Introduction
Material UI V5 is a popular, open-source library that provides pre-built UI components, themes, and styles for building user interfaces with React. Data tables are a common component used in web development to display data in a tabular format. In this article, I’ll show you how to use Material UI V5 to create stylish and functional data tables for your web applications.
Getting Started with Material UI V5
Before we dive into creating data tables, let’s first set up a new project with Material UI V5. To get started, we’ll need to install the Material UI V5 library through npm. Open up your terminal or command prompt and navigate to your project’s directory. Then, run the following command:
npm install @mui/material @emotion/react @emotion/styled
Once the installation is complete, we can set up a new project with Material UI V5. Create a new file called App.js
and add the following code:
import React from 'react';
import { Button } from '@mui/material';
function App() {
return (
<div>
<Button variant="contained">Click me</Button>
</div>
);
}
export default App;
In this example, we’ve imported the Button
component from Material UI V5 and used it in our App
component. With this starter code in place, we should see a basic button rendered on the page.
Creating Data Tables with Material UI V5
Now that we have our project set up with Material UI V5, let’s move on to creating data tables. Material UI V5 provides several components that can be combined to create a functional and attractive data table.
Choosing the Appropriate Components
The Table
component is the core component for creating data tables in Material UI V5. It provides the table structure and basic functionality such as sorting and pagination. We’ll also need to use the TableBody
, TableCell
, and TableRow
components to display the actual data in the table.
Setting up Data Sources
Before we can display data in our data table, we need a source of data. For this example, we’ll create an array of objects containing some sample data. Add the following code to your App
component:
const rows = [
{ id: 1, name: 'John Smith', email: '[email protected]', phone: '123-456-7890' },
{ id: 2, name: 'Jane Doe', email: '[email protected]', phone: '555-555-5555' },
{ id: 3, name: 'Bob Johnson', email: '[email protected]', phone: '555-123-4567' },
];
In this example, we’ve created an array of three objects, each representing a row of data in our table. Each object has an id
property and several other properties representing the fields we want to display in our table.
Configuring Tables with Basic Functionality
With our data source set up, we can now create our data table. Replace the code in your App
component with the following:
import React from 'react';
import { Table, TableBody, TableCell, TableContainer, TableHead, TableRow, Paper } from '@mui/material';
const rows = [
{ id: 1, name: 'John Smith', email: '[email protected]', phone: '123-456-7890' },
{ id: 2, name: 'Jane Doe', email: '[email protected]', phone: '555-555-5555' },
{ id: 3, name: 'Bob Johnson', email: '[email protected]', phone: '555-123-4567' },
];
function App() {
return (
<TableContainer component={Paper}>
<Table>
<TableHead>
<TableRow>
<TableCell>Name</TableCell>
<TableCell>Email</TableCell>
<TableCell>Phone</TableCell>
</TableRow>
</TableHead>
<TableBody>
{rows.map((row) => (
<TableRow key={row.id}>
<TableCell>{row.name}</TableCell>
<TableCell>{row.email}</TableCell>
<TableCell>{row.phone}</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</TableContainer>
);
}
export default App;
In this example, we’ve imported the necessary components from Material UI V5 and used them to create a simple data table. Inside the Table
component, we’ve created a TableHead
component with the column headers for our table. Inside the TableBody
component, we’ve used the map
function to iterate over our rows
array and create a <TableRow>
component for each row of data. Inside each TableRow
, we’ve used <TableCell>
components to display the data for each column.
Customizing Data Tables with Sorting, Filtering, and Pagination
Material UI V5 provides several built-in features that allow us to enhance our data tables with sorting, filtering, and pagination. Let’s take a look at how we can add these features to our table.
