Introduction:
Popular React UI framework Material UI provides a large selection of pre-built components to easily build responsive user interfaces. The library’s design approach, which is based on Google’s Material Design principles, has helped it become more well-liked among developers. The design framework of Material UI is crucial to the creation of user interfaces since it promotes consistency across the entire program.
Version 5 of the Material UI library was just launched, and among the many new features is theming. Theming enables designers to adapt the design system to their specific requirements. I will introduce you to Material UI theming, discuss its significance in UI development, and describe how you may use it in your application in this article.
The Basics of Material UI Theming:
Theming is a technique for personalizing the design system and adding unique aesthetics to individual components. The @emotion/styled package is developed on top of the Material UI theming, which makes configuring the design system simple. You must construct a theme object with the unique styles and configurations you wish to employ in order to leverage Material UI theming.
The @material-ui/core/styles module’s createTheme() function can be used to create a theme object. The options object is passed to the createTheme() function as seen below:
import { createTheme } from '@material-ui/core/styles';
const theme = createTheme({
palette: {
primary: {
main: '#ff9800',
contrastText: '#ffffff',
},
secondary: {
main: '#26a69a',
},
},
});
The options object passed is used to create the theme object. In this example, we configure the primary and secondary colors by setting their main color and text color in the palette object.
Customizing a Theme:
You can adapt the design system to your requirements by choosing from a variety of modification choices provided by Material UI theming. We will talk about a few of the customization options offered by Material UI theming in this section.
Colors:
The usage of colors in UI design is crucial, and Material UI theming enables developers to alter the color scheme employed in the UI components. The palette object in the options object given to the createTheme() function can be used to change the color scheme.
const theme = createTheme({
palette: {
primary: {
main: '#ff9800',
contrastText: '#ffffff',
},
secondary: {
main: '#26a69a',
},
error: {
main: '#f44336',
},
warning: {
main: '#ffeb3b',
},
info: {
main: '#2196f3',
},
success: {
main: '#4caf50',
},
},
});
The palette object contains the primary, secondary, error, warning, info, and success colors. In this example, we overwrite the primary, secondary, error, warning, info, and success colors.
Typography:
Another crucial component of UI design is typography, and Material UI theming enables developers to alter the font employed in the UI components. The typography object in the options object given to the createTheme() function can be used to alter the typography.
const theme = createTheme({
typography: {
fontFamily: "'Roboto', sans-serif",
h1: {
fontSize: '2rem',
},
h2: {
fontSize: '1.5rem',
},
h3: {
fontSize: '1rem',
},
h4: {
fontSize: '0.875rem',
},
h5: {
fontSize: '0.75rem',
},
h6: {
fontSize: '0.625rem',
},
subtitle1: {
fontWeight: 'bold',
},
},
});
The typography object contains the font family and styles for all the typography variants. In this example, we set the font family to Roboto, and we customize the font size and weight for the various typography variants.
Spacing:
Spacing also plays a crucial role in UI design, and Material UI theming offers a way to configure the spacing used in the components. To customize the spacing, you can use the spacing object in the options object passed to the createTheme() function.
const theme = createTheme({
spacing: 8,
});
In this example, we set the spacing to 8, which means that a spacing value of 1 equals 8px.
Overrides:
Material UI theming also allows you to override the default styles of a component. To override the styles of a component, you can use the withStyles() higher-order component provided by @material-ui/core/styles.
import { withStyles } from '@material-ui/core/styles';
const styles = (theme) => ({
root: {
backgroundColor: theme.palette.primary.main,
color: theme.palette.primary.contrastText,
},
});
const CustomComponent = withStyles(styles)(({ classes }) => (
<div className={classes.root}>Custom Component</div>
));
In this example, we override the styles of a custom component and apply the primary color to the background color and the primary text color to the text color.
Responsive Design:
A key component of UI development is responsive design, and Material UI theming offers a method for making responsive components. The @material-ui/core/styles makeStyles() hook can be used to generate responsive components. By utilizing the @media query, the makeStyles() hook enables you to define styles based on the current screen size.
