Introduction
As a web developer, I’m always on the lookout for new tools and technologies that can help me write better code faster. One tool that has caught my attention lately is Typescript JSX. In this article, I’m going to share my experience learning Typescript JSX and the benefits it has brought to my development process.
What is Typescript JSX?
Typescript JSX is a combination of two technologies: Typescript and JSX. Typescript is a superset of JavaScript that adds optional static typing to the language. JSX, on the other hand, is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files.
When combined, Typescript and JSX offer a powerful way to write dynamic and type-safe UI components for web applications. With Typescript, you can catch type-related errors at compile time, while with JSX, you can write more declarative and expressive code that closely resembles HTML.
Benefits of Learning Typescript JSX
Learning Typescript JSX offers several benefits to web developers, including:
- Type Safety – Typescript JSX allows you to catch errors at compile time by detecting type mismatches and other bugs in your code. This ensures that your code is more robust and easier to maintain.
- Better Code Organization – By using JSX, you can write more declarative and expressive code that closely resembles HTML. This makes your code more readable and easier to understand, which can save you time and effort when debugging and updating your code.
- Improved Developer Productivity – With Typescript JSX, you can write code faster and with fewer errors. This is because you can leverage the power of static typing and JSX syntax to write more efficient and scalable UI components.
Getting Started with Typescript JSX
To get started with Typescript JSX, you will need to set up your development environment. This involves installing and configuring several tools, including:
- Node.js and NPM – These are required to run and manage your project dependencies.
- TypeScript – This is the language that you will use to write your Typescript JSX code.
- React – This is a JavaScript library that simplifies the process of building UI components in web applications.
Once you’ve set up your development environment, you can start writing Typescript JSX code in your text editor or IDE of choice.
Understanding JSX Elements
In Typescript JSX, you can think of UI components as functions that return JSX elements. These JSX elements describe the structure and appearance of your UI components, similar to how HTML describes the structure and appearance of a web page.
To create a simple JSX element, you can write code like this:
const myElement = <h1>Hello World</h1>;
This code creates a new JSX element that renders an h1
heading with the text “Hello World”. You can then render this element in your web application using the ReactDOM.render()
method:
import React from 'react';
import ReactDOM from 'react-dom';
ReactDOM.render(myElement, document.getElementById('root'));
This code renders the myElement
JSX element in the HTML element with the ID ‘root’ in your web page.
Using Props in JSX Elements
Props are a powerful way to pass data from one component to another in your web application. In Typescript JSX, you can define props using interfaces or types, like this:
interface MyProps {
name: string;
}
const Greeting = ({ name }: MyProps) => {
return <h1>Hello {name}!</h1>;
};
This code defines a new interface called MyProps
that includes a single property called name
of type string
. It then defines a new functional component called Greeting
that accepts an object with MyProps
as its argument and returns a JSX element that renders the name
property.
You can then pass data to this component when you render it, like this:
<Greeting name="Alice" />
This code passes a value of “Alice” as the name
prop to the Greeting
component, which then renders a heading that says “Hello Alice!”.
Typescript and JSX
Typescript offers several features that work well with JSX. One such feature is the ability to use types with your JSX code to catch type-related errors at compile time.
For example, you can define a custom type called MyElementProps
that includes several properties:
type MyElementProps = {
name: string;
age: number;
email: string;
};
You can then define a functional component that accepts a prop of type MyElementProps
:
const MyElement = ({ name, age, email }: MyElementProps) => {
return (
<div>
<h1>{name}</h1>
<p>{age}</p>
<a href={`mailto:${email}`}>{email}</a>
</div>
);
};
With this setup, Typescript will enforce that the name
prop is a string, the age
prop is a number, and the email
prop is a string that looks like an email address. This can save you time and effort when debugging your application, as you can catch errors related to incompatible types or missing props at compile time instead of at runtime.
Styling in Typescript JSX
Styling your JSX components is an important part of creating compelling and visually appealing web applications. There are several ways to style your JSX components in Typescript JSX, including using CSS and CSS modules.
