As a developer, I have always been fascinated by the way avatars enhance the user experience in web applications. They provide a personalized touch and make users feel more connected to the platform. That’s why I was excited to learn that Material UI V5 had revamped their avatar component. In this article, I’ll share my journey of exploring the avatars in Material UI V5, and how to incorporate them into your next project.
First, a little background about Material UI V5. It’s a popular styling library for React apps that provides pre-built components for UI development. It follows the Material Design principles, which offer a clean and modern interface. Material UI V5 is full of new features and improvements that make it a powerful tool for building responsive apps. One of these improvements is the revamped avatar component.
So, what exactly are avatars? In a nutshell, avatars are images that represent people. In web applications, avatars can be used to represent users, products, or anything else that needs an image identifier. With Material UI V5 avatar component, you can create beautiful and customizable avatars that perfectly match your application’s aesthetics.
Let’s dive in and see how you can incorporate avatars into your Material UI V5 project.
Setting up Material UI V5
Before we jump into the avatar component, we need to set up our project with Material UI V5. If you haven’t done it already, run the following command in your terminal:
npm install @mui/material
This will install Material UI v5 and the required dependencies. Now, we can dive into the avatar component.
Understanding avatars in Material UI V5
The avatar component is a part of Material UI’s core set of components. As I mentioned earlier, it’s used to represent a person or an entity in an application. Material UI V5 offers several types of avatars, including Image, Icon, and Text avatars. Let’s take a closer look at each type.
- Image avatar: This avatar displays an image as its source. You can use this avatar to represent a user’s profile picture, a product image, or any other image that represents an entity.
- Icon avatar: This avatar displays an icon as its source. You can use this avatar to represent an entity without using an image.
- Text avatar: This avatar creates an image from the initials of a person’s name or any other string value. You can use this avatar as a fallback when an image or icon isn’t available.
Now that we understand the different types of avatars in Material UI V5, let’s see how we can implement them in our project.
Implementing avatars in Material UI V5
The Avatar component is easy to use, and you can customize it to match your application’s design. Let’s take a look at how we can implement each type of avatar.
Using Image avatar
Here’s an example of how you can use the Image avatar component in your project:
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
export default function ImageAvatar() {
return (
<Avatar
src="/static/images/avatar/1.jpg"
alt="avatar"
/>
);
}
In the above example, we’re using the avatar with its image source. You can also customize the size of the avatar by passing the size props:
<Avatar
src="/static/images/avatar/1.jpg"
alt="avatar"
sx={{ width: 56, height: 56 }}
/>
Using the size property, you can make the avatar larger or smaller depending on your needs.
Using Icon avatar
Here’s an example of how you can use the Icon avatar component in your project:
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
import AddCircleIcon from '@mui/icons-material/AddCircle';
export default function IconAvatar() {
return (
<Avatar>
<AddCircleIcon />
</Avatar>
);
}
In the above example, we’re using the Icon avatar with a material icon as its source. You can also customize the size and color of the icon by passing the appropriate props:
<Avatar sx={{ backgroundColor: 'red' }}>
<AddCircleIcon sx={{ fontSize: 40 }} />
</Avatar>
Using the sx property, you can customize the background color of the avatar, and using the fontSize property, you can increase or decrease the size of the icon.
Using Text avatar
Here’s an example of how you can use the Text avatar component in your project:
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
export default function TextAvatar() {
const name = 'John Doe';
return (
<Avatar sx={{ bgcolor: 'purple' }}>JD</Avatar>
);
}
In the above example, we’re using the Text Avatar component to show the initials of a name. You can also customize the background color and font size of the avatar by passing the appropriate props:
<Avatar sx={{ bgcolor: 'orange', fontSize: 24 }}>JD</Avatar>
Using the bgcolor property, you can change the background color of the avatar, and using the fontSize property, you can increase or decrease the size of the initials.
Combining avatars with other Material UI V5 components
The avatar component can be combined with other Material UI components to create a better user experience. Here are some examples of how you can use avatars with other Material UI components:
Avatar with Card component
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
import Card from '@mui/material/Card';
import CardHeader from '@mui/material/CardHeader';
export default function AvatarWithCard() {
return (
<Card>
<CardHeader
avatar={
<Avatar
src="/static/images/avatar/1.jpg"
alt="avatar"
/>
}
title="John Doe"
subheader="Developer"
/>
</Card>
);
}
In the above example, we’re using the Avatar component with the Card component to show information about a user. The Avatar component is used as the user’s profile picture, and the Card component is used to display additional information about the user.
