React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that allow the developers to control such features of a component as initialization, updating, and destruction of a component.
A lifecycle in React JS is like a sequence of events that a component goes through from the point where it is first initialized to the point where it is removed from the UI. Lifecycle hooks in React are methods that are invoked automatically at specific stages of the lifecycle of a component. By using these hooks, a developer can execute his or her customized code, specific to the current lifecycle stage of the component.
Here are the lifecycle phases of a component in React:
1) Mounting phase – During this phase, the component is being created along with its initial state and props. Once it is created, it is rendered onto the DOM. The following lifecycle hooks can be used in the mounting phase
a) constructor – This is invoked when the component is first created. It is used to initialize the state of the component.
b) getDerivedStateFromProps – It is invoked whenever the state or props of the component change, which sets up the initial state of the component.
c) componentDidMount – This is invoked immediately after the component is rendered into the DOM. It is used to make any necessary HTTP requests and set up events.
2) Updating phase – This phase is triggered when the props or state of the component are changed. The following lifecycle hooks can be used in the updating phase.
a) shouldComponentUpdate – This is invoked before rendering the component to the DOM. It is used to compare the current props and state of the component with the new values to check if the component needs to be updated or not.
b) getSnapshotBeforeUpdate – This is invoked immediately before the DOM is updated with the new values. It is used to retrieve information about the current state of the component.
c) componentDidUpdate – This is invoked immediately after the component is updated in the DOM. It is used to make any necessary DOM updates or implement any side effects based on the changes made to the component.
3) Unmounting phase – This phase is triggered when the component is removed from the DOM, either by a parent component or by the user. The following lifecycle hook is used in the unmounting phase.
a) componentWillUnmount – This is invoked immediately before the component is removed from the DOM. It is used to clean up any resources being used by the component, such as timers or event listeners.
Let us now take a closer look at each of these lifecycle hooks in more detail.
Mounting Phase
- Constructor
The constructor is invoked when the component is first created. This is where you can initialize the state of the component, bind methods to the component, and set up any necessary variables. You can use this.props in the constructor since props are being passed to the component’s constructor.
- getDerivedStateFromProps
The getDerivedStateFromProps is invoked every time there is a change in the props or state of the component. This lifecycle method should be used carefully, as it might cause unnecessary rerenders of the component if not used wisely. It should only be used when you want to update the state of the component based on the updated props.
- componentDidMount
The componentDidMount lifecycle method is called immediately after the component is rendered to the DOM. This is where you can make any necessary HTTP requests for data, set up event listeners, or interact with the DOM directly. This is a perfect place to set up animation actions that require DOM nodes to be present. You can also use this method to queue up the data necessary for initial state in props so that you don’t have to make a second call.
Updating Phase
- shouldComponentUpdate
This is called before the component is about to update. The developer can check if the component state has changed using this method and stop the update if not required. The shouldComponentUpdate is a good place to write an optimization code, as a lot of computation can be saved by bypassing unwanted updates.
- getSnapshotBeforeUpdate
The getSnapshotBeforeUpdate lifecycle method is called immediately before React updates the DOM with the new changes. This method provides a way to capture the previous state of the component before it is updated. The return value of this method is passed as a parameter to componentDidUpdate.
- componentDidUpdate
This method is called immediately after the component has been updated in the DOM. This is the perfect place to make any necessary DOM updates or implement any side effects, such as updating the scroll position of the page when new content is added dynamically. This is also where you can add logic to check if a certain updated value requires a specific action, such as browsing history.
Unmounting Phase
- componentWillUnmount
The componentWillUnmount method is called immediately before a component is removed from the DOM. This is where you can clean up any resources that the component used, such as event listeners, timers, or any other global variables. The componentWillUnmount ensures correct manual memory management.
Conclusion
In conclusion, handling lifecycle methods in the right way in React can save a lot of computation and can allow for significant optimization. It is always beneficial to think of lifecycle methods that can be used to improve the performance of the application while simultaneously getting the expected results. These lifecycle hooks are important to have an efficient and clean codebase, and combining them with other great features of React JS will take your application to the next level.
