Introduction
Welcome to the Svelte universe! Look no further if you’re seeking for a fresh, lightweight framework for your upcoming web development project. Svelte is intended to be lightweight and simple to learn, while also making web development easier and more effective.
I’ll walk you through a quick start tutorial for Svelte in this article to help you get started. Along the way, I will share some tips and methods to help you code like a pro. We will cover everything from installation to deployment.
Installation and Setup
Svelte needs to be set up on our computer before we can begin using it. It’s quick and easy, which is fantastic news.
Making ensuring Node.js is installed on your machine should be your first priority. Once you have Node.js, you can use the npm package manager to install Svelte. Run the following command after opening your terminal:
npm install -g svelte
Svelte will be installed worldwide on your system as a result of this command, making it accessible from anywhere.
After installing Svelte, we must set up our development environment. Utilizing the slimline app template is the simplest way to get started. Let’s start by using the following command to create a new Svelte app:
npx degit sveltejs/template my-svelte-app
This will create a new Svelte app in a folder named my-svelte-app. Navigate to this folder by running the following command:
cd my-svelte-app
Now, let’s install the necessary dependencies by running the following command:
npm install
Basics of Svelte
Svelte’s component-based architecture is one of its many outstanding features. An independent piece of code called a component can be utilized repeatedly throughout your project.
A component is simple to make. Simply create a new file with any name you like in the src folder. Let’s make a component called MyComponent.svelte as an illustration.
To define our component in this file, we can utilize HTML, JavaScript, and CSS. Here is an illustration of what a component might resemble:
<script>
export let name;
</script>
<h1>Hello {name}!</h1>
<style>
h1 {
color: red;
}
</style>
This component will display a red header that says “Hello” followed by whatever name is passed to it.
To use this component elsewhere in your app, simply import it by adding the following line of code to your app.js file:
import MyComponent from ‘./MyComponent.svelte’;
After importing the component, you can use it in your HTML like this:
<MyComponent name="John" />
This will display the MyComponent with the name “John” passed to it.
Data Binding
One of the most powerful features of Svelte is data binding. Data binding allows you to keep your app in sync with the state of your data.
There are two types of data binding in Svelte: one-way and two-way. One-way data binding updates the state of your app when the data changes. Two-way data binding updates both the state and the data when either is changed.
Here’s an example of one-way data binding:
<script>
let count = 0;
function handleClick() {
count += 1;
}
</script>
<button on:click={handleClick}>
Clicked {count} times
</button>
This code defines a variable called “count” and a function called “handleClick” that increments the count when the button is clicked. The “onClick” attribute of the button binds the “handleClick” function to the click event of the button.
Svelte and CSS
Styling components in Svelte is easy. You can use CSS just like you would in any other web app. However, there is a difference in how styles are applied in Svelte.
In Svelte, styles are scoped to the component. This means that any styles you define in a component only apply to that component and its children.
Here’s an example of how you might style a component in Svelte:
<style>
h1 {
color: red;
}
</style>
<h1>Hello World!</h1>
This code defines a style for the h1 element that sets the font color to red. Because the style is defined inside the component, it is scoped to that component.
Svelte and Routing
Svelte makes it easy to implement routing in your app. The @sveltejs/kit library provides a simple and efficient way to handle routes.
To use routing, first, you need to install the @sveltejs/kit library. You can do this by running the following command:
npm install –save-dev @sveltejs/kit
After installing the library, you can add routes to your app by adding a routes.js file to your src folder. Here’s an example of what a routes.js file might look like:
import { load } from '@sveltejs/kit';
export const routes = [
{
// Home page
name: 'index',
pattern: '/',
load: () => load('src/routes/index.svelte'),
},
{
// About page
name: 'about',
pattern: '/about',
load: () => load('src/routes/about.svelte'),
},
];
This code defines two routes: one for the home page and one for the about page. The “load” function loads the Svelte component associated with the route.
Deployment
It’s time to deploy your Svelte app once you’ve done developing it. Although there are many options, using Vercel is one of the simplest ways to launch a Svelte app.
Vercel is a cloud platform for serverless operations and static websites. It offers a free hosting package that includes SSL encryption, a custom domain, and automatic deployment from Git.
You must to register for a Vercel account before you can deploy your Svelte app there. You can connect your Git repository to Vercel once you have an account. Every time you push changes to your repository, your app will automatically deploy.
Conclusion
Congratulations! You completed the Svelte fast start manual. We went over everything, from setup to deployment, and I gave you some pointers on how to code like an expert along the way.
Even though this guide just scratches the surface of what Svelte can achieve, I hope it provided you a sense of how effective and potent it can be. You can create web apps more quickly and easily using Svelte.
more successfully than before. You can easily create scalable and maintainable web programs by utilizing Svelte’s component-based architecture, data binding, and routing features.
