Introduction:
As a programmer, I have always been fascinated with the power of NodeJS. It is a popular JavaScript runtime that can be used for server-side scripting. The beauty of NodeJS is that it allows for easy handling of I/O operations. However, sometimes the complexities of a project may go beyond just JavaScript coding, and therein lies the beauty of C++ API in NodeJS.
In this article, I’ll cover the integration of the C++ Embedder API in NodeJS, highlighting the reasons why it is an important tool for JavaScript developers. I’ll also provide guidance on how to set up and configure the environment for the use of C++ API in NodeJS. In addition, I’ll explore creating simple and complex C++ add-ons and offer tips on debugging the C++ code in NodeJS. Lastly, I’ll provide an evaluation of the advantages and disadvantages of using C++ API in NodeJS.
Setting up C++ Embedder API in NodeJS
Before we dive into the world of creating C++ add-ons, it is imperative to set up the environment. The installation process is simple, and all that is required is the installation of NodeJS and the C++ Embedder API.
The package.json file should include “node-addon-api”: “^3.0.0” under the dependencies.
{"name": "example-addon",
"version": "1.0.0",
"description": "Example module",
"main": "index.js",
"dependencies": {
"node-addon-api": "^3.0.0"
}
}
The .npmrc file should include a line to include clang on your devDependencies. Be sure that C++ compiler is installed.
CXX=clang++
After installing the C++ Embedder API, it is important to configure your environment. This will involve writing functions, such as macros, which will allow for ease of coding for C++ add-ons.
Creating C++ Addons in Node JS
C++ Addons are essentially C++ extensions written specifically for NodeJS. These add-ons allow for a seamless interface between the NodeJS JavaScript code and the C++ language.
To create a simple C++ add-on, one can use the N-API (Node-API). Node-API is a stable module introduced in NodeJS 8. The following code example shows the basic logic behind a simple add-on:
include
Napi::String ExampleMethod(const Napi::CallbackInfo& info) {
Napi::Env env = info.Env();
return Napi::String::New(env, "This is a simple example string");
}
Napi::Object Init(Napi::Env env, Napi::Object exports) {
exports.Set(Napi::String::New(env, "exampleMethod"),
Napi::Function::New(env, ExampleMethod));
return exports;
}
To add the C++ add-on to NodeJS, the following code should be executed:
const addon = require(‘bindings’)(‘exampleAddon.node’);
Building Complex C++ Addons
The syntax of C++ is quite different from JavaScript, but fear not, as it translates well into NodeJS. A good example of a complex C++ addon is one that deals with file I/O operations.
This example code shows an addon that reads a file and outputs the content:
Napi::String ReadFile(const Napi::CallbackInfo& info) {
Napi::Env env = info.Env();
if (info.Length() < 1 || !info[0].IsString()) { Napi::TypeError::New(env, "String expected").ThrowAsJavaScriptException(); return env.Null(); } std::string path = info[0].As();
std::ifstream ifs(path);
if (!ifs.is_open()) {
Napi::TypeError::New(env, "Error: File not found!").ThrowAsJavaScriptException();
return env.Null();
}
std::stringstream ss;
ss << ifs.rdbuf();
return Napi::String::New(env, ss.str());
}
Napi::Object Init(Napi::Env env, Napi::Object exports) {
exports.Set(
Napi::String::New(env, "readFile"),
Napi::Function::New(env, ReadFile));
return exports;
}
Debugging C++ Addons in NodeJS
Debugging C++ can be quite complex, especially if one is transitioning from JavaScript, but there are tools available. NodeJS comes equipped with the GDB debugger, which can be used to debug C++ add-ons.
To use GDB, first begin by compiling your C++ code using the -g flag. This flag informs the compiler to include debugging symbols in the compilation process.
Pros and Cons of using C++ Embedder API in NodeJS
Advantages:
- C++ is a high-performance language and can execute certain tasks much faster than JavaScript.
- It allows for better memory management.
- The C++ Embedder API comes with a vast set of libraries that are not available in common Node packages.
- C++ Embedder API allows for seamless use of platform-specific native libraries (written in C++).
Disadvantages:
- C++ is complex, and as such, transitioning from JavaScript can be a bit daunting for some developers.
- Debugging C++ code is more complicated than debugging JavaScript.
- Writing optimal C++ code requires a lot of expertise and experience.