Sorting
To add sorting functionality to our table, we’ll need to use the TableSortLabel
component. This component displays a clickable label that allows users to sort the table by a particular column. Add the following code to your App
component, after the TableHead
component:
const [order, setOrder] = React.useState('asc');
const [orderBy, setOrderBy] = React.useState('name');
const handleSortRequest = (property) => {
const isAsc = orderBy === property && order === 'asc';
setOrder(isAsc ? 'desc' : 'asc');
setOrderBy(property);
};
function EnhancedTableHead(props) {
const { order, orderBy, onRequestSort } = props;
const createSortHandler = (property) => (event) => {
onRequestSort(property);
};
return (
<TableHead>
<TableRow>
<TableCell>
<TableSortLabel
active={orderBy === 'name'}
direction={orderBy === 'name' ? order : 'asc'}
onClick={createSortHandler('name')}
>
Name
</TableSortLabel>
</TableCell>
<TableCell>
<TableSortLabel
active={orderBy === 'email'}
direction={orderBy === 'email' ? order : 'asc'}
onClick={createSortHandler('email')}
>
Email
</TableSortLabel>
</TableCell>
<TableCell>
<TableSortLabel
active={orderBy === 'phone'}
direction={orderBy === 'phone' ? order : 'asc'}
onClick={createSortHandler('phone')}
>
Phone
</TableSortLabel>
</TableCell>
</TableRow>
</TableHead>
);
}
function App() {
const [order, setOrder] = React.useState('asc');
const [orderBy, setOrderBy] = React.useState('name');
const handleSortRequest = (property) => {
const isAsc = orderBy === property && order === 'asc';
setOrder(isAsc ? 'desc' : 'asc');
setOrderBy(property);
};
return (
<TableContainer component={Paper}>
<Table>
<EnhancedTableHead order={order} orderBy={orderBy} onRequestSort={handleSortRequest} />
<TableBody>
{rows.map((row) => (
<TableRow key={row.id}>
<TableCell>{row.name}</TableCell>
<TableCell>{row.email}</TableCell>
<TableCell>{row.phone}</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</TableContainer>
);
}
export default App;
In this example, we’ve created a new component called EnhancedTableHead
that wraps our existing TableHead
component. Inside this component, we’ve used TableSortLabel
components to create clickable labels for each column header. When a user clicks on a label, the handleSortRequest
function is called with the property that corresponds to the clicked label. This function then sets the orderBy
and order
states appropriately. Finally, we pass these states and the handleSortRequest
function down to our EnhancedTableHead
component and use them to update our TableBody
component.
Filtering
To add filtering functionality to our table, we can use the TextField
component from Material UI V5. This component allows users to enter a search query and filters the table by matching the query against the data in each row. Add the following code to your App
component, after the TableContainer
component:
const [filter, setFilter] = React.useState('');
const handleFilterChange = (event) => {
setFilter(event.target.value);
};
const filteredRows = rows.filter((row) =>
row.name.toLowerCase().includes(filter.toLowerCase()) ||
row.email.toLowerCase().includes(filter.toLowerCase()) ||
row.phone.toLowerCase().includes(filter.toLowerCase())
);
return (
<TableContainer component={Paper}>
<TextField
label="Search"
variant="outlined"
margin="normal"
value={filter}
onChange={handleFilterChange}
fullWidth
/>
<Table>
<EnhancedTableHead order={order} orderBy={orderBy} onRequestSort={handleSortRequest} />
<TableBody>
{filteredRows.map((row) => (
<TableRow key={row.id}>
<TableCell>{row.name}</TableCell>
<TableCell>{row.email}</TableCell>
<TableCell>{row.phone}</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</TableContainer>
);
In this example, we’ve added a new state called filter
that represents the user’s search query. We’ve also added a new function called handleFilterChange
that updates the filter
state when the user types in the search box. We then use the filter
state to create a new filteredRows
array that contains only the rows that match the search query. Finally, we update our TableBody
component to use filteredRows
instead of rows
.