There are numerous online resources, including the official Svelte documentation, courses, and discussion boards, that can be used to continue learning Svelte. Investigate the various Svelte third-party libraries that are accessible if you want to expand the features and functionality of your project without having to start from scratch.
Never forget that learning a new technology requires practice, so don’t be hesitant to get started right away. Explore Svelte’s various features and try out various strategies to find which one suits your project the best.
Svelte is an effective and lightweight web development framework that can enable you to create scalable and maintainable web projects more quickly than previously. Svelte is unquestionably a tool worth investigating for your upcoming web development project because of its simple installation and setup, component-based architecture, data binding, routing functionality, and deployment choices. Coding is fun!
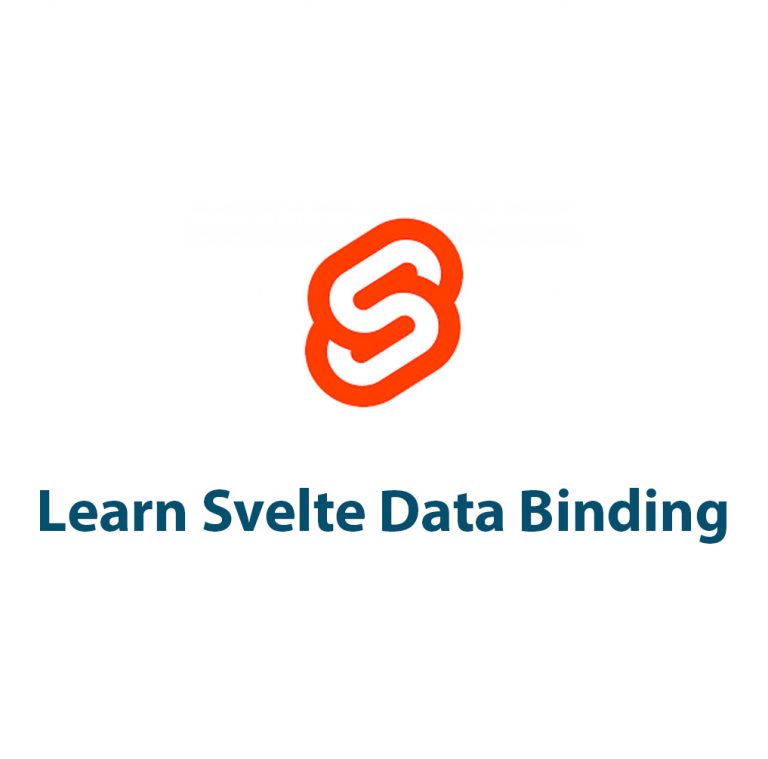
Svelte Data Binding Tutorial