Avatar with List component
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
import List from '@mui/material/List';
import ListItem from '@mui/material/ListItem';
import ListItemAvatar from '@mui/material/ListItemAvatar';
import ListItemText from '@mui/material/ListItemText';
export default function AvatarWithList() {
return (
<List>
<ListItem>
<ListItemAvatar>
<Avatar>
<AddCircleIcon />
</Avatar>
</ListItemAvatar>
<ListItemText primary="Create a project" />
</ListItem>
<ListItem>
<ListItemAvatar>
<Avatar>
<EditIcon />
</Avatar>
</ListItemAvatar>
<ListItemText primary="Edit a project" />
</ListItem>
<ListItem>
<ListItemAvatar>
<Avatar>
<DeleteIcon />
</Avatar>
</ListItemAvatar>
<ListItemText primary="Delete a project" />
</ListItem>
</List>
);
}
In the above example, we’re using the Icon avatar component with the List component to display a list of actions. The Icon avatar component is used to display an icon for each action, and the List component is used to display the text associated with each action.
Avatar with AppBar component
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
import AppBar from '@mui/material/AppBar';
import Toolbar from '@mui/material/Toolbar';
import Typography from '@mui/material/Typography';
export default function AvatarWithAppBar() {
return (
<AppBar position="static">
<Toolbar>
<Typography variant="h6" sx={{ flexGrow: 1 }}>
My App
</Typography>
<Avatar src="/static/images/avatar/1.jpg" alt="avatar" />
</Toolbar>
</AppBar>
);
}
In the above example, we’re using the Image avatar component with the AppBar component to display the user’s profile picture in the header. This provides a more personalized experience for the user and makes the application feel more welcoming.
Advanced techniques for using avatars in Material UI V5
Material UI V5 offers some advanced techniques for using avatars in your project. Here are a few examples:
Using Gravatar with Avatar component
Gravatar is a service that provides users with a unique profile picture based on their email address. You can use Gravatar to generate a profile picture for users who haven’t uploaded an image by themselves. Here’s an example of how to use Gravatar with the Avatar component:
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
import md5 from 'md5';
export default function GravatarAvatar(props) {
const { email, size } = props;
const hash = md5(email);
return (
<Avatar
src={`https://www.gravatar.com/avatar/${hash}?s=${size}&d=identicon`}
alt="avatar"
/>
);
}
In the above example, we’re using the md5 library to generate a hash of the user’s email address. We then pass that hash to the Gravatar URL to generate the user’s profile picture.
Using LazyLoad with Avatar component
In some cases, you may have a large number of avatars on a single page, which can significantly impact the page’s performance. To improve the performance, you can use the LazyLoad library to only load the avatars when they come into the user’s view.
Here’s an example of how to use LazyLoad with the Avatar component:
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
import LazyLoad from 'react-lazyload';
export default function LazyLoadAvatar() {
return (
<LazyLoad height={100} once>
<Avatar
src="/static/images/avatar/1.jpg"
alt="avatar"
/>
</LazyLoad>
);
}
In the above example, we’re using the LazyLoad component from the react-lazyload library to only load the avatar when it comes into the user’s view. We’re also setting the height to 100px to provide a better user experience.
Using SVG images with Avatar component
Material UI V5 also supports SVG images as the source of an avatar. Here’s an example of how to use SVG images with the Avatar component:
import * as React from 'react';
import Avatar from '@mui/material/Avatar';
export default function SvgAvatar() {
return (
<Avatar>
<svg width="100%" height="100%" viewBox="0 0 24 24">
<path
fill="#000000"
d="M20.01 11H13V3.99l-2-2L3.99 11H2v2h3.5c.33 2.75 2.47 5 5.5 5s5.17-2.25 5.5-5h3.01v-2zM12 16.5c-1.93 0-3.5-1.57-3.5-3.5S10.07 9.5 12 9.5s3.5 1.57 3.5 3.5-1.57 3.5-3.5 3.5z"
/>
</svg>
</Avatar>
);
}
In the above example, we’re using SVG images with the Avatar component to display an icon. We’re using the svg element to write our SVG code directly into the component.
Conclusion
In this article, we explored the revamped avatar component in Material UI V5 and how to incorporate avatars into your project. We learned about the different types of avatars, including Image, Icon, and Text avatars. We also learned about using avatars with other Material UI components and some advanced techniques for using avatars, such as Gravatar, LazyLoad, and SVG images.
Material UI V5’s avatar component is a powerful tool for building web applications. It provides a personalized touch and makes users feel more connected to the platform. I hope this article has given you some ideas on how you can use avatars in your next project.