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles((theme) => ({
root: {
[theme.breakpoints.down('sm')]: {
backgroundColor: '#f00',
},
[theme.breakpoints.up('md')]: {
backgroundColor: '#0f0',
},
[theme.breakpoints.up('lg')]: {
backgroundColor: '#00f',
},
},
}));
const ResponsiveComponent = () => {
const classes = useStyles();
return <div className={classes.root}>Responsive Component</div>;
};
In this example, we use the makeStyles() hook to define styles based on the current screen size using the breakpoint API offered by Material UI.
Implementing Material UI Theming:
If you want to use icons in your application, you’ll also need to install the @material-ui/icons package in addition to the @material-ui/core package. Create a theme object with your unique styles and configurations once the packages have been installed.
import { createTheme } from '@material-ui/core/styles';
const theme = createTheme({
palette: {
primary: {
main:'#ff9800',
contrastText: '#ffffff',
},
secondary: {
main: '#26a69a',
},
error: {
main: '#f44336',
},
},
typography: {
fontFamily: "'Roboto', sans-serif",
h1: {
fontSize: '2rem',
},
},
spacing: 8,
});
In this example, we create a theme object that sets the primary, secondary, and error colors along with custom typography and spacing.
Once you have created the theme object, you need to use it in your application by wrapping the top-level component with the ThemeProvider component provided by @material-ui/core/styles.
import { ThemeProvider } from '@material-ui/core/styles';
const App = () => {
return (
<ThemeProvider theme={theme}>
<div>My Application</div>
</ThemeProvider>
);
};
In this example, we wrap the top-level component with the ThemeProvider component and pass the theme object we created as a prop to the component.
Upgrading to Material UI V5 Theming:
If you’re already using Material UI in your application, you might wonder about the differences between version 4 and version 5 of the library. In this section, we will compare Material UI V4 theming with Material UI V5 theming.
Material UI V4 Theming:
In version 4 of Material UI, theming was based on the createMuiTheme() function, which took an options object similar to the createTheme() function. However, the options object in Material UI V4 theming had different keys than the options object in Material UI V5 theming.
Material UI V5 Theming:
In version 5 of Material UI, theming is based on the createTheme() function, which takes an options object that contains the same keys as the options object in Material UI V4 theming. However, Material UI V5 theming introduces some new features, such as the ThemeProvider component and the color system.
Upgrading to Material UI V5 Theming:
You must update the packages to the most recent versions and modify your codebase in order to switch to Material UI V5 theming. Replace the MuiThemeProvider component with the ThemeProvider component, and change the color scheme to utilize the new color names provided by Material UI V5 theming. In particular, replace the createMuiTheme() function with the createTheme() function.
Conclusion:
We introduced Material UI theming and its significance in UI development to you in this article. We talked about the different ways you may personalize Material UI theming, including with colors, font, spacing, and overrides. Additionally, we provided guidance for improving your implementation of Material UI theming and described how to include it into your application.
We trust that this post has helped you gain a thorough understanding of Material UI theming and how it can enhance the user interface design system. You may design stunning, responsive user interfaces that are suited to your own requirements by using Material UI theming. Why not give it a shot then?
