Introduction
React JS is a popular JavaScript library that has gained a lot of popularity since its introduction in 2013. It has been adopted by many web developers who use it to build robust and reliable web applications. With React JS, developers can create user interfaces by building reusable UI components, which can be combined to create complex applications.
React JS is known for its declarative approach to building UI components, which makes it easy to create and manage complex user interfaces. However, this does not mean that building React applications can be done without knowledge of the underlying key concepts and techniques. One of the essential concepts in React JS is the idea of “Hooks.”
Hooks are a relatively new addition to React JS and were introduced in version 16.8. They are a set of functions that allow developers to use React state and other features without writing classes in React. This article seeks to provide an in-depth understanding of Hooks in React JS and how to use them to create robust and reliable web applications.
Getting Started with React Hooks
Before we dive into mastering Hooks in React JS, let’s take some time to understand the basic concepts and capabilities of Hooks. Hooks are functions that allow us to use state and other React features in functional components. This means we can now “hook” into the React component lifecycle with functional components.
One of the most popular Hooks in React JS is the useState Hook. The useState Hook allows us to add state to functional components. We can now set and update state without using the setState method or creating a class component. Here’s an example of how to use the useState Hook:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
}
return (
<div>
<h1>{count}</h1>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
In this example, we import the useState Hook from the React library and use it to declare a state variable called “count.” We initialize the count to zero, and we also declare a function called “setCount” that allows us to update the count state. Finally, we render the count variable in a heading and create a button that calls the “increment” function when clicked.
Understanding useState Hook
The useState Hook is a function that returns an array with two values: the current state and a function that updates the state. Here’s how it works:
const [state, setState] = useState(initialState);
The first argument to the useState function is the initial state of the component. In the previous example, we initialized the count to zero. useState then returns an array with two values: the current state (count) and a function that allows us to update the state (setCount).
When we call the setCount function, React will re-render the component and update the count based on the new value. This is how Hooks allow us to manage state in functional components without the need to use classes.
Usage of useState
The useState Hook can be used in many ways. For example, it can be used to create a simple form that takes user input and stores it in state. Here’s an example:
import React, { useState } from 'react';
const Form = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
console.log(`Username: ${username}, Password: ${password}`);
}
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input type="text" value={username} onChange={(e) => setUsername(e.target.value)} />
</label>
<label>
Password:
<input type="password" value={password} onChange={(e) => setPassword(e.target.value)} />
</label>
<button type="submit">Submit</button>
</form>
);
}
export default Form;
In this example, we use the useState Hook to declare two states: “username” and “password.” We then render a form with the two inputs, which are set to their respective states using the value attribute. We also add event handlers for the onChange events of the inputs to capture the user input and update the corresponding state.
Finally, we add a handleSubmit function to log the user input when the submit button is clicked. This example shows how easy it is to manage state with Hooks and how it can be used to create interactive forms.
Understanding useEffect Hook
Hooks also provide another useful Hook called useEffect. This Hook allows us to manage side effects in functional components. Side effects include things like fetching data from an API, setting up event listeners, updating the DOM, and more.
Here’s an example of how to use the useEffect Hook to fetch data from an API:
import React, { useState, useEffect } from 'react';
const DataList = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch("https://jsonplaceholder.typicode.com/posts")
.then(response => response.json())
.then(json => setData(json));
}, []);
return (
<ul>
{data.map(item => <li key={item.id}>{item.title}</li>)}
</ul>
);
}
export default DataList;
In this example, we use the useState Hook to declare a state called “data.” We then use the useEffect Hook to fetch data from the API and set the state of the component. The useEffect Hook also takes a second argument, which is an array of dependencies. We pass an empty array to the dependencies array to ensure that the effect runs only once.
Finally, we render the “data” state as a list of items using the map method. This example shows how Hooks can be used to manage side effects in functional components and how to use the useEffect Hook to fetch data from an API.
Advanced Hooks
Hooks are not limited to useState and useEffect. React JS provides several other Hooks, including:
- useContext Hook: This Hook allows us to consume a context within a functional component. Context provides a way to pass data through the component tree without having to pass props down manually at every level.
- useReducer Hook: This Hook allows us to manage complex state and state transitions in a functional component. It’s similar to the Redux pattern, where state is managed outside the component.
- useRef Hook: This Hook allows us to access the DOM node of a component – useful in managing focus on input fields or scrolling to a particular element.
These Hooks can help make our code more efficient and functional. They can also lead to improved code readability and easier to manage codebase.
Understanding The Lesser Used But Powerful Hooks
Understanding the useCallback hook
The useCallback hook is a useful tool in the React library that is used to create a memoized callback function. This function is meant to be optimized for performance, meaning that it can help prevent unnecessary rerenders and improve the overall efficiency of your application.
To understand how the useCallback hook works, it is important to first understand what a callback function is. In React, a callback function is a function that is passed as a prop to a child component. This function is then called by the child component and can be used to update state or perform other actions.
For example, imagine you have a parent component that has a child component that displays a list of items. When the user clicks on an item in the list, you want to update the state of the parent component to show more details about the clicked item. To do this, you would pass a callback function as a prop to the child component that is called when an item is clicked.
Here is an example of what this might look like:
import React, { useState } from 'react';
import ItemList from './ItemList';
function ParentComponent() {
const [selectedItem, setSelectedItem] = useState(null);
const handleItemClick = (item) => {
setSelectedItem(item);
}
return (
<div>
<h2>Parent Component</h2>
<ItemList onItemClick={handleItemClick} />
{selectedItem && <div>{`Selected Item: ${selectedItem}`}</div>}
</div>
);
}
In this example, the ParentComponent has a state variable called selectedItem that starts off as null. It also has a callback function called handleItemClick that updates the value of selectedItem when an item is clicked. The ItemList component is a child component that displays a list of items and calls the onItemClick function when an item is clicked.
Now, let’s say that the ItemList component was rendering a large number of items (say, hundreds or even thousands). Every time the user clicked on an item, the handleItemClick function would be called, and the selectedItem state would be updated. However, because handleItemClick is a new function every time ParentComponent is rerendered, React would have to rerender all of the child components as well, even if they didn’t need to be updated.
This is where the useCallback hook comes in. By using useCallback to memoize the handleItemClick function, we can tell React to only create a new version of the function if its dependencies (in this case, the setSelectedItem function and the selectedItem state) have changed. This can help prevent unnecessary rerenders and improve performance.
Here is an example of how to use useCallback to memoize the handleItemClick function:
import React, { useState, useCallback } from 'react';
import ItemList from './ItemList';
function ParentComponent() {
const [selectedItem, setSelectedItem] = useState(null);
const handleItemClick = useCallback((item) => {
setSelectedItem(item);
}, [setSelectedItem]);
return (
<div>
<h2>Parent Component</h2>
<ItemList onItemClick={handleItemClick} />
{selectedItem && <div>{`Selected Item: ${selectedItem}`}</div>}
</div>
);
}
In this example, we wrap the handleItemClick function in the useCallback hook. We pass an array of dependencies as the second argument to useCallback. In this case, the only dependency is the setSelectedItem function. This means that React will only create a new version of handleItemClick if the setSelectedItem function changes.