Pagination
To add pagination functionality to our table, we can use the TablePagination
component from Material UI V5. This component allows users to navigate through the pages of data in the table. Add the following code to your App
component, after the TableHead
component:
const [page, setPage] = React.useState(0);
const [rowsPerPage, setRowsPerPage] = React.useState(5);
const handleChangePage = (event, newPage) => {
setPage(newPage);
};
const handleChangeRowsPerPage = (event) => {
setRowsPerPage(parseInt(event.target.value, 10));
setPage(0);
};
return (
<TableContainer component={Paper}>
<TextField
label="Search"
variant="outlined"
margin="normal"
value={filter}
onChange={handleFilterChange}
fullWidth
/>
<Table>
<EnhancedTableHead order={order} orderBy={orderBy} onRequestSort={handleSortRequest} />
<TableBody>
{filteredRows.slice(page * rowsPerPage, page * rowsPerPage + rowsPerPage).map((row) => (
<TableRow key={row.id}>
<TableCell>{row.name}</TableCell>
<TableCell>{row.email}</TableCell>
<TableCell>{row.phone}</TableCell>
</TableRow>
))}
</TableBody>
<TablePagination
rowsPerPageOptions={[5, 10, 25]}
component="div"
count={filteredRows.length}
rowsPerPage={rowsPerPage}
page={page}
onPageChange={handleChangePage}
onRowsPerPageChange={handleChangeRowsPerPage}
/>
</Table>
</TableContainer>
);
In this example, we’ve added two new states called page
and rowsPerPage
that represent the current page and number of rows per page, respectively. We’ve also added two new functions called handleChangePage
and handleChangeRowsPerPage
that update these states when the user interacts with the pagination component. We then use these states to slice our filteredRows
array and display only the rows that should be visible on the current page. Finally, we add the TablePagination
component to our table, passing in the necessary states and functions and customizing the appearance and behavior of the pagination component.
Adding Tooltips and Icons to Data Tables
Material UI V5 provides several components that can be used to add tooltips and icons to our data table. Let’s take a look at how we can use these components to enhance our table.
Tooltips
To add tooltips to our table, we can use the Tooltip
component from Material UI V5. This component displays a small popup with additional information when a user hovers over a particular element. Add the following code to your App
component, inside the TableCell
components:
import { Tooltip } from '@mui/material';
<TableCell>
<Tooltip title="View details">
<IconButton>
<VisibilityIcon />
</IconButton>
</Tooltip>
</TableCell>
In this example, we’ve added a Tooltip
component around an IconButton
component that displays a VisibilityIcon
. When the user hovers over the icon, a tooltip with the text “View details” will appear.
Icons
To add icons to our table, we can use the Icon
component from Material UI V5. This component displays a small graphic or symbol that can be used to represent a particular action or piece of information. Add the following code to your App
component, inside the TableCell
components:
import { Icon } from '@mui/material';
<TableCell>
<Icon>
{row.favorite ? 'favorite' : 'favorite_border'}
</Icon>
</TableCell>
In this example, we’ve added an Icon
component that displays a heart icon if the row is marked as a favorite, and a heart outline if it is not. We use the ternary operator to choose the appropriate icon based on the favorite
property of the row object.
Conclusion
In this article, we’ve walked through the steps to create a data table with Material UI V5. We’ve covered how to add sorting, filtering, pagination, tooltips, and icons to our table, and provided code snippets for each feature. Material UI V5 provides a rich set of components and features that can help create clean, modern, and user-friendly data tables for your web applications. By following the examples in this article and exploring the Material UI V5 documentation, you too can create rich and interactive data tables that enhance the user experience of your application.
