Introduction
Have you ever had to work on a React application and found yourself dealing with different environments or sensitive data? Setting up environment variables can make your life easier and provide a safer way to store information that should not be accessible to the public.
In this article, we’re going to look at how to use environment variables with React. You’ll learn what environment variables are, why they are important for React applications, and how to set them up. We will also cover best practices for working with environment variables, such as keeping sensitive information secure and testing them properly.
Setting Up Environment Variables
Before diving into how to use environment variables in React, we need to go over how to set them up.
- Installing and Configuring dotenv
The dotenv package is an npm package that allows us to read variables specified in a .env file into Node.js’ process.env
.
To use the package, install it via npm.
npm install dotenv
After installing, create and configure a .env file in your project directory. The dotenv configuration does not automatically occur in a React application, so you’ll need to configure dotenv yourself at the beginning of your application.
- Create A .env File
The .env file is where you’ll keep all the environment variables that your application requires. Each variable should have a name and a value separated by an equals sign. The variable name can be uppercase or lowercase, but it’s a common practice to write them in uppercase letters. Syntactically, there should be no spaces on either side of the equals sign.
Here’s what a basic .env file will look like:
SERVER_URL=http://localhost:8080/
APP_SECRET=S3cr3t!
The above file contains two variables: SERVER_URL and APP_SECRET. The server URL and application secret are essential for managing React applications that might need to communicate with backend API services or use sensitive information like passwords or API keys.
- Configure Environment Variables in Different Environments
It’s common to have different configurations for your applications in development, testing, and production environments. Fortunately, the dotenv package makes it easy to manage your React application’s configuration for these different environments.
To configure dotenv for different environments, you can create separate .env files, one for each environment. For instance, you’ll have a .env.development file for development environments and a .env.production file for production environments.
To use the configuration files differently during deployment, you should use your CI/CD pipeline or your hosting solution to set the proper value to the NODE_ENV
environment variable.
Using Environment Variables in React
Now that you have your environment variables set up, it’s time to use them in your React application. We’ll look at the different methods to inject environment variables at build time and access them via a component.
- Injecting Environment Variables at Build Time
One way of using environment variables is to build your React application with the proper environment configuration. To do so, you’ll need to rely on a module bundler like Webpack to inject them at build time.
Webpack provides a method of inserting the environment variables from your .env file into your code through its EnvironmentPlugin.
Here is an example of setting the node in the webpack.config.js
file to production:
const webpack = require("webpack");
const dotenv = require("dotenv");
dotenv.config();
module.exports = {
...
plugins: [
new webpack.EnvironmentPlugin(['NODE_ENV', 'SERVER_URL'])
]
...
}
By passing an array of environment variables required during build time, the values from .env
automatically get injected into your React application’s production build.
- Accessing Environment Variables Through process.env
Using process.env
in your React code helps access the environment variables defined in your .env file at runtime. This technique is usable for both client or server-side applications.
Since Create-React-App automatically configures Webpack for our React application, assigning environment variables in our React code is as simple as importing them using process.env
.
Here’s a simple example of using an environment variable in a component:
import React from 'react';
function App() {
const serverUrl = process.env.SERVER_URL;
return (
<div className="App">
<h1>Server URL: {serverUrl}</h1>
</div>
);
}
export default App;
In this example, we can access the SERVER_URL
value defined in our .env file using process.env.SERVER_URL
.
Note that when we refer to an environment variable’s value with the process.env.VARIABLE_NAME
syntax, the value we get is a string. Thus, if you want to get a boolean or integer value, you’ll need to use JSON.parse()
or parseInt()
.
- Implementing Environment Variables in React Components and Modules
Incorporating environment variables in React can be a breeze if you follow the best practices. It’s important to remember that React’s design promotes separating component logic from component definition. Therefore, we must apply these principles to implement environment variables in React components, including modules and service files.
The approach is simple. You can create a configuration module that exposes the necessary variables to all modules that need them.
Here’s a basic example of a configuration module:
// config.js
const SERVER_URL = process.env.REACT_APP_SERVER_URL || 'http://localhost:3000';
export { SERVER_URL };
You can make available a set of variables expected to be present in a React environment. In the example above, we provided a default value of http://localhost:3000
if however, the SERVER_URL environment variable is not present.
With this, you can now import your configuration file in your React components and use the values during runtime.
import {SERVER_URL} from './config';
console.log(SERVER_URL);
Best Practices for Working with Environment Variables
When working with environment variables, several best practices will help keep your React application secure, and running efficiently.
- Keeping Sensitive Information Secure
Environment variables can include sensitive data such as API keys, user credentials, or other data that should not be public. It’s best practice to keep them secure by storing them in a .env file not included in the version control system or accessible to the public.