By using useCallback in this way, we can help improve the performance of our application by preventing unnecessary rerenders. It is important to note that useCallback should only be used when you have a legitimate performance concern and when you know that the function being memoized will be called frequently. If you are unsure, it is typically better to avoid using useCallback and allow React to handle updates as it normally would.
Understanding the useMemo hook
One of the latest features that make React stand out is the useMemo hook. It’s one of the utility hooks that can be used in functional components. This hook is designed to optimize performance by caching the results of functions and computations and returning them based on dependencies. This way, the application will not recompute the results if the dependencies do not change.
The useMemo hook is used to memoize a value that is generated from a method or function. Memoization is a technique that is used to cache the results of a computation. When the same computation is required again, the cached result is returned instead of computing it again. Memoization is used to optimize the performance of an application by reducing the amount of work that needs to be done.
The useMemo hook comes in handy when you are dealing with data that may change frequently. When you call a function repeatedly with the same arguments, it can take a lot of resources to calculate. With the useMemo hook, the calculation is only done when the data has changed, which saves system resources and improves the performance of the application.
The syntax of the useMemo hook is simple. It takes two arguments: a callback function and an array of dependencies. The callback function contains the code that calculates the memoized value. The dependencies are the variables that will trigger a re-calculation when they change. If the dependencies are empty, the memoized value will be calculated only once.
const memoizedValue = useMemo(() => {
// code to generate memoized value
}, [dependency1, dependency2])
In the example above, the useMemo hook is used to memoize the value generated by the function that is passed as the first argument. The second argument is an array of dependencies, and the memoized value will only be recalculated if any of these dependencies change.
The useMemo hook is used in situations where expensive computations are required to generate a value. Instead of running the calculation every time a component is rendered, the useMemo hook ensures that the value is cached and only recomputed when the dependencies change. This can significantly improve the performance of an application.
One common use case for the useMemo hook is in sorting or filtering data. Sorting and filtering can be resource-intensive, especially when dealing with large datasets. The useMemo hook can be used to memoize the sorted or filtered data, which reduces the amount of work required to generate the data.
For example, consider a component that displays a table of data that can be sorted by clicking on the table header. In this case, the sorted data can be memoized using the useMemo hook as follows:
const sortedData = useMemo(() => {
if (sortField && sortOrder) {
// sort the data using the specified field and order
return data.sort((a, b) => {
if (sortOrder === 'asc') {
return a[sortField] > b[sortField] ? 1 : -1
} else {
return a[sortField] < b[sortField] ? 1 : -1
}
})
} else {
// return the data unsorted
return data
}
}, [data, sortField, sortOrder])
In this example, the sortedData is memoized using the useMemo hook. The callback function sorts the data based on the specified sortField and sortOrder. The dependencies are the data, sortField, and sortOrder. The sorted data will only be computed when these dependencies change.
Another use case for the useMemo hook is in optimizing rendering performance. There are situations where a component may need to render a large number of child components. This can be resource-intensive and can lead to a slow rendering experience. The useMemo hook can be used to memoize the child components, which reduces the amount of work required to render them.
For example, consider a components that displays a list of items. Each item has a complex child component that takes a long time to render. In this case, the child component can be memoized using the useMemo hook as follows:
const itemList = useMemo(() => {
return items.map((item) => {
return (
<Item key={item.id}>
{useMemo(() => (
<ComplexChildComponent data={item.data} />
), [item.data])}
</Item>
)
})
}, [items])
In this example, the itemList is memoized using the useMemo hook. The callback function maps the items to a list of JSX elements, each containing a memoized child component. The dependency of the child component is the item data. The child component will only be re-rendered when the item data changes.
In conclusion, the useMemo hook is a powerful tool that can be used to optimize React applications. It can be used to memoize expensive calculations and improve rendering performance. When used correctly, the useMemo hook can significantly improve the performance of an application and make it more responsive to user interactions.
Understanding the useImperativeHandle hook
One of React’s greatest strengths is its ability to simplify the management of stateful components. However, when it comes to managing component behavior, things can become a little more challenging. That’s where the useImperativeHandle
hook comes in.
The useImperativeHandle
hook is an advanced React feature that allows developers to expose specific functions of a child component to its parent. This can be particularly useful when you need to manage component behavior such as scrolling, animations, or form submissions, and you don’t want to rely on prop changes or callbacks.
The useImperativeHandle
hook enables developers to define custom methods that can be called outside of the component. You can think of it as a way to create an API for your child component that you can interact with from the parent component.
Here is an example – you may have a child component that renders a list of items. You could use the useImperativeHandle
hook to create a method on the child component that allows the parent component to scroll the list to a particular item. By doing so, you can abstract the scrolling functionality into the child component and make it reusable across different parent components.
This hook is particularly useful when building reusable component libraries, where you may not know how the component will be used. By exposing a simple API, you can empower developers to use your components in ways that suit their specific needs.
The useImperativeHandle
hook is also useful when dealing with legacy code or integrating third-party components. Sometimes it’s necessary to interact with a child component’s methods directly, and this hook offers a way to do just that.
Before diving into how to use the useImperativeHandle
hook, let’s take a closer look at the useRef
hook and why it’s a required dependency.
The useRef
Hook in React
The useRef
hook is another powerful feature of React that’s often used in conjunction with the useImperativeHandle
hook.
In React, components can be declared as either functional or class-based. With functional components, you don’t have the ability to store state values using this.state
. Instead, you use the useState
hook to manage state, but this only provides access to the latest value of state.
If we want to store a mutable value that persists between renders, we use the useRef
hook. The useRef
hook creates a mutable reference to an object and persists it throughout the component’s lifetime.
One important use of the useRef
hook is to store references to HTML elements. This enables you to interact with those elements in your component.
For instance, if you have a form component that you want to submit using JavaScript instead of the default behavior, you can store a reference to the form element using useRef
. You can then call the .submit()
method on the form element, triggering the submit event.
The useRef
hook can also be used with the useEffect
hook to achieve the same effect as the old componentDidMount
lifecycle method. You can store a reference to the component’s mounted state and modify it using useEffect
without triggering a re-render.
Now that we know a bit more about the useRef
hook let’s look at how it works with the useImperativeHandle
hook to create a child component API.
Using the useImperativeHandle
Hook in React
The useImperativeHandle
hook is defined in the React library and included in the react
package. To use this hook in your code, you need to first import it at the top of your file:
import { useRef, useImperativeHandle } from 'react';
Next, you can define a functional component that takes a ref as a parameter and creates a mutable reference to it using the useRef
hook:
function ChildComponent(props, ref) {
const childRef = useRef(null);
useImperativeHandle(ref, () => ({
// Define methods to expose to parent component
}));
return <div ref={childRef}>Child component</div>;
}
In this code snippet, we pass in a reference object as a second parameter to the ChildComponent
function. We then assign this to a mutable reference using the useRef
hook. This assigns a unique value to childRef
, which can be accessed from the parent component.
The useImperativeHandle
hook is then called and takes two arguments. The first is the ref, and the second is a function that returns an object containing the methods you want to expose to the parent component.