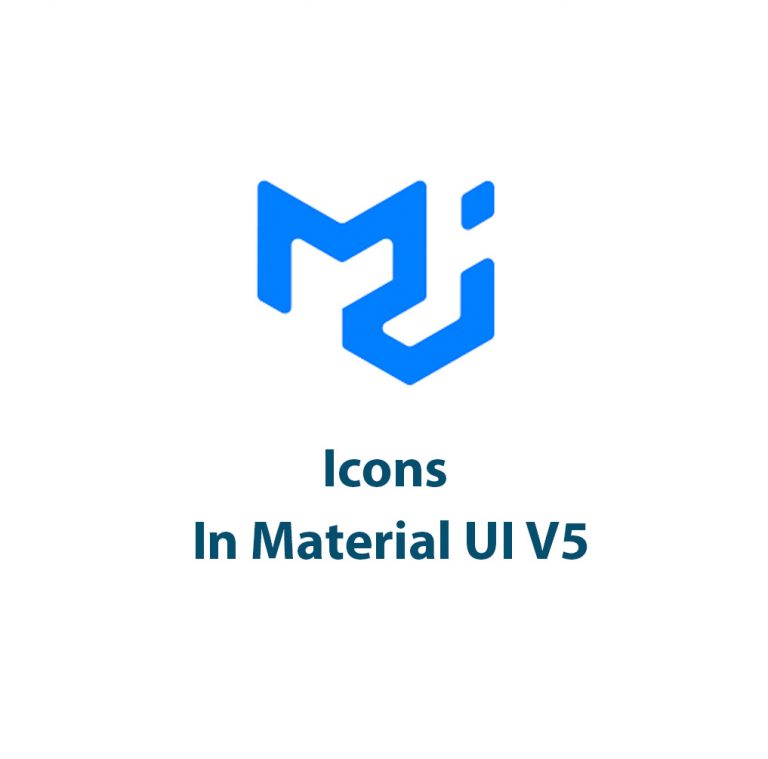
Icons In Material UI V5
Introduction: Material UI is a popular open-source framework for building web applications. Built on top of React, it provides a set of pre-built and customizable components, including typography, forms, and icons. In Material UI, icons play a crucial role in designing visually appealing interfaces and enabling smooth user experience. With the recent release of Material […]
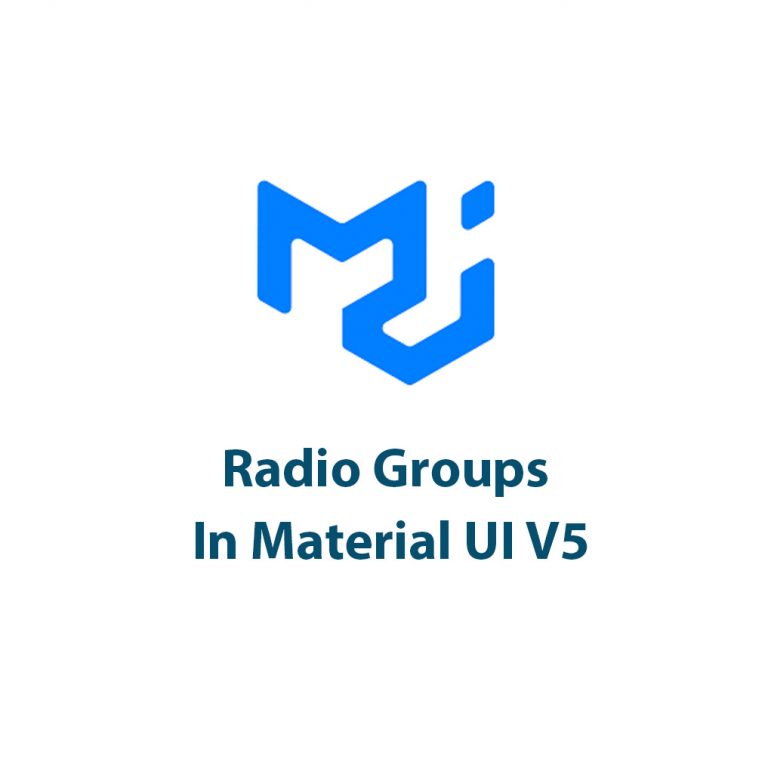
Radio Groups In Material UI V5
Introduction Hello everyone! Material UI has long been one of my favorite UI libraries, and with the recent release of version 5, I thought it would be a great time to explore one of its fundamental components: Radio Groups. In this article, we’ll dive into what Radio Groups are, how they can be used in […]
