As a developer, I cannot stress enough the importance of creating a seamless user experience when it comes to authentication. One of the most popular and convenient ways to allow users to authenticate is by using Google Login. In this article, we’ll explore how to use Google Login with React.
Before we delve deep into the “how-to,” let’s quickly take a look at what Google Login is and why it’s important. Google Login is a simple and secure way for users to log into web and mobile applications. When users log in with Google, they don’t have to worry about creating and remembering a new password for your app. It also saves time and reduces friction during the registration process.
Setup
Now, let’s get started with setting up a React app. If you don’t have create-react-app installed on your machine, go ahead and install it by running npm install -g create-react-app
. Next, create a new React project by running npx create-react-app google-login
. Finally, navigate into the newly created project by running cd google-login
and start your React app by running npm start
.
Creating a Google API Console Project
With the React app up and running, we need to create a new project in the Google API Console. If you don’t have a Google account, go ahead and create one because you’ll need it to create a new project. Once you’re signed in, navigate to the Google API Console and create a new project. Give your project a name and click the “Create” button.
Once your project is created, you’ll need to add the Google Login API. To do that, click on the “+ ENABLE APIS AND SERVICES” button and search for “Google Login API”. Click on it and then click on the “Enable” button.
Initializing Google Login
With the Google Login API enabled, we can start initializing Google Login in our React app. First, install the Google API client library by running npm install google-auth-library --save
. We’ll also need to create a client ID by navigating to the “Credentials” tab in the Google API Console and clicking on the “Create Credentials” button. Choose “OAuth client ID” from the dropdown and follow the instructions to create a new client ID.
Now that we’ve created the client ID, let’s authenticate users with Google Login. First, let’s create a googleLogin()
function that’ll handle the authentication process.
const { OAuth2Client } = require('google-auth-library');
const client = new OAuth2Client(process.env.CLIENT_ID);
async function googleLogin(req, res) {
const { token } = req.body;
try {
const ticket = await client.verifyIdToken({
idToken: token,
audience: process.env.CLIENT_ID,
});
const { name, email, picture } = ticket.getPayload();
res.status(200).json({ name, email, picture });
} catch (error) {
console.error(error);
res.status(401).send('Invalid authentication token');
}
}
module.exports = { googleLogin };
In this code, we’re first importing the OAuth2Client
class from the google-auth-library
. We’re also creating a new instance of OAuth2Client
and passing in our client ID as an environment variable. We’re then creating an async
function called googleLogin
that will handle the authentication process.
Handling Google Login in React
The googleLogin
function takes in a request object and a response object. It deconstructs the token
property from the req.body
object and verifies the ID token using our OAuth2Client
instance. If the token is valid, it extracts the user’s name, email, and picture from the token’s payload and returns them in the response object with a 200 status code. If the token is invalid, it returns a 401 status code with an error message.
With the googleLogin
function created, let’s add the Google Login button to our React component. Add the following code to your React component’s render()
function:
render() {
return (
<div>
<button onClick={this.handleGoogleLogin}>Log in with Google</button>
</div>
);
}
In this code, we’re adding a button with an onClick
event listener that will call the handleGoogleLogin
function when clicked.
We also need to create a state for Google Login. Add the following code to your React component’s constructor:
constructor(props) {
super(props);
this.state = {
user: null,
error: null,
};
this.handleGoogleLogin = this.handleGoogleLogin.bind(this);
}
In this code, we’re creating a state object that has two properties: user
and error
. The user
property is set to null
by default, while the error
property is also set to null
.
Now that we’ve created the state object, we need to update it when the user successfully logs in. Add the following code to your React component:
async handleGoogleLogin() {
const googleUser = await window.gapi.auth2.getAuthInstance().signIn({ scope: 'profile email' });
const token = googleUser.getAuthResponse().id_token;
try {
const response = await fetch('/api/google-login', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ token }),
});
const { name, email, picture } = await response.json();
this.setState({ user: { name, email, picture }, error: null });
} catch (error) {
this.setState({ user: null, error: error.message || 'Invalid authentication token' });
}
}
In this code, we first call window.gapi.auth2.getAuthInstance().signIn()
to prompt the user to log in with their Google account. Once the user logs in, we extract the ID token from the user’s auth response.
We then make a POST
request to our server-side googleLogin()
endpoint with the ID token as the request body. If the response is successful, we extract the user’s name, email, and picture from the response and set the user
property in our state object to an object with those values. If the response is unsuccessful, we set the error
property in our state object to the error message.
And that’s it! We’ve successfully created a Google Login button and handled the authentication process with React.
To recap, we started by setting up a React app using create-react-app. We then created a new project in the Google API Console and
added the Google Login API. We initialized Google Login by installing the Google API client library, creating a client ID, and authenticating users with Google Login with our googleLogin()
function.
