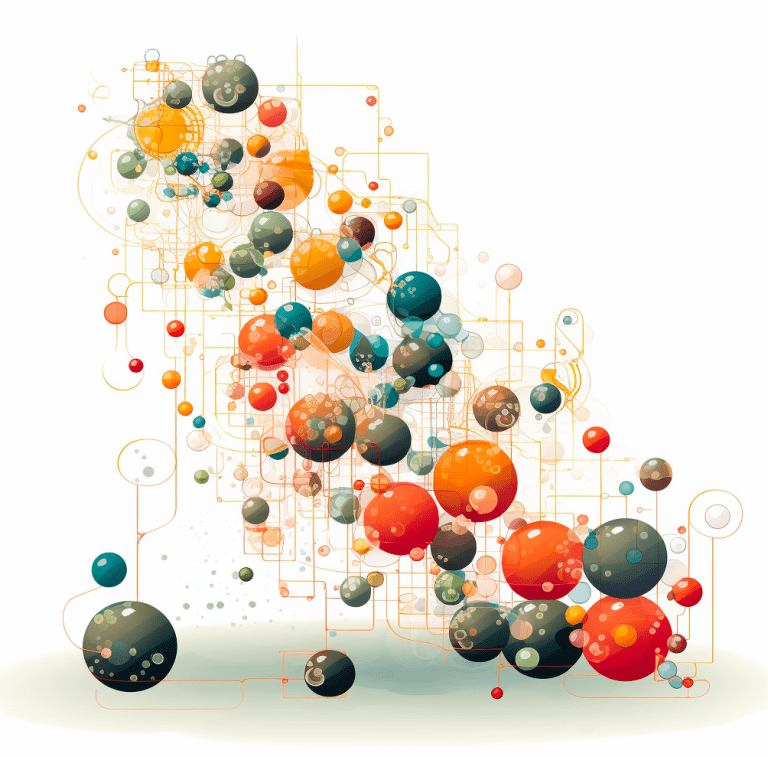
How To Write Bubble Sort: In Typescript
Introduction Bubble sort is a popular sorting algorithm that is commonly used in computer science, and has been around for many years. The algorithm sorts an array of elements by continuously swapping neighboring elements until the array is sorted. In this article, we will be discussing how to implement bubble sort in Typescript, which is […]
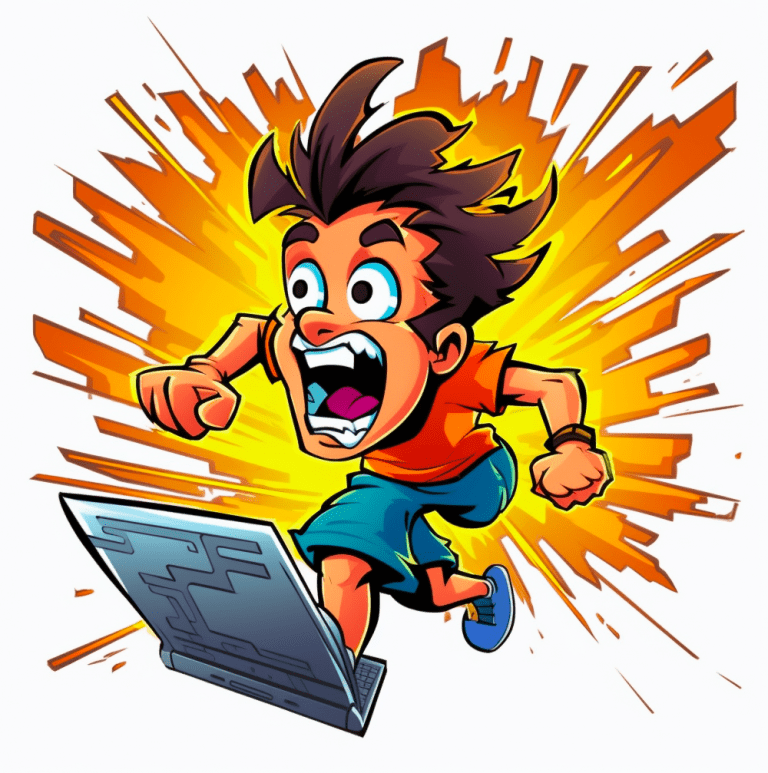
How To Write Quicksort: In Typescript
Introduction: When we talk about sorting algorithms, Quick Sort is one of the most widely used ones. It is a fast, efficient, and in-place algorithm. Typescript on the other hand, is a superset of Javascript which adds static typing, class-based object-oriented programming, and improved syntax to the language. It has gained popularity among developers due […]