Introduction
As a software developer who is always looking for efficient ways to write clean code, I discovered Typescript and the concept of iterators. Typescript is a JavaScript superset that enables the creation of more structured and maintainable code. In this article, I am introducing Typescript iterators and delving into their different types, how to use them, their advantages, limitations, and how they compare to generators.
What are Typescript Iterators?
An iterator is an object that implements the iteration protocol that enables looping over objects such as Arrays, Maps, and Sets. Typescript iterators are similar to iterators in JavaScript, with an additional emphasis on type annotations.
Types of Iterators
There are three types of iterators in Typescript, which are:
- Array Iterators:
An array is a collection of elements, and the array iterator contains a function that returns values sequentially and one at a time from the array. The array iterator object comes with two methods, which arenext()
andreturn()
. Thenext()
method returns the next element in the array, and thereturn()
method stops the iteration. - Map Iterators:
Maps are collections that store key-value pairs of elements. The Map iterator contains a function that returns the key-value pairs sequentially. The Map iterator object has two methods known asnext()
andreturn()
. Thenext()
method returns an object with thevalue
property set to the current key-value pair being iterated over. Thereturn()
method stops the iteration. - Set Iterators:
Sets are also collections that store unique elements. The Set iterator contains a function that returns the set elements sequentially. The Set iterator object has two methods known asnext()
andreturn()
. Thenext()
method returns an object with thevalue
property set to the current element being iterated over. Thereturn()
method stops the iteration.
How to use Typescript Iterators
Using Typescript iterators is very similar to using iterators in JavaScript.
Basics of creating Iterators:
To create a new iterator, you need to implement the iteration protocol by declaring an Iterable
interface and an Iterator
interface as illustrated below.
interface Iterable<T> {
[Symbol.iterator](): Iterator<T>;
}
interface Iterator<T> {
next(value?: any): IteratorResult<T>;
return?(value?: any): IteratorResult<T>;
throw?(e?: any): IteratorResult<T>;
}
Working with for…of loop:
The for…of statement in Typescript is used to iterate objects that implement the iterable interface. The loop statement is illustrated below.
for (let value of iterable) {
// code block that gets executed
}
Implementing custom iterators:
You can implement custom iterators in Typescript by creating iterable objects that can be iterated over using for…of loops. Custom iterators enable software developers to define their own objects such as DOM trees and databases to return values sequentially.
Advantages of using Typescript Iterators
- Enhanced Programming Experience:
Typescript iterators can make software development easier and quicker. As iterators enable software developers to access the data they want and need from arrays, maps, and sets in a specific and structured manner. - Improved Code Readability:
Typescript iterators can make software code easier to read, understand, and maintain. The use of iterators makes code cleaner, and developers can extract only the needed data instead of processing all the data. - Reduced Code Complexity:
Typescript iterators make it easier for developers to write more sophisticated and efficient algorithms. Iterators can reduce the complexity of code, improve the readability of code, and prevent developers from making hard-to-find errors.
Limitations of Typescript Iterators
- Additional Overhead:
Typescript iterators add additional overhead to software code, which can slow down the performance of the software application. The additional overhead happens when using custom iterators to define large data structures. - Limitations to Specific Data Structures:
Typescript iterators have limitations to specific data structure types such as arrays, maps, and sets. Custom objects cannot implement theIterable
interface.
Comparison between Typescript Iterators and Generators
Both Typescript iterators and generators enable software developers to iterate over objects, however, there are differences in how they function.
Functionality Comparison:
Typescript iterators return a set of defined objects, while generators return a sequence of values, not a set of objects. Typescript iterators perform the role of a data structure, whereas generators perform the role of a function.
Use Cases Comparison:
Typescript iterators are more efficient for accessing data in objects such as arrays, maps, and sets. Generators, on the other hand, are suitable for accessing data that cannot be easily defined by an object, such as data from a WebSocket.
Conclusion
To sum it all up, Typescript iterators are similar to iterators in JavaScript, with an additional focus on type annotations. They come in three flavors, which are array iterators, map iterators, and set iterators, with a similar implementation in each case. The advantages of Typescript iterators include the improvement of the programming experience, code readability, and the reduction of code complexity. Their limitations relate to additional overheads to software code and their restricted usage to specific data structures. Finally, Typescript iterators and generators differ in their functionality and use cases, with Typescript iterators better suited to accessing data in objects such as arrays, maps, and sets. Further research into Typescript iterators and generators is encouraged, with attention given to the implementation and use of custom iterators and their performance.