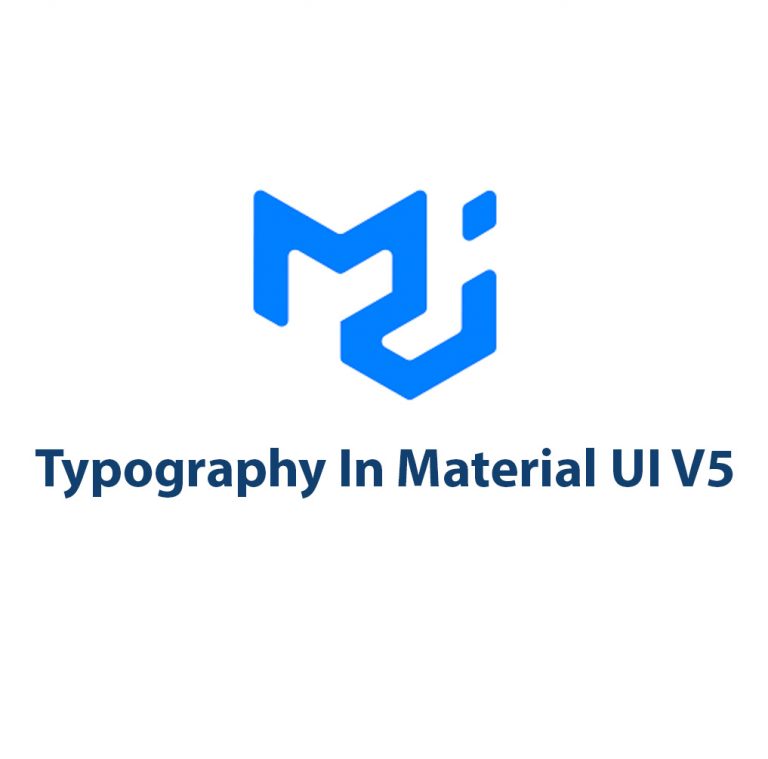
Typography In Material UI V5: A Closer Look
Introduction As a designer and developer, I’ve always been obsessed with typography. There’s something special about the way certain fonts look on a page or screen that can make or break the overall success of a design. As Material UI continues to evolve and improve, the latest version, Material UI V5, offers some exciting changes […]
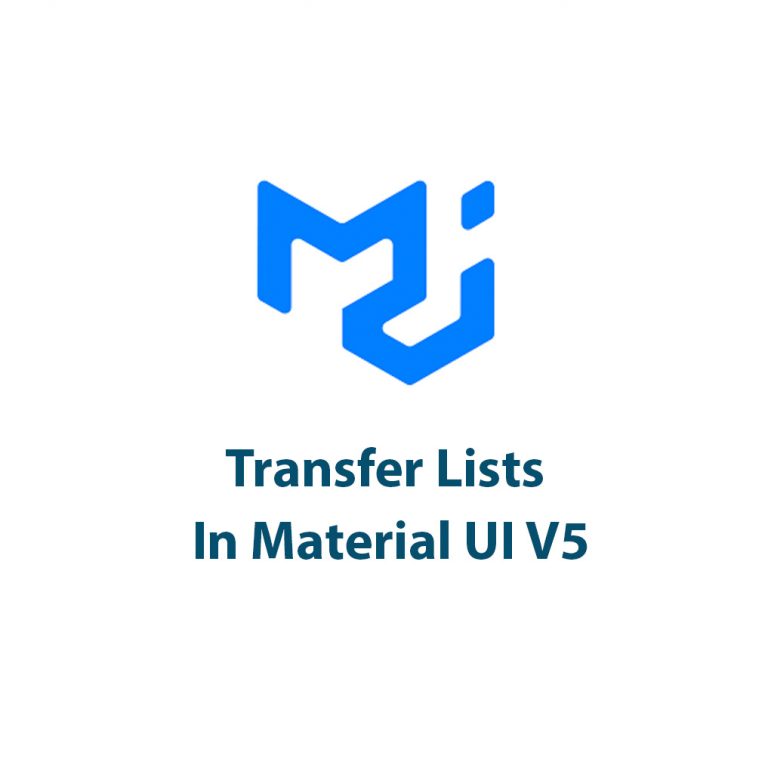
Transfer Lists In Material UI V5
Introduction I remember the first time I stumbled upon transfer lists while working on a project. I was perplexed by the concept, but as I delved deeper, I realized the tremendous benefits of using transfer lists in web development. With the release of Material UI v5, the developers have made it even easier to incorporate […]
