As a web developer, I understand the importance of data visualization in presenting complex information in an understandable and visually appealing way. That’s why I’ve been using ChartJS 2, a powerful JavaScript library, for creating charts in my React apps. In this article, I’ll show you how to set up and use ChartJS 2 in your React applications and explore some of its advanced features.
Setting Up ChartJS 2 in a React App
To get started, you first need to install ChartJS 2 and include it in your React app. You can install the library using npm:
npm install chart.js --save
Next, include the library in your React component:
import Chart from 'chart.js';
You also need to configure ChartJS 2 to work with React. One way to do this is by using a library like react-chartjs-2, which is a wrapper around ChartJS 2 that provides a React-friendly interface.
Creating Basic Charts
Once you have set up ChartJS 2, you can start creating charts. To create a basic line chart, for example, you can use the following code:
import { Line } from 'react-chartjs-2';
const data = {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [
{
label: 'My First dataset',
data: [65, 59, 80, 81, 56, 55, 40],
borderColor: 'rgba(255, 99, 132, 1)',
backgroundColor: 'rgba(255, 99, 132, 0.2)',
},
],
};
const MyLineChart = () => <Line data={data} />;
Here, we import the Line component from react-chartjs-2 and define the data we want to display in the chart. We then pass the data to the Line component as a prop. The line chart will automatically render with the provided data.
Creating a bar chart is similar:
import { Bar } from 'react-chartjs-2';
const data = {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [
{
label: 'My First dataset',
data: [65, 59, 80, 81, 56, 55, 40],
borderColor: 'rgba(255, 99, 132, 1)',
backgroundColor: 'rgba(255, 99, 132, 0.2)',
},
],
};
const MyBarChart = () => <Bar data={data} />;
And creating a pie chart:
import { Pie } from 'react-chartjs-2';
const data = {
labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'],
datasets: [
{
label: '# of Votes',
data: [12, 19, 3, 5, 2, 3],
backgroundColor: [
'rgba(255, 99, 132, 0.2)',
'rgba(54, 162, 235, 0.2)',
'rgba(255, 206, 86, 0.2)',
'rgba(75, 192, 192, 0.2)',
'rgba(153, 102, 255, 0.2)',
'rgba(255, 159, 64, 0.2)',
],
borderColor: [
'rgba(255, 99, 132, 1)',
'rgba(54, 162, 235, 1)',
'rgba(255, 206, 86, 1)',
'rgba(75, 192, 192, 1)',
'rgba(153, 102, 255, 1)',
'rgba(255, 159, 64, 1)',
],
borderWidth: 1,
},
],
};
const MyPieChart = () => <Pie data={data} />;
Adding Interactivity and Animation to Charts
ChartJS 2 also provides powerful tools for adding interactivity and animation to your charts. For instance, you can add hover effects to your charts to highlight data points as the user mouses over them. You can do this by including the hover option in your chart configuration:
const data = {
// ...
};
const options = {
// ...
hover: {
mode: 'nearest',
intersect: true,
},
};
const MyLineChart = () => <Line data={data} options={options} />;
You can also add animations to your charts by including the animation option in your chart configuration:
const options = {
animation: {
duration: 2000,
easing: 'easeInQuad',
},
};
const MyLineChart = () => <Line data={data} options={options} />;
Creating Combination Charts
ChartJS 2 also allows you to create combination charts, which are charts that display multiple data series on the same chart. For example, you might create a chart that displays the monthly temperature and rainfall for a particular location.
To create a combination chart, you simply define multiple datasets in your chart configuration. For example:
const data = {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [
{
label: 'Temperature',
data: [19, 20, 21, 22, 23, 24, 25],
type: 'line',
yAxisID: 'temperature',
},
{
label: 'Rainfall',
data: [13, 20, 6, 8, 3, 5, 10],
type: 'bar',
yAxisID: 'rainfall',
},
],
};
const options = {
scales: {
temperature: {
position: 'left',
},
rainfall: {
position: 'right',
},
},
};
const MyComboChart = () => <Line data={data} options={options} />;
Here, we define two datasets, one for temperature and one for rainfall, and tell ChartJS 2 to display them on the same chart. We also specify separate y-axes for each dataset using the yAxisID property.
