Introduction:
Hey, developers! A very potent tool that has recently become more and more well-liked among developers is TypeScript. I’m here to demonstrate how to use TypeScript with Svelte, a dependable and effective web app framework, so you can take use of all the amazing capabilities that TypeScript has to offer.
Let me quickly explain what TypeScript and Svelte are before we get down to the nitty-gritty. Because TypeScript extends JavaScript with new capabilities like static typing, which enables quicker and safer development, it is known as a superset of JavaScript. Svelte, on the other hand, is a framework that turns your code into highly efficient and effective online apps.
I. Getting Started with TypeScript and Svelte:
Before we start, make sure that you have Node.js installed on your computer. To begin, let’s install Svelte. Open your terminal and type the following command:
npx degit sveltejs/template my-svelte-app
This command will download the latest Svelte starter files and extract them into a new directory named my-svelte-app
.
Next, change your directory to my-svelte-app
with the following command:
cd my-svelte-app
Now we can add TypeScript to our project. First, install the Svelte TypeScript Preprocessor with this command:
npm install --save-dev svelte-preprocess
Next, install TypeScript:
npm install --save-dev typescript
Finally, add a tsconfig.json
file to the root of your project directory with the following command:
npx tsc --init
Inside the tsconfig.json
file, change the target
property to "es2017"
, and save the changes.
II. Writing Component in TypeScript with Svelte:
Let’s get our hands dirty and start building some components. First, let’s check out what a Svelte component looks like without TypeScript. Here’s an example:
<!-- App.svelte -->
<script>
let name = '';
function handleInput(event) {
name = event.target.value;
}
</script>
<h1>Hello {name}!</h1>
<input type="text" on:input={handleInput}>
This is a very basic Svelte component that renders an input field and a heading that displays the value of the input.
To use TypeScript with Svelte, we need to make sure our component file has a .svelte.ts
or .svelte.d.ts
extension. Let’s rename the above file to App.svelte.ts
and add some TypeScript code.
<!-- App.svelte.ts -->
<script>
let name: string = '';
function handleInput(event: InputEvent) {
const element = event.target as HTMLInputElement;
name = element.value;
}
</script>
<h1>Hello {name}!</h1>
<input type="text" on:input={handleInput}>
All we have done here is added a type definition for the variable name
and the argument in the event handler function. In the handleInput
function, we need to use type casting to get the correct type from the target
property. Building components with TypeScript in Svelte is that easy!
III. Svelte Store with TypeScript:
Svelte stores provide a way for components to hold reactive data that is only updated when needed. Stores can be an essential part of a Svelte application, and using TypeScript makes them even more precise.
Here’s an example of how to create a store in Svelte with TypeScript:
<!-- CounterStore.ts -->
import { writable } from 'svelte/store';
export interface CounterStoreType {
count: number;
increment: () => void;
decrement: () => void;
}
export function counterStore(): CounterStoreType {
const { subscribe, set, update } = writable(0);
function increment() {
update(value => value + 1);
}
function decrement() {
update(value => value - 1);
}
return {
increment,
decrement,
subscribe,
get count() {
return subscribe(value => value);
}
};
}
In this code, we’ve created a new Counter Store that has a count property and two methods to increment and decrement the count value. The store is then returned as an object with the methods and count property.
IV. Advanced Features of TypeScript in Svelte:
TypeScript decorators are a really powerful tool that allows us to add extra functionality to our components. Here’s an example of how to use a TypeScript decorator in Svelte:
<!-- WithClassName.ts -->
import { SvelteComponent } from 'svelte';
export function withClassName<T extends new (...args: any[]) => SvelteComponent>(component: T) {
return class extends component {
className: string = '';
constructor(...args: any[]) {
super(...args);
}
$on(eventName: string, callback: () => void) {
this.className = 'my-class';
super.$on(eventName, callback);
}
};
}
In this code, we’ve created a TypeScript decorator function that adds a className property to our Svelte component and sets it to “my-class” in the $on
method of our component. Now, every time a component that uses this decorator is initialized, it will automatically have a className property set to “my-class”.
