As a developer, I am always looking for ways to make my code more organized and efficient. One tool that has helped me achieve this goal is Typescript modules. In this article, I will be discussing Typescript modules in-depth, including how to create and import them, how to declare dependencies, and the benefits of using modules in your Typescript projects.
Typescript Modules: What are They?
Before we dive into the complexities of Typescript modules, it’s important to understand what exactly they are. In Typescript, a module is a separate file that contains code related to a specific functionality. This code can be imported and used in other files, allowing you to easily split your code into smaller and more manageable parts.
Typescript supports three types of modules: file-per-class, single-file modules, and multi-file modules. Each type has its advantages and disadvantages, and it’s important to understand the differences between them before deciding which one to use in your project.
Creating and Importing Modules in Typescript
The first step in using Typescript modules is creating and importing them. Let’s take a closer look at each type of module and the steps involved in creating and importing them.
File-per-Class
A file-per-class module is a module where each class in your project is defined in its own separate file. This approach is often used in object-oriented programming, where each class is treated as a separate entity and given its own file.
To create a file-per-class module in Typescript, you simply create a new file for each class in your project and export the class from the file using the export keyword. Here’s an example:
// Car.ts
export class Car {
make: string;
model: string;
year: number;
constructor(make: string, model: string, year: number) {
this.make = make;
this.model = model;
this.year = year;
}
start() {
console.log('Starting car...');
}
stop() {
console.log('Stopping car...');
}
}
To import the class in another file, you use the import keyword followed by the name of the class and the path to the file:
import { Car } from './Car';
const myCar = new Car('Toyota', 'Corolla', 2022);
Single-File Modules
A single-file module is a module that contains all of the code for a specific functionality in a single file. This approach is often used for smaller projects or for modules that only contain a small amount of code.
To create a single-file module in Typescript, you simply define all of the code for the module in a single file and export the functionality using the export keyword. Here’s an example:
// utils.ts
export function square(x: number): number {
return x * x;
}
export function cube(x: number): number {
return x * x * x;
}
To import the module in another file, you use the import keyword followed by the name of the function and the path to the file:
import { square } from './utils';
const result = square(5);
console.log(result); // Output: 25
Multi-File Modules
A multi-file module is a module that contains multiple files, each containing a specific part of the functionality for the module. This approach is often used for larger projects or for modules that contain a lot of code.
To create a multi-file module in Typescript, you create a separate file for each part of the module and use the export keyword to make the functionality available to other parts of the module. Here’s an example:
// Math.ts
export * from './Addition';
export * from './Subtraction';
export * from './Multiplication';
export * from './Division';
In this example, the Math module contains code related to basic arithmetic operations. Each operation is defined in a separate file, and the Math module simply exports all of the functionality using the export * syntax.
To import the module in another file, you would use the import keyword followed by the name of the part of the module you want to use and the path to the file:
import { Add } from './Math';
const result = Add(5, 10);
console.log(result); // Output: 15
Exporting and Importing Multiple Members with a Namespace
Sometimes, you may want to export and import multiple members from a module using a namespace. A namespace allows you to group related functionality together under a single name, making it easier to use and organize your code.
To export multiple members from a module using a namespace, you would define the namespace at the top of the module and then use the export keyword to export each member:
// Utils.ts
namespace MathUtils {
export function square(x: number): number {
return x * x;
}
export function cube(x: number): number {
return x * x * x;
}
}
export default MathUtils;
To import the namespace in another file, you would use the import keyword followed by the namespace name and the path to the file:
import MathUtils from './Utils';
const result1 = MathUtils.square(5);
const result2 = MathUtils.cube(5);
console.log(result1); // Output: 25
console.log(result2); // Output: 125
Declaring Module Dependencies
In larger projects, it’s not uncommon for different parts of the project to depend on each other. To manage these dependencies, Typescript allows you to declare dependencies between modules using the reference tag or external module systems.
Using the Reference Tag
To use the reference tag for declaring module dependencies, you simply add a reference tag at the top of each file that contains a reference to the module it depends on. Here’s an example:
// FileA.ts
/// <reference path="./FileB" />
export function DoSomething() {
const result = Add(5, 10);
console.log(result);
}
In this example, the reference tag tells the Typescript compiler that FileA depends on FileB and should be compiled and loaded in the correct order.
Use of Module Systems
Alternatively, you can use external module systems like CommonJS or AMD to manage module dependencies. To use an external module system, you would define your modules using the appropriate syntax and then use a module bundler like Webpack or Browserify to compile and bundle the modules into a single file for use in the browser.
Benefits of Using Typescript Modules
Now that we’ve covered the basics of Typescript modules, let’s take a look at the benefits of using modules in your Typescript projects.
- Code Organization: Modules allow you to split your code into smaller, more manageable parts, making it easier to read, understand, and maintain.