Let’s say we want to create a method that allows the parent component to scroll the child component to a specific position. We can do this by adding a method called scrollToPosition
to the object returned by useImperativeHandle
:
function ChildComponent(props, ref) {
const childRef = useRef(null);
useImperativeHandle(ref, () => ({
scrollToPosition: (position) => {
childRef.current.scrollTo(0, position);
},
}));
return <div ref={childRef}>Child component</div>;
}
In this example, we’re using the .scrollTo()
method on the childRef.current
object to scroll the child component to a specific position on the y-axis.
Now that we’ve defined a method on the child component, we can access it from the parent component by using the useRef
hook and passing it to the ChildComponent
function:
function ParentComponent() {
const childRef = useRef(null);
const handleButtonClick = () => {
childRef.current.scrollToPosition(500);
};
return (
<>
<button onClick={handleButtonClick}>Scroll down</button>
<ChildComponent ref={childRef} />
</>
);
}
In this example, we create a ref using the useRef
hook and pass it into the ChildComponent
function using the ref
prop. We then define a method called handleButtonClick
that calls the scrollToPosition
method on the childRef.current
object.
Finally, we render the ParentComponent
and the ChildComponent
inside it, passing the childRef
ref object as a prop to ChildComponent
.
Conclusion:
In conclusion, the useImperativeHandle
hook is a powerful tool that allows developers to create specific APIs for child components and expose only the necessary functionality to the parent components. This hook can be extremely useful in situations where you want to manage component behavior and don’t want to rely entirely on props or callbacks. By combining the useImperativeHandle
hook with the useRef
hook, we can easily create reusable components and integrate them with legacy code or third-party libraries effortlessly. With these tools at your disposal, you can create complex and engaging user interfaces that are easily maintainable and performant.
Understanding the useLayoutEffect hook
When working with React, it is common to come across situations where components that you have rendered do not have the intended layout. One common situation where this can occur is when you’re working with a component that relies on the size or position of its parent container.
To address these situations, React provides a hook known as useLayoutEffect. useLayoutEffect is similar to useEffect, but the key difference is that useLayoutEffect runs synchronously, immediately after DOM mutations occur but before the browser has painted these changes. This behavior makes it useful for adjusting the layout of a component based on certain conditions.
In this article, we will explore the key details surrounding useLayoutEffect, including its syntax, use cases, and general best practices.
The Syntax of useLayoutEffect
The syntax for useLayoutEffect is very similar to that of useEffect. After importing the hook from the React library at the beginning of your file, you can use it as follows:
import { useLayoutEffect } from 'react';
function ExampleComponent() {
useLayoutEffect(() => {
// Your logic goes here
}, []);
// Your component JSX goes here
}
Much like useEffect, you provide a callback function as the first argument to useLayoutEffect. This callback function contains the code that you want to run when the component re-renders. However, one key difference from useEffect is the array of dependencies, which is passed in as the second argument.
In both cases, the second argument array informs React regarding which variables your useEffect or useLayoutEffect callback function relies on. By passing an empty array, you are telling the hook to never re-run your callback function if the component is updated or mounted.
When to Use useLayoutEffect
The primary use case for useLayoutEffect is to interact with the DOM and to adjust the layout of a component based on measured sizes or positions. This often needs to be done synchronously before the browser has had a chance to repaint the page.
Here are a few possible scenarios where useLayoutEffect could be useful:
- Making use of third-party libraries like Material-UI or Ant Design that rely on getting the precise size of their container element.
- Rendering a carousel or slider that requires specific dimensions and positioning to work correctly.
- Animating elements that require precise dimensions and positioning to achieve the intended effect.
It’s worth noting that since useLayoutEffect runs synchronously, it can potentially block browser rendering and lead to a slow user experience. Therefore, useLayoutEffect only when necessary and avoid any performance-intensive operations.
Concerns with useLayoutEffect
While useLayoutEffect is useful for interacting with the DOM, you must be mindful about the performance implications of using it. React provides an alternative, useEffect, that runs asynchronously and therefore avoids the potential blocking of rendering.
So why use useLayoutEffect over useEffect? Sometimes you need to make sure that you’ve applied styles or dimensions to the DOM before the browser performs its rendering. In situations where you need precise control over the layout of a component, useLayoutEffect makes the most sense.
It is also important to note that useLayoutEffect is only compatible with server-side rendering through frameworks like Next.js or Gatsby. Server-side rendering relies on the layout to generate an HTML document, so it is crucial that useLayoutEffect works with server-side rendering.
Lastly, it’s important to follow best practices when using useLayoutEffect. You should avoid putting anything other than layout-related code inside of it. Overusing useLayoutEffect can lead to complicated and error-prone code.
Conclusion
useLayoutEffect is just one of many hooks available in the React library. However, it serves an essential role in the creation of robust and dynamic layouts. Understanding when to use useLayoutEffect and when to use useEffect can help you create highly optimized React code that doesn’t slow down the user experience.
In summary, useLayoutEffect is great for when you need to interact with the DOM to adjust the layout of a component before the browser repaints the page. It is also a more performant alternative to useEffect when dealing with layout-related code. By leveraging useLayoutEffect, you can create dynamic and responsive UIs that provide an excellent user experience.
Understanding the useDebugValue hook
React is a popular frontend library for building user interfaces. One of its features is the useDebugValue hook, which helps developers debug their code by exposing additional data during development. The useDebugValue hook is used to provide additional information about custom hooks and can be very useful in finding and fixing errors.
In this section, we will dive into what the useDebugValue hook is used for in React. Specifically, we will cover the following topics:
- What is the useDebugValue hook?
- Why use the useDebugValue hook?
- How to use the useDebugValue hook?
- A use case for the useDebugValue hook
What is the useDebugValue hook?
The useDebugValue hook is a tool provided by React that helps developers debug their code by exposing additional data during development. Specifically, it is used for debugging custom hooks. A custom hook is a function that uses one or more built-in hooks to solve a specific problem.
Hooks in React allow developers to manage state, perform side-effects, and manipulate the DOM in a reusable way. They help break up logic into smaller and more manageable pieces, making it easier to maintain and debug code.
The useDebugValue hook is a way to decorate a custom hook with additional information. When a developer uses the React DevTools to inspect the state of a component, the useDebugValue hook provides additional information about what is happening inside the custom hook. This information can help to quickly identify problems and fix them.
Why use the useDebugValue hook?
There are several reasons why developers might want to use the useDebugValue hook:
- Tracking variables: The useDebugValue hook is useful for tracking the values of variables inside a custom hook. This can be especially helpful when a hook depends on several variables that change frequently.
- Monitoring hook flow: The useDebugValue hook can help developers monitor the flow of hook calls. It provides helpful information about the order in which hooks are called, when they are re-rendered, and what changes are made to their state.
- Debugging logic: By providing additional information about a hook, the useDebugValue hook can help developers debug complex logic. When a developer understands what a hook is doing, they can more easily identify problems and fix them.
Overall, the useDebugValue hook can help developers save time and effort when debugging custom hooks.
How to use the useDebugValue hook?