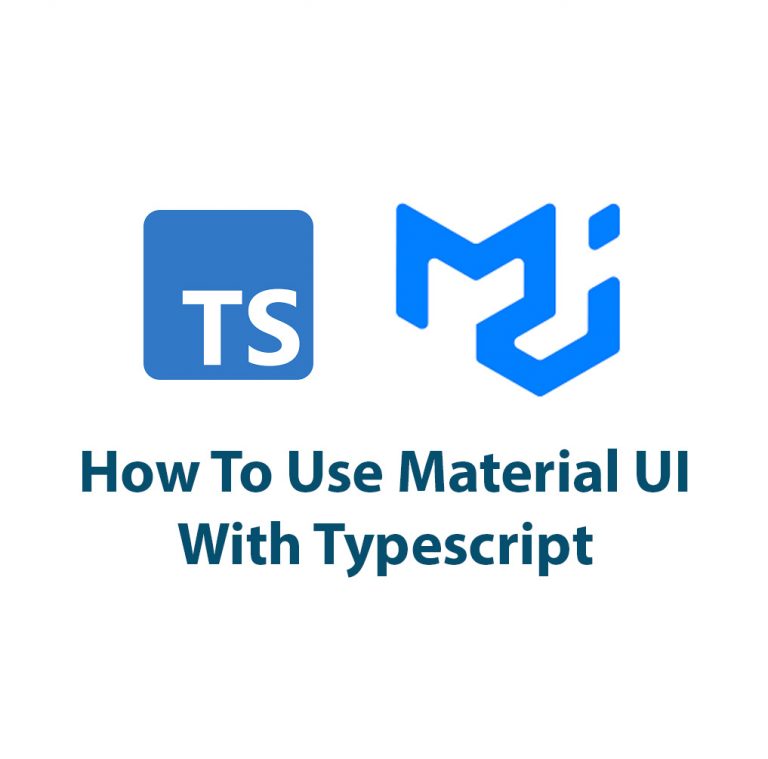
Use Material UI V5 With Typescript
Introduction Hello everyone! In this article, we are going to talk about Material UI V5 with Typescript. If you are a React developer, you must have heard of Material UI. It is one of the most popular React UI component libraries out there. On the other hand, Typescript is a superset of JavaScript that provides […]
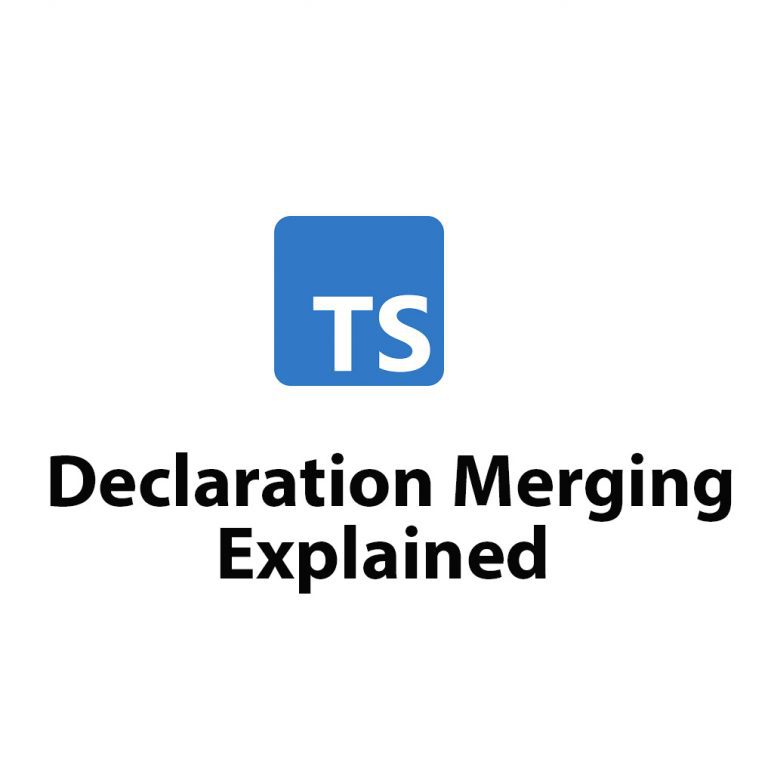
Typescript Declaration Merging
Introduction Typescript Declaration Merging is an essential feature of the Typescript language, which allows developers to extend existing code without modifying it. It can be a bit complex and