- Reusability: Modules can be shared between different parts of your project or even between multiple projects, allowing you to reuse code and reduce duplication.
- Separation of Concerns: Modules allow you to separate different parts of your code according to their functionality, making it easier to change or update specific parts of your code without affecting the rest of the project.
- Scalability: Modules allow you to add new functionality to your project without having to refactor existing code or worry about breaking other parts of the project.
Conclusion
In conclusion, Typescript modules are an essential tool for any developer working with Typescript. Whether you’re working on a small project or a large-scale enterprise application, modules can help you organize your code, improve reusability, and make your projects more manageable and scalable. So if you haven’t already started using modules in your Typescript projects, now is the time to start.
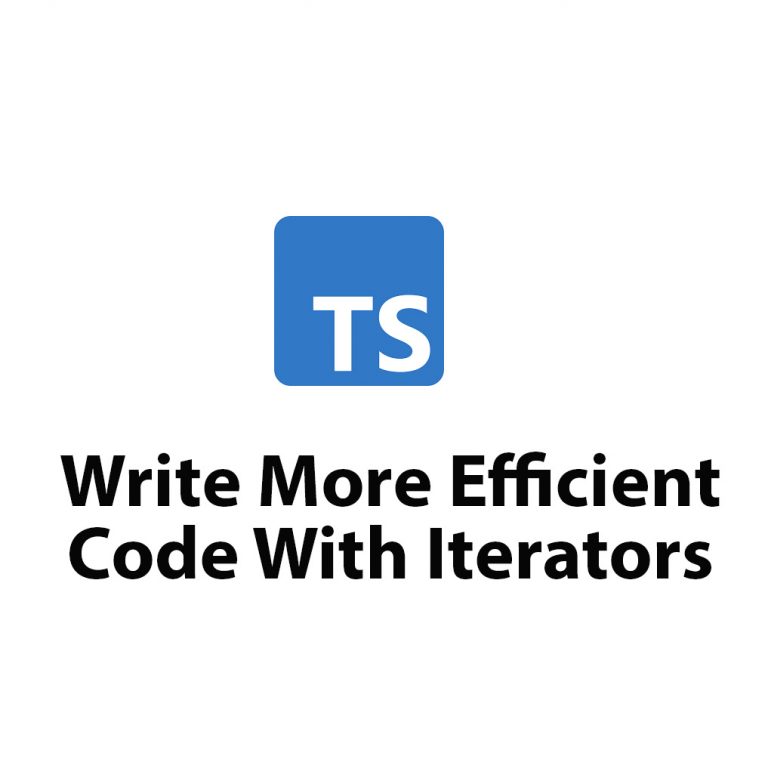
Learning Typescript Iterators
