Introduction
React JS has become one of the most popular frontend frameworks for web development. It allows developers to create complex and dynamic user interfaces with ease. Routing is an essential part of web development, and React Router is one such library that is used to manage routing in React applications.
In this article, we’ll cover the basics of routing in React JS using React Router. We’ll discuss how to get started with React Router, navigate between different components, pass props, handle nested routes, and handle errors.
Getting Started with React Router
Before we dive into the details of React Router, let’s start by installing React Router and setting up the basic components. React Router is available as a separate library, which we can install via the npm package manager.
npm install react-router-dom
The three main components that we use in React Router are Router, Route, and Routes. The Router component is the root component and is responsible for managing the application’s history and location. The Route component is used to define a new route and map it to a specific component. The Routes component is used to group Route components and ensures that at most one Route is rendered at a time.
Once we have installed React Router, we can import these components and use them in our application.
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
function App() {
return (
<Router>
<Routes>
<Route path="/" element={Home} />
<Route path="/about" element={About} />
<Route path="/contact" element={Contact} />
</Routes>
</Router>
);
}
In the above code, we’ve defined three routes: one for the Home component, one for the About component, and one for the Contact component. The exact keyword is used for the Home component to ensure that it only matches the home route ‘/’.
Navigating in React Router
Once we have set up our routes, we can use the Link component to navigate between them. Link is used in place of the traditional anchor tag to ensure that the page is not refreshed on navigation.
import { Link } from 'react-router-dom';
function App() {
return (
<Router>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
<Routes>
<Route path="/" element={Home} />
<Route path="/about" element={About} />
<Route path="/contact" element={Contact} />
</Routes>
</Router>
);
}
In the above code, we’ve added a navbar with three links to the different routes. When one of the links is clicked, the respective component will be rendered.
If we want to highlight the active link, we can use the NavLink component. NavLink is similar to Link but adds an active class when the link is active.
import { NavLink } from 'react-router-dom';
function App() {
return (
<Router>
<nav>
<ul>
<li>
<NavLink exact activeClassName="active" to="/">
Home
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/about">
About
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/contact">
Contact
</NavLink>
</li>
</ul>
</nav>
<Routes>
<Route path="/" element={Home} />
<Route path="/about" element={About} />
<Route path="/contact" element={Contact} />
</Routes>
</Router>
);
}
In the above code, we’ve used NavLink instead of Link and added the activeClassName prop to highlight the active link with the class ‘active’.
Redirecting in React Router
In some cases, we may want to redirect the user to a different route. This can be accomplished using the useNavigate hook.
import { Navigate } from 'react-router-dom';
function App() {
const [isLoggedIn, setIsLoggedIn] = useState(false);
const navigation = useNavigate();
return (
<Router>
<nav>
<ul>
<li>
<NavLink exact activeClassName="active" to="/">
Home
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/about">
About
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/contact">
Contact
</NavLink>
</li>
</ul>
</nav>
<Routes>
<Route path="/" element={Home} />
<Route path="/about" element={About} />
<Route path="/contact" element={Contact} />
<Route path="/login">
{isLoggedIn ? <Navigate to="/"/> : <LoginForm />}
</Route>
</Routes>
</Router>
);
}
In the above code, we’ve added a new Route for the login page. If the user is already logged in, they will be redirected to the home page. Otherwise, the LoginForm component will be rendered.
Passing Props in React Router
In some cases, we may want to pass props to the component being rendered by the Route. This can be accomplished using the render method instead of the component prop.
function App() {
const [username, setUsername] = useState('');
return (
<Router>
<nav>
<ul>
<li>
<NavLink exact activeClassName="active" to="/">
Home
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/about">
About
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/contact">
Contact
</NavLink>
</li>
</ul>
</nav>
<Routes>
<Route path="/" element={Home} />
<Route path="/about" element={About} />
<Route
path="/profile"
render={(props) => <Profile {...props} username={username} />}
/>
<Route path="/contact" element={Contact} />
</Routes>
</Router>
);
}
In the above code, we’ve added a new Route for the Profile page and passed the username prop to the Profile component using the render method.
file component and pass the history object to the component as a prop.
Programmatic Navigation in React Router
In addition to navigating using links and buttons, we can also navigate programmatically using the history object.
The history object is available to components rendered by a Route. It allows us to navigate, go back, and forward.
import { useNavigate } from 'react-router-dom';
function LoginForm() {
const navigation = useNavigate();
const handleSubmit = (event) => {
event.preventDefault();
navigation.navigate('/');
};
return (
<div>
<h1>Login</h1>
<form onSubmit={handleSubmit}>
<label>
Username
<input type="text" />
</label>
<label>
Password
<input type="password" />
</label>
<button type="submit">Submit</button>
</form>
</div>
);
}
In the above code, we’ve used the useHistory hook to get the history object and used history.push to navigate to the home route on form submission.
