Introduction
React Hook Form is a popular tool used in building forms in React.js. It is a performant library that simplifies the process of building forms by reducing boilerplate code and providing convenient features. This article is aimed at introducing the basic concepts of React Hook Form and how to use it to create forms in React.js.
Understanding React Hook Form
Before we dive into the details of how to use React Hook Form, it is important to understand what it is and how it works. React Hook Form is a powerful library that helps developers build forms in a more organized and efficient way. The library works by utilizing React’s hooks, which are special functions that are used to add state and other React functionalities to functional components.
Installation
To use React Hook Form in a project, it is important to install it first. React Hook Form can be installed via node package manager (npm) or yarn. To install it via npm, run the following command in the terminal:
npm install react-hook-form
After installation, you can then import the library into any React component where you want to use it.
Basic Form Setup
Creating a simple form in React Hook Form goes through four main steps:
- Create a form component
- Define form fields
- Implement form validation
- Send form data
Let’s take these steps one after the other.
Creating a Form Component
To create a form component, use the useForm hook provided by React Hook Form to initialize the form object. This object includes various properties and methods that are used to manage the form’s state:
import React from 'react';
import { useForm } from 'react-hook-form';
const MyFormComponent = () => {
const { register, handleSubmit } = useForm();
return (
<form onSubmit={handleSubmit((data) => console.log(data))}>
{/* form fields */}
</form>
);
};
export default MyFormComponent;
In the above example, the useForm hook initializes two variables, register and handleSubmit, which are used in the form component. register is a function used to register an input in the form while handleSubmit is a function that is triggered when the form is submitted. The form fields will be defined in the next step.
Defining Form Fields
The easiest way to define form fields in React Hook Form is to use the register function. This function is used to connect an input element to the form object, which in turn sets up the input element with the necessary form attributes. For instance, to define an email input field, register is called as shown below:
<label>
Email
<input type="email" {...register('email')} />
</label>
Note that the register function is passed an argument with the name of the input field. This name is used to identify the input field in the form object. The spread operator in the input element means that all other input attributes will also be passed to the element.
Implementing Form Validation
Form validation is necessary to ensure that users enter data in the required format. React Hook Form provides an easy way to implement validation.
First, the rules for validation are defined using the yup library, like so:
import * as yup from 'yup';
const schema = yup.object().shape({
email: yup.string().required().email(),
password: yup.string().required().min(8),
});
Here, the schema variable holds the validation rules for the email and password fields. In this example, the email field is set as required and must be in valid email format, while the password field is set as required and must have a minimum of eight characters.
To implement the validation, the register function is called with an object of validation rules, like so:
<input type="email" {...register('email', { required: true, pattern: /^\S+@\S+$/i })} />
Here, the email input field is given two validation rules, which were earlier defined using yup. “Required” makes sure the user enters a value into the input field, while “pattern” checks if the text entered matches the given regular expression.
When the form is submitted, React Hook Form automatically validates the form according to the specified rules. If the validation fails, it will display an error message indicating what went wrong.
Sending Form Data
After the form is validated, the form data can then be sent to the server. This is done using the handleSubmit function, which triggers a function passed to it as an argument whenever the form is submitted. In the example below, the form data is logged to the console when the form is submitted:
<form onSubmit={handleSubmit((data) => console.log(data))}>
Advanced Usage of React Hook Form
Now that we have seen how to create a simple form using React Hook Form, let’s look at some more advanced concepts.
Custom Form Validation
Sometimes, the built-in validation rules might not be enough for complex form validation. In this case, we can make use of the validate function provided by React Hook Form. This function is used to create custom validation rules for inputs in the form object.
Consider the following example:
const validatePhoneNumber = (value) => {
const phoneRegex = /^\+\d{1,3}\s\d{8,}$/;
if (!phoneRegex.test(value)) {
return "This is not a valid phone number";
}
};
const { register, handleSubmit, formState: { errors } } = useForm();
<input
type="tel"
{...register("phonenumber", { validate: validatePhoneNumber })}
/>
{errors.phonenumber && (
<p className="errorMessage">{errors.phonenumber.message}</p>
)}
Here, a custom validation rule is defined to check if the phone number input is correctly formatted. If the rule fails, an error message is shown under the input field.