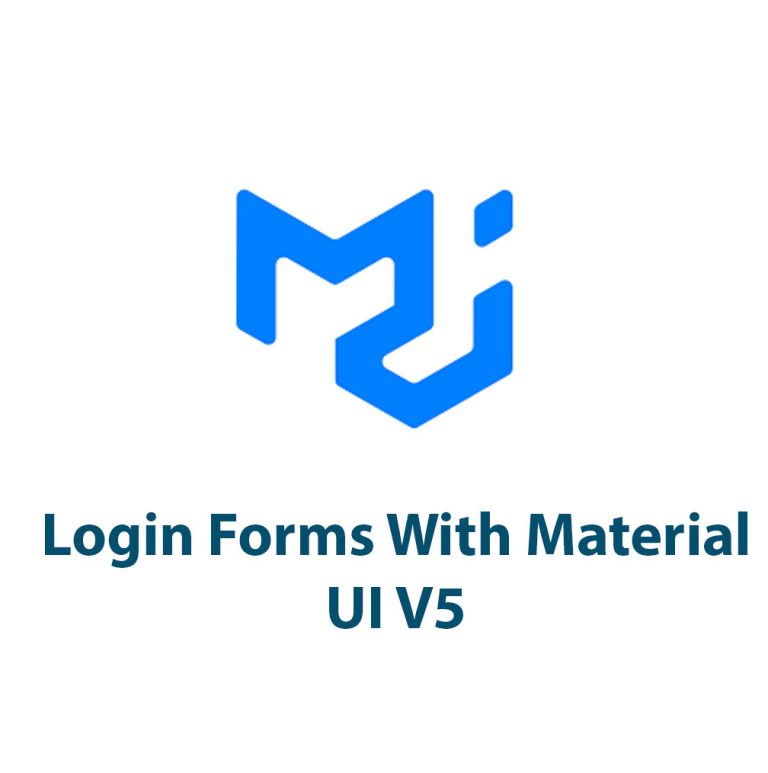
Create Login Forms With Material UI v5
Introduction As a web developer, I’m always on the lookout for efficient ways to create stunning user interfaces. That’s why I’m excited to explore Material UI V5, a library of React components that make it easy to build beautiful web apps. And in this article, I’m going to focus on one essential element of any […]
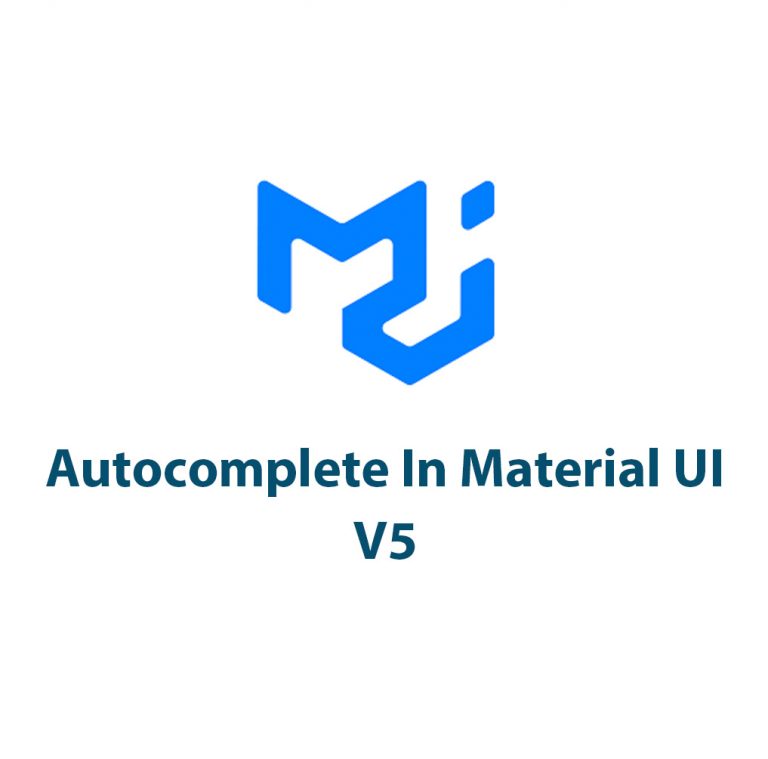
Autocomplete In Material UI V5
Introduction Hello everyone, today I am going to talk about one of the most useful components in Material UI V5 – Autocomplete. Material UI is a popular React UI framework that provides a wide range of pre-designed components to make the development of complex user interfaces simpler. Autocomplete is an intuitive component that provides suggestions […]