Nested Routes in React Router
Nested routes are used when one component contains other components that have their own routes. To create nested routes, we need to first define the parent Route and then nest the child routes within it.
function App() {
return (
<Router>
<nav>
<ul>
<li>
<NavLink exact activeClassName="active" to="/">
Home
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/about">
About
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/contact">
Contact
</NavLink>
</li>
</ul>
</nav>
<Routes>
<Route path="/" element={Home} />
<Route path="/about" element={About} />
<Route path="/contact" element={Contact} />
<Route path="/users/:id" element={User} />
<Route path="/users" element={Users} />
</Routes>
</Router>
);
}
function Users() {
return (
<div>
<h1Users</h1>
<p>Select a user</p>
<ul>
<li>
<Link to="/users/1">User 1</Link>
</li>
<li>
<Link to="/users/2">User 2</Link>
</li>
<li>
<Link to="/users/3">User 3</Link>
</li>
</ul>
</div>
);
}
function User(props) {
const { id } = props.match.params;
return (
<div>
<h1>User {id}</h1>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit.</p>
</div>
);
}
In the above code, we have nested routes for the Users component. The Users component renders a list of users with links to their respective pages. The User component is rendered when a user link is clicked, and the user’s id is passed as a prop.
Handling 404 Errors in React Router
In some cases, we may want to handle 404 errors when a user navigates to a non-existent route. This can be accomplished using a wildcard Route that is defined at the end of all other Routes.
function App() {
return (
<Router>
<nav>
<ul>
