As someone who is just getting started with Node JS, hearing about buffers might be a bit confusing at first. What are they exactly, and why should you care about them? I know I was pretty perplexed by this concept when I first started working with Node JS. However, once I understood what buffers were and how to use them, I realized how useful they are. In this article, I’m going to share with you everything you need to know about working with buffers in Node JS.
What are Buffers?
A buffer is a temporary storage area that holds binary data. It is essentially an array of integers, which can range from 0 to 255. Buffers are useful for storing binary data, such as images or audio files, and are used to manipulate and manipulate data at a low level.
In Node JS, buffers are an essential part of working with streams, and are used frequently by the built-in modules. They are also commonly used in network programming scenarios.
Getting Started with Buffers
Now that we know what a buffer is, let’s create one. The following code creates a buffer with a size of 5 bytes:
const buffer = Buffer.alloc(5);
The Buffer.alloc()
function allocates a new buffer, with a specified size. We can then write some data to the buffer, using the write()
function:
buffer.write("hello");
Looking at the buffer object, we see the raw binary data that has been written to it:
<Buffer 68 65 6c 6c 6f>
The data is represented in hexadecimal format, as buffers store binary data. We can convert this data to a string using the toString()
function:
console.log(buffer.toString());
This outputs:
hello
Advanced Features of Buffers
There are many advanced features of buffers, which we can use to manipulate data in more interesting ways. One feature is combining buffers. We can combine two buffers together using the concat()
function:
const buffer1 = Buffer.from('hello');
const buffer2 = Buffer.from('world');
const result = Buffer.concat([buffer1, buffer2]);
console.log(result.toString());
The above code combines two buffers into a new buffer, and outputs:
helloworld
We can even compare buffers to determine if they are equal using the compare()
function:
const buffer1 = Buffer.from('hello');
const buffer2 = Buffer.from('hello');
const result = buffer1.compare(buffer2);
console.log(result);
The output of the above code is 0, because the contents of both buffers are equal.
Buffers and Streams
Buffers are commonly used in Node JS streams. A stream is a continuous flow of data that can be read or written. In a standard file read or write operation, Node JS loads the entire file into memory, which can be problematic for large files. However, with streams, Node JS reads and writes data in smaller chunks, making it a more efficient way of handling large files.
We can use buffers with streams by passing them to the read()
and write()
functions.
const fs = require('fs');
const fileReadStream = fs.createReadStream('./largefile.txt');
const fileWriteStream = fs.createWriteStream('./smallfile.txt');
fileReadStream.on('data', function(chunk) {
fileWriteStream.write(chunk);
});
The above code reads data from a large file and writes it to a new file in smaller chunks. Because buffers are used in streams by default, we don’t need to create them explicitly.
Best Practices and Use Cases
Now that we know what buffers are and how to use them, let’s discuss some best practices and common use cases.
One common scenario where buffers are used is in network programming. When we send data over a network, it is usually in binary format. Buffers come in handy when we need to manipulate binary data before sending it.
Another use case for buffers is when dealing with large files. Reading and writing large files in memory can quickly consume a lot of resources. However, with streams and buffers, we can process files in smaller chunks, which is a more efficient way of handling them.
When using buffers, it is essential to ensure that you are using them efficiently. If you are working with large amounts of data, creating too many buffers can quickly consume a lot of memory. Always consider how much data you are working with before starting to write your program.
Conclusion
In conclusion, buffers are a fundamental part of working with Node JS. Although they can be a bit confusing at first, they are essential for low-level binary data manipulation. Now that you know what buffers are, and how to use them, I encourage you to experiment with them in your own programs. Remember to always be mindful of creating too many buffers, and ensure that you are using them efficiently.