With CSS modules, you can write CSS code that applies only to specific JSX components. This can help you avoid class name collisions and keep your CSS code organized.
Here’s an example of how to use CSS modules in Typescript JSX:
import styles from './MyComponent.module.css';
const MyComponent = () => {
return (
<div className={styles.myComponent}>
<h1>My Component</h1>
<p>Some text here</p>
</div>
);
};
In this code, we’re importing a CSS module from a file called MyComponent.module.css
. We then apply this CSS code to our div
element by setting its className
to styles.myComponent
.
You can then define the styles for this component in the MyComponent.module.css
file, like this:
.myComponent {
background-color: #f4f4f4;
padding: 1rem;
}
This code defines a new CSS class called myComponent
that sets the background color to #f4f4f4
and adds a padding of 1rem
.
Handling Events in Typescript JSX
Events are an important part of building interactive web applications, and Typescript JSX makes it easy to handle events in your code.
To add an event handler to a JSX element, you can use the onEventName
syntax. For example, to add a click event handler to a button, you can write code like this:
const handleClick = () => {
console.log('Button Clicked!');
};
const MyButton = () => {
return <button onClick={handleClick}>Click Me</button>;
};
This code defines a new function called handleClick
that logs a message to the console. It then defines a new MyButton
component that renders a button and attaches the handleClick
function to its onClick
event.
You can then use this button component in your web application like this:
<MyButton />
When you click the button, it will trigger the handleClick
function and log the message “Button Clicked!” to the console.
Conditional Rendering in Typescript JSX
Conditional rendering is an important concept in web development, and Typescript JSX provides several ways to handle it.
One common way to handle conditional rendering in Typescript JSX is by using ternary operators. For example, let’s say you have a component that renders a heading and a paragraph based on a boolean prop called showText
.
You can define this component like this:
interface MyComponentProps {
showText: boolean;
}
const MyComponent = ({ showText }: MyComponentProps) => {
return (
<div>
{showText ? <h1>Hello World</h1> : null}
{showText ? <p>Some text here</p> : null}
</div>
);
};
In this code, we’re using the ternary operator (?
) to conditionally render the heading and paragraph elements based on the showText
prop.
Conclusion