Conclusion:
C++ Embedded API in NodeJS is a powerful instrument that enables the creation of high-performance web applications. Its advantages outweigh its disadvantages, and with patience and practice, C++ coding can become second nature to a JavaScript developer. The development of C++ add-ons in NodeJS offers an unparalleled degree of freedom and creativity, enabling the creation of intricate programs that are sure to impress even the most seasoned programmers. While the integration of C++ API in NodeJS may seem odd to some developers, it is a powerful tool that should be in every NodeJS developer’s toolbox.
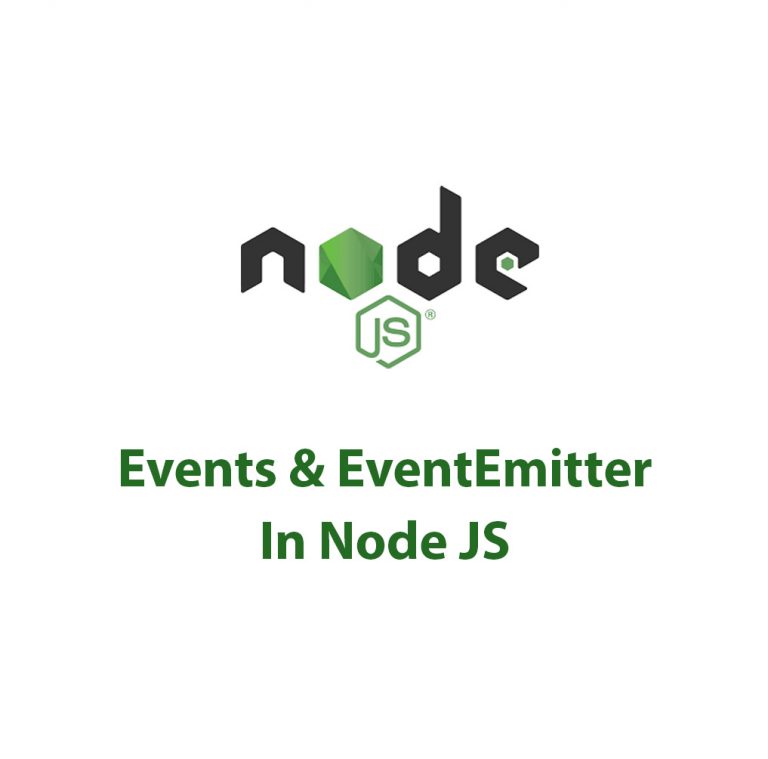
Events In Node JS
Introduction As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought. […]
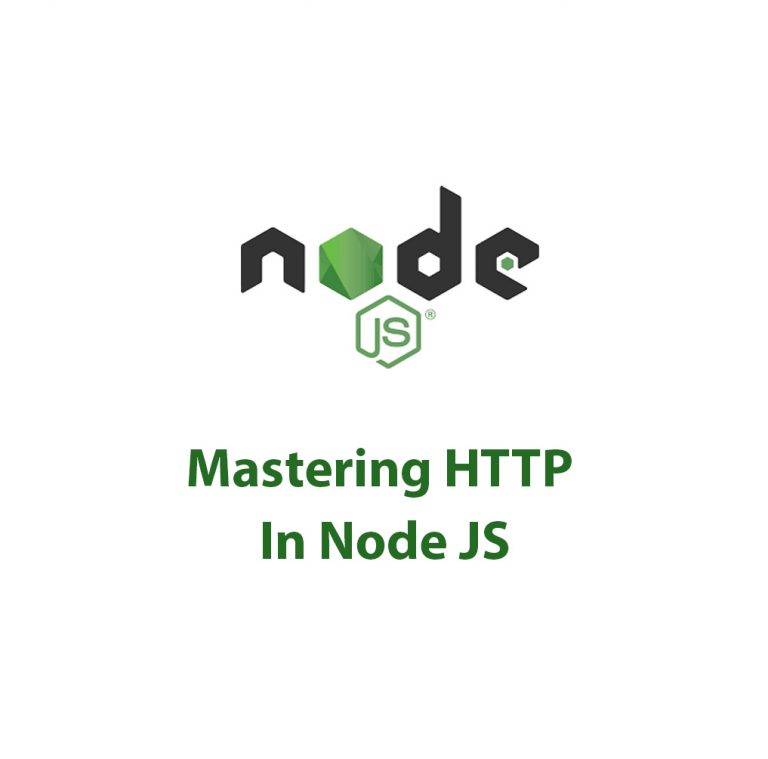