Real World Applications
ChartJS 2 is a powerful tool that is used in a wide range of real world applications. For example, it is used by popular online tools like Asana, Trello, and GitHub to display detailed analytics and project insights.
One example of how ChartJS 2 can be used in a real world scenario is a sales dashboard for an e-commerce website. With ChartJS 2, developers can create a visually appealing and interactive dashboard that displays key performance metrics like revenue, conversion rate, and website traffic.
To create a revenue chart, you might use the following code:
const data = {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [
{
label: 'Sales',
data: [1000, 2000, 3000, 4000, 5000, 6000, 7000],
backgroundColor: 'rgba(255, 99, 132, 0.2)',
borderColor: 'rgba(255, 99, 132, 1)',
borderWidth: 1,
},
],
};
const options = {
scales: {
yAxes: [
{
ticks: {
beginAtZero: true,
},
},
],
},
};
const SalesChart = () => <Bar data={data} options={options} />;
In this example, we create a bar chart that displays monthly sales revenue over a period of seven months. Additionally, we define the chart properties to display labels on the X-axis for each month, begin at zero on the Y-axis, and set the color of the chart.
By adding similar charts for conversion rates, website traffic, and other metrics, the dashboard can provide a comprehensive overview of the website’s performance in real time.
Conclusion
ChartJS 2 is a powerful data visualization library that provides web developers with the tools they need to create visually appealing and informative charts. Its compatibility with React makes it an ideal choice for building modern web applications. With the ability to create a wide range of chart types, add interactivity and animation, and handle complex data sets, ChartJS 2 offers endless possibilities for creating engaging and informative charts.
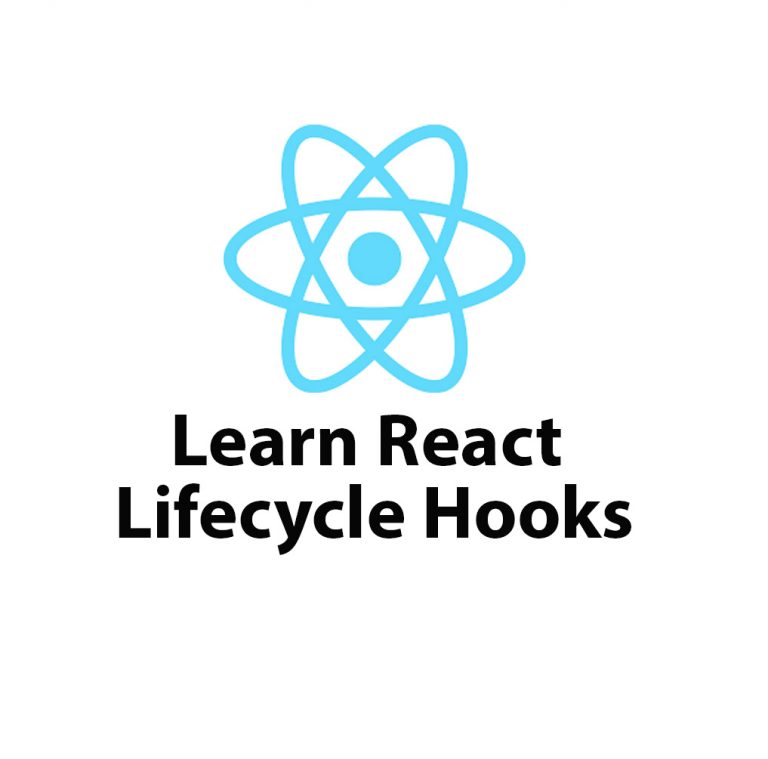
Lifecycle Hooks in React JS – Introduction
React JS is one of the most popular front-end frameworks used by developers worldwide. It offers great flexibility and reusability because of its component-based architecture. A component in React is an independent and reusable piece of UI, and it can be thought of as a function of state and props. React provides lifecycle hooks that […]
