Introduction:
React is a powerful JavaScript library with a growing presence in web development today. With its powerful rendering capabilities and cross-functional library, it has become an important skill for developers to learn. In order to prepare for React interviews, it is important to familiarize yourself with React interview questions, which cover a range of topics, including React basics, component architecture, advanced concepts, React hooks, React Router and Real-World Situational questions.
Basic React Interview Questions:
What is React and how does it work?
React is a JavaScript library which is used to build user interfaces or UI components. It is based on the concept of a Virtual DOM (Document Object Model), which represents the structure of the UI components in memory to improve overall performance by minimizing the number of real DOM manipulations. React uses a simple syntax format called JSX, which allows developers to define HTML-like tags in their JavaScript code.
What is JSX? How does it differ from HTML?
JSX is a syntax format similar to HTML, but written in JavaScript code. JSX helps developers to write React views or UI components in a simple and intuitive way. JSX is different from HTML, in that it can be used to compose and maintain UI components within a JavaScript file with logical flow, therefore helping to maintain a cleaner codebase.
What is a component in React?
A component is a reusable piece of code with specific functionality. React components are reusable, modular, and include both presentation and behavior. Components are used to represent different parts of UI, such as buttons, forms, text boxes, etc. Components can contain other components and are responsible for rendering the UI based on user input or data changes.
What is state in React? How is it different from props?
State refers to the condition of the React component at any given time. It consists of the data stored within a component, which is manipulated or changed based on events or other user interactions. Props, on the other hand, refer to properties that are passed down from a parent component to a child component. Props are read-only and help to maintain the component’s structure and behavior.
React Component Architecture:
Explain React component lifecycle methods?
React provides lifecycle methods to help manage component creation, output, and destruction. React’s lifecycle has three main stages: Mounting (creating), Updating (rendering), and Unmounting (removing). These methods provide hooks to interact and manage components at different stages of the lifecycle. For example, componentDidMount is called when a component is mounted in the DOM, and componentDidUpdate is called when the component is updated with new data or input.
What is the difference between the Virtual DOM and the Real DOM?
The Virtual DOM is a way to minimize the number of actual DOM manipulations by using a copy or simulation of the DOM. The Virtual DOM is lighter and faster than the Real DOM, which provides better performance and faster rendering. The Reconciler is responsible for comparing the Virtual DOM with the Real DOM and updating the DOM components based on any changes.
What is the purpose of keys in React? How do you define them?
Keys are used by React to identify unique component instances or elements in an array. Keys help React identify which elements have changed or been removed, which ensures that components are re-rendered with accurate information. Keys are defined by adding a unique ID attribute or ‘key’ to each element in a component’s array.
Explain the difference between a container component and a presentational component.
A container component handles data input, holds state, and might interact directly with an API, for example, a User component that fetches data about users. A presentational component, on the other hand, handles the output aspect of the component, providing a visual representation of the data, for example, a UserListing component that shows a list of users using a table or other visual representation.
Advanced React Interview Questions:
Can you explain Redux and how would you use it with React?
Redux is a predictable state container for JavaScript apps that can help manage the state of an application more easily. When used with React, Redux provides a central store to hold application state and rendering logic in one location. The React-Redux library provides a way to connect the React view layer to the Redux store through Provider and connect components.
What are higher order components (HOC)?
Higher order components or HOCs are functions that receive a component and return a new component. HOCs are used to manipulate the component’s behavior and provide additional functionality or data. HOCs can also be used to share common code between components, making them reusable and easier to maintain.
What is JSX and why is it used?
JSX is a syntax extension for JavaScript that helps simplify the way React components are built. JSX allows users to write code that resembles HTML and combine functionality and presentation elements in one concise file. JSX helps keep the codebase modular and gives developers an intuitive and clear method for building out user interfaces.
How would you optimize a React application’s rendering performance?
