Have you ever found yourself in a situation where your code was causing errors and you couldn’t quite pinpoint the issue? If so, you may want to consider using assertion testing with Node JS. Assertion testing is a great way to ensure that your code is functioning as intended and catching errors before they become bigger problems. In this article, we’ll cover how to set up and use assertion testing with Node JS, including some advanced techniques and common mistakes to avoid.
First off, let’s start with a brief overview of assertion testing. Simply put, assertion testing is a way of writing tests to ensure that your application’s code works as expected. These tests are written in a way that asserts certain outcomes or behaviors, and if those assertions fail, it means there’s an issue in your code.
So, how do we set up assertion testing with Node JS? The first step is to install Node JS if you haven’t already. You can do this by visiting the official Node JS website and following their installation instructions. Once that’s done, you’ll need to add an assertion library to your project. There are many assertion libraries available for Node JS, including Chai and Jest. For this article, we’ll be using the built-in assertion library that comes with Node JS.
To set up the built-in assertion library, you’ll need to add the following line of code to your test file:
const assert = require('assert');
This line of code imports the assert library into your test file, allowing you to use its functions to write your tests.
Now that we’ve set up our testing environment, we can start writing our first assertion test. Let’s say we have a function that adds two numbers together:
function addNumbers(a, b) {
return a + b;
}
We can write an assertion test for this function to ensure that it’s working properly:
assert.equal(addNumbers(2, 3), 5);
This assertion test checks whether addNumbers(2, 3) returns 5. If it does, the test passes. If it doesn’t, the test fails, indicating that there’s an issue with our addNumbers function.
But what about more complex tests? Let’s say our application has a user authentication system, and we want to ensure that only registered users can access certain pages. We can write an assertion test for this scenario like so:
const user = {
name: 'John Doe',
email: '[email protected]',
password: 'password123'
};
function authenticateUser(email, password) {
if (email === user.email && password === user.password) {
return true;
} else {
return false;
}
}
describe('User Authentication', () => {
it('should authenticate a registered user', () => {
assert.equal(authenticateUser('[email protected]', 'password123'), true);
});
it('should not authenticate an unregistered user', () => {
assert.equal(authenticateUser('[email protected]', 'password123'), false);
});
it('should not authenticate a user with an incorrect password', () => {
assert.equal(authenticateUser('[email protected]', 'incorrectpassword'), false);
});
});
This example uses the Mocha testing framework to write descriptive tests using the it() and describe() functions. Our authenticateUser function takes in an email and password, and checks whether they match the user object’s email and password properties. We’ve written three different tests to ensure that our authentication function is working properly.
Now that we’ve written our tests, we need to run them and interpret the results. To run our tests, we can use the command line and navigate to our project’s directory. Once there, we can run the following command:
npm test
This command will run our test file and output the results to the console. If any of our tests fail, we’ll see an error message indicating which test(s) failed and why.
So, we’ve covered the basics of assertion testing with Node JS. But what about more advanced techniques? One common technique is mocking and stubbing, which involves creating fake data or functions to simulate certain scenarios in your tests. Another technique is test coverage, which involves measuring the proportion of code that’s been tested. These techniques can help you write more thorough tests and catch potential issues before they become real problems.
But be careful – there are also common mistakes to avoid when writing assertion tests. One mistake is writing too general or insufficient tests, which can lead to missed errors. Another mistake is writing tests that are too dependent on the implementation details of your code, which can lead to brittle tests that break when you make changes to your code.
In conclusion, assertion testing with Node JS is a powerful tool for ensuring that your application’s code is functioning as intended. With a little setup and some basic knowledge, you can write effective and comprehensive tests that catch issues before they become bigger problems. So, next time you find yourself struggling with an error in your code, give assertion testing a try. You might just be surprised at how effective it can be!