Using the useDebugValue hook is relatively straightforward. To use the hook, you add it to your custom hook and pass in a value. React then takes care of the rest, providing the additional information to the React DevTools during development.
Here is an example of a custom hook that uses the useDebugValue hook:
import { useState, useEffect, useDebugValue } from 'react';
function useTimer() {
const [seconds, setSeconds] = useState(0);
const [isActive, setIsActive] = useState(false);
useEffect(() => {
let intervalId;
if (isActive) {
intervalId = setInterval(() => {
setSeconds(prevSeconds => prevSeconds + 1);
}, 1000);
}
return () => {
clearInterval(intervalId);
};
}, [isActive]);
useDebugValue({ seconds, isActive }, ({ seconds, isActive }) => `Seconds: ${seconds}, Active: ${isActive}`);
return { seconds, isActive, toggleTimer: () => setIsActive(!isActive) };
}
In this example, the useTimer custom hook tracks the number of seconds that have passed and whether or not the timer is active. It has a toggleTimer function that toggles the isActive state.
The useDebugValue hook is used to provide additional information about the seconds and isActive states. The first argument to the hook is an object containing those states. The second argument is a function that returns a string containing the information to be displayed in the React DevTools.
In this case, the useDebugValue hook returns the “Seconds” and “Active” values in a string format that is easy to read. Developers can then view this information in the React DevTools to identify any issues.
A use case for the useDebugValue hook
One use case for the useDebugValue hook is in tracking variables inside a custom hook. For example, consider a custom hook that fetches data from an API:
import { useState, useEffect, useDebugValue } from 'react';
function useApiData(url) {
const [data, setData] = useState(null);
const [isLoading, setIsLoading] = useState(true);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(json => {
setData(json);
setIsLoading(false);
});
}, [url]);
useDebugValue({ data, isLoading });
return { data, isLoading };
}
In this example, the useApiData custom hook fetches data from an API and tracks the data and loading state. By adding the useDebugValue hook, developers can quickly see the current values of the data and isLoading variables.
Conclusion
The useDebugValue hook is a powerful tool for debugging custom hooks in React. By providing additional information about the state of variables, the order of hook calls, and the logic being used, developers can more easily identify problems and fix them. The useDebugValue hook can save developers time and effort during development, making it a valuable tool in any React project.
Understanding the useCallbackDebugValue hook
React has become one of the most popular front-end libraries for building user interfaces. One of the reasons for its popularity is its ability to create reusable components that make building complex applications much easier. To make these components even more powerful, React introduced hooks in version 16.8.
One of the most widely used hooks is the useCallback hook. This hook is used to memoize functions so that they only re-render if the inputs have changed. However, when it comes to debugging, this hook can be a bit of a pain. This is where the useCallbackDebugValue hook comes in.
The useCallbackDebugValue hook is a relatively new addition to the React library. It is a debugging tool that allows developers to see why a function is being re-rendered. With the useCallbackDebugValue hook, a function can be given an additional “label” that will be displayed in the React DevTools. This label can then be used to determine when the function is being called and why it is being re-rendered.
To use the useCallbackDebugValue hook, you must first import it from the React library. Then you can wrap your function in the hook and give it a label. When the function is called, the label will be displayed in the React DevTools, making it much easier to debug.
Here’s an example of how to use the useCallbackDebugValue hook:
import { useCallback, useCallbackDebugValue } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const handleClick = useCallback(() => {
setCount(count + 1);
}, [count]);
useCallbackDebugValue(handleClick, ['increment']);
return (
<div>
<button onClick={handleClick}>Increment</button>
<p>Count: {count}</p>
</div>
);
};
In this example, we have a simple component that displays a count and a button. When the button is clicked, the count is incremented. We are using the useCallback hook to memoize the handleClick function, so it only re-renders when the count changes.
Now, we have added the useCallbackDebugValue hook on the handleClick function to give it a label of “increment”. This label will be displayed in the React DevTools, making it easier to determine why the function is being re-rendered.
If we open the React DevTools, we can see the label in the function list:
![React DevTools][1]
This label can be used to determine exactly when and why the handleClick function is being called. For example, if we click the button a few times, we can see that the function is only called when the count changes:
![React DevTools][2]
As you can see, the useCallbackDebugValue hook is a powerful debugging tool that can save developers a lot of time when debugging complex React applications. By giving functions descriptive labels, we can easily determine why they are being called and when they are being re-rendered.
It is important to note that this hook should only be used for debugging purposes and should not be included in production code. It is also important to remember that adding too many labels can cause performance issues, so it should be used sparingly.
In conclusion, the useCallbackDebugValue hook is a useful debugging tool that can help developers quickly diagnose and debug problems in their React applications. By giving functions descriptive labels, we can easily determine why they are being called and when they are being re-rendered. If you are working on a complex React project, this hook can be a real lifesaver. However, it is important to remember that it should only be used for debugging purposes and should be used sparingly to avoid performance issues.
Understanding the useTransition hook
The useTransition hook in React is a powerful tool used to manage CSS transitions and animations in high-traffic, dynamic web applications. This hook helps developers smooth out the user interface (UI) by managing the transition between different states of a component.
The useTransition hook is a part of the React library, which is responsible for rendering UI components on the web. This hook is used in combination with the useState hook, a commonly used hook in React for managing component state. Together, these two hooks enable developers to create dynamic and interactive user interfaces.
In essence, the useTransition hook helps manage the transition between two states of a component by setting up a “buffer” period between the two states where nothing is rendered until the transition is complete. This creates a smoother visual effect when one component is being replaced by another on the screen.
When a transition is initiated, React creates a snapshot of the current component state. It then updates the state to match the desired transition state, and sets a “pending” state for the component. This means that for a few milliseconds, nothing is rendered on the screen. Once the transition is complete, the new component state is displayed, and the “pending” state is removed.
The useTransition hook is especially useful in cases where there is a lot of data being loaded from a server or in cases where there are a lot of user interactions that require constant updating of the component state. In such cases, the useTransition hook helps to manage the updates and prevent the UI from becoming unresponsive or “jumpy”.
One of the key benefits of using the useTransition hook is that it allows developers to create more intuitive and engaging user interfaces that feel more natural and polished. For example, the useTransition hook can be used to create a spinner or loading icon that appears when data is being loaded from a server. The spinner can then disappear once the data has been successfully loaded, indicating to the user that the task is complete.
Another benefit of using the useTransition hook is that it can help improve the performance of web applications. By managing the transition between different states of a component, the useTransition hook reduces the amount of time that the browser spends rendering the component. This can lead to faster load times and a smoother browsing experience for users.
Overall, the useTransition hook is a powerful tool that can help developers create more dynamic and engaging user interfaces for their web applications. By managing the transition between different states of a component, the useTransition hook helps to create a smoother and more polished UI, while also improving the performance of the application.
Understanding the useDeferredValue hook
As a front-end developer, you are well familiar with the concept of rendering components in React. In fact, you might be so comfortable with it that you might think there is nothing more to learn. Well, think again. React, as a fast-moving and growth-oriented library, introduces new features and functionalities frequently. One such feature is the useDeferredValue hook.