Introduction Good day! Are you considering learning Svelte’s data binding? You’ve arrived at the proper location. We will cover all you need to know about data binding in Svelte in this article. Let’s start by discussing the fundamentals. Describe Svelte. Similar to React and Vue, it is a web framework that manages the development of […]
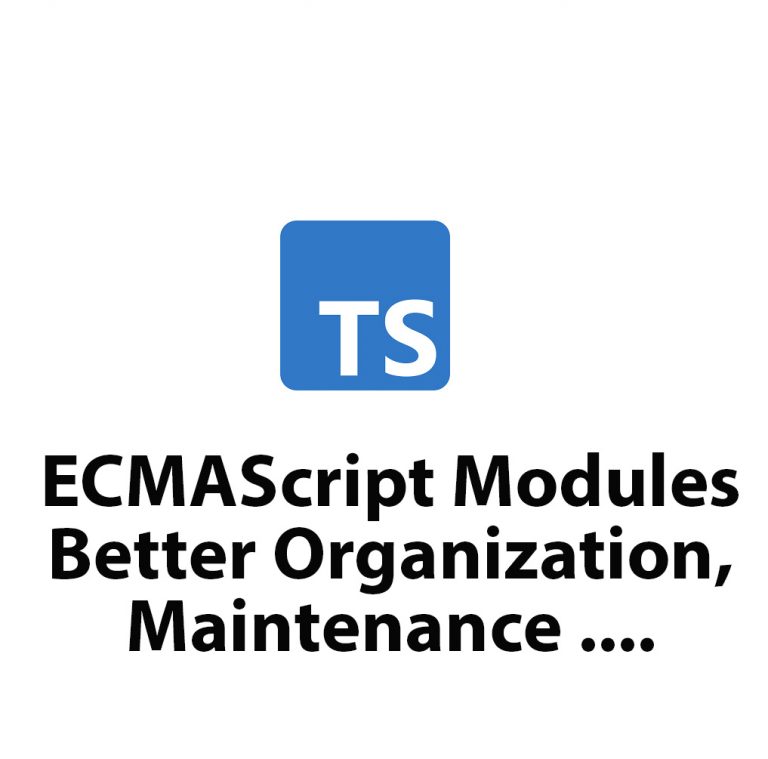
ECMAScript Modules in Node JS
As a software developer and avid Node JS user, I’ve always been on the lookout for ways to improve my workflow and simplify code maintenance. One of the most recent additions to Node JS that has greatly helped me achieve these goals is the implementation of ECMAScript (ES) Modules. ES Modules are a standard format […]
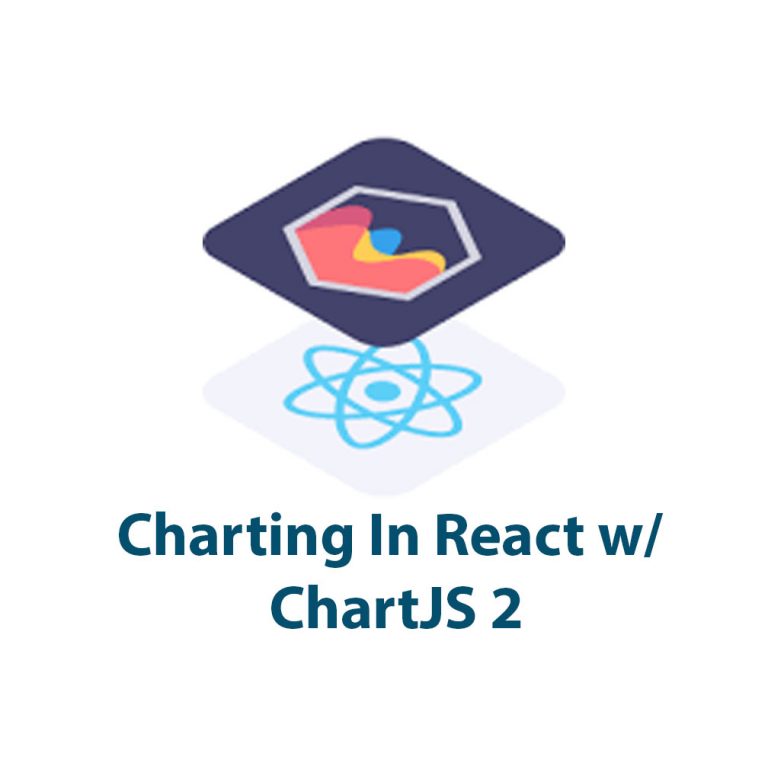
React Charts With ChartJS 2
As a web developer, I understand the importance of data visualization in presenting complex information in an understandable and visually appealing way. That’s why I’ve been using ChartJS 2, a powerful JavaScript library, for creating charts in my React apps. In this article, I’ll show you how to set up and use ChartJS 2 in […]
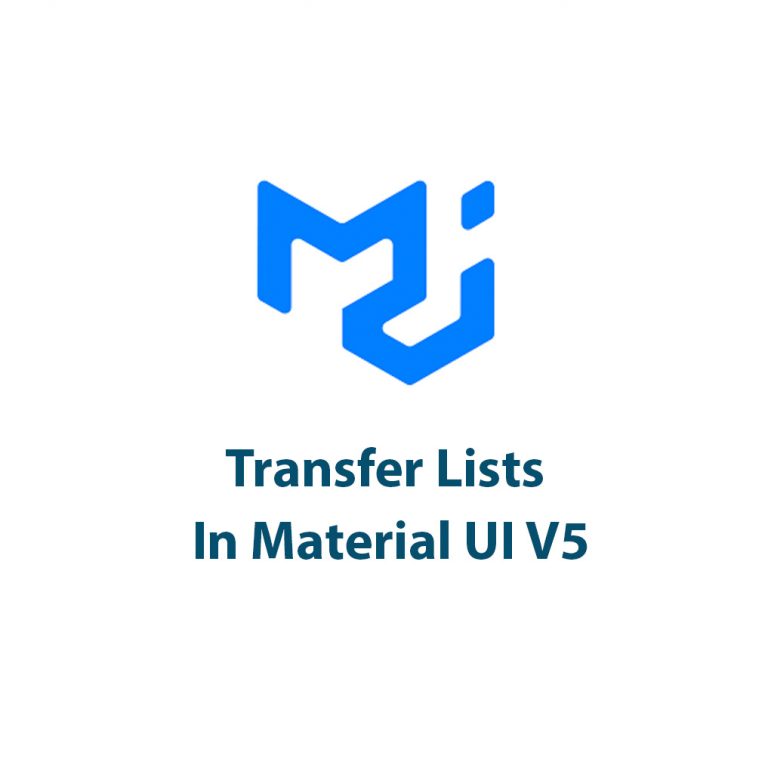
Transfer Lists In Material UI V5