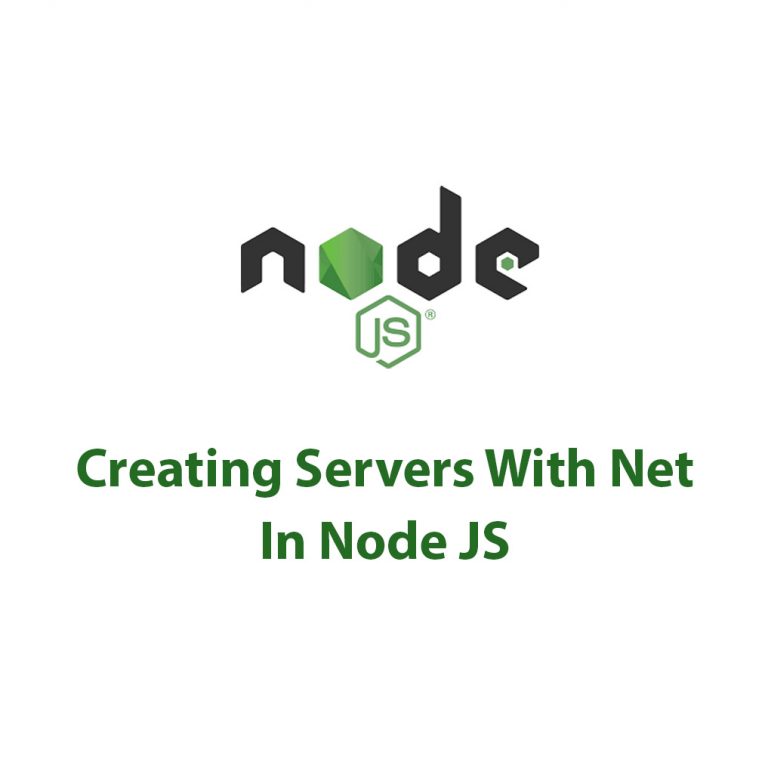
Working With The Net Package In Node JS
As a Node.js developer, I have always been fascinated by the vast array of modules and packages that can be used to simplify the development process. One such package that has long intrigued me is the Net package. In this article, I’ll delve deep into what the Net package is, how to set it up, […]
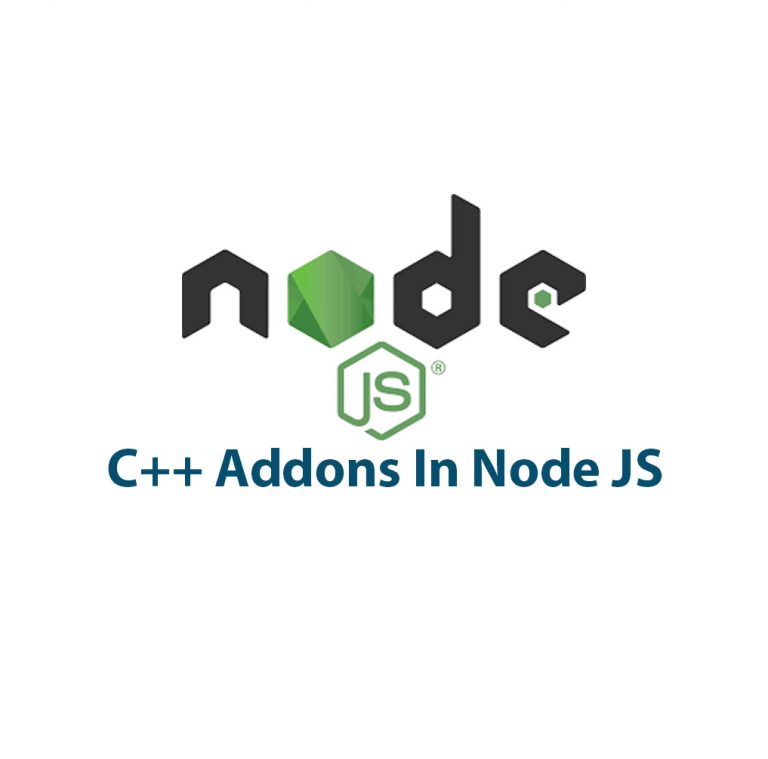
C++ Addons In Node JS
Introduction: As a software developer, I am always looking for ways to improve the performance of my applications. One way to achieve