Working With HTTP In Node JS
Introduction As a developer, you may have come across the term HTTP quite a few times. It stands for Hypertext Transfer Protocol and is the backbone of how the internet works. It is the protocol you use when you visit a website, send emails, and watch videos online. In the world of programming, HTTP is […]
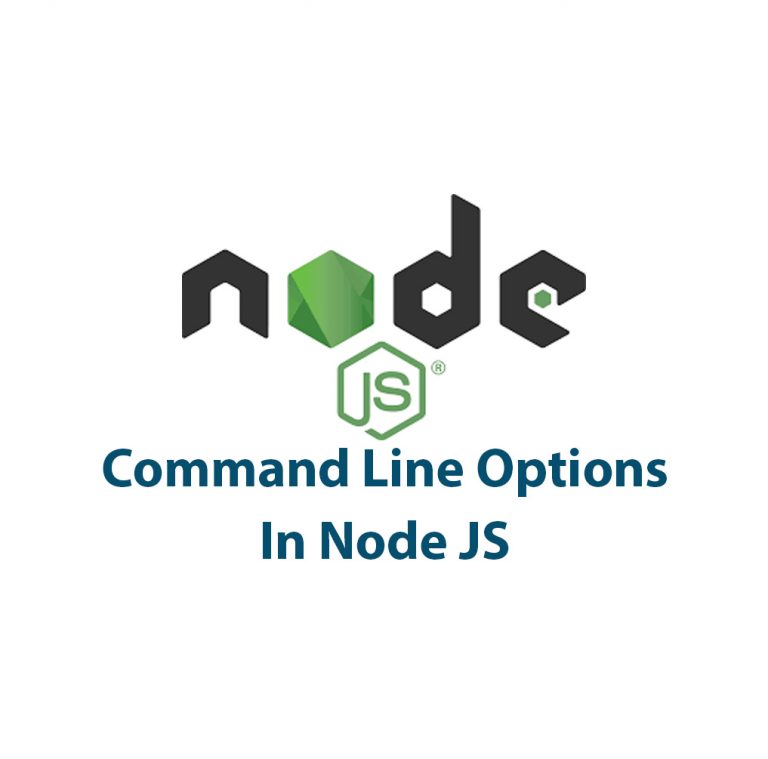
Command Line Options In Node JS
As a developer who loves working with Node JS, I’ve found myself using command line options in many of my projects. Command line options can make our Node JS programs more user-friendly and powerful. In this article, I’ll explain what command line options are, how to parse them in Node JS, how to use them […]
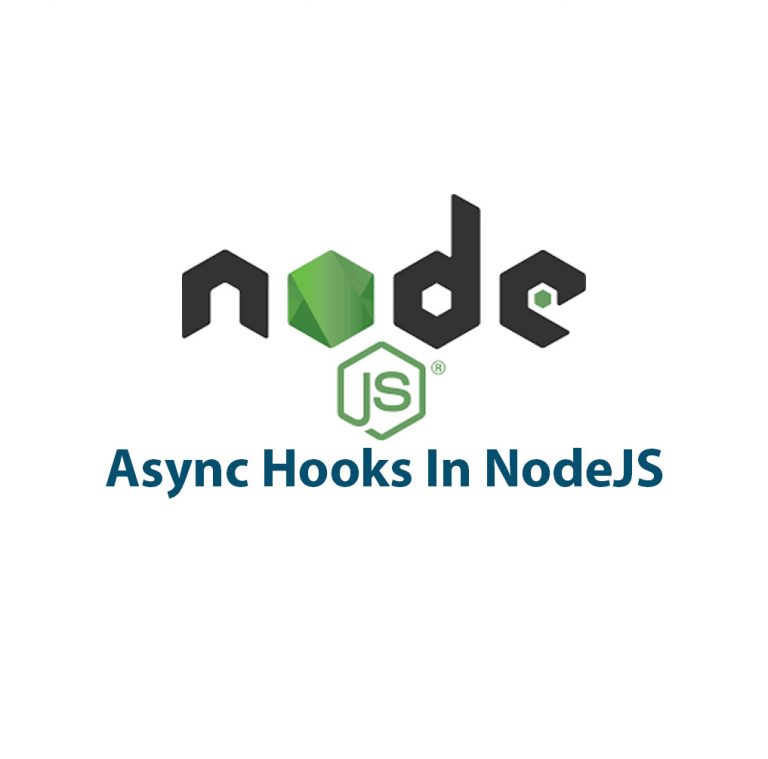
Async Hooks In Node JS