The useDeferredValue hook is one of the latest additions to React, and it can be utilized to improve your application’s performance. It solves the problem of janky or slow user interfaces when working with large amounts of data, creating smooth and fast interfaces even when dealing with large-scale data.
So, what is the useDeferredValue hook?
The useDeferredValue hook is a hook that controls the rate at which a value can change. It allows developers to delay the update of a value, hence improving application performance. This means that the useDeferredValue hook can be used to update large data sets while ensuring that the user interface remains fast and seamless, without any lag or slowdowns.
The useDeferredValue hook is useful when dealing with a scenario whereby data must be updated frequently. A good example of this is when working with lists or tables. When updating data that is displayed in a table or list, it can take a lot of processing power and resources, which can significantly impact the performance of the application.
In a typical React application, whenever a state value is updated, the component will re-render, even if the data has not changed or if the update is not necessary. The useDeferredValue hook solves this issue by creating a buffer that delays the render, giving the application time to process the update and offering a smoother experience by reducing the number of re-renders that occur.
For example, if you have a list of items that need to be updated frequently, the useDeferredValue hook can be used to ensure that the update process is smooth and does not impact the performance of the application. By setting a delay, you can ensure that the component will only re-render after the data has been updated, ensuring that the application does not become unresponsive while processing the update.
How to use the useDeferredValue hook
To use the useDeferredValue hook, you first need to import it from the ‘react’ library.
import { useDeferredValue } from 'react';
Once imported, you can use the hook in your component by passing the value that you want to defer along with a delay value. The delay value determines the rate at which the value can change, and it is measured in milliseconds. The following code snippet illustrates how to use the useDeferredValue hook.
import { useDeferredValue } from 'react';
const MyComponent = () => {
const [data, setData] = useState([]);
const deferredData = useDeferredValue(data, 100);
const updateData = () => {
const newData = fetchNewData(); // some function that fetches new data
setData(newData);
};
return (
<div>
<button onClick={updateData}>Update Data</button>
<Table data={deferredData} />
</div>
);
};
In the example above, the useDeferredValue hook is used to defer the updating of the ‘data’ state value to 100 milliseconds. This means that whenever the ‘data’ state value changes, the component will not immediately re-render. Instead, the component will wait for 100 milliseconds before re-rendering. This ensures the smooth updating of the user interface, even when dealing with large data sets.
Conclusion
React is an ever-growing and ever-changing library, and the useDeferredValue hook is just one of its latest additions. The useDeferredValue hook can be used to improve the performance of your application when working with large data sets, ensuring a smoother user interface and preventing lag or slowdowns.
By delaying the updates and reducing the number of re-renders, the useDeferredValue hook can help improve the efficiency of your application and offer a better user experience. With this hook, you can ensure that your application can handle large amounts of data without compromising on performance or responsiveness.
Understanding the useTransition hook
The useTransition hook is one of the latest additions to React’s arsenal of hooks, and it is designed to help developers implement smooth transitions in their web applications. In essence, a transition is an animation that occurs when a component changes from one state to another. For example, when a user clicks a button, the component might transition from a default state to a “loading” state while it processes the request. When the processing is complete, it could transition back to the default state again.
Transitions are an important part of enhancing the user experience in web applications. They act as visual cues that help users understand what is happening in the application. Without transitions, users can be left feeling uncertain about the state of the application, which can lead to frustration and confusion.
Using the useTransition hook, developers can implement these transitions with ease. The hook works by allowing developers to specify two different component states: the starting state and the ending state. When the component changes from one state to the other, the hook will animate the transition between the two states, providing a smooth and polished user experience.
The useTransition hook is easy to use and can be added to any functional component in your React application. To use the hook, you simply import it from the React library and call it in your component like any other hook. The hook takes two arguments: the configuration object and the key of the object you are transitioning. Let’s take a closer look at each of these arguments.
The Configuration Object
The configuration object is where you specify the settings for the transition. There are several properties that you can set in this object, including:
- Timeout: This property sets the duration of the transition in milliseconds.
- Delay: This property sets the delay before the transition starts.
- Suspense: This property tells React whether to delay rendering the new state until the transition is complete.
- ChunkSize: This property sets the number of updates that should be batched together before the transition is triggered.
- ExitBeforeEnter: This property determines whether the exiting element should be animated before the entering element.
These properties can be set as follows:
const [state, setState] = useState(false);
const [isActive, setIsActive] = useTransition(() => state, null, {
timeout: 500,
delay: 0,
suspense: false,
chunkSize: 1,
exitBeforeEnter: true,
});
In this example, we are setting the timeout to 500 milliseconds, which means the transition will take half a second to complete. We are not setting any delay, so the transition will start immediately. The suspense property is set to false, meaning that React will not wait to render the new state until the transition is complete. The chunkSize is set to 1, which means that each update will trigger the transition. Finally, we are setting the exitBeforeEnter property to true, meaning that the exiting element will be animated before the entering element.
The Key
The second argument to the useTransition hook is the key of the object you want to transition. This is the property that will trigger the transition when it changes. For example, if you have a button that changes its text from “Submit” to “Processing”, you would set the key to “text” to trigger the transition.
const [state, setState] = useState({text: "Submit"});
const [isActive, setIsActive] = useTransition(() => state.text, "text", {
timeout: 500,
delay: 0,
suspense: false,
chunkSize: 1,
});
In this example, we are setting the key to “text”, which means that the transition will be triggered when the text property of the state object changes. When the user clicks the button, we can update the state like this:
const handleClick = () => {
setState({text: "Processing"});
};
This will trigger the transition, and the component will animate from the “Submit” state to the “Processing” state.
Conclusion
The useTransition hook is a powerful tool for implementing smooth and polished transitions in React applications. With its simple API and powerful configuration options, it’s easy to get started and create engaging user experiences. Whether you’re designing a simple button or a complex form, the useTransition hook can help you take your application to the next level.
Understanding the useSubscription hook
The useSubscription hook is an advanced feature provided by the React team that enables developers to subscribe to a remote data source and automatically update the UI when new data becomes available. It is a powerful tool for building real-time applications and can be used in combination with other libraries like GraphQL or Apollo to create highly dynamic and responsive web interfaces. In this article, we will explore the useSubscription hook and its various applications in detail.
As the name suggests, the useSubscription hook works by establishing a WebSocket connection between the client and server to receive data updates in real-time. This connection is maintained throughout the lifetime of the component, and any data received through the WebSocket is automatically processed and updated on the UI. The useSubscription hook abstracts away much of the complexity associated with WebSocket programming, making it easy for developers to build real-time applications without having to write complex event handlers.
One common use case for the useSubscription hook is building real-time chat applications. In chat applications, messages are being sent and received by different users in real-time, and these messages need to be displayed on the UI as they arrive. With the useSubscription hook, developers can easily establish a WebSocket connection to the chat server and receive new messages as they are being sent. The hook updates the UI with the new message, allowing users to see the conversation as it unfolds. This feature makes the chat application feel much more natural and responsive, improving the overall user experience.