We then added the Google Login button to our React component, created a state object for Google Login, and updated the state object when the user successfully logs in.
While we’ve covered the basics of how to use Google Login with React, keep in mind that there are many nuances and edge cases to consider. For example, you may want to handle the case where the user denies permission for your app to access their Google account. You may also want to handle the case where the user logs out of your app or logs out of their Google account.
To learn more about Google Login with React, I highly recommend reading the documentation for the Google APIs Client Library for JavaScript and the React documentation on handling events.
Conclusion
In conclusion, Google Login is a simple and convenient way to allow users to log into your web or mobile application without having to worry about creating and remembering a new password. Combining Google Login with React can create a seamless and user-friendly authentication process. By following the steps outlined in this article, you’ll be on your way to implementing Google Login with React in no time!
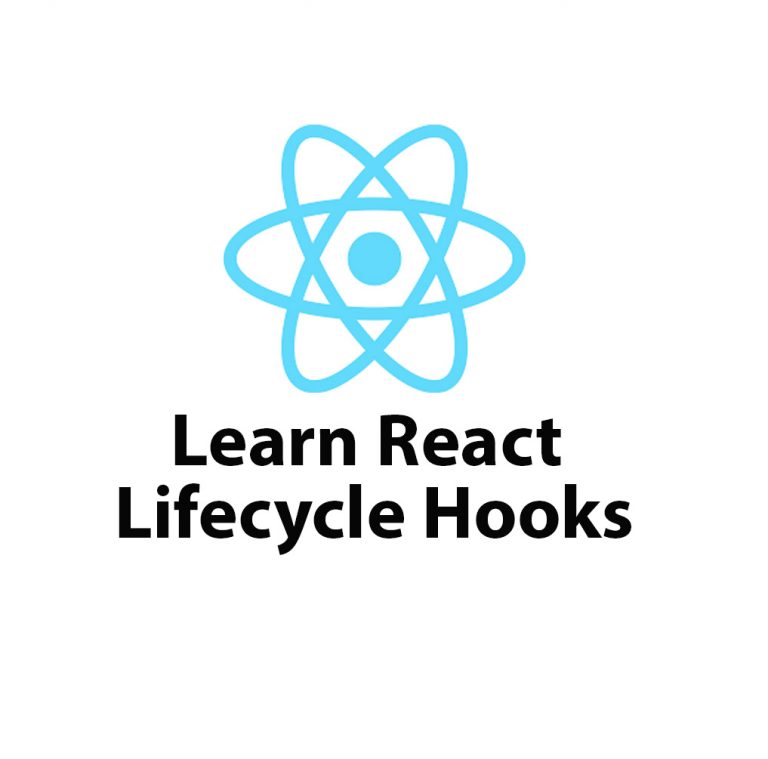
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]
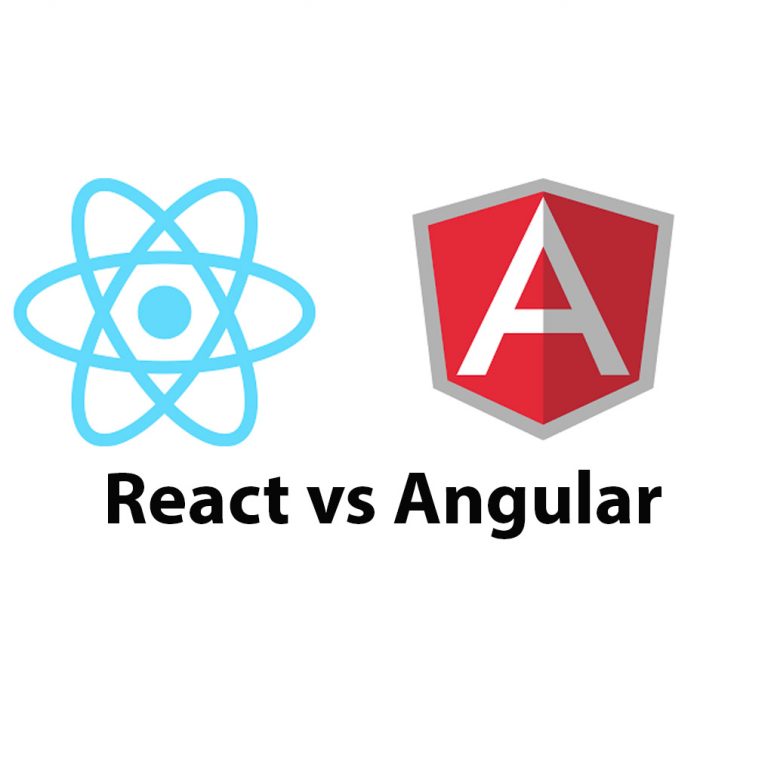
React JS vs Angular: A Comprehensive Guide
Introduction Web development has come a long way since the earlier days of static HTML pages. Web applications have become more dynamic, interactive and more like native applications. This transformation has brought about the rise of JavaScript frameworks and libraries, which have become essential in building web applications. However, choosing between these libraries or frameworks […]
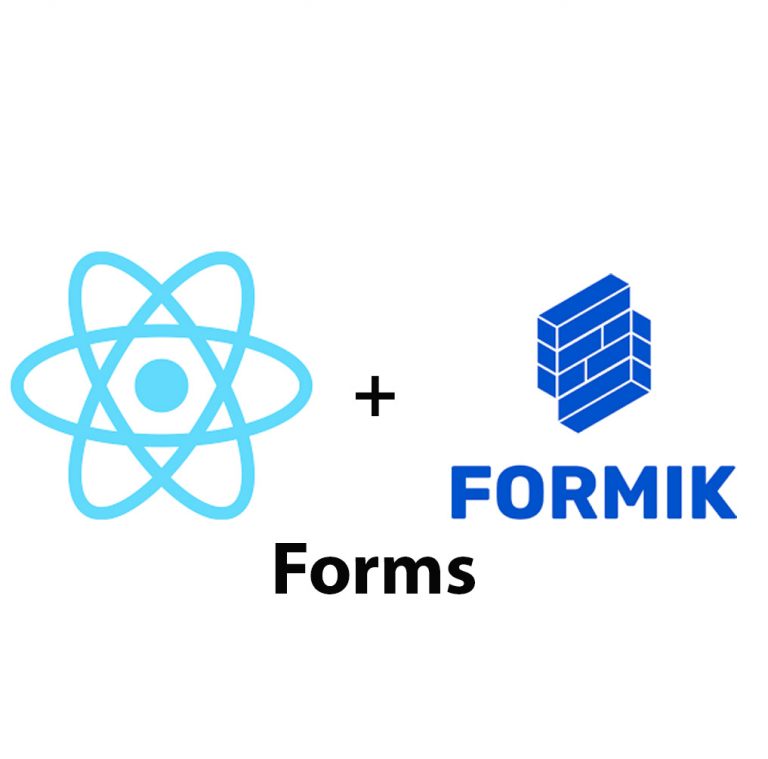
Create Forms In React JS Using Formik
Introduction React JS is a widely used system for building complex and dynamic web applications. It allows developers to work with reusable components in a more efficient way than traditional JavaScript frameworks. One crucial aspect of developing web applications is building forms, which are used to collect user input and send it to a server. […]
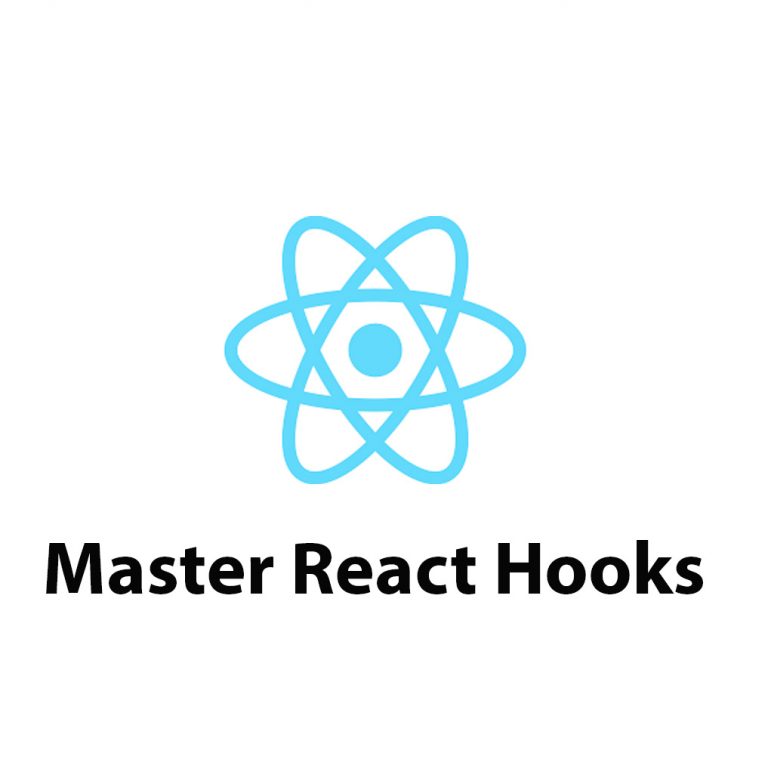
Mastering Hooks In React JS