Accessing Form Data
Sometimes, we might want to access data from the form object on demand. React Hook Form provides an easy way to do this using the getValues function. The function is called with an optional argument, an object containing the names of the inputs we want to extract data for. Here is an example:
import React from 'react';
import { useForm } from 'react-hook-form';
const MyFormComponent = () => {
const { register, handleSubmit, getValues} = useForm();
const logData = () => {
console.log(getValues({ nest: true }));
};
return (
<form onSubmit={handleSubmit((data) => console.log(data))}>
<input type="text" {...register("firstName")} />
<input type="text" {...register("lastName")} />
<input type="submit" value="Submit" onClick={logData} />
</form>
);
};
export default MyFormComponent;
In the example above, when the user clicks the Submit button, the getValues function is called with the “nest” option passed as true. This returns an object containing the values of all the inputs inside the form. The object can then be logged to the console or manipulated however we want.
React Hook Form with Redux
Using React Hook Form along with Redux can be quite powerful. We can use React Hook Form to manage inputs, validations and form submission while Redux manages the state of the whole application.
First, we need to create a Redux store where the form state is managed. Let’s assume the form state has two properties, “values” and “errors”:
import { createStore } from 'redux';
const initialState = {
values: {},
errors: {}
};
function formReducer(state = initialState, action) {
switch (action.type) {
case 'SET_VALUES':
return { ...state, values: action.payload };
case 'SET_ERRORS':
return { ...state, errors: action.payload };
default:
return state;
}
}
const store = createStore(formReducer);
Next, we need to connect the store to React Hook Form using the useReducer hook. Here is an example:
import { useReducer } from 'react';
import { useForm } from 'react-hook-form';
const { register, handleSubmit } = useForm();
const [state, dispatch] = useReducer(formReducer, initialState);
<form onSubmit={handleSubmit((data) => console.log(data))}>
<input type="text" {...register("firstName")} />
<input type="text" {...register("lastName")} />
<button type="submit" onClick={() => submitForm(state, dispatch)}>Submit</button>
</form>
function submitForm(state, dispatch) {
// perform any additional validation here
if (Object.keys(state.errors).length === 0) {
// no errors, dispatch form data to Redux store
dispatch({ type: 'SET_VALUES', payload: state.values });
} else {
// dispatch errors to Redux store
dispatch({ type: 'SET_ERRORS', payload: state.errors });
}
}
Here, we use the useReducer hook to initialize the form state and dispatch actions to the Redux store. When the submit button is clicked, the submitForm function is called which performs additional validation and dispatches data to the Redux store.
Conclusion
React Hook Form is a powerful and efficient way to build form inputs in React. In this article, we have seen how to install, use, and validate forms using React Hook Form. We also saw how to create custom form validation rules, access form data, and use React Hook Form with Redux. With these basics and advanced concepts, you can build robust and efficient forms in React.js.