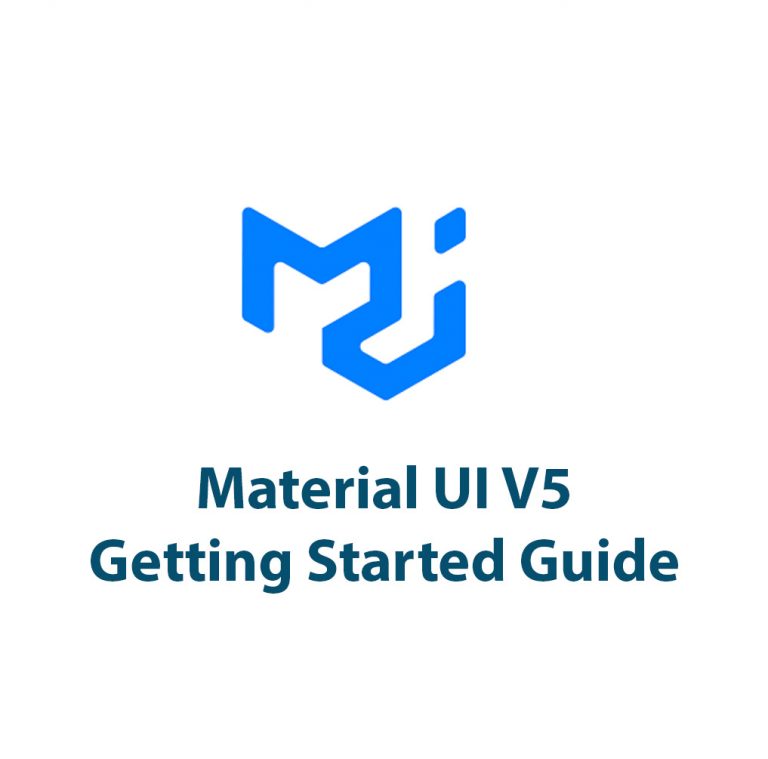
Material UI V5: Getting Started Guide
Introduction Hello, fellow online professionals! Are you prepared to explore the fascinating Material UI V5 world? You’ve come to the correct place if you’re trying to improve the appearance and feel of your online applications. The powerful framework that implements Google’s Material Design principles, Material UI V5, will be demonstrated to you step-by-step in this […]
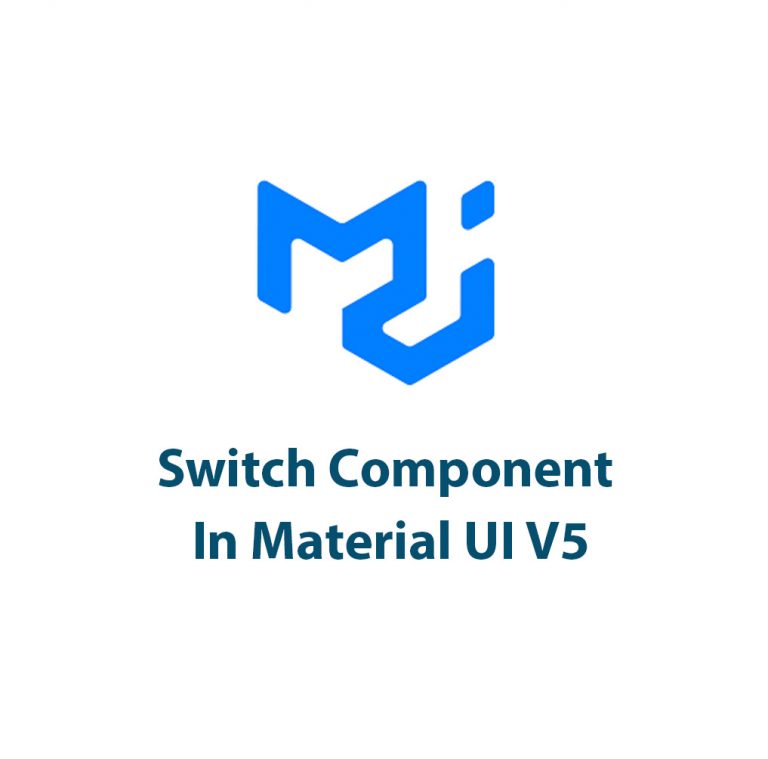
Switch Component In Material UI V5
Hey there, fellow developers! Are you excited about exploring the latest version of Material UI and all the new features it brings? If so, you’ve landed in the right place because today we’re going to dive deep into the Switch Component in Material UI v5. Introduction Before we get into the nitty-gritty of the Switch […]