Introduction As a software developer who is always looking for efficient ways to write clean code, I discovered Typescript and the concept of iterators. Typescript is a JavaScript superset that enables the creation of more structured and maintainable code. In this article, I am introducing Typescript iterators and delving into their different types, how to […]
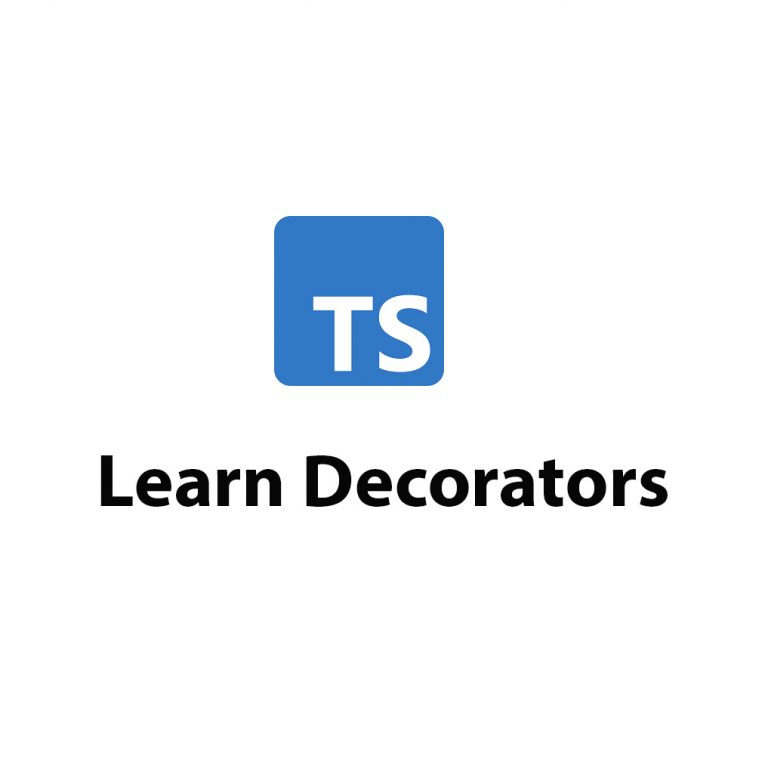
Mastering Typescript Decorators
As a software developer, I am constantly looking for ways to improve the efficiency and effectiveness of my work. One of the most powerful tools in my arsenal has been typescript decorators. In this article, I will dive deep into the world of typescript decorators, exploring their history, various types, and usage in software development. […]
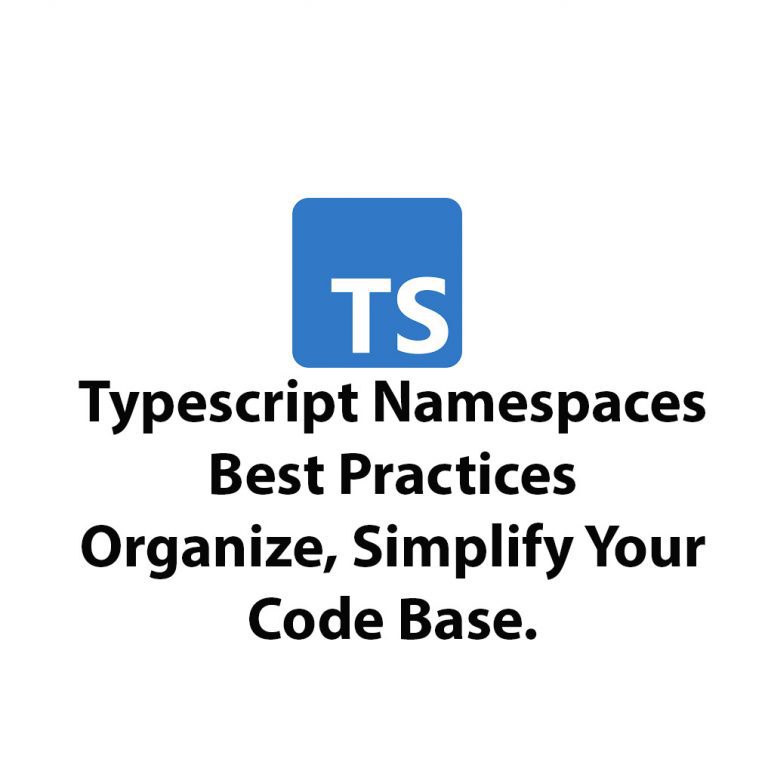
Typescript Namespaces: Best Practices
As a software developer, I have been intrigued by TypeScript’s ability to introduce new features to the JavaScript language while also minimizing the potential for runtime errors. One of the best things about TypeScript is that it provides excellent support for large codebases, and one of the features that can help with this is Namespaces. […]
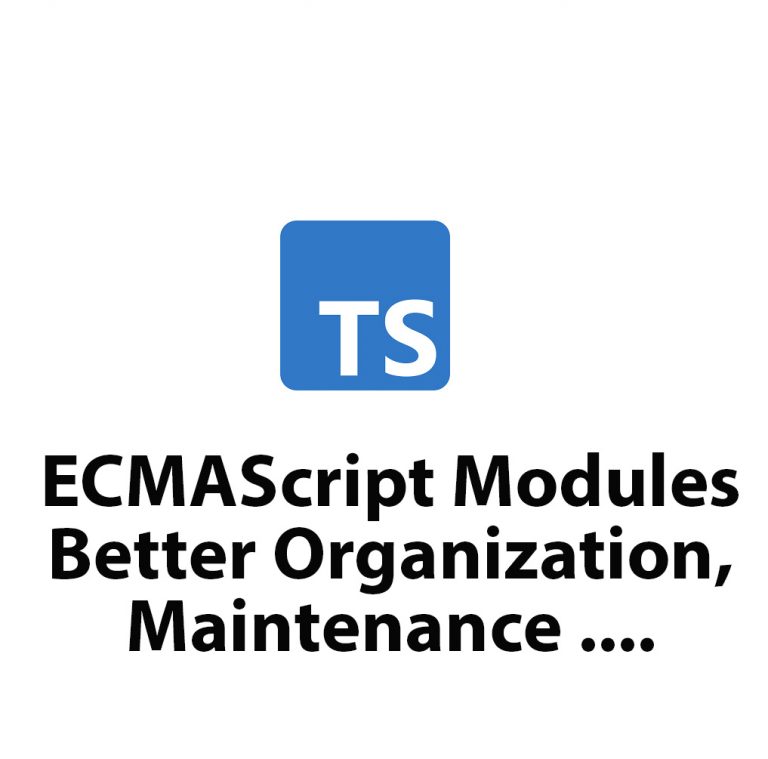
ECMAScript Modules in Node JS