<li>
<NavLink exact activeClassName="active" to="/">
Home
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/about">
About
</NavLink>
</li>
<li>
<NavLink activeClassName="active" to="/contact">
Contact
</NavLink>
</li>
</ul>
</nav>
<Routes>
<Route path="/" element={Home} />
<Route path="/about" element={About} />
<Route path="/contact" element={Contact} />
<Route path="/users/:id" element={User} />
<Route path="/users" element={Users} />
<Route element={NotFound} />
</Routes>
</Router>
);
}
function NotFound() {
return (
<div>
<h1>404 Not Found</h1>
<p>The page you are looking for does not exist.</p>
</div>
);
}
In the above code, we’ve added a NotFound component to handle 404 errors. The Route component with no path prop matches any route that hasn’t been matched yet and renders the NotFound component.
Conclusion
React Router is an essential tool for managing routing in React JS applications. With React Router, we can easily create nested routes, handle 404 errors, pass props, and navigate programmatically. By following the basics outlined in this article, you can easily get started with React Router and create dynamic and interactive web applications.
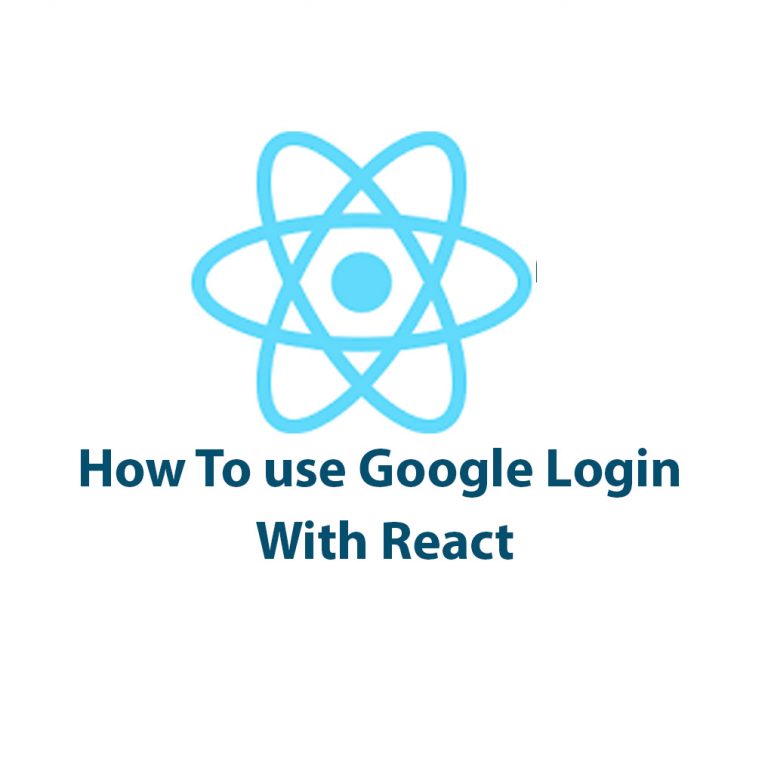
How To Use Google Login With React
As a developer, I cannot stress enough the importance of creating a seamless user experience when it comes to authentication. One of the most popular and convenient ways to allow users to authenticate is by using Google Login. In this article, we’ll explore how to use Google Login with React. Before we delve deep into […]
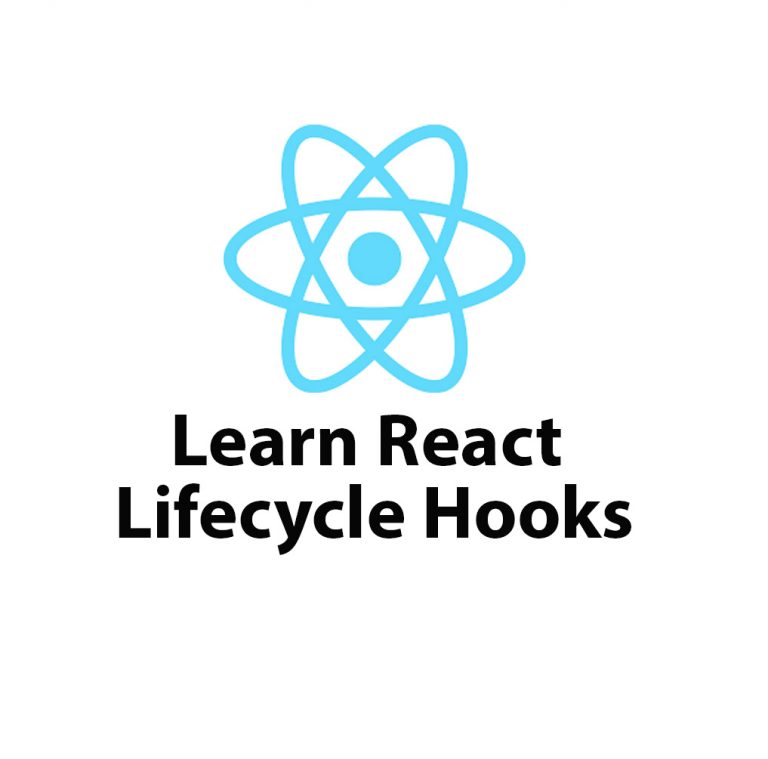
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]