Introduction React JS is a popular JavaScript library that has gained a lot of popularity since its introduction in 2013. It has been adopted by many web developers who use it to build robust and reliable web applications. With React JS, developers can create user interfaces by building reusable UI components, which can be combined […]
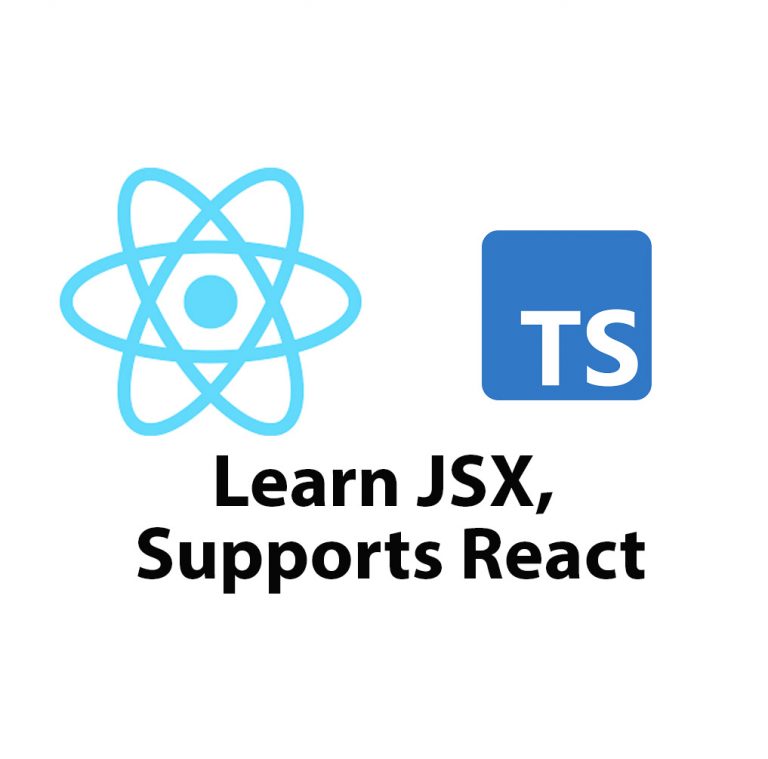
What Is JSX and how to use it.
Introduction As a web developer, I’m always on the lookout for new tools and technologies that can help me write better code faster. One tool that has caught my attention lately is Typescript JSX. In this article, I’m going to share my experience learning Typescript JSX and the benefits it has brought to my development […]
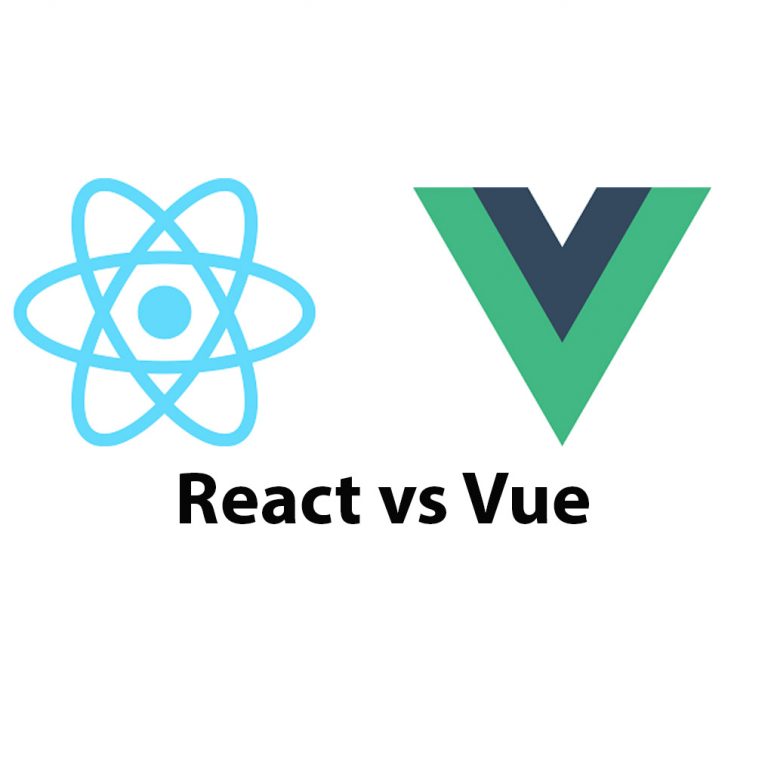
React JS vs Vue: A Comprehensive Guide
Introduction ReactJS and Vue are two of the most popular JavaScript libraries used to develop dynamic user interfaces. These libraries have gained widespread popularity due to their flexibility, efficiency, and ease of use. ReactJS was developed by Facebook, and Vue was created by Evan You. In this article, we are going to evaluate and compare […]