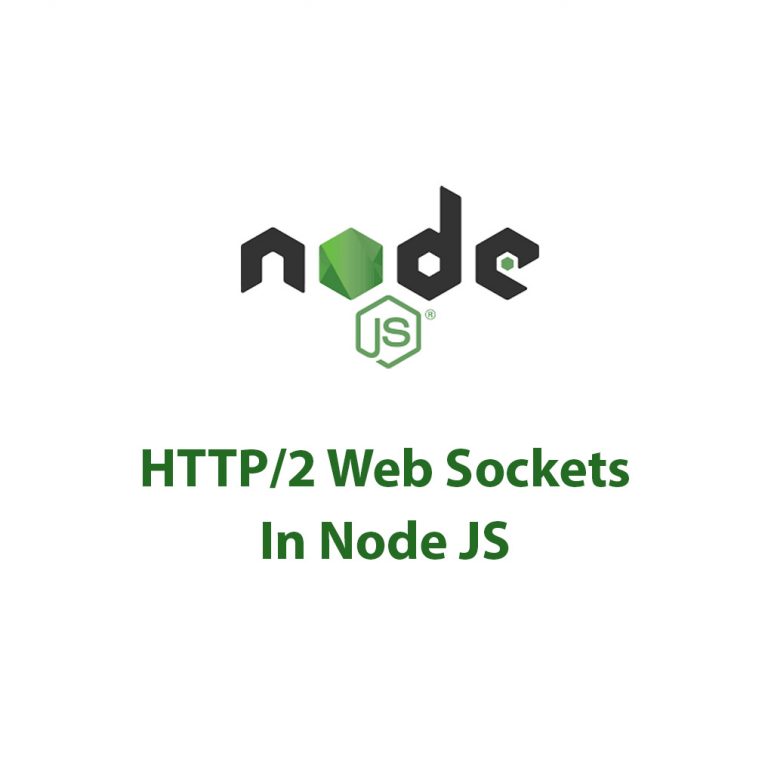
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
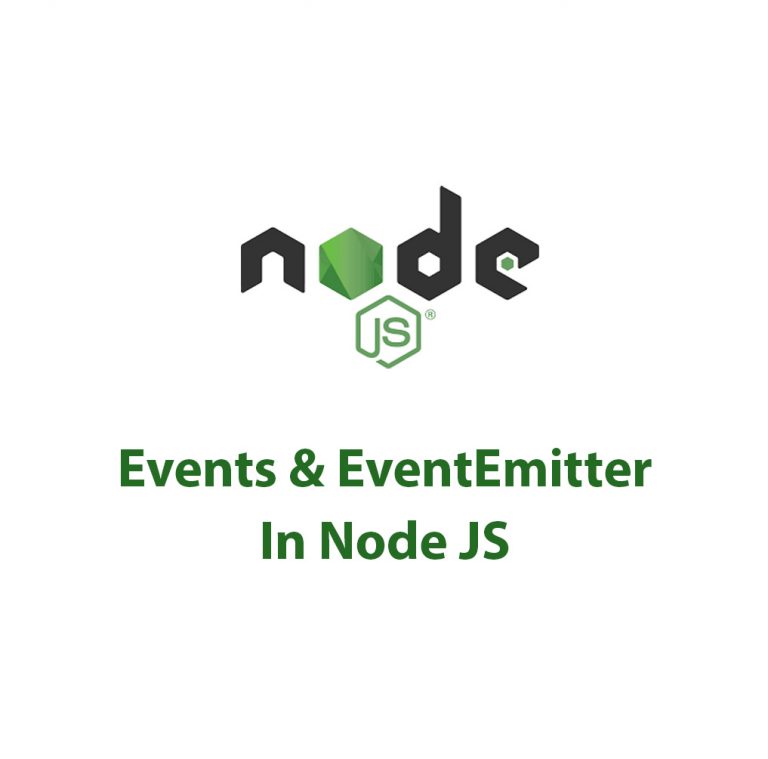
Events In Node JS
Introduction As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought. […]
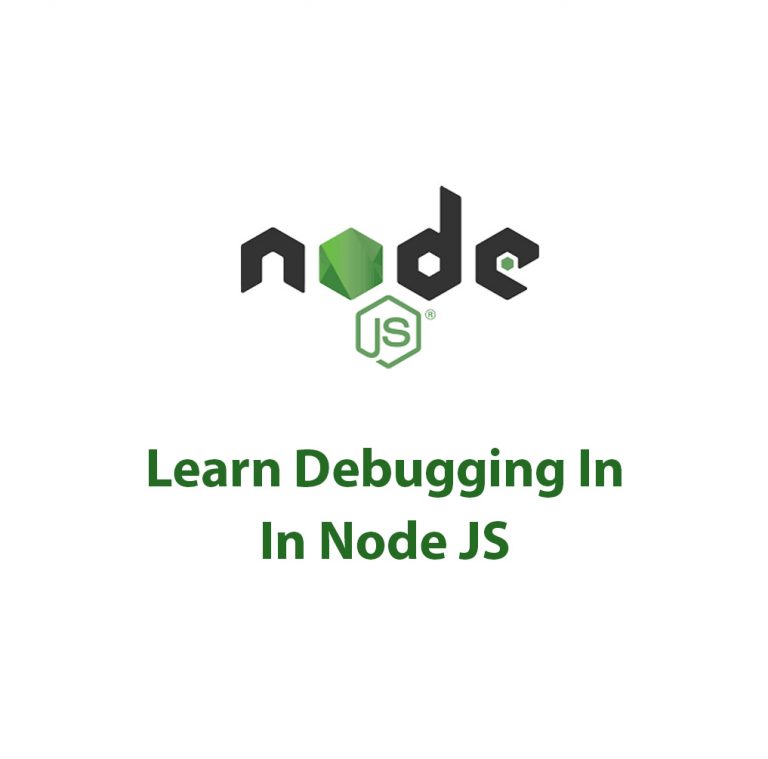
Debugger In Node JS
Debugger In Node JS: Getting a Deeper Understanding One thing we might all have in common as developers in the always changing tech industry is the ongoing need to come up with new and better approaches to