Another use case for the useSubscription hook is building real-time collaboration applications, such as Google Docs. In these applications, multiple users can edit the same document simultaneously, and their changes need to be reflected in real-time on all other users’ screens. With the useSubscription hook, developers can subscribe to the document’s server and receive updates as they come in. The hook ensures that all users’ changes are synced and displayed on the document in real-time, making collaboration much more seamless and effective.
One advantage of using the useSubscription hook over traditional polling or long-polling techniques is that it reduces the load on the server and improves the overall performance of the application. Unlike polling techniques, where the client sends frequent requests to the server to check if new data is available, the useSubscription hook establishes a WebSocket connection that stays open for as long as the client is active. This connection enables the server to push data to the client as soon as it becomes available, reducing the likelihood of delays or missed updates.
Another advantage of the useSubscription hook is that it simplifies the codebase and reduces the amount of boilerplate required for building real-time applications. Before the useSubscription hook, developers would have to write complex WebSocket code that would need to handle edge cases, such as connection timeouts and retries. With the useSubscription hook, much of this complexity is abstracted away, allowing developers to focus on building the core application logic.
In addition to these advantages, the useSubscription hook also supports a range of configuration options that make it highly customizable. For example, developers can specify the subscription query, the update handler function, and the onError handler function, allowing them to fine-tune the subscription behavior to suit their specific needs.
In conclusion, the useSubscription hook is a powerful tool for building real-time applications in React. It simplifies the codebase and reduces the amount of boilerplate required for establishing WebSocket connections. The useSubscription hook supports a range of configuration options that make it highly flexible and customizable. It is an essential tool for building real-time applications such as chat, collaboration tools, and other applications that require up-to-date data in real-time. For developers looking to take their real-time applications to the next level, the useSubscription hook is an excellent starting point.
Best Practices for Using Hooks In React JS
To make the most of Hooks in React JS, it is essential to follow some best practices.
- Naming conventions for custom Hooks
When creating custom Hooks, it is important to follow naming conventions to make it easier for other developers to use them. Custom Hooks should be prefixed with “use” to indicate that they are Hooks. For example, “useFetchData” is a good name for a custom Hook that fetches data from an API.
- Testing Hooks
Hooks can be tested the same way we test functions and classes. It is essential to isolate the Hook from other components and test it in isolation. We can use Jest and React Testing Library to test Hooks.
- Avoiding common mistakes when using Hooks
One of the most common mistakes when using Hooks is forgetting to add the dependencies to the useEffect Hook. When the dependencies are not provided, the Hook will be called every time the component is rendered, leading to performance issues. Other common mistakes when using Hooks include:
- Mutating state directly
- Not using the correct ordering of calls to Hooks
- Not using Hooks conditionally
Do Hooks Replace Redux
Hooks and Redux have been two popular choices for managing state in React applications. Both have their benefits and drawbacks, but with the introduction of hooks in React 16.8, many developers have been questioning whether hooks replace Redux.
Before diving into whether hooks replace Redux, it’s important to understand what each of them does. Redux is a state management library that follows a strict unidirectional data flow pattern. It has a centralized store that holds the application state, and components can dispatch actions to update the store. The store then notifies subscribed components of the state change, and they can re-render with the new data.
Hooks, on the other hand, are a new feature introduced in React that let you use state and lifecycle methods in functional components. Prior to hooks, functional components were limited to only rendering UI elements and not managing state, but with hooks, you can use local state, manage side effects, and access lifecycle methods.
So, do hooks replace Redux? The short answer is no, they don’t. While hooks do offer some state management capabilities, they are not a direct replacement for Redux, which provides a more robust and efficient solution for managing complex state in large applications.
However, that’s not to say that hooks don’t have their place in the state management landscape. In fact, they can be an excellent complement to Redux, providing a way to manage local UI-specific state while Redux handles the global app state.
One of the primary benefits of using hooks is the reduced boilerplate and improved readability of functional components. With hooks, you can abstract away the often verbose class component syntax and get straight to the business of rendering UI elements and managing local state.
Additionally, the useState and useEffect hooks allow you to easily manage state and side effects, respectively, without having to rely on external libraries or custom solutions. This can simplify your codebase and reduce the number of dependencies you need to manage.
However, there are some limitations to using hooks for state management, particularly when it comes to larger, more complex applications. Hooks can become unwieldy and hard to manage when you have a large number of components that need to share state, or when you have complex data structures that require more than simple state updates.
This is where Redux shines. With its strict unidirectional data flow and centralized store, Redux provides a clear and efficient way to manage state across a large application. Its reducers let you easily update the store with new data, and the React Redux library provides a seamless way to connect your components to the store.
Furthermore, Redux provides powerful debugging tools and middleware that can help you track and manage your state more effectively. This can be especially helpful when dealing with complex data structures and interactions between components.
Another key benefit of Redux is its ability to handle asynchronous data fetching and updates. While hooks can certainly handle asynchronous operations, it often requires extra coding and can be more error-prone than the tools provided by Redux. Redux Thunk and Redux Saga provide powerful middleware that make it easy to handle complex asynchronous operations in a synchronous way.
Of course, there are also drawbacks to using Redux. Its strict unidirectional data flow can be difficult to understand and implement correctly, especially for those new to the library. Additionally, Redux can be seen as heavyweight and overkill for smaller applications, where hooks might provide a simpler and more elegant solution.
Ultimately, the question of whether hooks replace Redux depends on the specific needs of your application. For smaller, simpler applications, hooks may provide an efficient and elegant solution for managing UI-specific state. For larger, more complex applications, Redux provides a robust and scalable approach to state management that can handle a wide range of use cases.
However, even in larger applications, hooks can still play a valuable role. They can be used to manage local UI state, simplify code, and improve readability. And with the ability to use hooks alongside Redux, you can have the best of both worlds, leveraging the benefits of both solutions to create a more efficient and maintainable application.
In conclusion, while hooks and Redux are not direct replacements for each other, they can be used together to create powerful, scalable, and efficient applications. Understanding the strengths and limitations of both solutions can help you make informed decisions about how to manage state in your own React projects.
React Hooks vs Classes
Hooks and classes are two different ways to create components in React, and each has its own benefits and drawbacks. In this article, we will explore the difference between hooks and classes in React so that you can make an informed decision on which one is right for you.
What are React Hooks?
React Hooks is a feature that was introduced in React version 16.8. It is a set of functions that allow you to use state and other React features without having to write a class. This is beneficial because it makes React code more concise and easy to read.
The two most commonly used hooks are useState and useEffect. useState allows you to store a piece of state in a function component, while useEffect allows you to perform side effects like fetching data, subscribing to events, or manipulating the DOM.
One of the biggest advantages of using React Hooks is that it allows you to write reusable code in a functional programming style. By separating state and side effects into separate hooks, you can compose your components in different ways without having to rewrite the same code over and over again.
Another advantage of using hooks is that they are easier to test than class components. Since hooks are just functions, you can easily test them using a library like Jest or Enzyme. This makes it easier to write robust and reliable code.
What are React Class Components?
React Class Components are the traditional way of creating components in React. They are JavaScript classes that extend the React.Component class and have a render method that returns a JSX template.