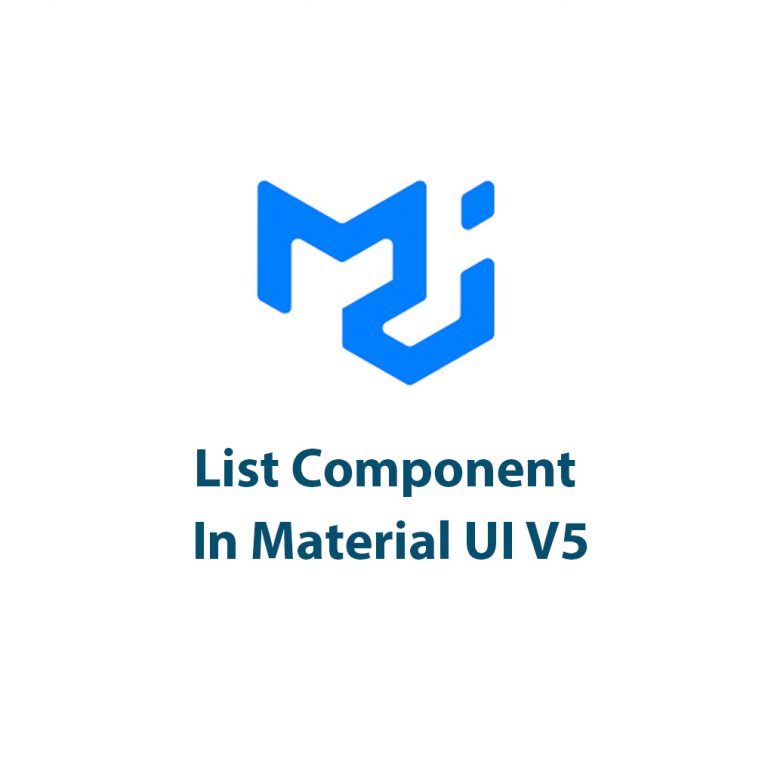
Lists In Material UI V5
Introduction As a UX designer and front-end developer, I’m always on the lookout for tools and libraries that make my job easier. When I first discovered Material UI, I was impressed by how it simplified UI development and improved the consistency of my designs. In this article, I want to focus specifically on lists in […]
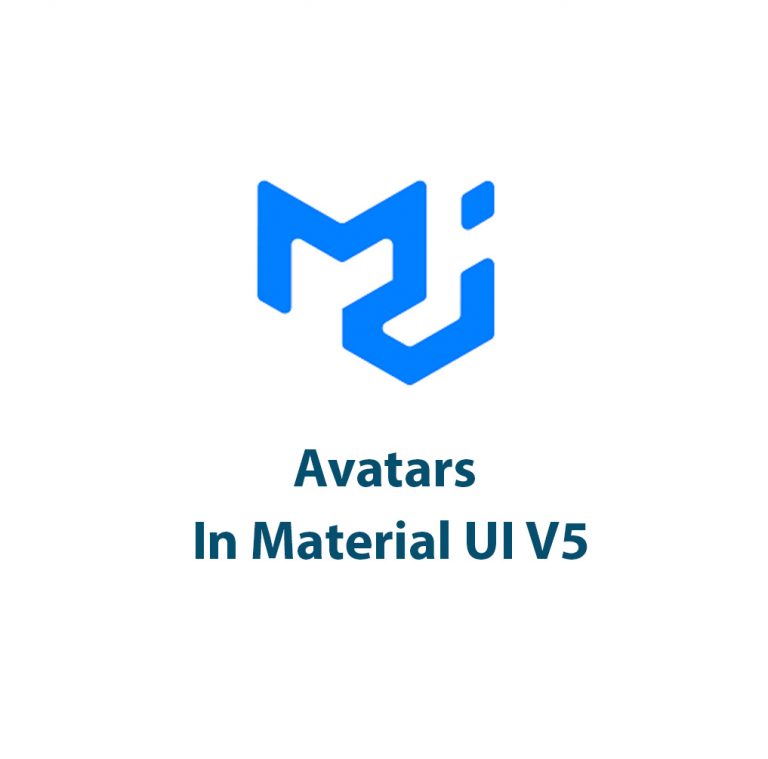
Avatars In Material UI V5
As a developer, I have always been fascinated by the way avatars enhance the user experience in web applications. They provide a personalized touch and make users feel more connected to the platform. That’s why I was excited to learn that Material UI V5 had revamped their avatar component. In this article, I’ll share my […]