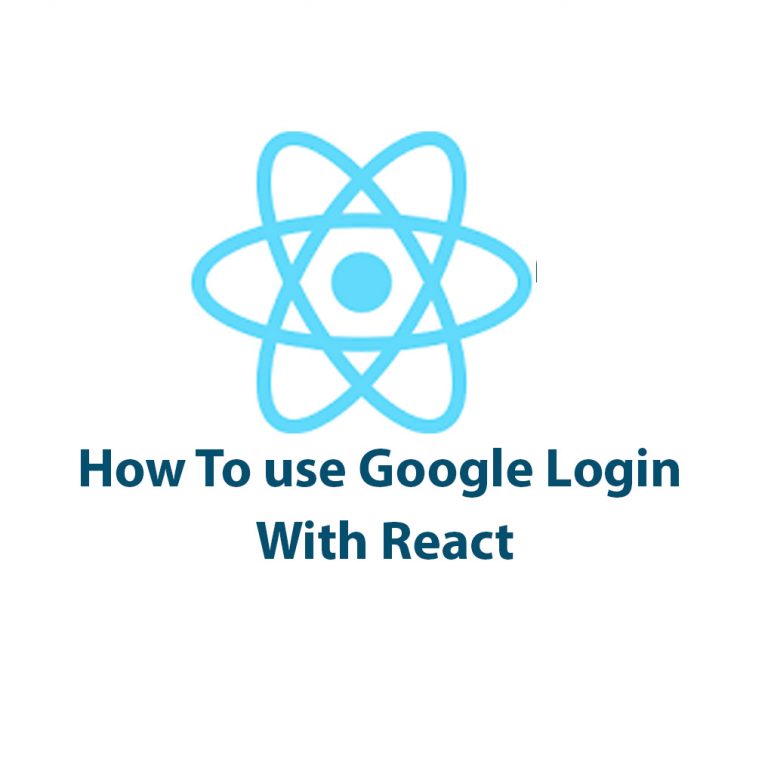
How To Use Google Login With React
As a developer, I cannot stress enough the importance of creating a seamless user experience when it comes to authentication. One of the most popular and convenient ways to allow users to authenticate is by using Google Login. In this article, we’ll explore how to use Google Login with React. Before we delve deep into […]
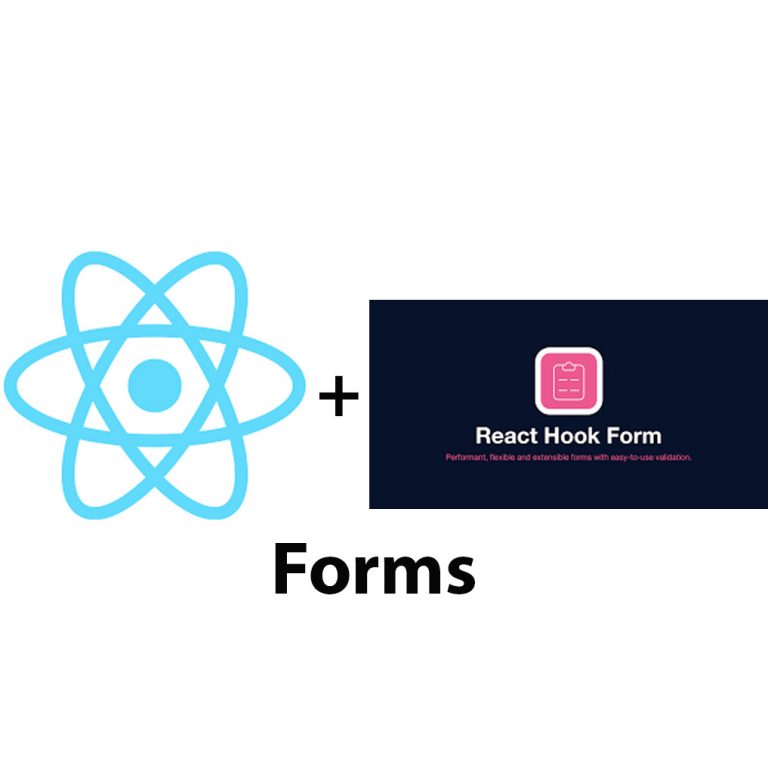
Create Forms In React Using React Hook Form
Introduction React Hook Form is a popular tool used in building forms in React.js. It is a performant library that simplifies the process of building forms by reducing boilerplate code and providing convenient features. This article is aimed at introducing the basic concepts of React Hook Form and how to use it to create forms […]