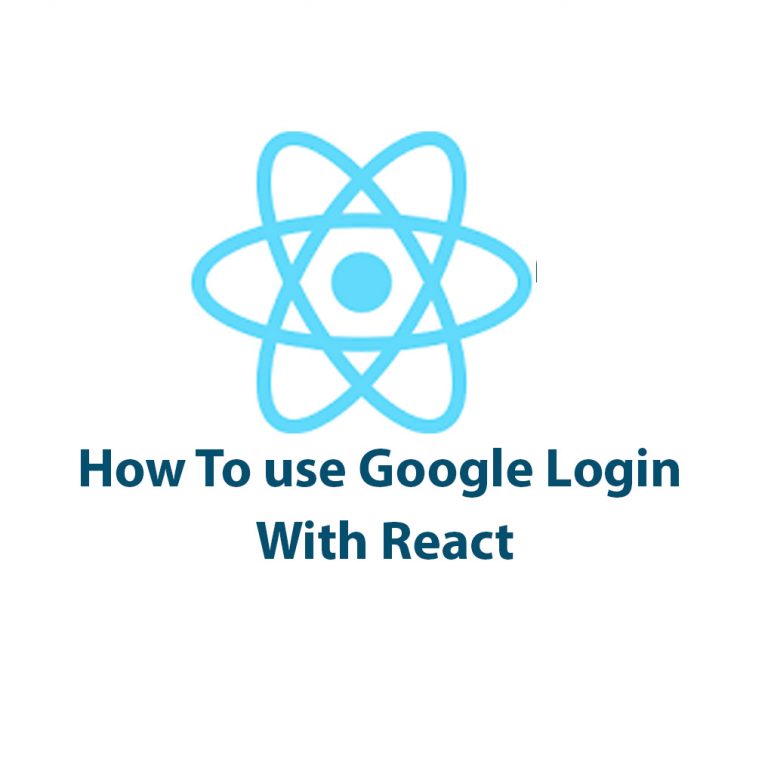
How To Use Google Login With React
As a developer, I cannot stress enough the importance of creating a seamless user experience when it comes to authentication. One of the most popular and convenient ways to allow users to authenticate is by using Google Login. In this article, we’ll explore how to use Google Login with React. Before we delve deep into […]
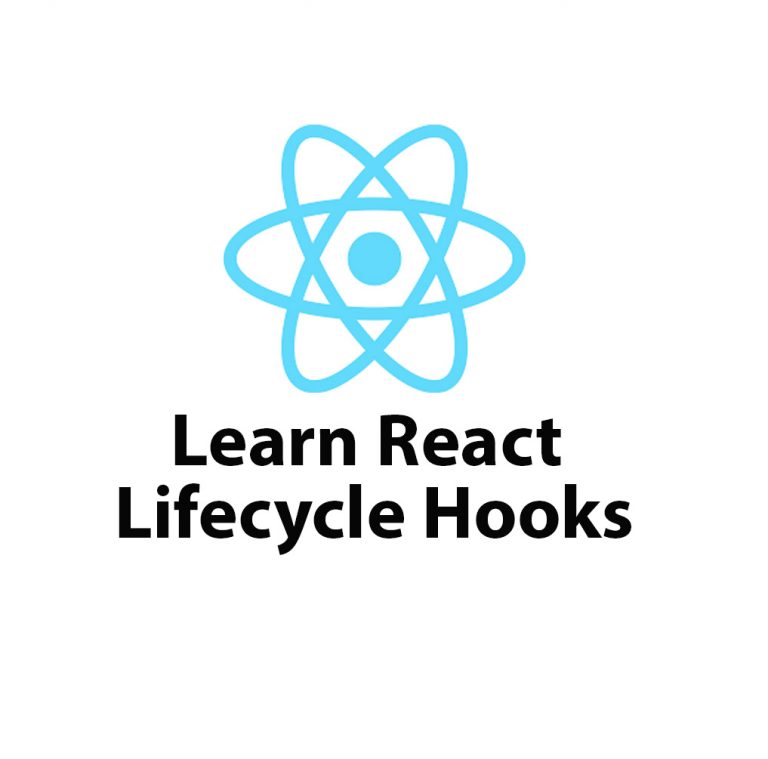
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]
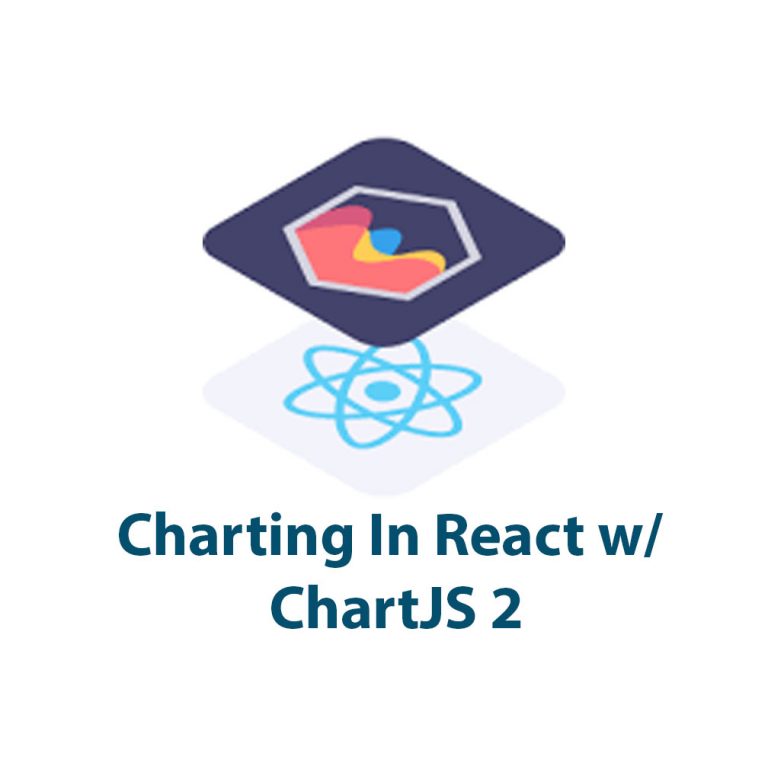
React Charts With ChartJS 2
As a web developer, I understand the importance of data visualization in presenting complex information in an understandable and visually appealing way. That’s