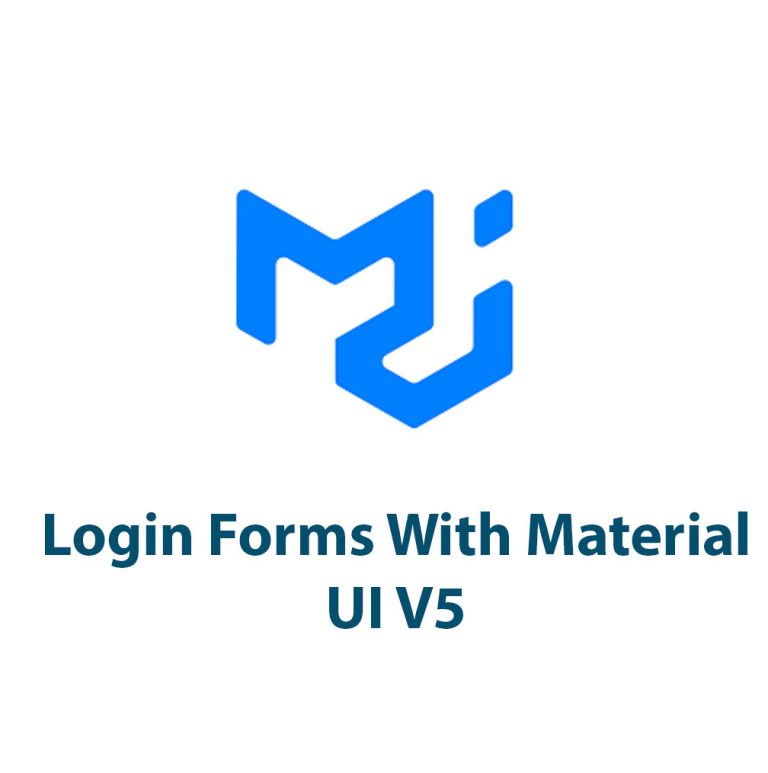
Create Login Forms With Material UI v5
Introduction As a web developer, I’m always on the lookout for efficient ways to create stunning user interfaces. That’s why I’m excited to explore Material UI V5, a library of React components that make it easy to build beautiful web apps. And in this article, I’m going to focus on one essential element of any […]
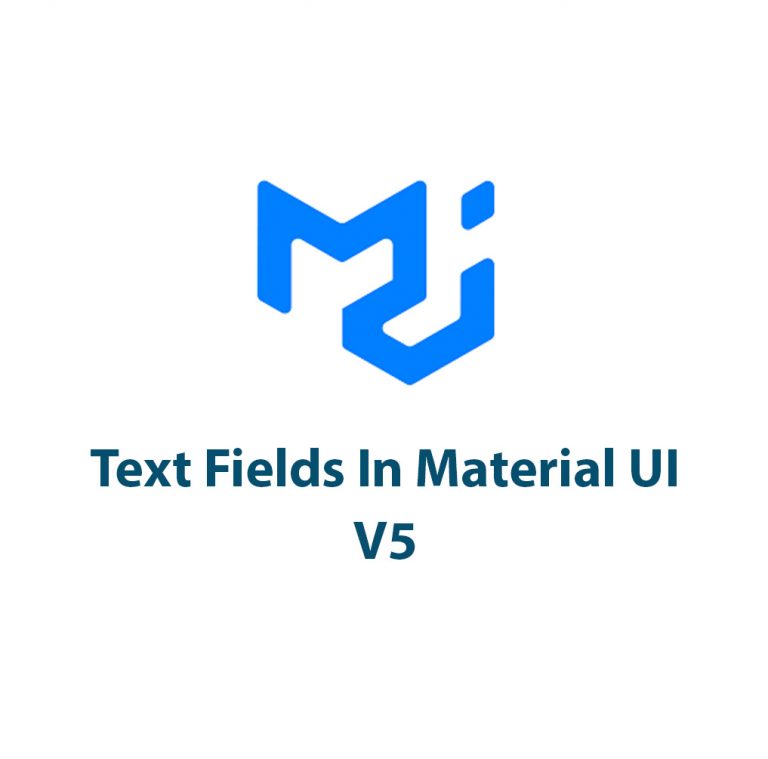
Text Fields In Material UI V5
I. Introduction As the popularity of Material UI as a user interface kit continues to rise, developers have found themselves working with text fields more often. Text