overwhelming for beginners, but once you understand how it works, you’ll find it to be a powerful tool. As a developer, you will come across scenarios […]
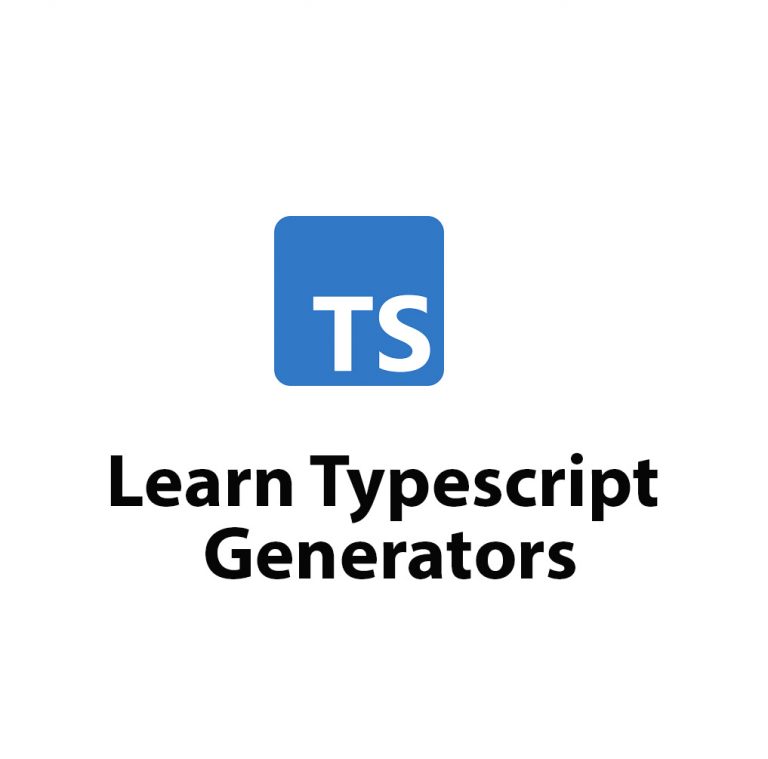
Learn Typescript Generators
Introduction TypeScript is a typed superset of JavaScript that brings static type checking to JavaScript development. TypeScript adds some much-needed structure and safety to JavaScript programming by providing tools for catching errors at compile time instead of run time. A generator is a feature that TypeScript borrows from Python. Generators are a special kind of […]
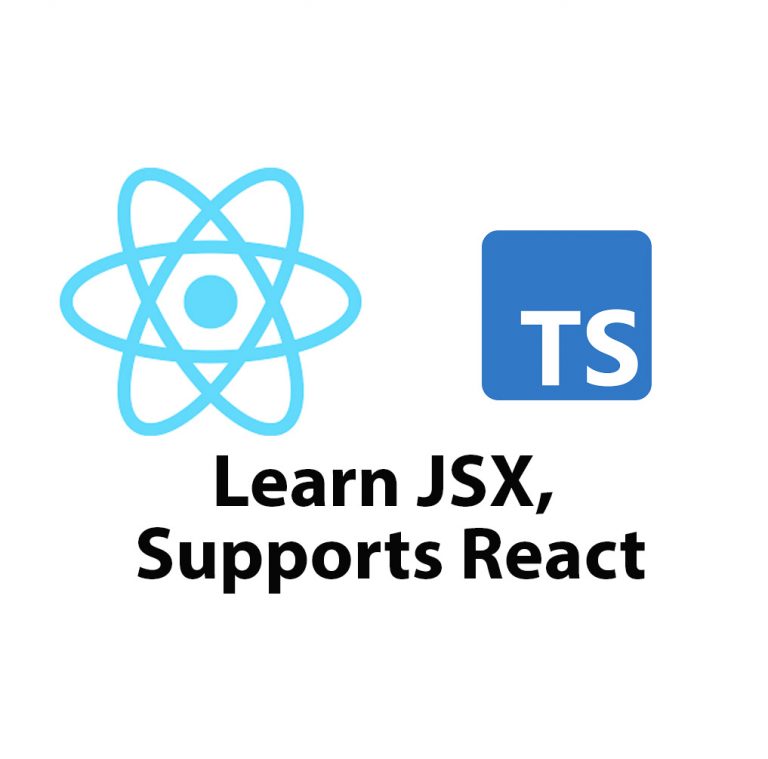
What Is JSX and how to use it.
