Introduction
Hello everyone! Today, we’re going to go deeply into routing, one of the key elements of web development. Have you ever navigated to a different page on the same website by clicking a link while you were on the same website? That is routing magic, my friend!
Let me explain why routing is so important in web development before we get started. Routing allows us to load various web pages as separate pages while still providing our users with a smooth navigation experience. When it comes to web applications, where dynamic content must be loaded on the same page, this distinction becomes even more crucial.
We will introduce you to routing in Svelte in this post and give you a detailed how-to manual to get you started.
Getting Started with Routing in Svelte
We must first construct a fundamental Svelte project before we can begin using routing in Svelte. Don’t worry if you’re new to Svelte. It’s a great structure that’s simple to understand and effective to implement. The default routing solution for Svelte applications, Svelte Router, needs to be installed and configured after we have set up our fundamental Svelte project.
Route parameters, connections, nested routes, and other capabilities are among the many that Svelte Router offers. Of course, there are additional routing options that can be used with Svelte, but for the purposes of this guide, we’ll just utilize Svelte Router.
Creating routes in Svelte is a breeze with Svelte Router. To create a new route, all we need to do is add it as a child component of a router component. For example, suppose you want to create a new route for a blog post with the URL segment /blog/post-id
. In that case, you can define it in your Svelte Router component as follows:
<Route path="/blog/:postId" component={BlogPost}/>
Here, BlogPost
is a Svelte component that will be displayed when the user navigates to /blog/post-id
.
You might have noticed the :postId
section in the path. This segment is called a route parameter and enables us to pass dynamic data to the component we want to render. We’ll dive deeper into this topic in the next section.
Navigating Between Routes
Now that we’ve got the basics of routing in Svelte covered, let’s talk about how to navigate between routes. Svelte Router comes equipped with a handy Link
component we can use to create clickable links that take users to different routes.
Here’s an example of how to use the Link
component:
<Link to="/blog/post-1">Go to Blog Post 1</Link>
When the user clicks the link, they will be taken to the /blog/post-1
route, rendering the component we defined earlier.
If we want to handle navigation programmatically, we can use Svelte Router’s navigate
function. This function lets us navigate to a new page programmatically.
import { navigate } from 'svelte-router-spa';
function navigateToBlog() {
navigate('/blog');
}
You might also want to restrict user access to certain pages or even authenticate them before allowing them to access a page. This is where navigation guards come in handy. We can use navigation guards to protect our routes in a way that prevents unauthorised user access.
Handling Dynamic Routes
Route parameters in Svelte Router allow us to pass data to components that are rendered with a specific route. Data that’s needed to retrieve information from an API, database, or local storage can be passed as route parameters.
When defining a route with route parameters, we can specify the parameter using a leading colon followed by the parameter name (e.g., /:paramName
).
<Route path="/blog/:postId/name/:authorName" component={BlogPost}/>
In the above example, we pass two parameters to the BlogPost
component, “postId” and “authorName”, which we can access in the component’s props.
export let postId;
export let authorName;
let post;
onMount(async () => {
post = await fetchPost(postId, authorName);
});
Now, when a user navigates to /blog/3/name/John+Doe
, the BlogPost
component receives postId=3
and authorName='John Doe'
in its props.
Building Nested Routes
Nested routes are useful when we want to specify different routes for different sections of an application. With Svelte Router, we can do this easily by defining a Router
component that contains child Route
components.
<Router>
<Route path="/blog" component={Blog}/>
<Route path="/about" component={About}/>
</Router>
When the user navigates to /blog
, the Blog
component is rendered, and similarly, when the user navigates to /about
, the About component is rendered.
Of course, you can also nest routes within other routes, allowing you to create deeply nested routes if that is required.
Optimising Routing in Svelte
Our online apps should load pages instantly. This also holds true for routing. Thankfully, Svelte Router has capabilities that enable us to maximize routing performance.
One such feature is lazy loading. When a route is lazy-loaded, the JavaScript and other resources are not loaded until the user clicks the link to the route. By doing this, we may prevent our application from loading resources that a user might never utilize.