fields are one of the most essential elements in any form and Material UI has made using them fun and straightforward.This article seeks to highlight the features, use, […]
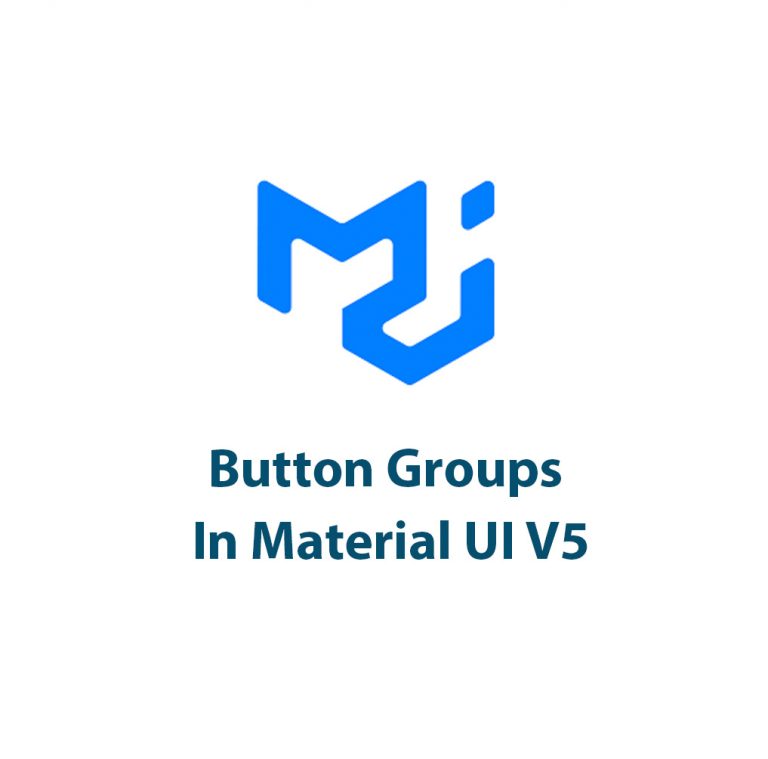
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
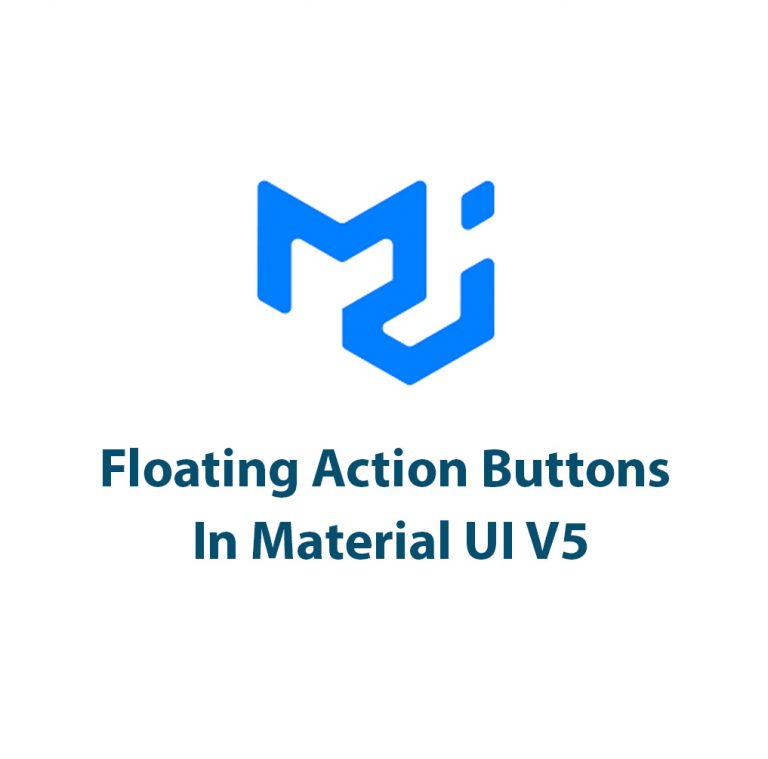
Floating Action Buttons In Material UI V5
Introduction Hey there fellow developers! Have you ever wanted to create a floating action button that seamlessly integrates with your project’s design? Well, look no further than Material UI V5 floating action buttons! Floating action buttons, or FABs, have become a popular design element in web design due to their ease of use and functionality. […]
