Have you ever wondered how some of the most secure websites and applications keep your data safe from malicious attacks? Well, one of the answers lies in the use of cryptography! Cryptography is the art of writing or solving codes and ciphers, and it has been around for centuries. In the world of computer science, cryptography plays a major role in ensuring data security and privacy.
In this article, we will explore the Crypto package in Node JS and how it can be used for secure cryptography. As a developer, I always strive to make sure the data of my users is secure, and that’s why I’m excited to share with you my knowledge of cryptography and Node JS.
Let’s start by understanding what the Crypto package is and how it can be installed and used in a Node JS project.
What is the Crypto Package?
The Crypto package is a built-in module in Node JS that provides cryptographic functionality. It can be used for a variety of purposes, such as hashing, encryption, decryption, and digital signatures. The Crypto package is built on top of OpenSSL, which is a full-featured open-source toolkit for SSL/TLS encryption.
To use the Crypto package, you first need to install Node JS on your system. Once Node JS is installed, the Crypto package is readily available. You can import the Crypto package into your Node JS project using the following line of code:
const crypto = require('crypto');
With this simple line of code, you can access all the cryptographic functionality provided by the Crypto package.
Hashing
Hashing is a process of converting data of any size into a fixed size output known as a hash. The hash can then be used to verify the identity of the data, as any small change in the input data will produce a vastly different hash. In other words, hashing is a one-way function that is used to provide an additional layer of security to sensitive data, such as passwords.
With the Crypto package, hashing is as simple as calling the createHash()
function and passing in the type of hash you wish to use. Here’s an example:
const crypto = require('crypto');
const myData = 'Data to be hashed';
const hash = crypto.createHash('sha256').update(myData).digest('hex');
console.log(hash);
In the above code snippet, we create a variable myData
that holds the data we want to hash. Then we call the createHash()
function and pass in the type of hash we want to use, which in our example is SHA-256. We then call the update()
function and pass in our data to be hashed. Finally, we call the digest()
function and pass in the format we want the hash to be returned in, which in our case is hexadecimal.
The output of the code snippet will be the hash of our data in hexadecimal format.
Hashing is often used to hash passwords for storage in a database. When a user signs up for an account on a website, for example, their password is hashed and stored in the database. When the user logs in, their password is hashed again, and the result is compared with the stored hash to determine if the password is correct.
Encryption
Encryption is a process of converting plain text into a ciphertext that can only be read by someone who has the key to decrypt it. It is one of the most widely used methods of securing data in transit and at rest. The Crypto package in Node JS provides a variety of encryption algorithms that can be used to encrypt data.
To encrypt data using the Crypto package, we use the createCipheriv()
function. Here’s an example:
const crypto = require('crypto');
const myData = 'Data to be encrypted';
const key = crypto.randomBytes(32);
const iv = crypto.randomBytes(16);
const cipher = crypto.createCipheriv('aes-256-cbc', key, iv);
let encrypted = cipher.update(myData);
encrypted = Buffer.concat([encrypted, cipher.final()]);
console.log('Encrypted:', encrypted.toString('hex'));
In this example, we first create a variable myData
that holds the data we want to encrypt. We then generate a random key and initialization vector (IV) using the randomBytes()
function. The key and IV are used to encrypt the data using the createCipheriv()
function with the AES-256-CBC encryption algorithm. The update()
function is called to encrypt the data, and the final()
function is called to finalize the encryption. Finally, the encrypted data is concatenated and returned in hexadecimal format.
Decryption is just as easy with the Crypto package. To decrypt the data, we use the createDecipheriv()
function. Here’s an example:
const crypto = require('crypto');
const encrypted = 'Encrypted data in hexadecimal format';
const key = crypto.randomBytes(32);
const iv = crypto.randomBytes(16);
const decipher = crypto.createDecipheriv('aes-256-cbc', key, iv);
let decrypted = decipher.update(Buffer.from(encrypted, 'hex'));
decrypted = Buffer.concat([decrypted, decipher.final()]);
console.log('Decrypted:', decrypted.toString());
In this example, we first create a variable encrypted
that holds the data we want to decrypt in hexadecimal format. We then generate a random key and IV using the randomBytes()
function. The key and IV are used to decrypt the data using the createDecipheriv()
function with the AES-256-CBC encryption algorithm. The update()
function is called to decrypt the data, and the final()
function is called to finalize the decryption. Finally, the decrypted data is concatenated and returned in plain text format.
Digital Signatures
Digital signatures are a way to ensure that a piece of data has not been tampered with and that it came from a trusted source. The Crypto package in Node JS provides a way to create and verify digital signatures using public key cryptography.
To create a digital signature, we use the createSign()
function. Here’s an example:
const crypto = require('crypto');
const myData = 'Data to be signed';
const privateKey = crypto.generateKeyPairSync('ec', {
namedCurve: 'sect239k1',
publicKeyEncoding: {
type: 'spki',
format: 'pem'
},
privateKeyEncoding: {
type: 'pkcs8',
format: 'pem',
},
}).privateKey;
const sign = crypto.createSign('SHA256');
sign.write(myData);
sign.end();
const signature = sign.sign(privateKey, 'hex');
console.log('Signature:', signature);
In this example, we first create a variable myData
that holds the data we want to sign. We then generate a random private key using the generateKeyPairSync()
function with the ECDSA algorithm. We then create a signer using the createSign()
function, passing in the SHA-256 hash algorithm. The write()
function is called to write the data to be signed, and the end()
function is called to finalize the signature. Finally, the signature is returned in hexadecimal format.