Typescript JSX is a powerful tool for web developers that can help to improve code organization, type safety, and developer productivity. By writing dynamic and type-safe UI components with Typescript JSX, you can create more efficient and scalable web applications that are easier to maintain and update.
In this article, we’ve covered the basics of Typescript JSX, including how to get started, how to use JSX elements and components, and how to handle styling, events, and conditional rendering. I hope this article has motivated you to start learning Typescript JSX and to explore its potential in your own web development projects.
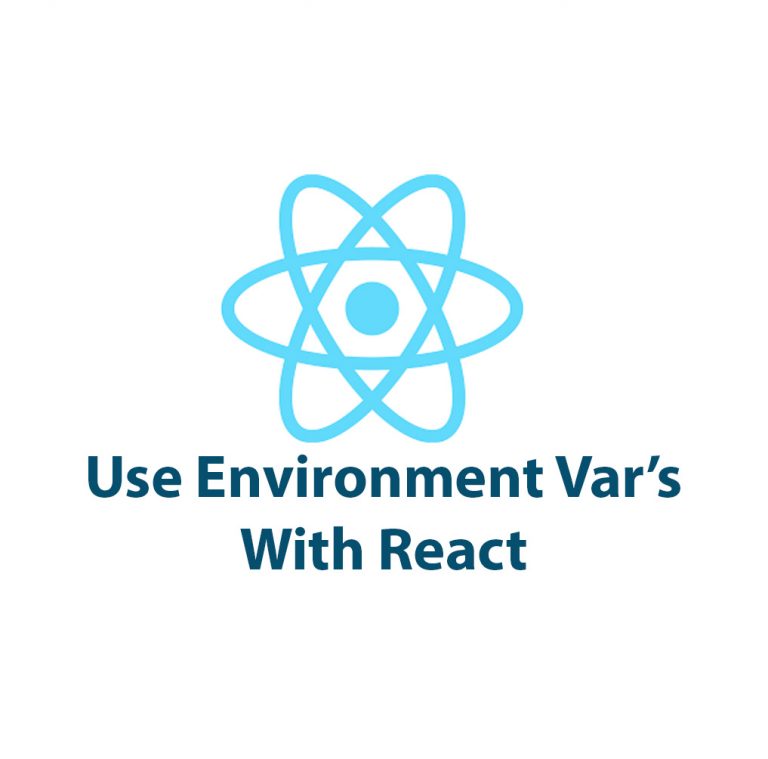
How To Use Environment Variables In React
Introduction Have you ever had to work on a React application and found yourself dealing with different environments or sensitive data? Setting up environment variables can make your life easier and provide a safer way to store information that should not be accessible to the public. In this article, we’re going to look at how […]
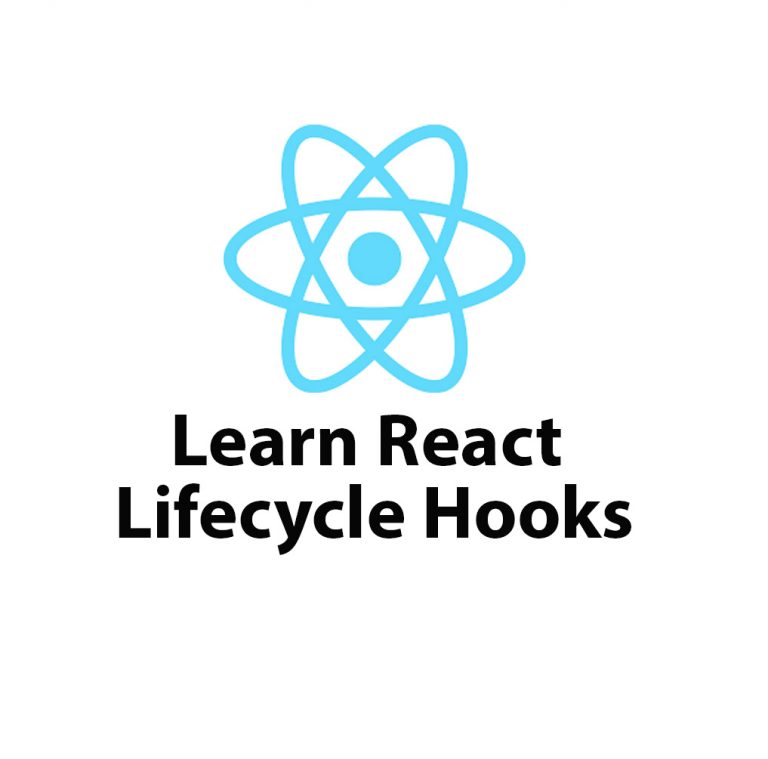
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]
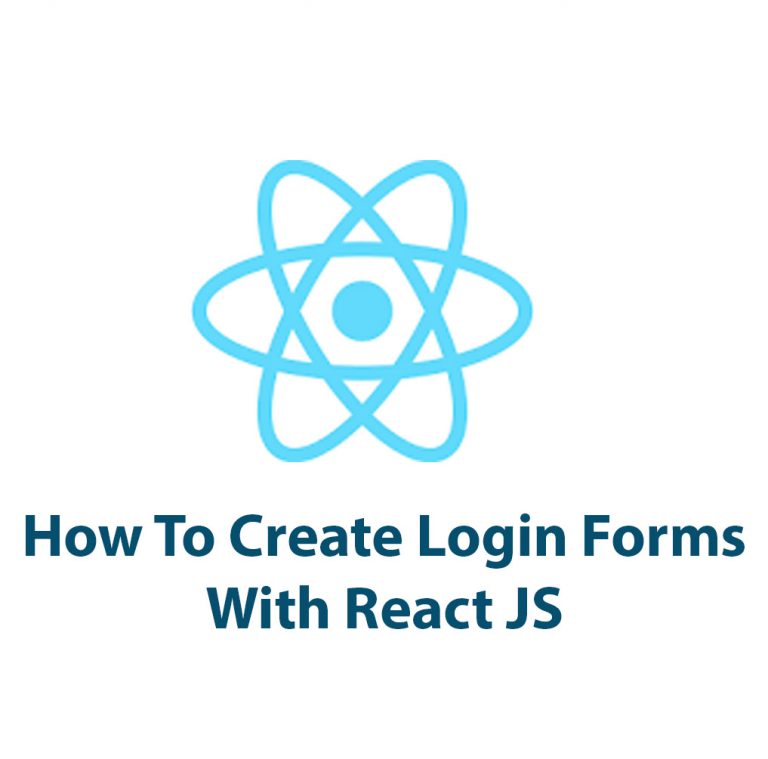
Create A Login Form In React
Creating a login form with React is an essential component in web development that can be accomplished in a few simple steps. In this article, we will walk through the process of creating a login form with React and explore its benefits. Before we begin, let’s take a moment to understand React. React is a […]