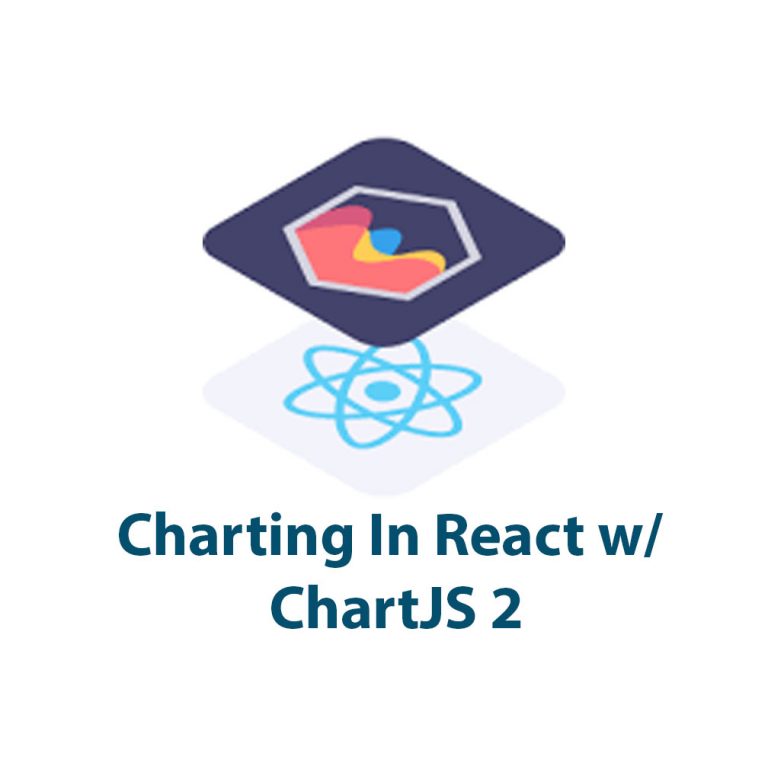
React Charts With ChartJS 2
As a web developer, I understand the importance of data visualization in presenting complex information in an understandable and visually appealing way. That’s why I’ve been using ChartJS 2, a powerful JavaScript library, for creating charts in my React apps. In this article, I’ll show you how to set up and use ChartJS 2 in […]
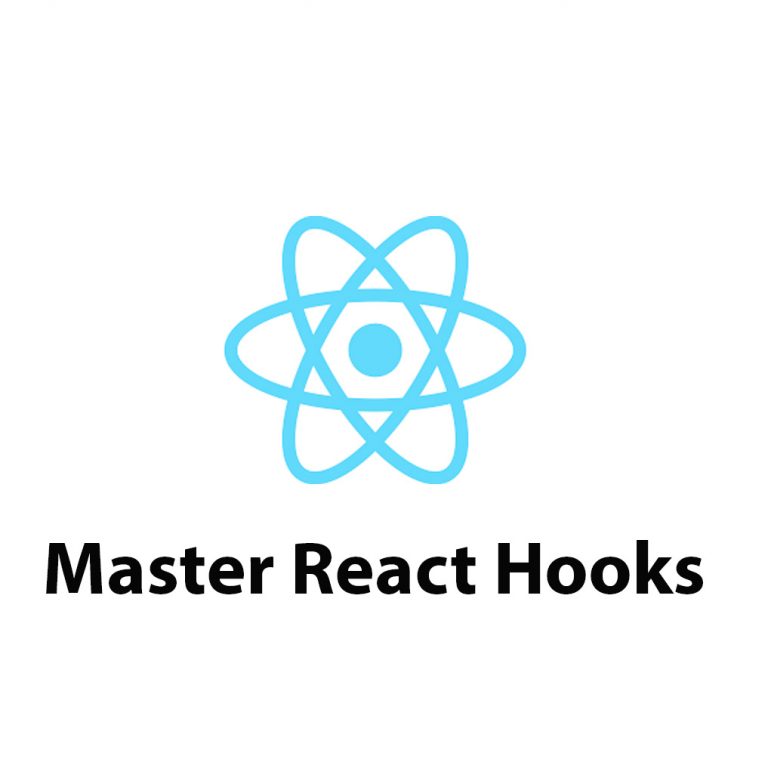
Mastering Hooks In React JS
Introduction React JS is a popular JavaScript library that has gained a lot of popularity since its introduction in 2013. It has been adopted by many web developers who use it to build robust and reliable web applications. With React JS, developers can create user interfaces by building reusable UI components, which can be combined […]
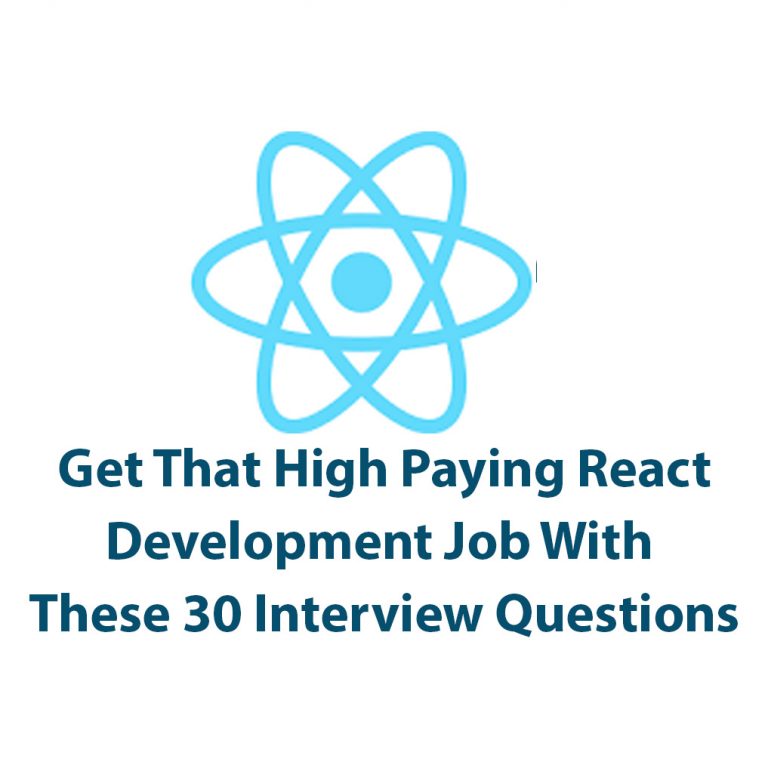