Class components use this.state to store state and lifecycle methods like componentDidMount and componentDidUpdate to perform side effects. They provide a familiar and intuitive pattern for managing complex state and behavior.
One of the advantages of using class components is that they have access to some more advanced features of React, such as error boundaries and static methods. These features are not available to functional components by default, and therefore can be useful in more complex applications.
Another advantage of using class components is that there is a wealth of documentation and examples available for them. Since class components have been around longer than hooks, there are more resources available to help you understand how they work.
What are the Differences Between Hooks and Class Components?
The biggest difference between hooks and class components is the way they manage state and perform side effects.
In class components, state is stored in this.state and can be modified using this.setState. Side effects are managed using lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount.
In functional components that use hooks, state is managed using the useState hook, and side effects are managed using the useEffect hook. These hooks provide a more declarative and concise way of managing state and side effects.
Another difference between hooks and class components is the way they handle context. In class components, you can use the this.context property to access a context provider’s value. In hooks, you can use the useContext hook to access context values.
Hooks also provide a better solution for code reuse. You can use a custom hook to reuse stateful logic without having to write a class or duplicate code. In class components, you would have to use inheritance or higher-order components to achieve the same result.
When to Use Hooks and When to Use Class Components?
As with most things in programming, the decision to use hooks or class components depends on your specific needs and the requirements of your application.
If you are building a simple application with a basic user interface, functional components with hooks may be the best choice. They provide a simple and easy-to-use API, while also being easy to test and debug.
On the other hand, if you are building a complex application with many connected components, class components may be a better choice. They provide a more structured approach to state management and can handle more complex side effects.
In general, hooks are best used for managing simple state and side effects, while class components are best used for managing complex state and behavior.
Conclusion
React Hooks and Class Components are two different ways to create components in React, each with its own benefits and drawbacks. While hooks are newer and more concise, class components provide a more familiar and structured approach to state management.
The decision to use hooks or class components depends on the specific needs of your application. If you are building a simple application with a basic user interface, functional components with hooks may be the best choice. On the other hand, if you are building a complex application with many connected components, class components may be a better choice.