- Organizing Environment Variables Effectively
It is tempting to have many environment variables with descriptive names, but over time, this could cause confusion and improperly update values. A better strategy is to investigate which variables are absolutely necessary, and organize them in
a logical manner. Naming conventions can be helpful, such as prefixing variable names with the name of the application or environment.
- Testing Environment Variables and Avoiding Common Mistakes
When managing environment variables, it’s essential to test and validate them regularly. Some common mistakes to avoid include misspelling variable names, using improper syntax for files, and incorrect path definitions. Regularly testing environment variables in a testing environment will ensure that they are working correctly and that any issues are caught before deployment.
Conclusion
In summary, environment variables are an essential part of a developer’s toolkit when working with React applications. They allow us to manage different environment configurations, securely store sensitive information and keep our applications running smoothly.
In this article, we’ve explored what environment variables are, why they are important for React applications, and how to set them up. We’ve also looked at different ways to inject them into our React application and how to use them via the process.env
syntax. We’ve also discussed best practices for working with environment variables and how to avoid common mistakes.
With this knowledge, you should be able to use environment variables effectively and safely in your React applications. Whether you’re building a personal project or working on a team, environment variables will help you keep your application secure and properly configured for different environments.
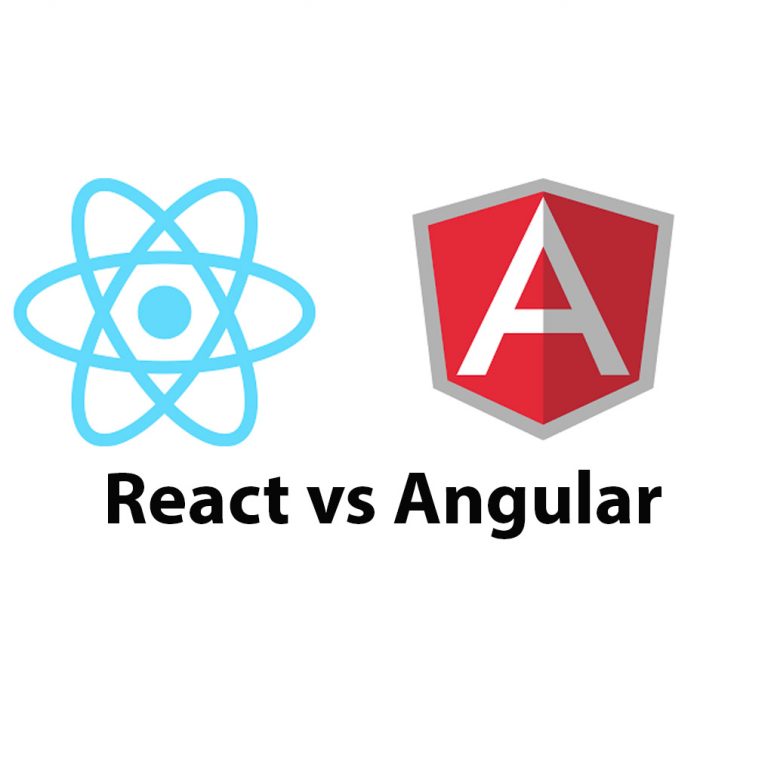
React JS vs Angular: A Comprehensive Guide
Introduction Web development has come a long way since the earlier days of static HTML pages. Web applications have become more dynamic, interactive and more like native applications. This transformation has brought about the rise of JavaScript frameworks and libraries, which have become essential in building web applications. However, choosing between these libraries or frameworks […]
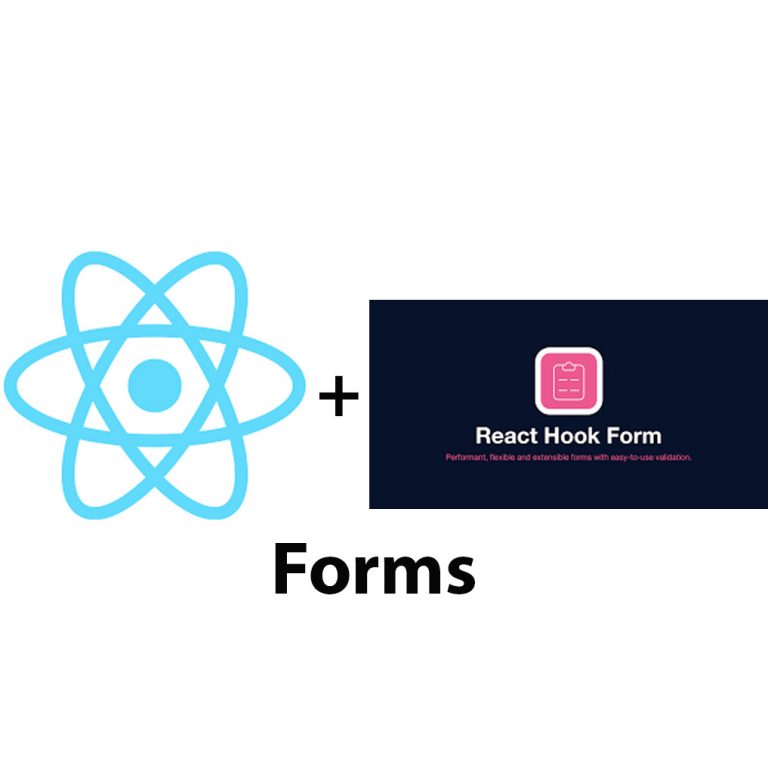
Create Forms In React Using React Hook Form
Introduction React Hook Form is a popular tool used in building forms in React.js. It is a performant library that simplifies the process of building forms by reducing boilerplate code and providing convenient features. This article is aimed at introducing the basic concepts of React Hook Form and how to use it to create forms […]