this is by using C++ Addons in Node JS. In this article, we will explore what C++ Addons are, why they are useful in Node JS, and how to create and use them. […]
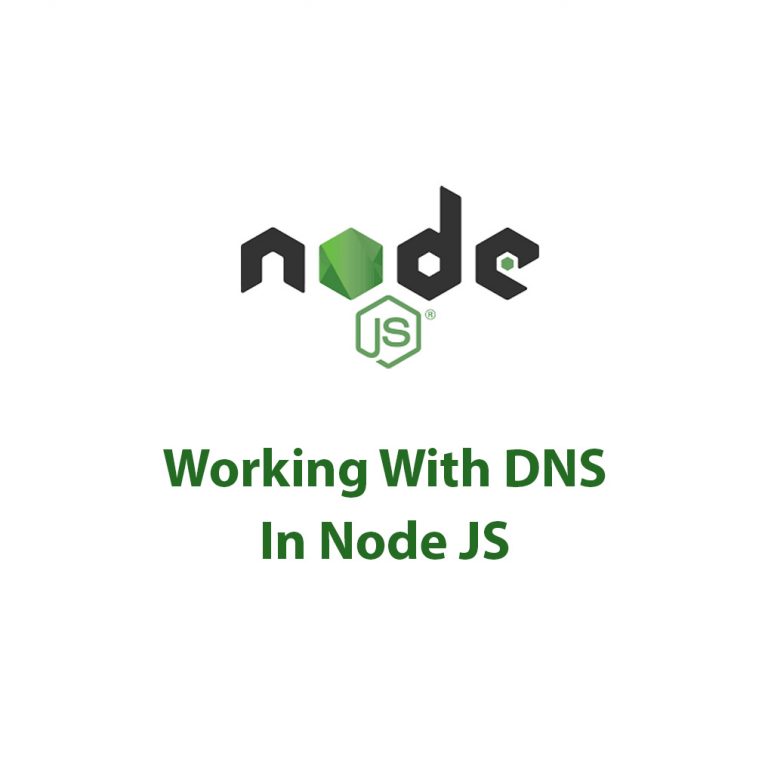
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]
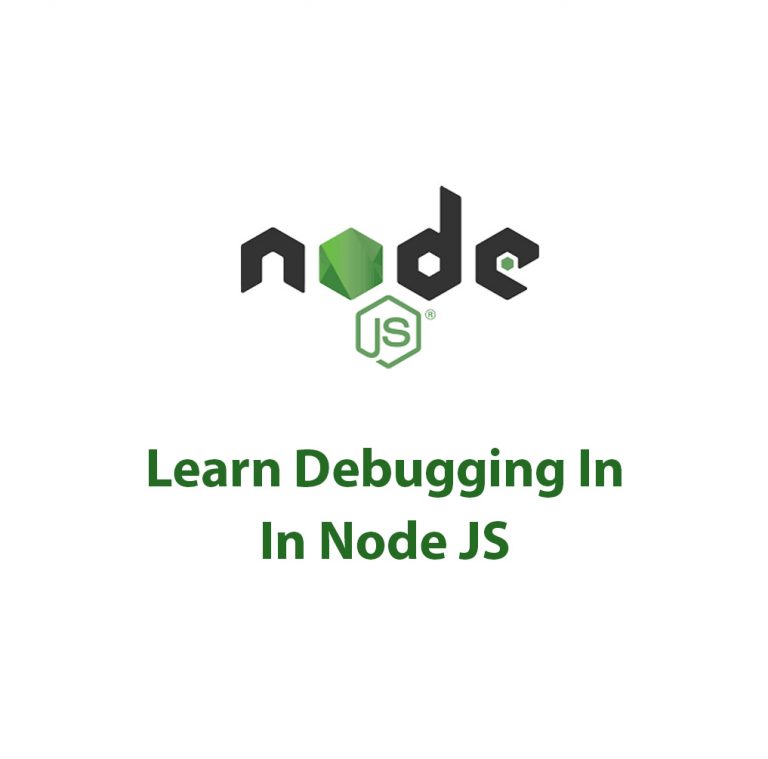
Debugger In Node JS
Debugger In Node JS: Getting a Deeper Understanding One thing we might all have in common as developers in the always changing tech industry is the ongoing need to come up with new and better approaches to debug our code. Since troubleshooting is a crucial step in the development process, we must be well-equipped with […]