By understanding the differences between hooks and class components, you can make an informed decision on which one to use in your next React project.
Conclusion
Hooks are a powerful addition to React JS and provide a new way to manage state and side effects in functional components. Understanding Hooks can lead to writing more robust, reliable, and scalable React applications.
In this article, we have covered the basics of Hooks in React JS, including the useState and useEffect Hooks, and learned how to use them to write functional components. We have also covered advanced Hooks, including useContext, useReducer, and useRef Hooks. Finally, we have explored some best practices for using Hooks in React JS to write efficient, functional, and readable code.
As you continue to learn more about React JS and Hooks, you’ll discover that Hooks create many opportunities to write maintainable and fault-tolerant codebases. Mastering Hooks will take your React game to the next level.
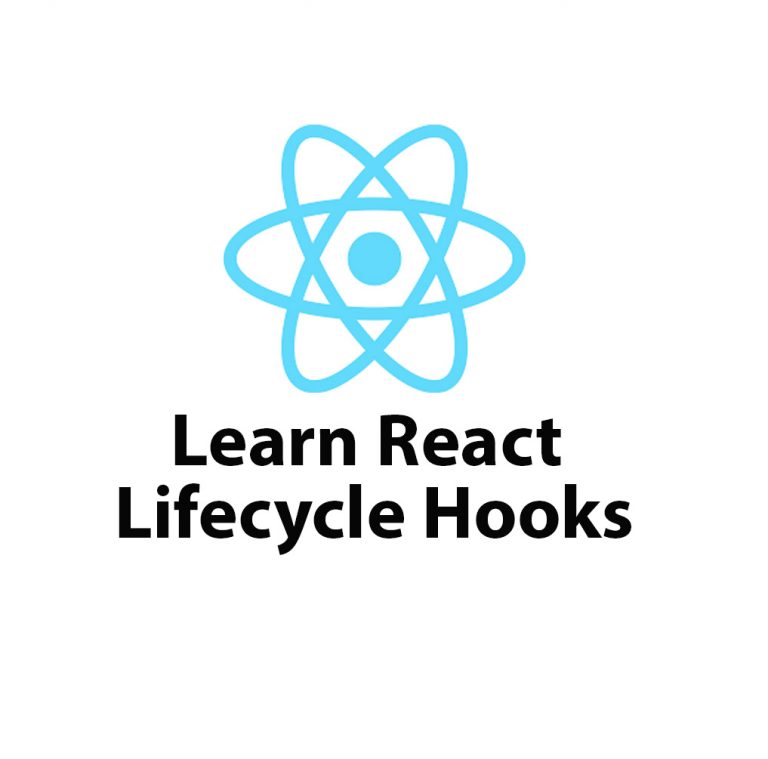
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]
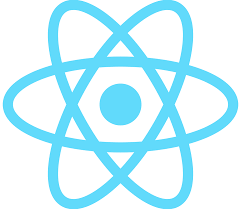
React JS Tutorial
React JS is an open-source JavaScript library that has revolutionized the way web developers create and build single-page applications. It is used by some of the biggest companies in the world, including Facebook, Instagram, Netflix, and Airbnb. If you’re passionate about web development and are looking to add new skills to your resume, then learning […]
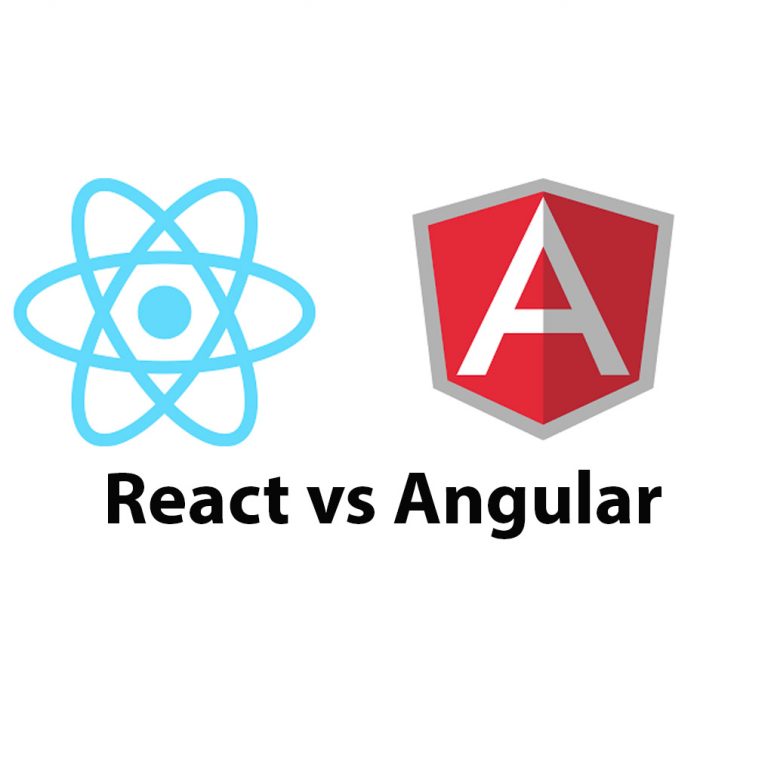
React JS vs Angular: A Comprehensive Guide
Introduction Web development has come a long way since the earlier days of static HTML pages. Web applications have become more dynamic, interactive and more like native applications. This transformation has brought about the rise of JavaScript frameworks and libraries, which have become essential in building web applications. However, choosing between these libraries or frameworks […]
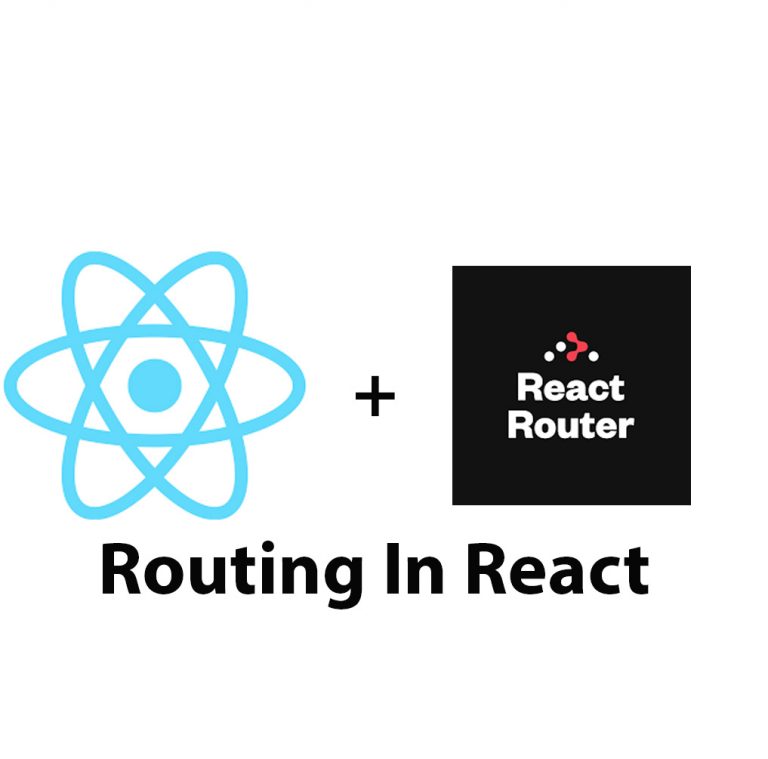
Routing In React JS Using React Router
Introduction React JS has become one of the most popular frontend frameworks for web development. It allows developers to create complex and dynamic user interfaces with ease. Routing is an essential part of web development, and React Router is one such library that is used to manage routing in React applications. In this article, we’ll […]
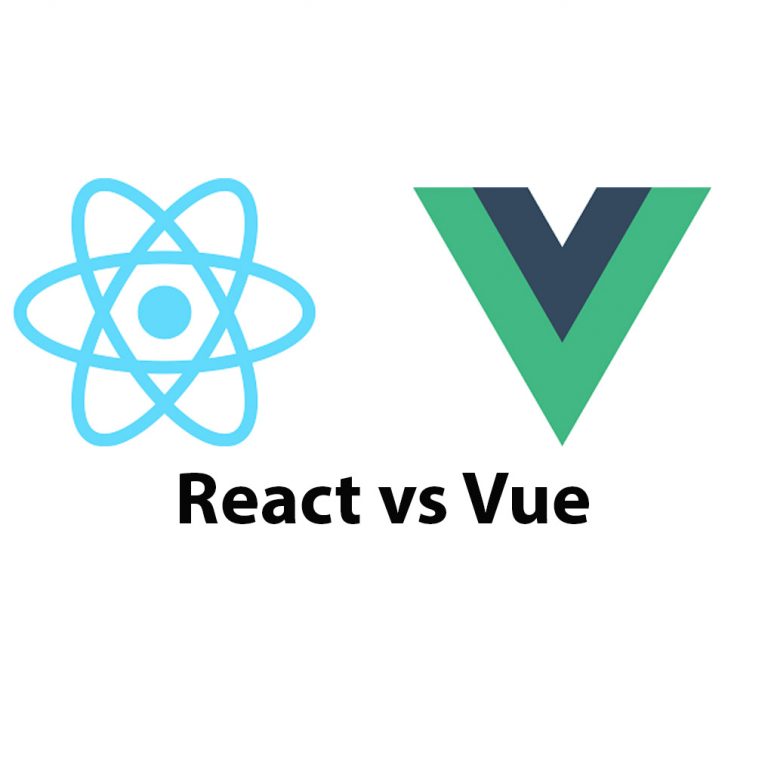
React JS vs Vue: A Comprehensive Guide
Introduction ReactJS and Vue are two of the most popular JavaScript libraries used to develop dynamic user interfaces. These libraries have gained widespread popularity due to their flexibility, efficiency, and ease of use. ReactJS was developed by Facebook, and Vue was created by Evan You. In this article, we are going to evaluate and compare […]
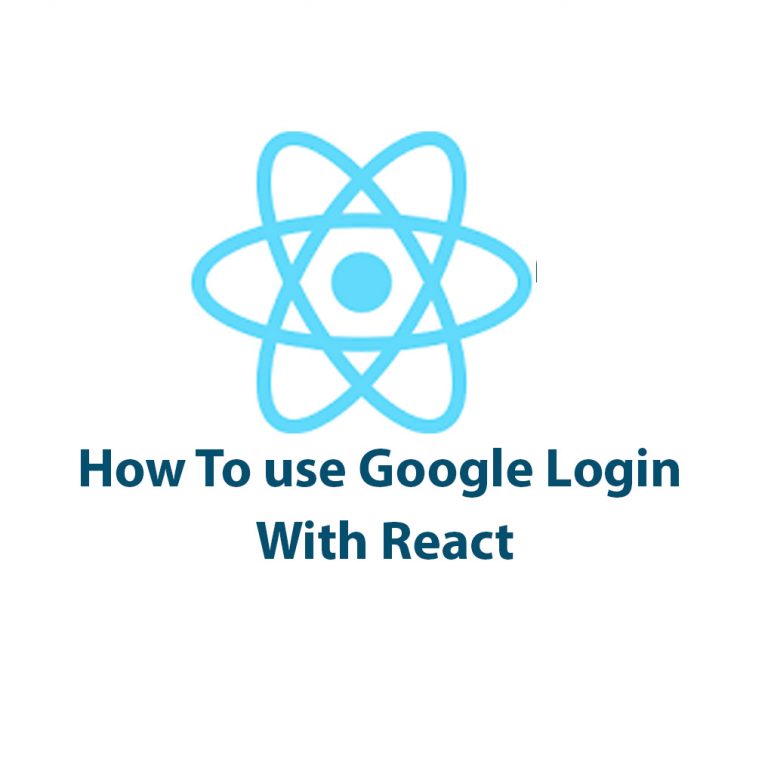
How To Use Google Login With React
As a developer, I cannot stress enough the importance of creating a seamless user experience when it comes to authentication. One of the most popular and convenient ways to allow users to authenticate is by using Google Login. In this article, we’ll explore how to use Google Login with React. Before we delve deep into […]