Introduction I remember the first time I stumbled upon transfer lists while working on a project. I was perplexed by the concept, but as I delved deeper, I realized the tremendous benefits of using transfer lists in web development. With the release of Material UI v5, the developers have made it even easier to incorporate […]
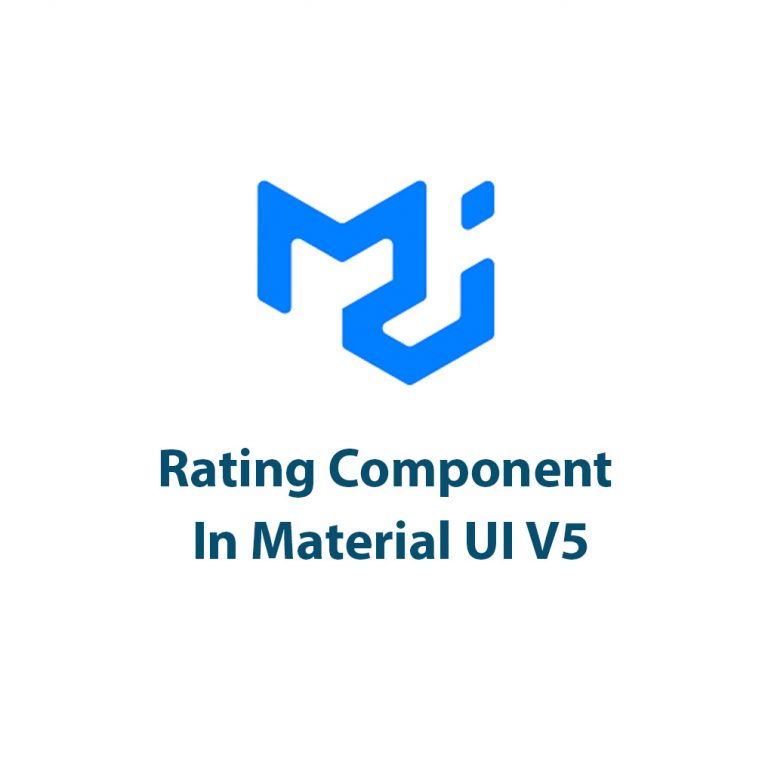
Rating Component In Material UI V5
Hello and welcome to this exciting article about the Rating Component in Material UI V5! As a web developer, I have come to really appreciate the simplicity and flexibility that this UI library provides, especially when it comes to components that add interactivity to user interfaces. In this article, I’m going to walk you through […]
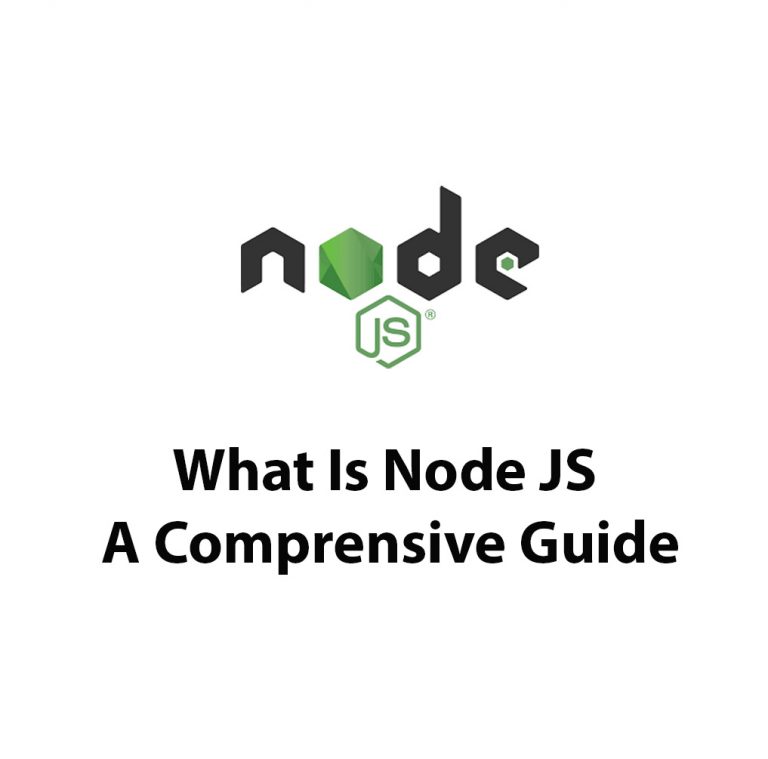
What Is Node JS: A Comprehensive Guide
Introduction As a full-stack developer, I have been working with various technologies over the past few years. But one technology that has caught my attention recently is NodeJS. With its event-driven and non-blocking I/O model, NodeJS has become an excellent choice for building real-time and highly-scalable applications. So, what is NodeJS, and how does it […]