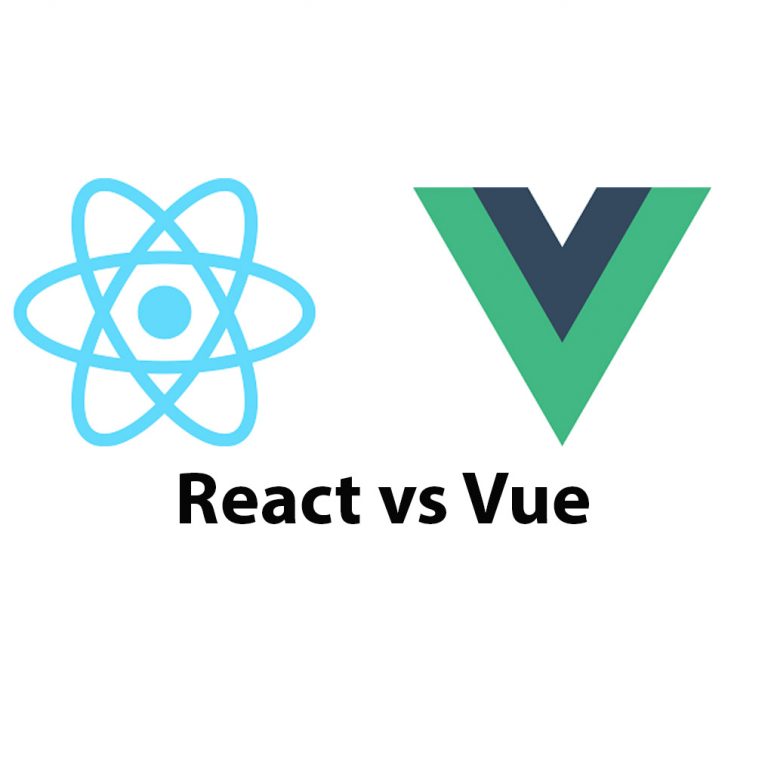
React JS vs Vue: A Comprehensive Guide
Introduction ReactJS and Vue are two of the most popular JavaScript libraries used to develop dynamic user interfaces. These libraries have gained widespread popularity due to their flexibility, efficiency, and ease of use. ReactJS was developed by Facebook, and Vue was created by Evan You. In this article, we are going to evaluate and compare […]
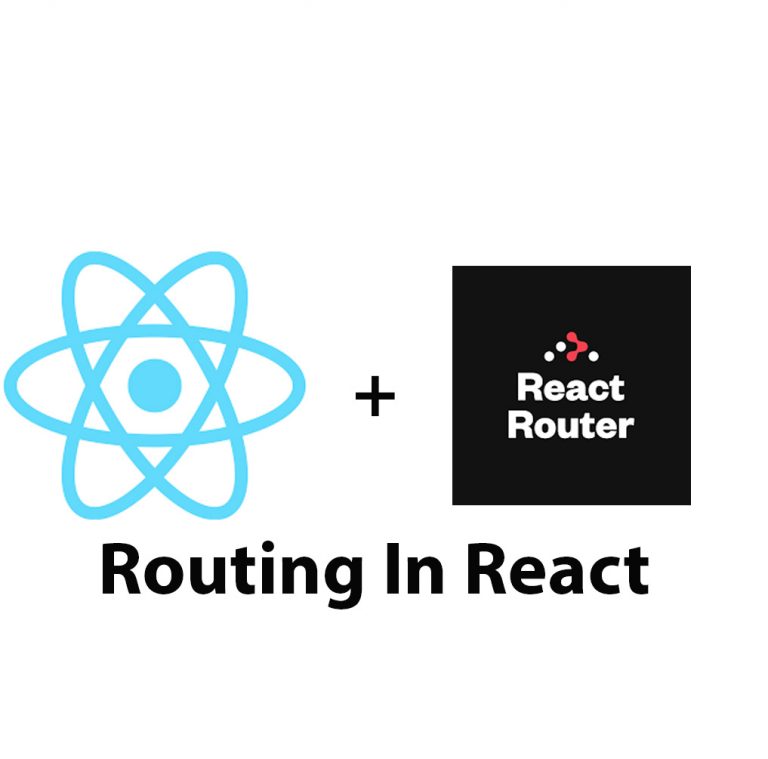
Routing In React JS Using React Router
Introduction React JS has become one of the most popular frontend frameworks for web development. It allows developers to create complex and dynamic user interfaces with ease. Routing is an essential part of web development, and React Router is one such library that is used to manage routing in React applications. In this article, we’ll […]
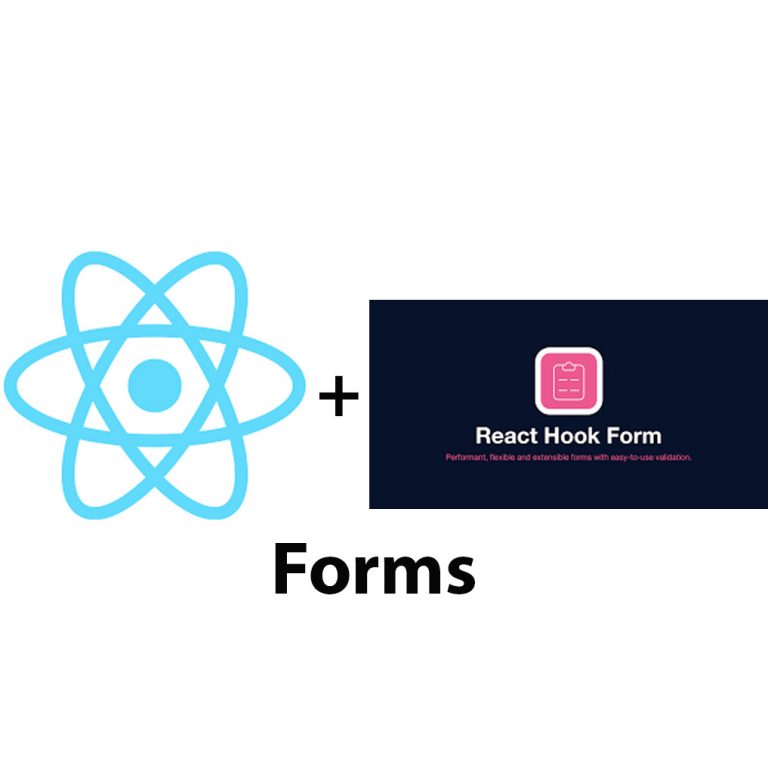
Create Forms In React Using React Hook Form
Introduction React Hook Form is a popular tool used in building forms in React.js. It is a performant library that simplifies the process of building forms by reducing boilerplate code and providing convenient features. This article is aimed at introducing the basic concepts of React Hook Form and how to use it to create forms […]