debug our code. Since troubleshooting is a crucial step in the development process, we must be well-equipped with […]
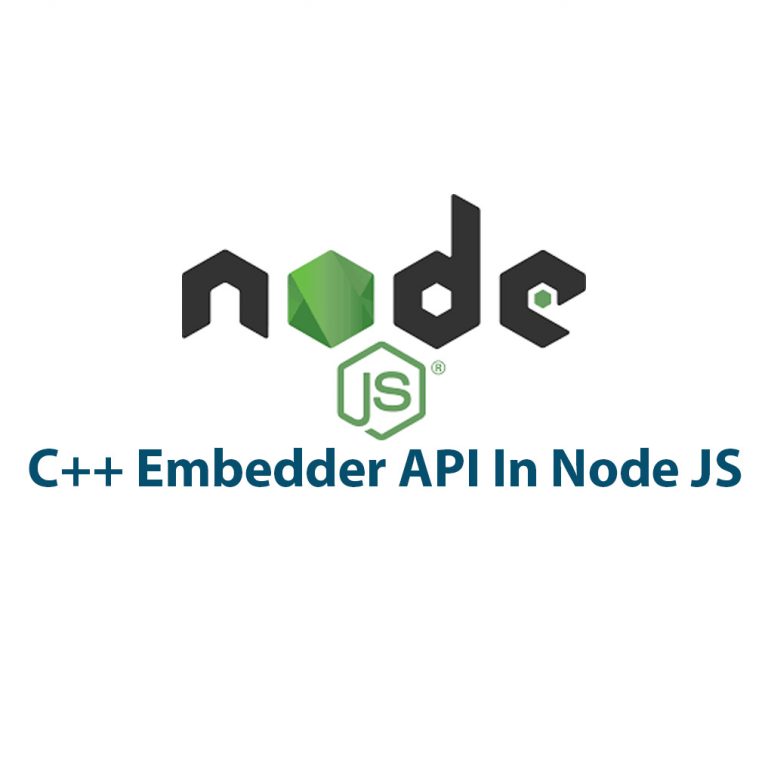
C++ Embedder API With Node JS
Introduction: As a programmer, I have always been fascinated with the power of NodeJS. It is a popular JavaScript runtime that can be used for server-side scripting. The beauty of NodeJS is that it allows for easy handling of I/O operations. However, sometimes the complexities of a project may go beyond just JavaScript coding, and […]