why I’ve been using ChartJS 2, a powerful JavaScript library, for creating charts in my React apps. In this article, I’ll show you how to set up and use ChartJS 2 in […]
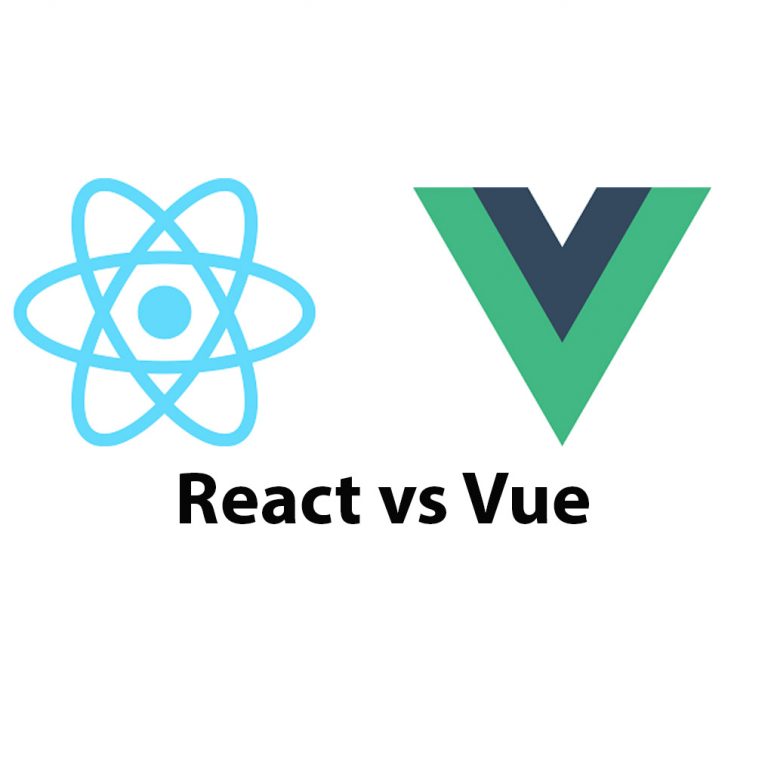
React JS vs Vue: A Comprehensive Guide
Introduction ReactJS and Vue are two of the most popular JavaScript libraries used to develop dynamic user interfaces. These libraries have gained widespread popularity due to their flexibility, efficiency, and ease of use. ReactJS was developed by Facebook, and Vue was created by Evan You. In this article, we are going to evaluate and compare […]
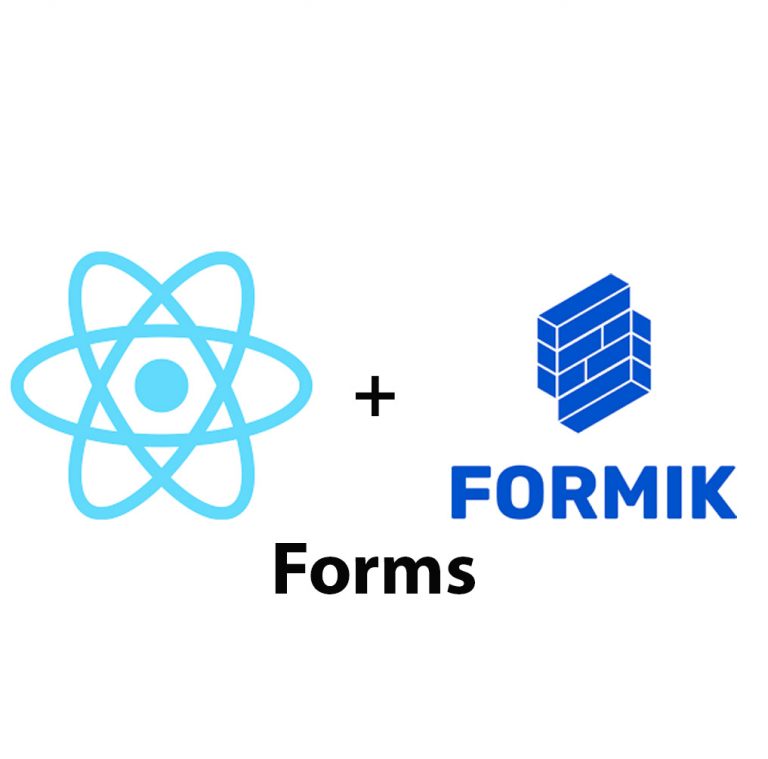
Create Forms In React JS Using Formik