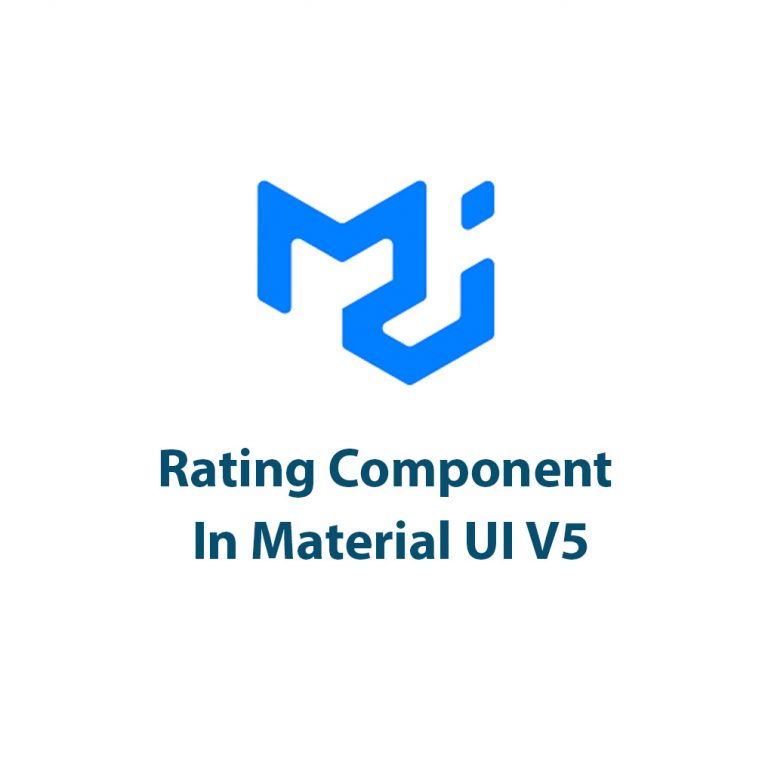
Rating Component In Material UI V5
Hello and welcome to this exciting article about the Rating Component in Material UI V5! As a web developer, I have come to really appreciate the simplicity and flexibility that this UI library provides, especially when it comes to components that add interactivity to user interfaces. In this article, I’m going to walk you through […]
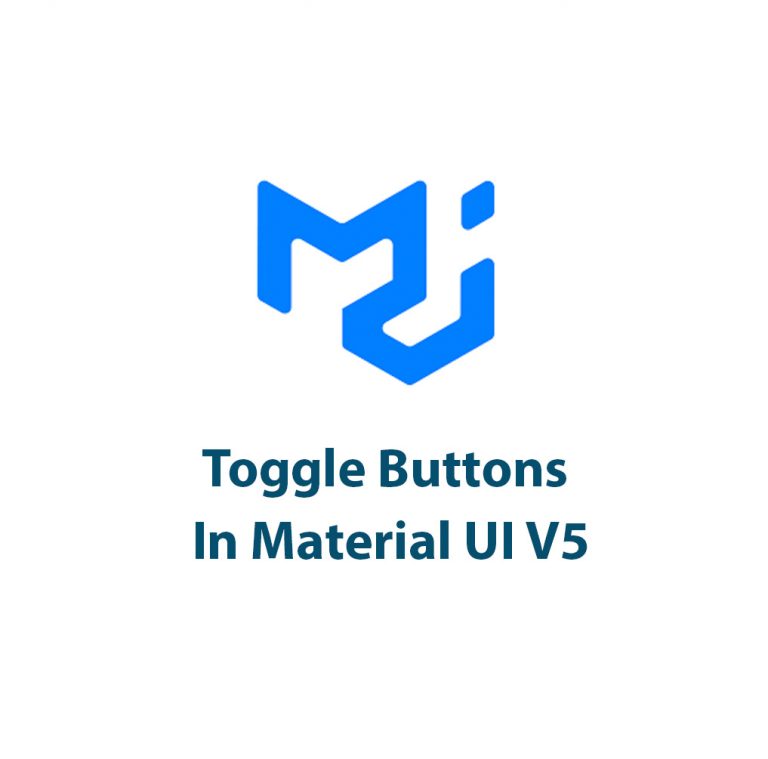
Toggle Buttons In Material UI V5
As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the […]