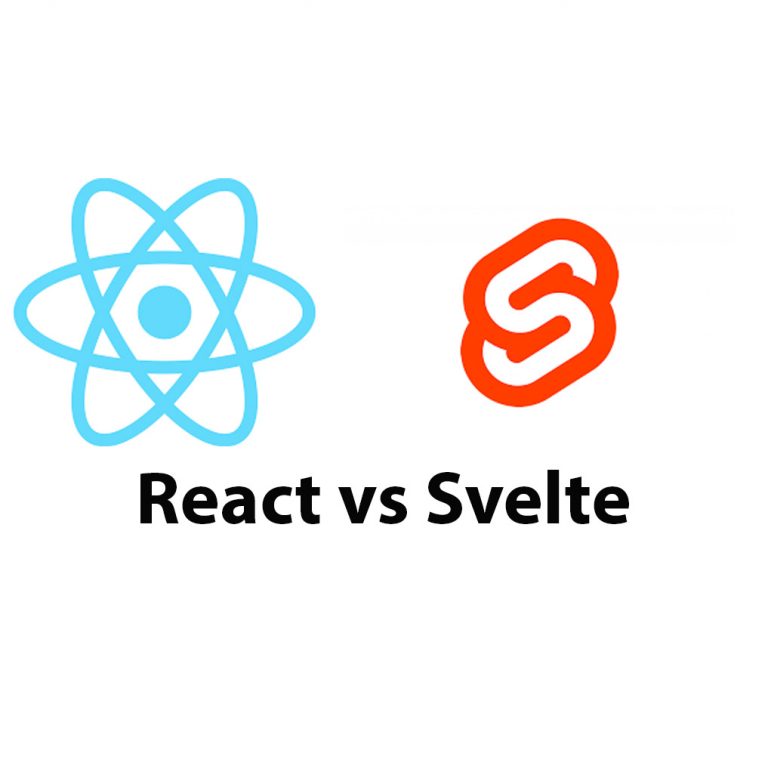
React JS vs Svelte
Introduction In the world of web development, there are many frameworks available for use. Two of the most popular frameworks are React JS and Svelte. React JS is an open-source JavaScript library developed by Facebook while Svelte is a relatively new framework developed by Rich Harris in 2016. In this article, we will compare React […]
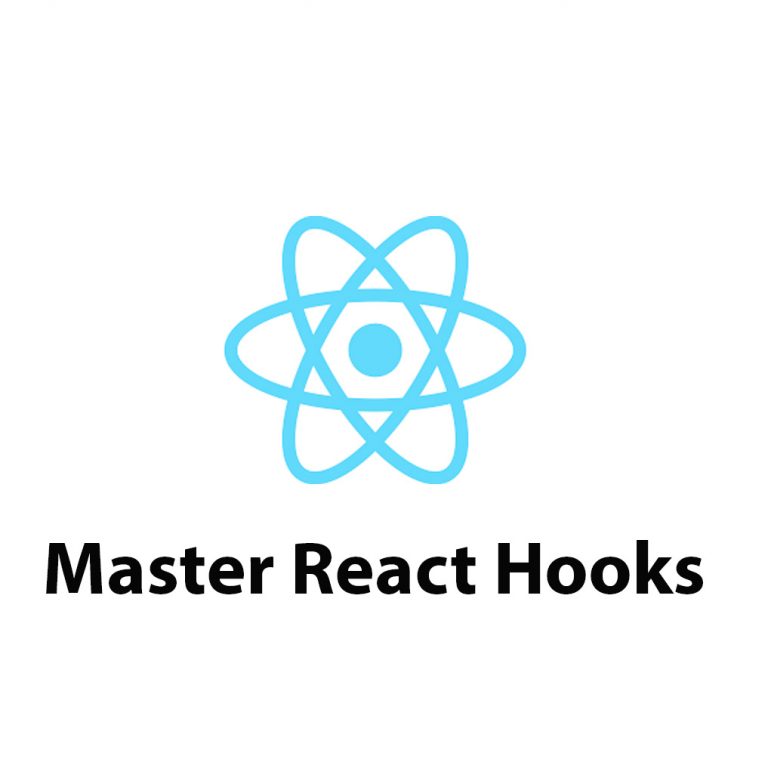
Mastering Hooks In React JS
Introduction React JS is a popular JavaScript library that has gained a lot of popularity since its introduction in 2013. It has been adopted by many web developers who use it to build robust and reliable web applications. With React JS, developers can create user interfaces by building reusable UI components, which can be combined […]
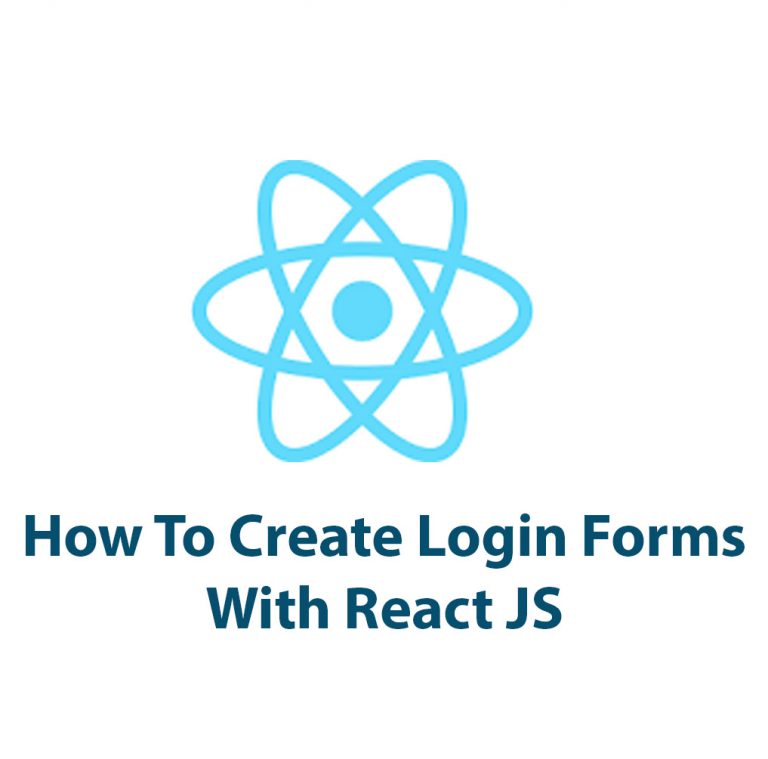
Create A Login Form In React