Introduction React JS is a widely used system for building complex and dynamic web applications. It allows developers to work with reusable components in a more efficient way than traditional JavaScript frameworks. One crucial aspect of developing web applications is building forms, which are used to collect user input and send it to a server. […]
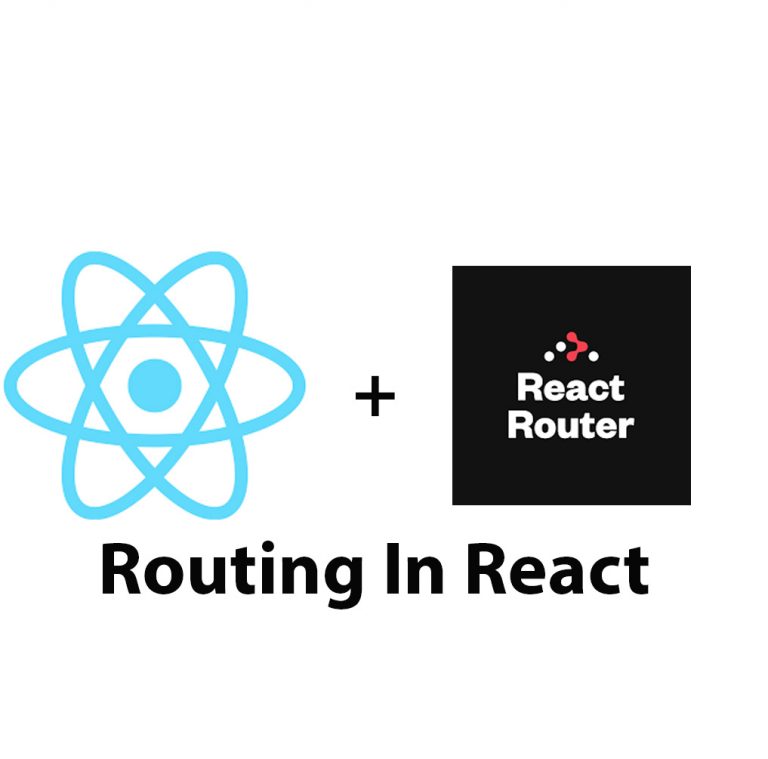
Routing In React JS Using React Router
Introduction React JS has become one of the most popular frontend frameworks for web development. It allows developers to create complex and dynamic user interfaces with ease. Routing is an essential part of web development, and React Router is one such library that is used to manage routing in React applications. In this article, we’ll […]