Introduction As a web developer, I’m always on the lookout for new tools and technologies that can help me write better code faster. One tool that has caught my attention lately is Typescript JSX. In this article, I’m going to share my experience learning Typescript JSX and the benefits it has brought to my development […]
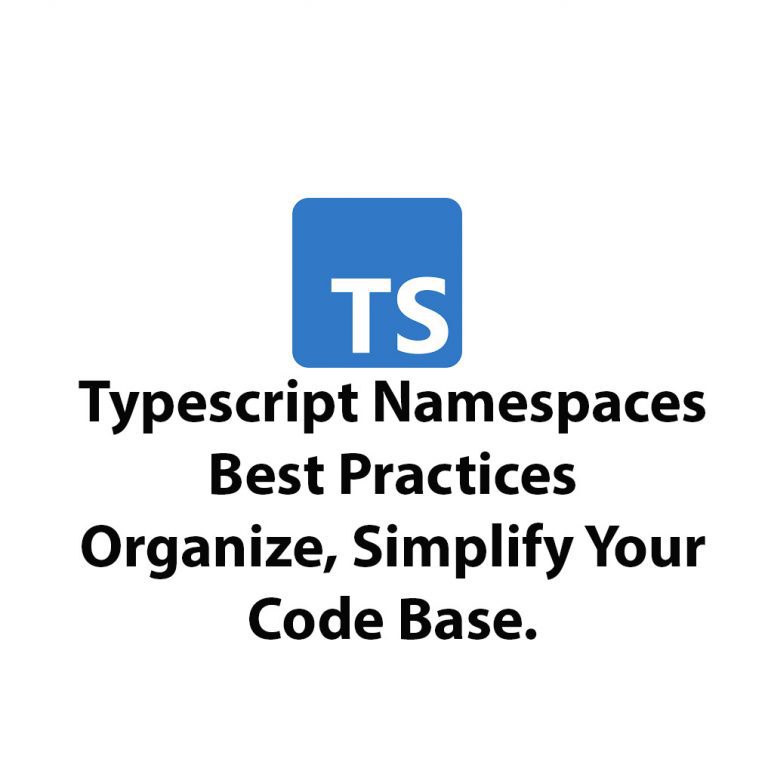
Typescript Namespaces: Best Practices
As a software developer, I have been intrigued by TypeScript’s ability to introduce new features to the JavaScript language while also minimizing the potential for runtime errors. One of the best things about TypeScript is that it provides excellent support for large codebases, and one of the features that can help with this is Namespaces. […]
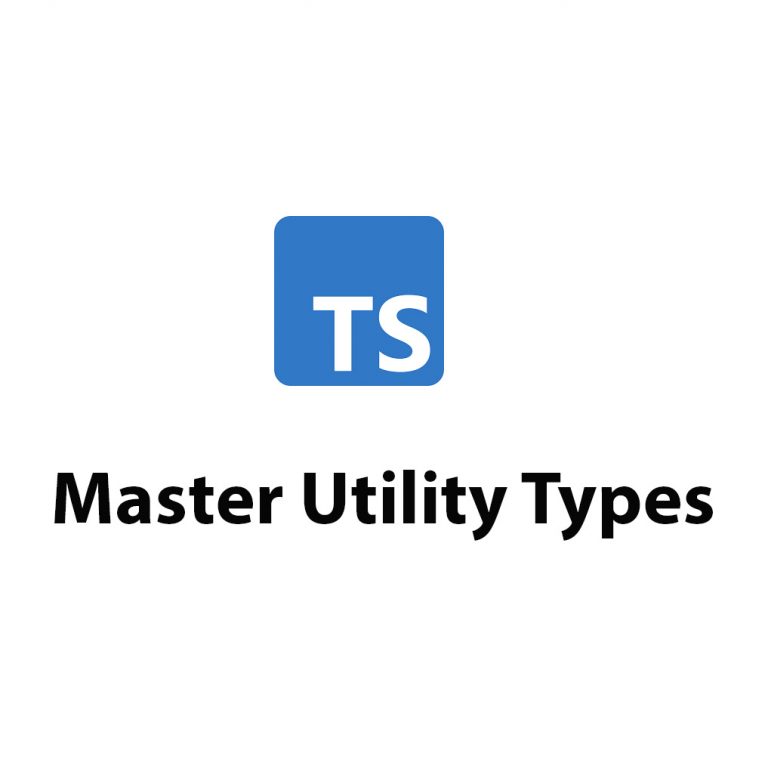
Typescript Utility Types
Introduction Hi there, I’m excited to talk to you today about Typescript Utility Types. First, let’s provide a brief overview of what Typescript is for those who may not be familiar with it. Typescript is an open-source programming language that builds on top of JavaScript by adding static types to code. This can help catch […]