Introduction: If you’re a Node.js developer, you’ve probably heard the term “Async Hooks” thrown around in conversation. But do you know what they are or how they work? In this article, I’ll be diving into the world of Async Hooks, explaining what they are and how to use them effectively. What are Async Hooks? Async […]
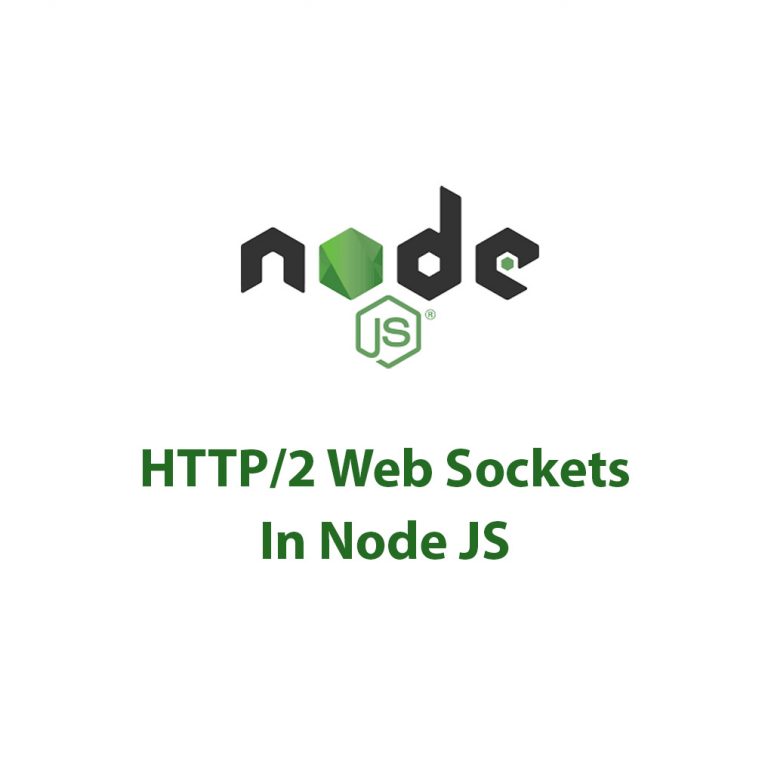
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
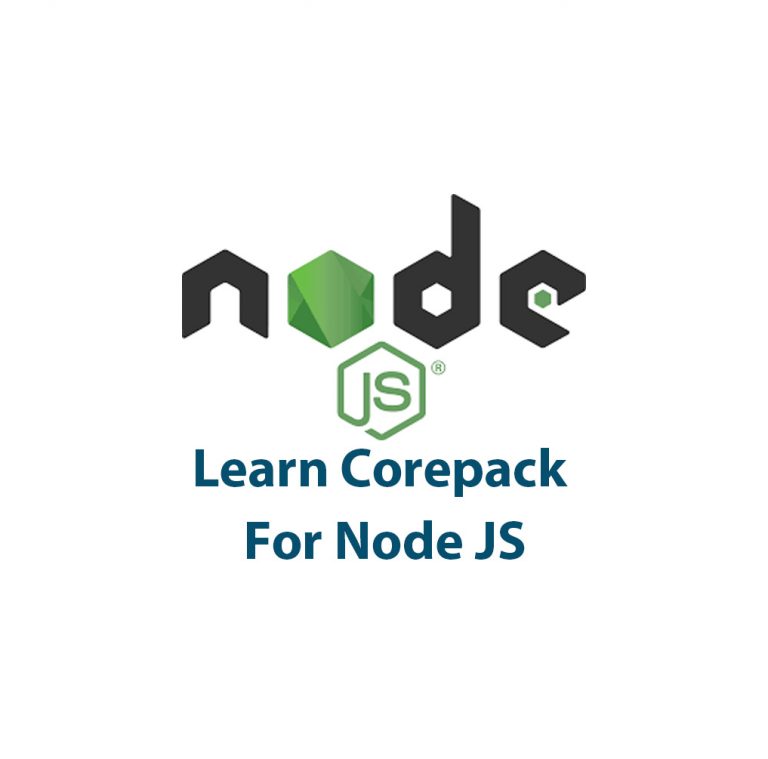
Corepack In Node JS
Have you ever worked on a Node JS project and struggled with managing dependencies? You’re not alone! Managing packages in Node JS can get confusing and messy quickly. That’s where Corepack comes in. In this article, we’ll be diving into Corepack in Node JS and how it can make dependency management a breeze. First of […]