There are several ways to optimize the rendering performance of a React application, including breaking down user interfaces into smaller and more manageable components, correctly structuring data flows between components, using Pure Components, and utilizing shouldComponentUpdate and PureComponent methods to improve performance and minimize unwanted re-renders.
React Hooks:
What are React Hooks and how do they differ from class components?
React Hooks are functions that allow developers to use state and other React features in function components. They help simplify the code and provide a cleaner and more efficient way to provide complex functionality and maintain dynamic data. React Hooks can be used in place of traditional component lifecycle methods in class components.
Explain useState and useEffect Hooks.
useState is a Hook for adding React state to function components. With useState, developers can set up state variables, manipulate and update them as needed. useEffect, on the other hand, is a Hook that replaces the Component Lifecycle Methods like componentDidMount and componentDidUpdate. useEffect allows developers to perform side-effects or actions based on changes in state, props, or other interactions.
What is useContext and how would you use it in a React application?
useContext is a Hook in React that can be used to share data between components at any level within the hierarchy regardless of how many levels down a function component may be. Developers define the provider component and use the useContext Hook to share data and update components that need access to the data.
How do you create a custom React Hook?
Custom Hooks are created in much the same way as regular React components, and can be used to abstract logic that is presented across multiple applications. Developers typically create custom Hooks and store them in a centralized location to make them reusable across an entire project.
React Router:
Explain React Router?
React Router is a library that provides navigation and routing components for single-page applications built with React. React Router allows users to map URL routes to components in a declarative and intuitive manner. It includes different types of routers such as BrowserRouter, HashRouter, and MemoryRouter.
What is the difference between BrowserRouter, HashRouter, and MemoryRouter?
BrowserRouter uses HTML browser history API to navigate between components, HashRouter uses the URL hash to navigate, and MemoryRouter saves state in memory rather than the browser history. BrowserRouter is the most commonly used router for traditional browser-based web applications, HashRouter is useful for environments like GitHub pages which only support single-page applications with an anchor tag fallback, and MemoryRouter is useful for testing and may provide added security.
How do you pass parameters through routes in React Router?
In React Router, users can pass parameters as URL parameters or through the router’s location property. URL parameters are defined within the router as a colon-separated string, then accessed within the component using the props.match.params.object. The history.push() function can be used to pass parameters through the router’s location property.
How would you handle 404 errors in React Router?
React Router provides a SideRoute component that can be used to catch 404 errors and render a custom component or error page. The SideRoute is typically wrapped in a Switch component, which provides logical switch-based routing, such as a final route that will render a 404 component if no other routes match.
Real-World Situational Questions:
Have you ever had to solve a difficult problem in a React app? Explain how you went about solving it.
In this interview question, it is essential to provide an example from a previous project and explain how the problem was approached. It is important to demonstrate the ability to solve problems and the thought processes involved in finding a solution.
Can you explain how you have implemented accessibility in a React application?
Accessibility in React applications involves the use of ARIA (Accessible Rich Internet Applications) to optimize the user experience for people with disabilities. This answer should describe how ARIA is used in React components and provide examples of voice activation, keyboard navigation, and screen readers.
Have you ever integrated a React application with third-party services? Which ones, and how did you go about it?
This interview question tests the candidate’s experience with integrations and APIs. The answer should describe the specific third-party services and the APIs involved, as well as the steps taken to integrate it with the React application. It’s important to highlight any issues encountered and how they were resolved.
How do you ensure that a component is unit tested?
The answer to this interview question should explain the importance of unit testing and how testing frameworks such as Jest or Enzyme can be used to test React components. It should also provide examples of testing specific functionality like props and state while using testing tools like snapshots to ensure visual consistency.
In conclusion, preparing for React interviews requires a good understanding of the technology’s essential concepts, including JSX, components, and state. It also requires a good understanding of advanced React concepts, such as React Hooks, React Router, and Redux. Practice through hands-on projects, code bootcamps, and courses can sharpen your skills. Employers want to know that a candidate has hands-on experience and the ability to solve real-world problems. With practice and the right mindset, anyone can excel in a React interview.