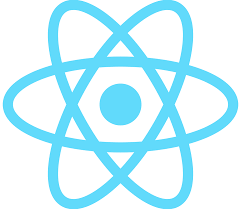
React JS Tutorial
React JS is an open-source JavaScript library that has revolutionized the way web developers create and build single-page applications. It is used by some of the biggest companies in the world, including Facebook, Instagram, Netflix, and Airbnb. If you’re passionate about web development and are looking to add new skills to your resume, then learning […]
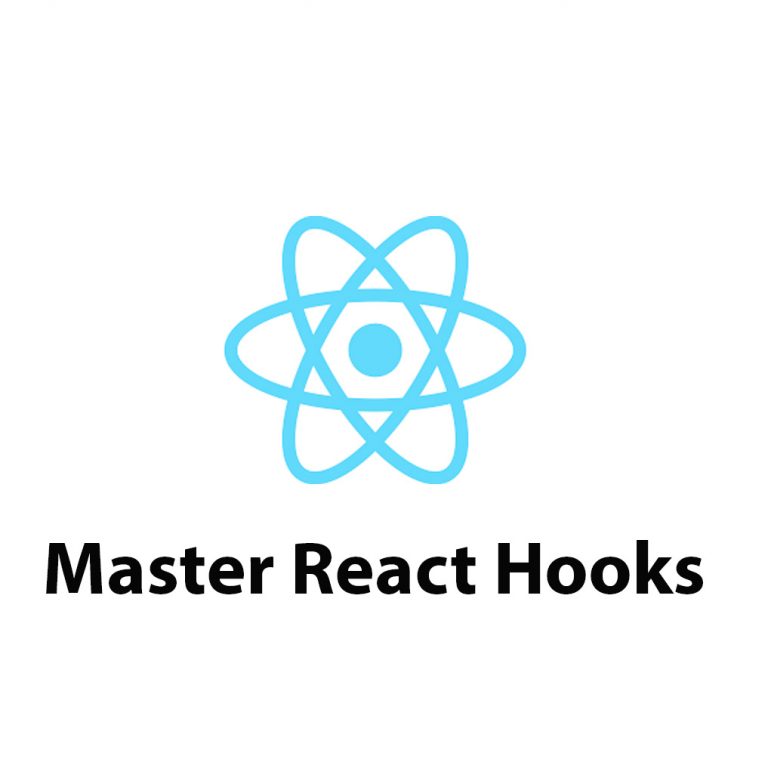
Mastering Hooks In React JS
Introduction React JS is a popular JavaScript library that has gained a lot of popularity since its introduction in 2013. It has been adopted by many web developers who use it to build robust and reliable web applications. With React JS, developers can create user interfaces by building reusable UI components, which can be combined […]
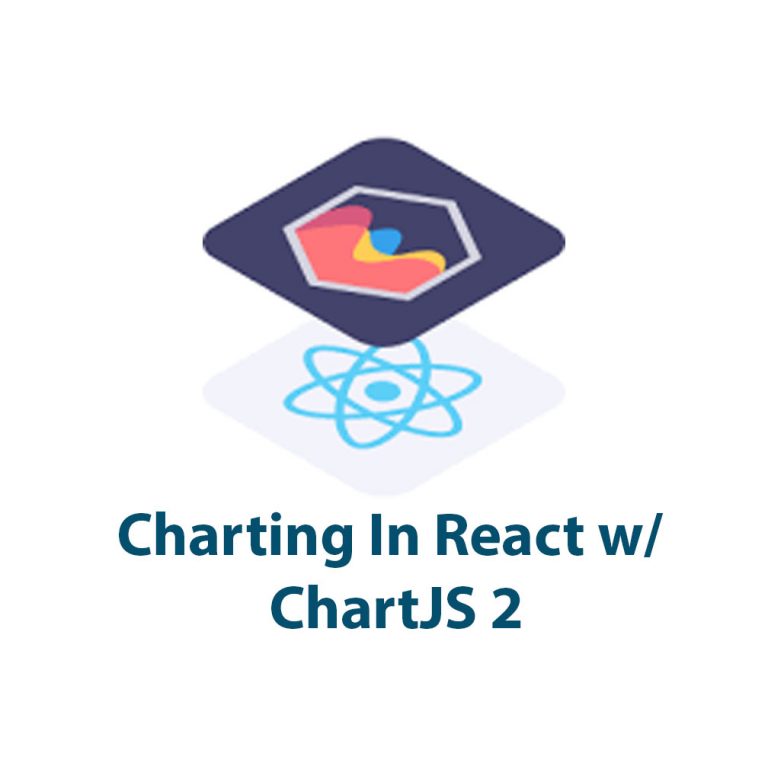
React Charts With ChartJS 2
As a web developer, I understand the importance of data visualization in presenting complex information in an understandable and visually appealing way. That’s why I’ve been using ChartJS 2, a powerful JavaScript library, for creating charts in my React apps. In this article, I’ll show you how to set up and use ChartJS 2 in […]
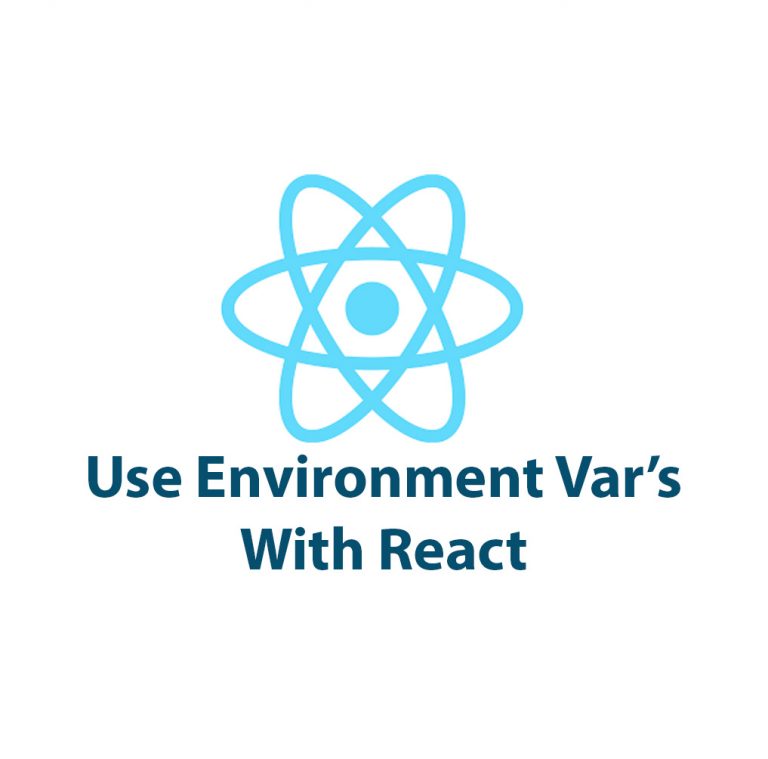
How To Use Environment Variables In React
Introduction Have you ever had to work on a React application and found yourself dealing with different environments or sensitive data? Setting up environment variables can make your life easier and provide a safer way to store information that should not be accessible to the public. In this article, we’re going to look at how […]