Creating a login form with React is an essential component in web development that can be accomplished in a few simple steps. In this article, we will walk through the process of creating a login form with React and explore its benefits. Before we begin, let’s take a moment to understand React. React is a […]
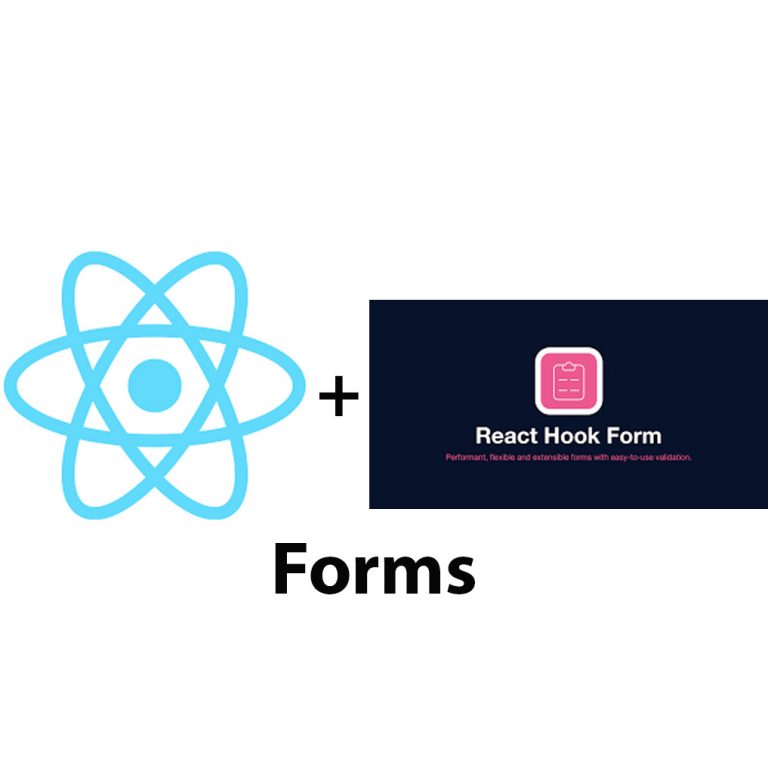
Create Forms In React Using React Hook Form
Introduction React Hook Form is a popular tool used in building forms in React.js. It is a performant library that simplifies the process of building forms by reducing boilerplate code and providing convenient features. This article is aimed at introducing the basic concepts of React Hook Form and how to use it to create forms […]
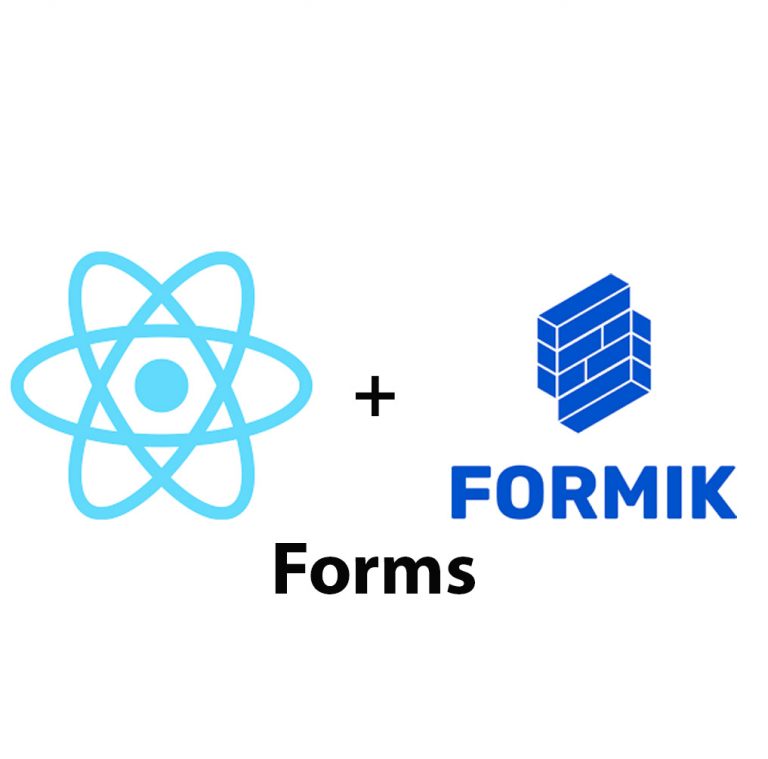
Create Forms In React JS Using Formik
Introduction React JS is a widely used system for building complex and dynamic web applications. It allows developers to work with reusable components in a more efficient way than traditional JavaScript frameworks. One crucial aspect of developing web applications is building forms, which are used to collect user input and send it to a server. […]