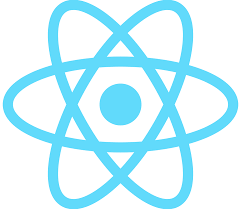
React JS Tutorial
React JS is an open-source JavaScript library that has revolutionized the way web developers create and build single-page applications. It is used by some of the biggest companies in the world, including Facebook, Instagram, Netflix, and Airbnb. If you’re passionate about web development and are looking to add new skills to your resume, then learning […]
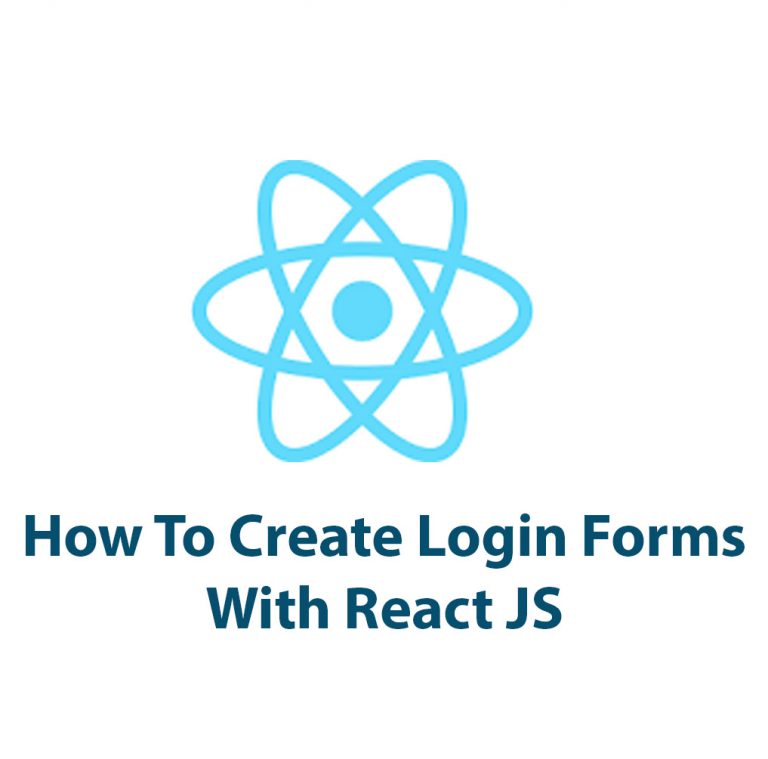
Create A Login Form In React
Creating a login form with React is an essential component in web development that can be accomplished in a few simple steps. In this article, we will walk through the process of creating a login form with React and explore its benefits. Before we begin, let’s take a moment to understand React. React is a […]
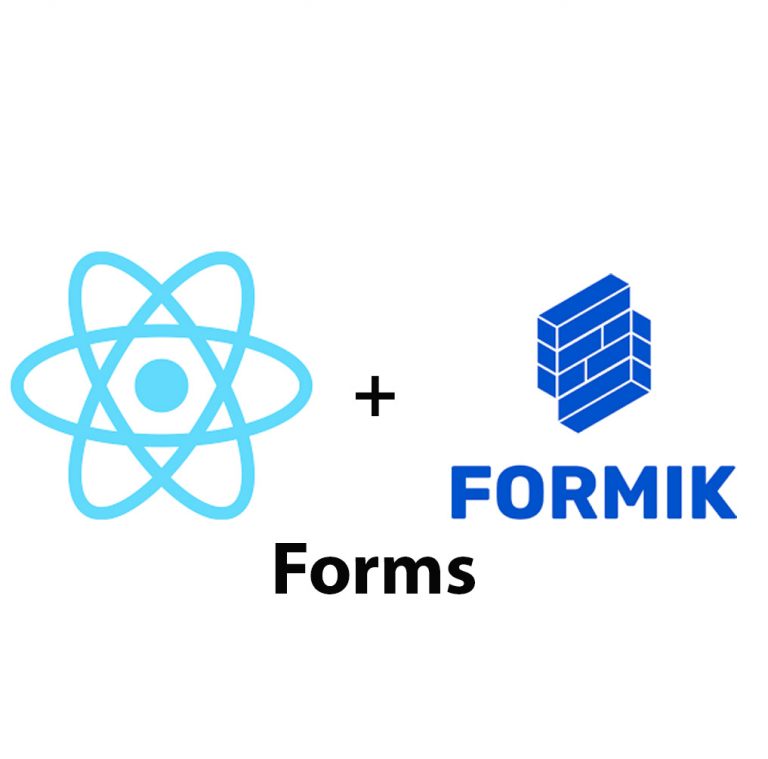