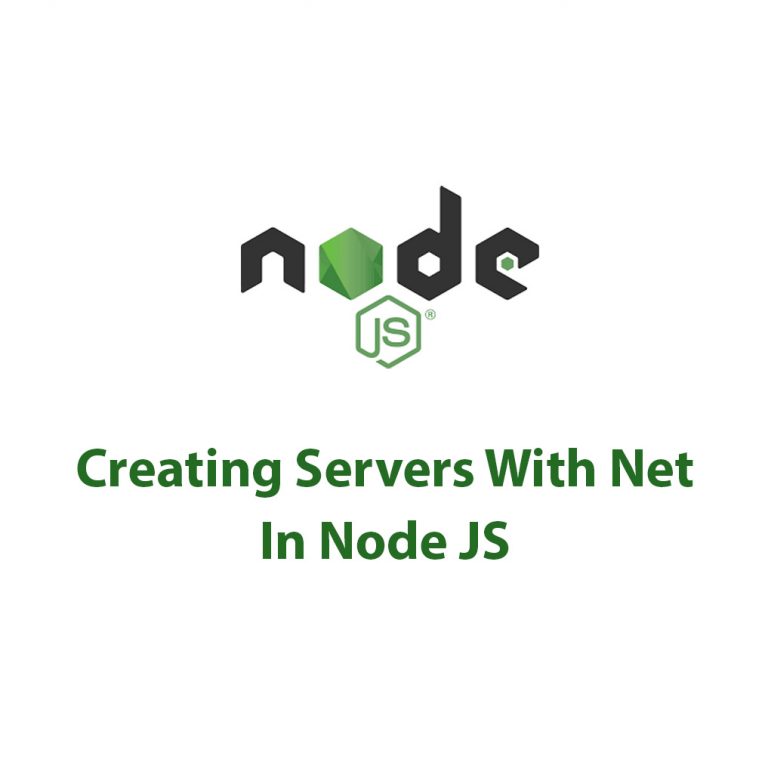
Working With The Net Package In Node JS
As a Node.js developer, I have always been fascinated by the vast array of modules and packages that can be used to simplify the development process. One such package that has long intrigued me is the Net package. In this article, I’ll delve deep into what the Net package is, how to set it up, […]
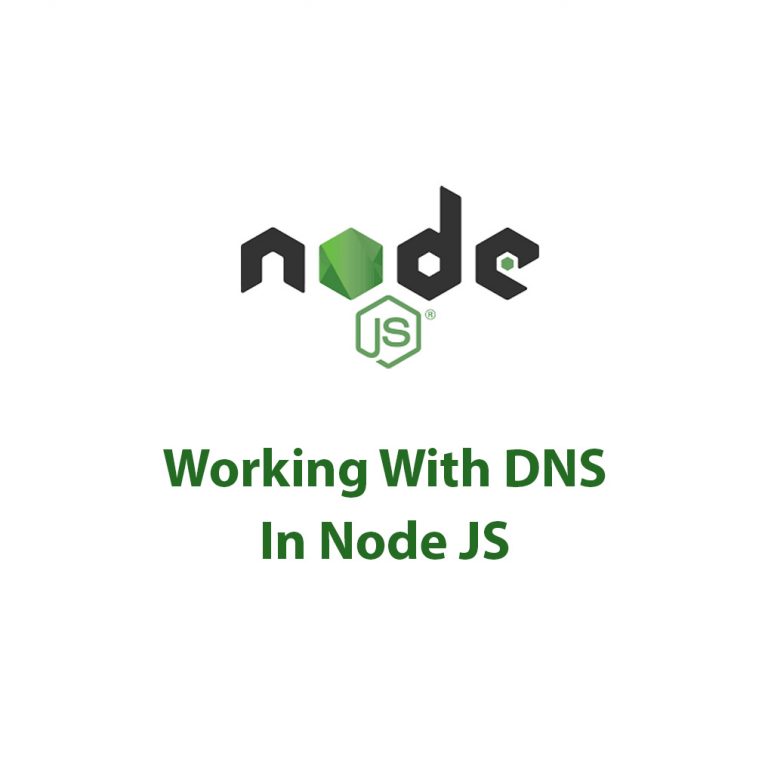
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]