V. Debugging Techniques:
Debugging TypeScript in Svelte can be challenging at times, especially when dealing with complicated components. Here are some debugging techniques that you can use:
- Use the
debugger
statement. Place thedebugger
statement anywhere in your code and run your app. When JavaScript execution hits that line, the browser will stop execution and allow you to inspect variables and step through your code. - Use the
console.log
statement. Console logging is a very basic way of debugging your code. Placeconsole.log
statements anywhere in your code to help you track down issues. - Use a TypeScript editor that supports debugging. Depending on your editor, there will be a wide array of debugging tools available to you, including setting breakpoints and inspecting variables.
Conclusion:
Folks, there you have it! a thorough tutorial on using TypeScript with Svelte. The fundamentals of getting started, creating components, utilizing stores, advanced capabilities, and debugging have all been covered. Web apps can be created with the very potent TypeScript language and Svelte framework.
will be adjusted and perform even better.
It’s crucial to remember that TypeScript can seem intimidating at first. For this reason, it’s crucial to introduce TypeScript gradually, component by component, in order to grow accustomed to it.
Working with Svelte with TypeScript is ultimately a terrific experience that will advance your web development expertise. The paradigm shift can take some getting accustomed to, but it’s well worthwhile.
I appreciate your company while we explore TypeScript and Svelte. Continue your research, experimentation, and coding! Happy progress!
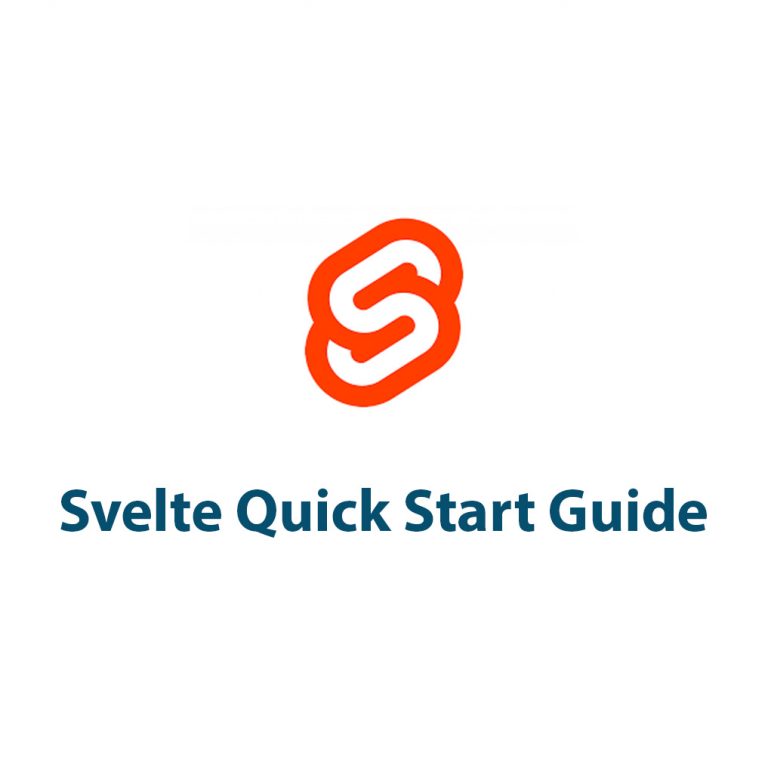
Svelte Quick Start Guide
Introduction Welcome to the Svelte universe! Look no further if you’re seeking for a fresh, lightweight framework for your upcoming web development project. Svelte is intended to be lightweight and simple to learn, while also making web development easier and more effective. I’ll walk you through a quick start tutorial for Svelte in this article […]
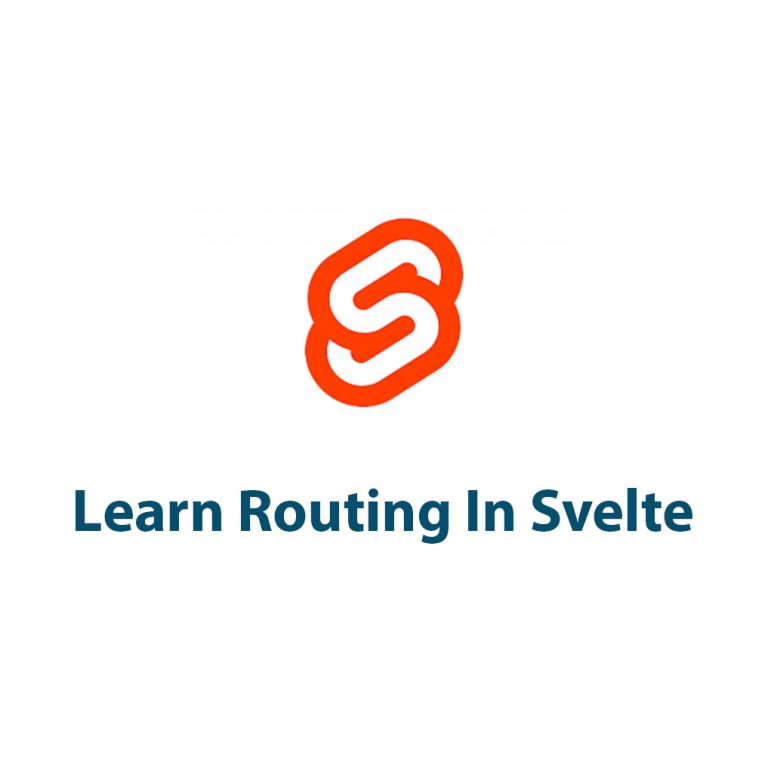
Svelte Routing Tutorial
Introduction Hello everyone! Today, we’re going to go deeply into routing, one of the key elements of web development. Have you ever navigated to a different page on the same website by clicking a link while you were on the same website? That is routing magic, my friend! Let me explain why routing is so […]
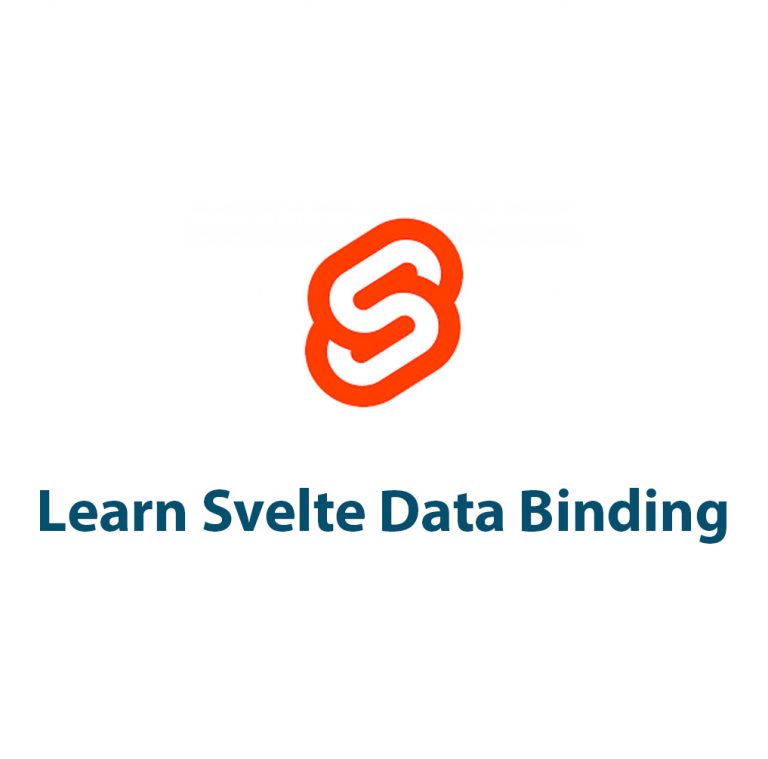
Svelte Data Binding Tutorial
Introduction Good day! Are you considering learning Svelte’s data binding? You’ve arrived at the proper location. We will cover all you need to know about data binding in Svelte in this article. Let’s start by discussing the fundamentals. Describe Svelte. Similar to React and Vue, it is a web framework that manages the development of […]