Create Forms In React JS Using Formik
Introduction React JS is a widely used system for building complex and dynamic web applications. It allows developers to work with reusable components in a more efficient way than traditional JavaScript frameworks. One crucial aspect of developing web applications is building forms, which are used to collect user input and send it to a server. […]
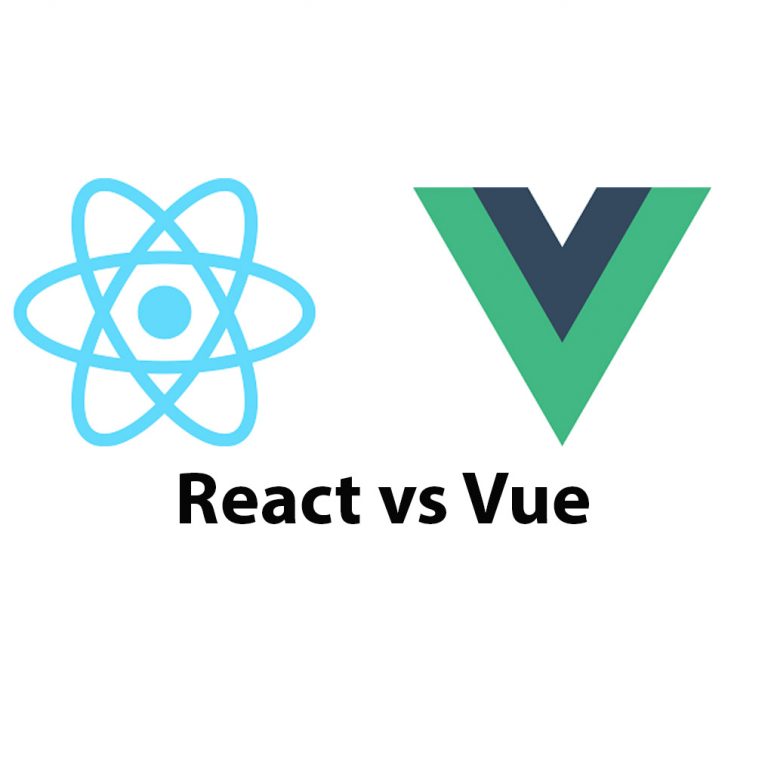
React JS vs Vue: A Comprehensive Guide
Introduction ReactJS and Vue are two of the most popular JavaScript libraries used to develop dynamic user interfaces. These libraries have gained widespread popularity due to their flexibility, efficiency, and ease of use. ReactJS was developed by Facebook, and Vue was created by Evan You. In this article, we are going to evaluate and compare […]