We may use our resources even more effectively thanks to the preloading function in Svelte Router. Preloading makes navigation instantaneous by obtaining the resources required for a route’s component before the user even accesses the route.
Additionally, we can cache commonly used routes to further speed up loading.
Advanced Routing Techniques with Svelte
With the help of Svelte Router’s even more sophisticated functions, we can work wonders with routing.
We may construct user interface (UI) elements that are uniform across numerous routes by using route layouts. Web apps frequently share UI components like footers and navigation. We can avoid having to develop these UI elements from scratch for each route by defining route layouts.
Users may occasionally view an outdated URL that is no longer valid. We may send such requests to a different URL using Svelte Router’s route aliases and redirects, preventing pointless 404 errors and improving user experience.
Another cutting-edge method that can greatly improve performance and SEO is server-side rendering. We can render application content on
Taking less time to load and show the content is to retrieve it from the server and send it back to the client as HTML.
Conclusion
That’s all, everyone! We sincerely hope you enjoyed reading this in-depth introduction about routing in Svelte. You ought to be able to develop advanced routing solutions for your web projects by now that you have a firm grasp of how Svelte’s routing system operates.
To create online apps that offer a seamless and responsive user experience, keep in mind that routing is crucial. Due to its extensive feature set and user-friendly interface, Svelte Router is a great option for enabling routing in Svelte applications.
Thank you for reading, and good luck with your web application development!
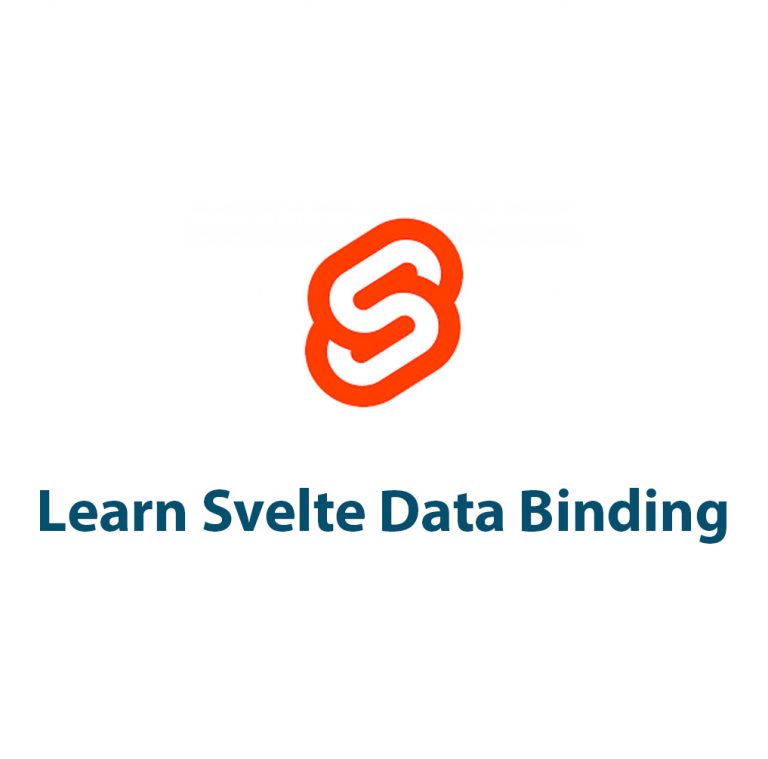
Svelte Data Binding Tutorial
Introduction Good day! Are you considering learning Svelte’s data binding? You’ve arrived at the proper location. We will cover all you need to know about data binding in Svelte in this article. Let’s start by discussing the fundamentals. Describe Svelte. Similar to React and Vue, it is a web framework that manages the development of […]
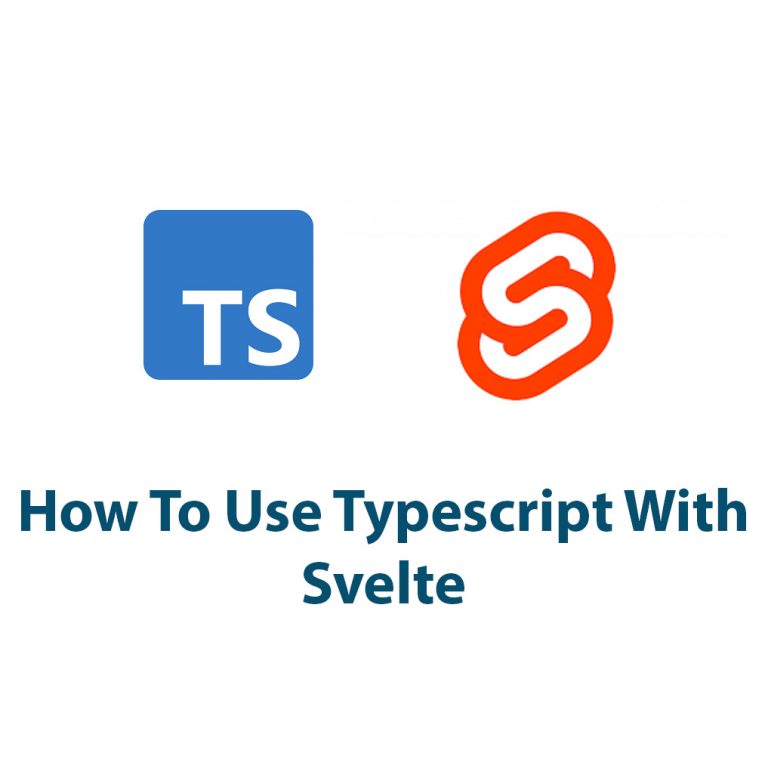
How To Use Typescript With Svelte
Introduction: Hey, developers! A very potent tool that has recently become more and more well-liked among developers is TypeScript. I’m here to demonstrate how to use TypeScript with Svelte, a dependable and effective web app framework, so you can take use of all the amazing capabilities that TypeScript has to offer. Let me quickly explain […]
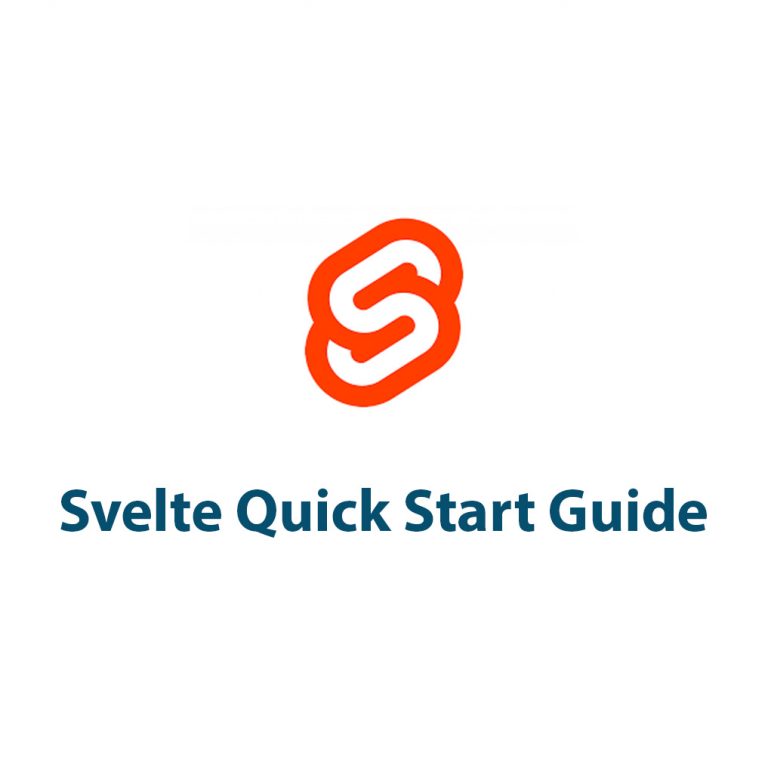
Svelte Quick Start Guide
Introduction Welcome to the Svelte universe! Look no further if you’re seeking for a fresh, lightweight framework for your upcoming web development project. Svelte is intended to be lightweight and simple to learn, while also making web development easier and more effective. I’ll walk you through a quick start tutorial for Svelte in this article […]