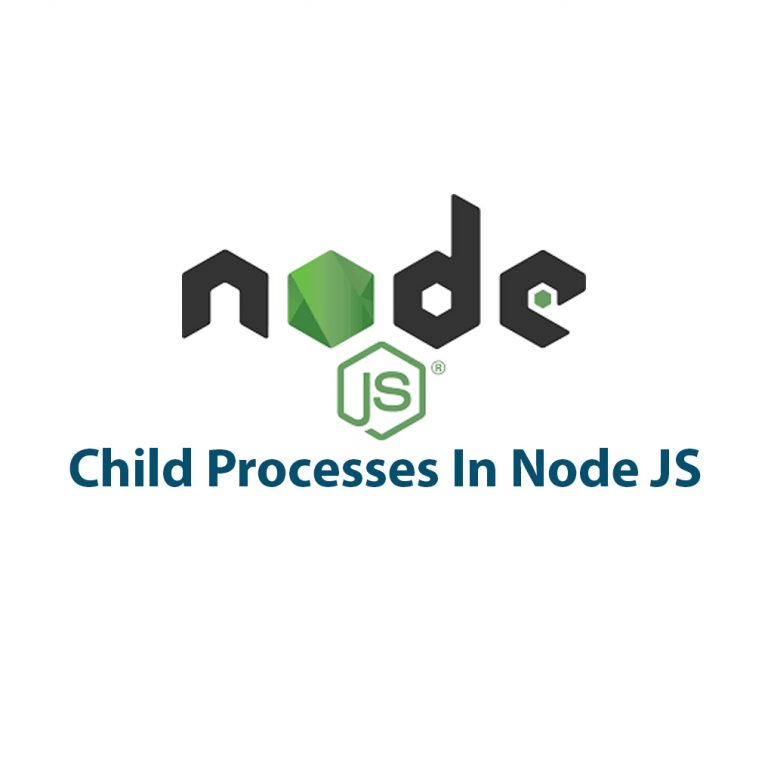
Child Processes In Node JS
Hey there! Today I’m going to talk about Child Processes in Node.js. As you may know, Node.js is a popular open-source, cross-platform, JavaScript runtime environment. It offers a lot of features out of the box, one of them being the ability to work with Child Processes. Child Processes allow you to run multiple processes simultaneously, […]
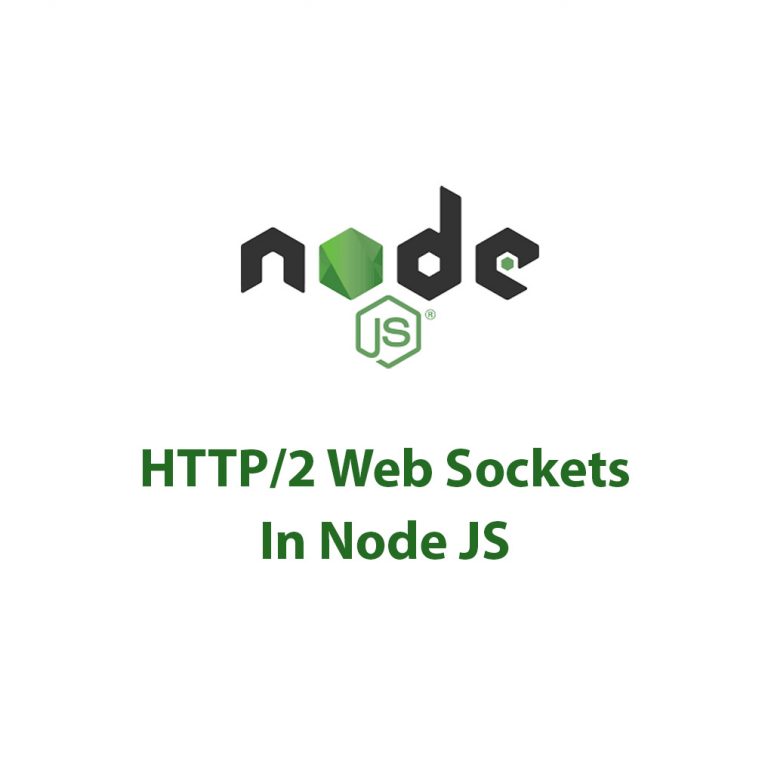
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]