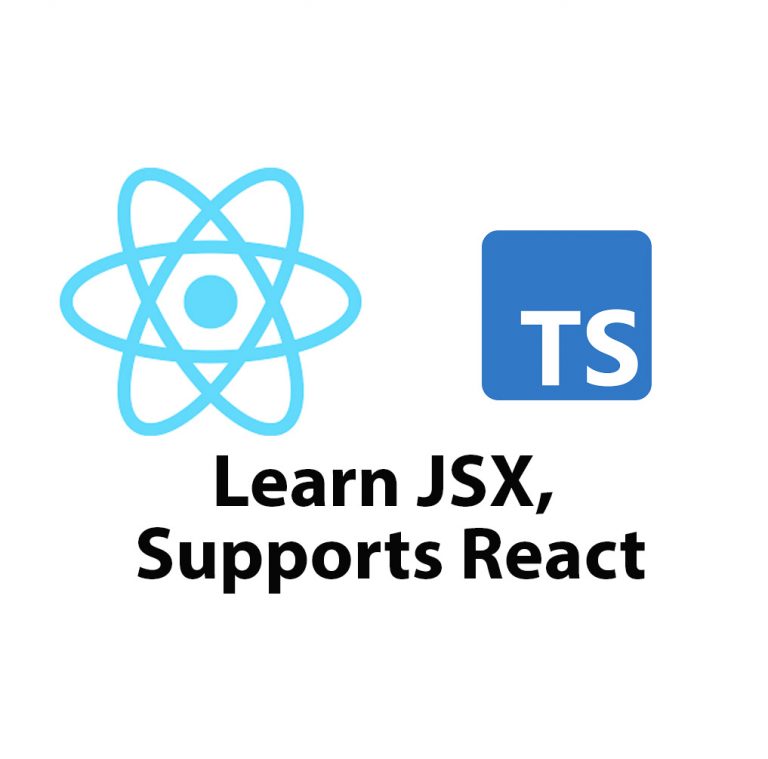
What Is JSX and how to use it.
Introduction As a web developer, I’m always on the lookout for new tools and technologies that can help me write better code faster. One tool that has caught my attention lately is Typescript JSX. In this article, I’m going to share my experience learning Typescript JSX and the benefits it has brought to my development […]
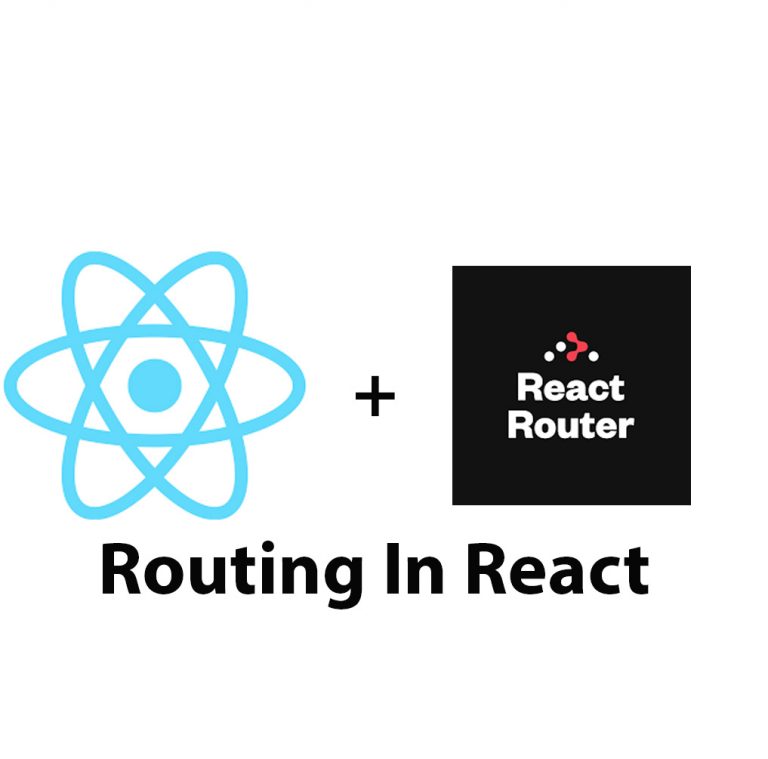
Routing In React JS Using React Router
Introduction React JS has become one of the most popular frontend frameworks for web development. It allows developers to create complex and dynamic user interfaces with ease. Routing is an essential part of web development, and React Router is one such library that is used to manage routing in React applications. In this article, we’ll […]