React JS Interview Questions (and answers)
Introduction: React is a powerful JavaScript library with a growing presence in web development today. With its powerful rendering capabilities and cross-functional library, it has become an important skill for developers to learn. In order to prepare for React interviews, it is important to familiarize yourself with React interview questions, which cover a range of […]
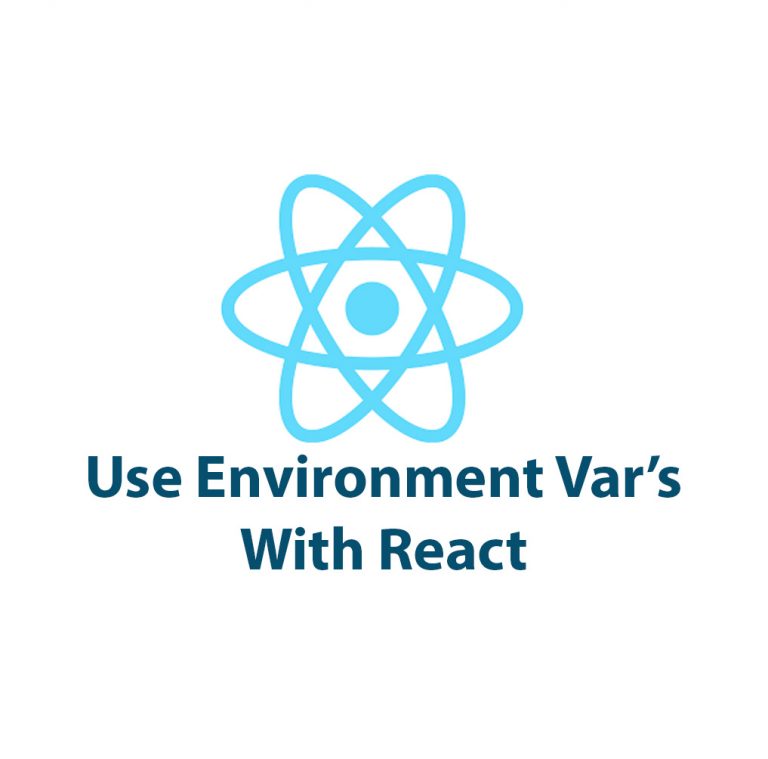
How To Use Environment Variables In React
Introduction Have you ever had to work on a React application and found yourself dealing with different environments or sensitive data? Setting up environment variables can make your life easier and provide a safer way to store information that should not be accessible to the public. In this article, we’re going to look at how […]