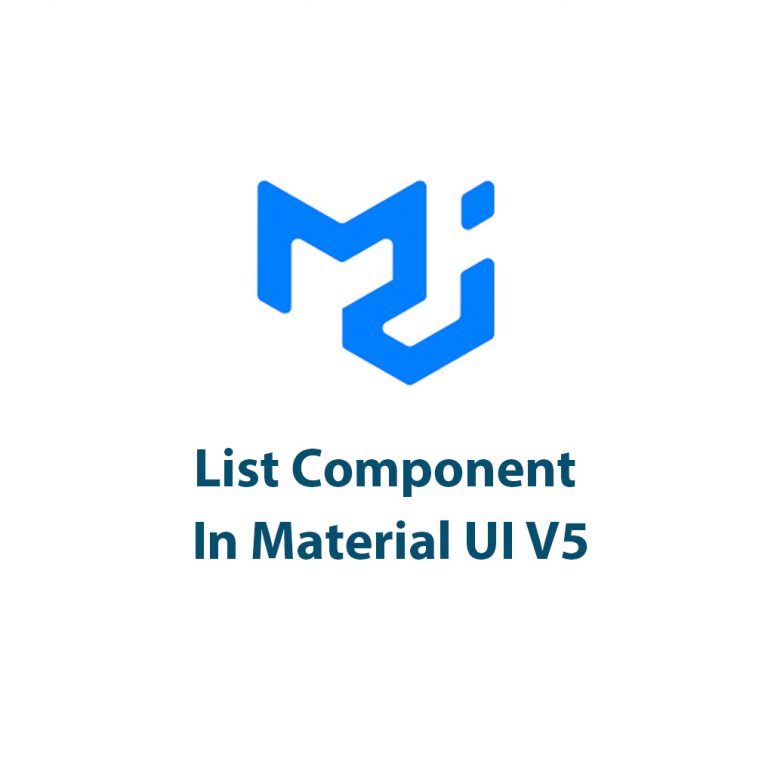
Lists In Material UI V5
Introduction As a UX designer and front-end developer, I’m always on the lookout for tools and libraries that make my job easier. When I first discovered Material UI, I was impressed by how it simplified UI development and improved the consistency of my designs. In this article, I want to focus specifically on lists in […]
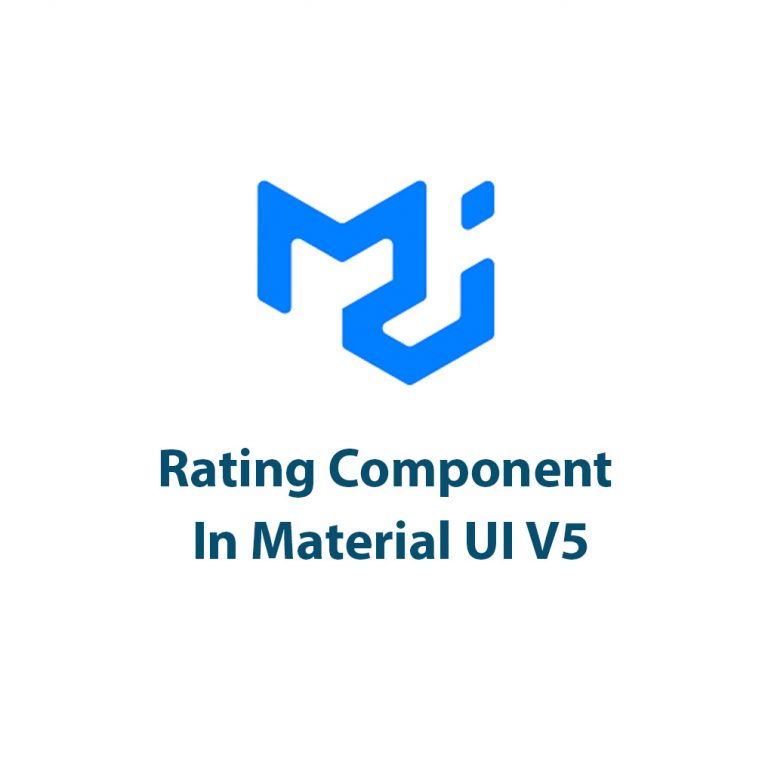
Rating Component In Material UI V5
Hello and welcome to this exciting article about the Rating Component in Material UI V5! As a web developer, I have come to really appreciate the simplicity and flexibility that this UI library provides, especially when it comes to components that add interactivity to user interfaces. In this article, I’m going to walk you through […]