As a software developer and avid Node JS user, I’ve always been on the lookout for ways to improve my workflow and simplify code maintenance. One of the most recent additions to Node JS that has greatly helped me achieve these goals is the implementation of ECMAScript (ES) Modules. ES Modules are a standard format […]
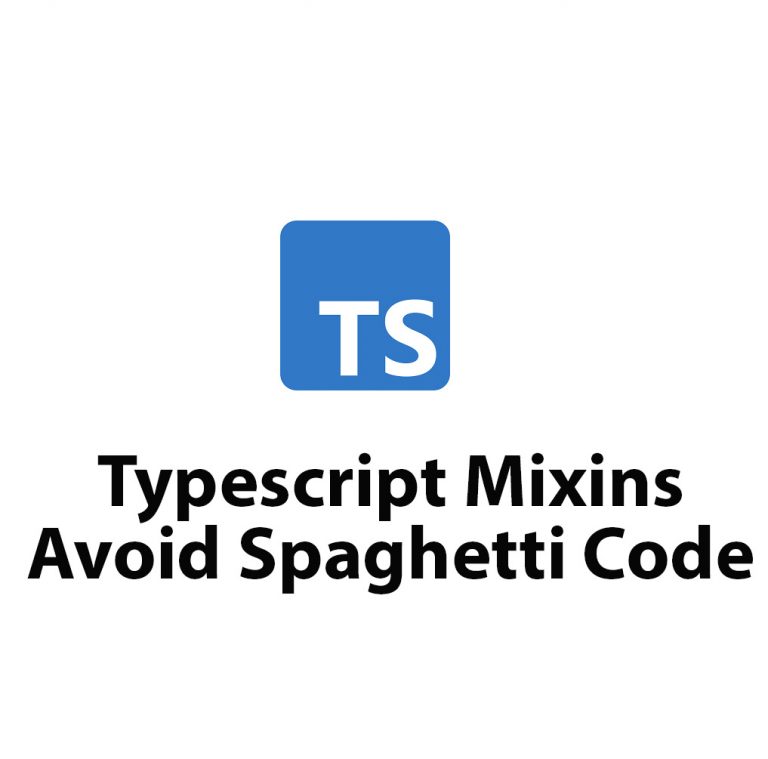
Learn Typescript Mixins: Avoid Spaghetti Code
As a software developer, I’ve been using Typescript for most of my latest projects. One of the most interesting features I’ve come across is Typescript Mixins. These allow for simple composability of classes and functions, which can help to reduce code complexity and improve maintainability. In this article, I’m going to provide a comprehensive guide […]
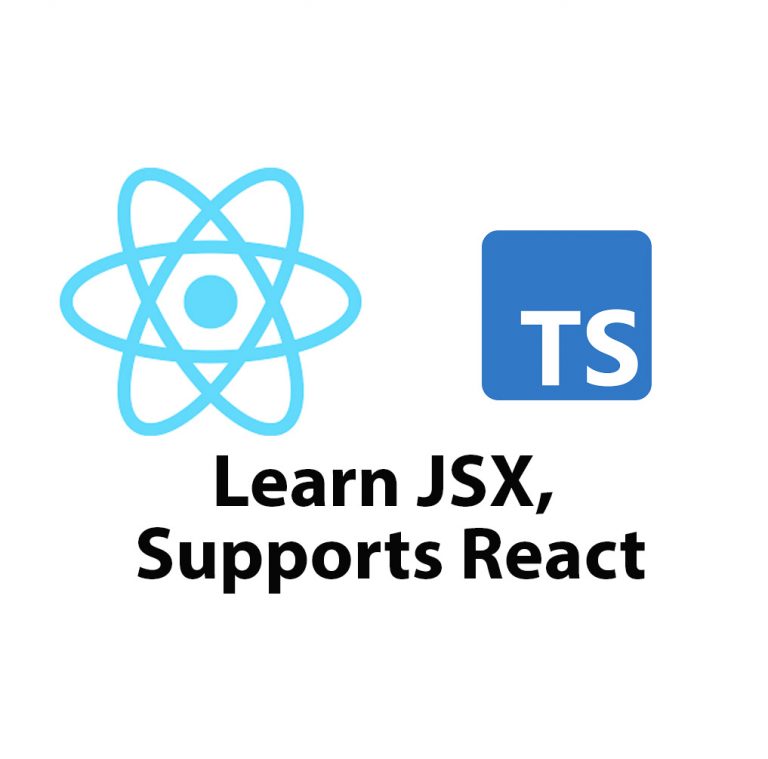
What Is JSX and how to use it.
Introduction As a web developer, I’m always on the lookout for new tools and technologies that can help me write better code faster. One tool that has caught my attention lately is Typescript JSX. In this article, I’m going to share my experience learning Typescript JSX and the benefits it has brought to my development […]