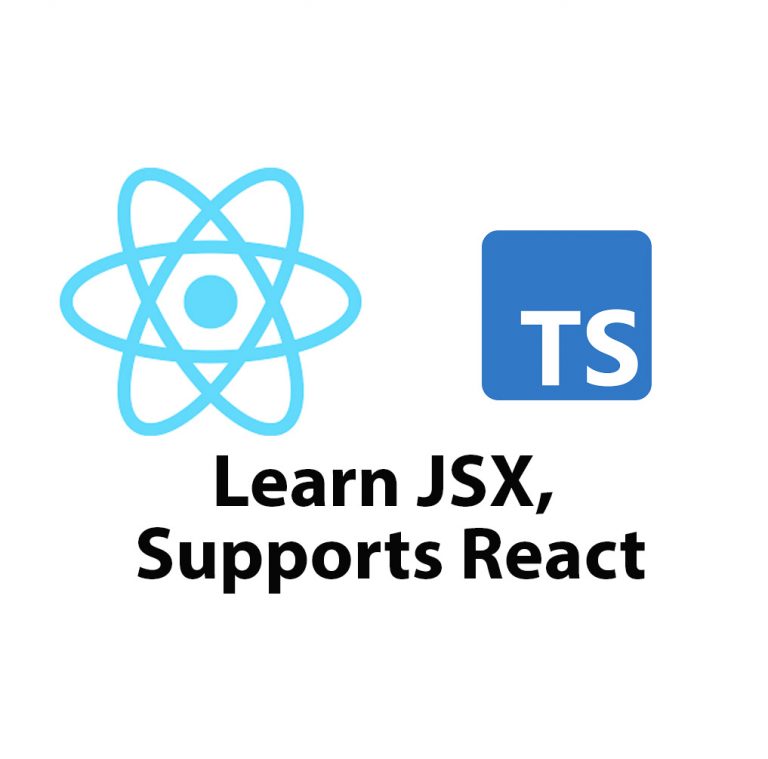
What Is JSX and how to use it.
Introduction As a web developer, I’m always on the lookout for new tools and technologies that can help me write better code faster. One tool that has caught my attention lately is Typescript JSX. In this article, I’m going to share my experience learning Typescript JSX and the benefits it has brought to my development […]
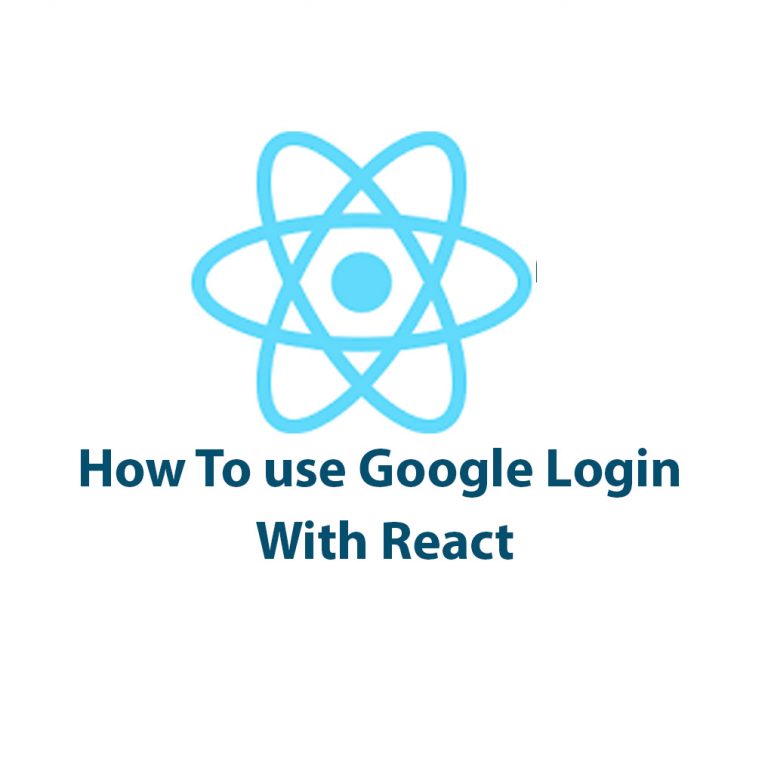
How To Use Google Login With React
As a developer, I cannot stress enough the importance of creating a seamless user experience when it comes to authentication. One of the most popular and convenient ways to allow users to authenticate is by using Google Login. In this article, we’ll explore how to use Google Login with React. Before we delve deep into […]
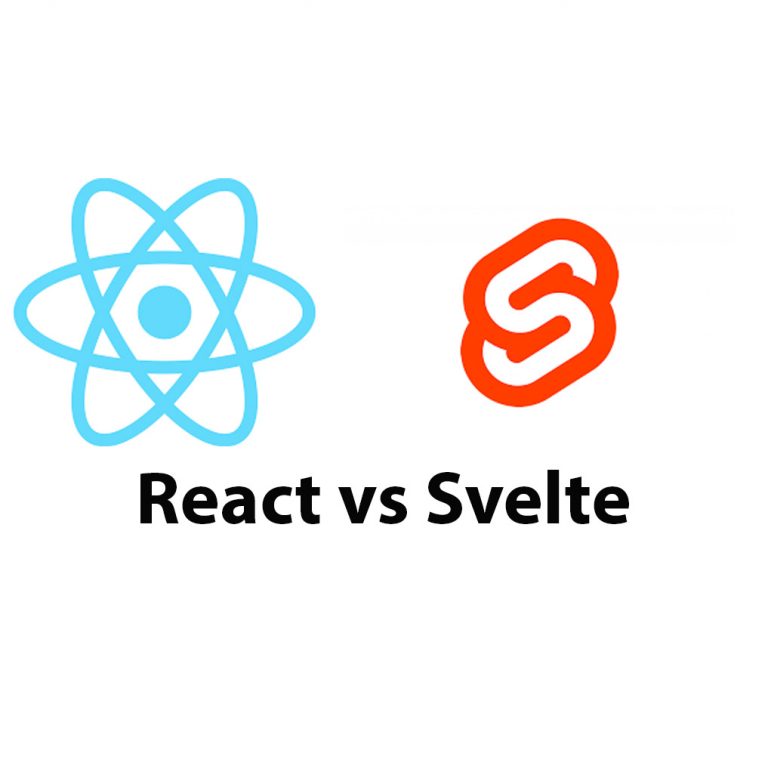
React JS vs Svelte
Introduction In the world of web development, there are many frameworks available for use. Two of the most popular frameworks are React JS and Svelte. React JS is an open-source JavaScript library developed by Facebook while Svelte is a relatively new framework developed by Rich Harris in 2016. In this article, we will compare React […]
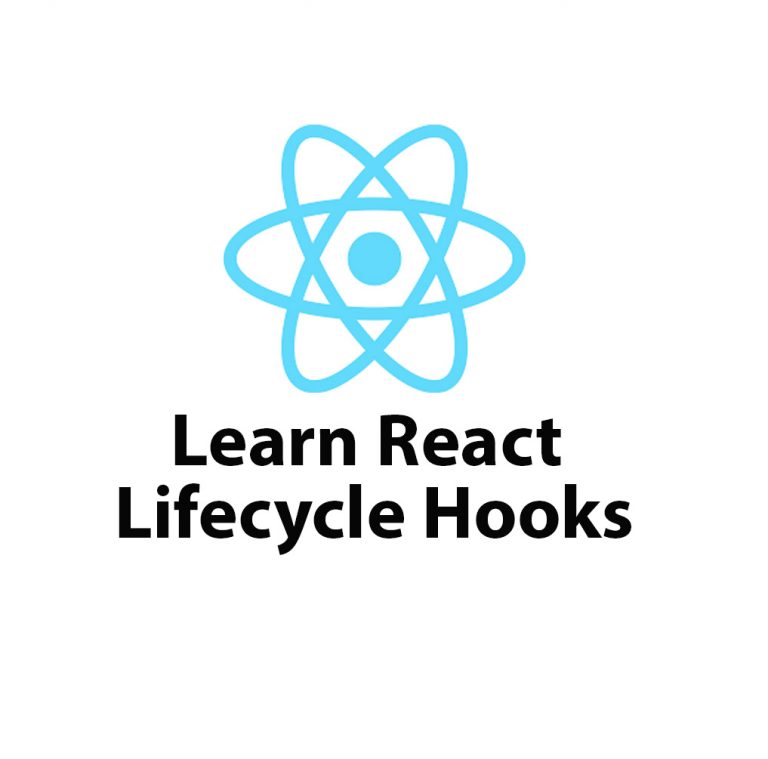
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]