To verify a digital signature, we use the createVerify()
function. Here’s an example:
const crypto = require('crypto');
const myData = 'Data to be signed';
const publicKey = crypto.generateKeyPairSync('ec', {
namedCurve: 'sect239k1',
publicKeyEncoding: {
type: 'spki',
format: 'pem'
},
privateKeyEncoding: {
type: 'pkcs8',
format: 'pem',
},
}).publicKey;
const signature = 'Signature in hexadecimal format';
const verify = crypto.createVerify('SHA256');
verify.write(myData);
verify.end();
console.log('Verified:', verify.verify(publicKey, signature, 'hex'));
In this example, we first create a variable myData
that holds the data we want to verify the signature of. We then generate a random public key using the generateKeyPairSync()
function with the ECDSA algorithm. We then create a verifier using the createVerify()
function, passing in the SHA-256 hash algorithm. The write()
function is called to write the data to be verified, and the end()
function is called to finalize the verification. Finally, the verify()
function is called with the public key and signature to verify the signature.
Conclusion
In conclusion, the Crypto package in Node JS provides a powerful set of tools for secure cryptography. From hashing and encryption to digital signatures, the Crypto package offers a wide range of functionalities that can be used to protect sensitive data in your applications. As a developer, it is crucial to understand the importance of cryptography and how it can enhance the security of your applications. I hope this article has provided useful insights into the world of cryptography and how it is implemented in Node JS.
If you’re interested in further exploring cryptography with Node JS, there are many resources available that can help you dive deeper into the topic. Keep learning and exploring new technologies to become a better developer!
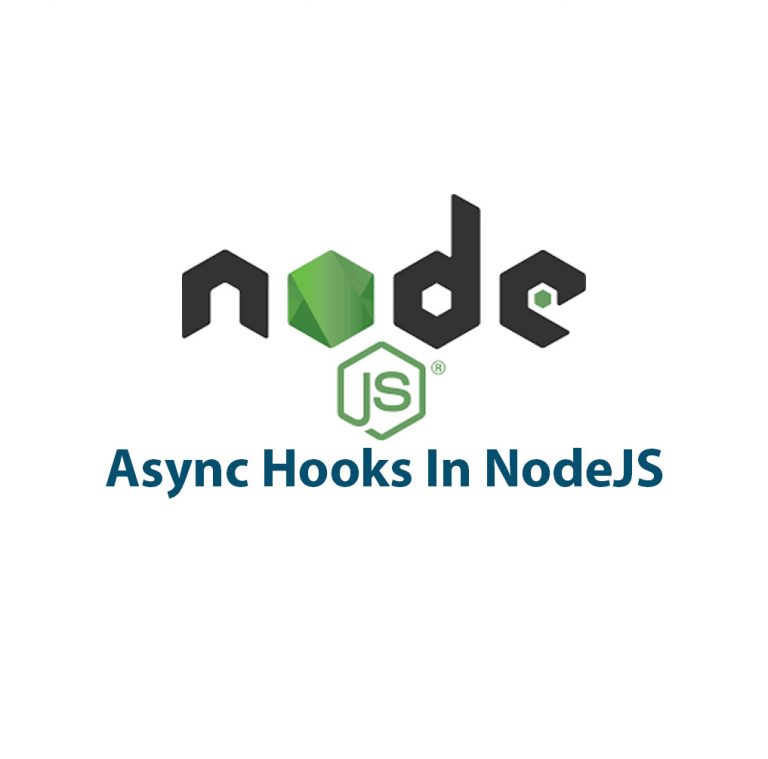
Async Hooks In Node JS
Introduction: If you’re a Node.js developer, you’ve probably heard the term “Async Hooks” thrown around in conversation. But do you know what they are or how they work? In this article, I’ll be diving into the world of Async Hooks, explaining what they are and how to use them effectively. What are Async Hooks? Async […]
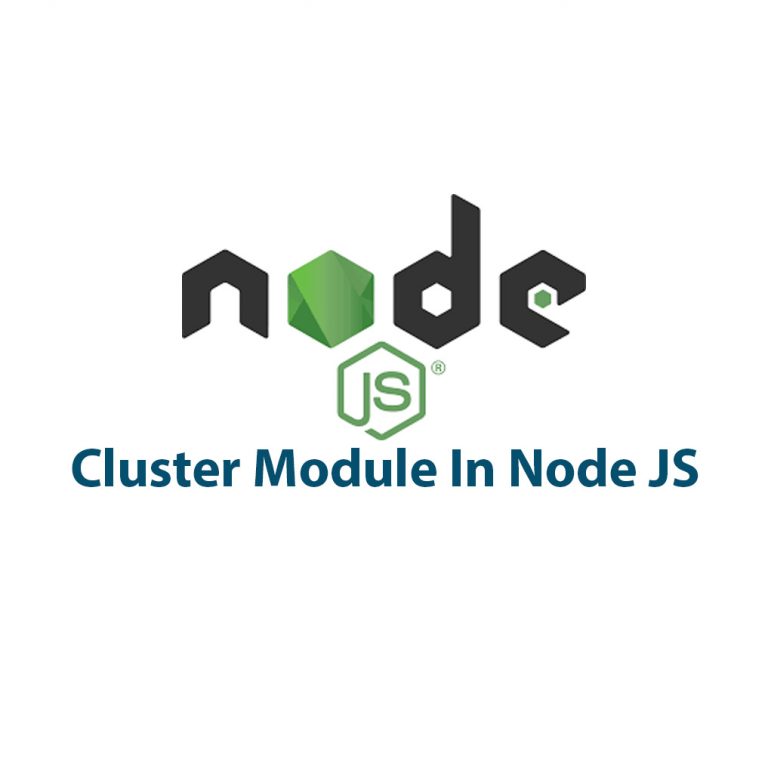
How To Cluster In Node JS
As a developer, I’ve always been interested in exploring different ways to improve the performance of my Node JS applications. One tool that has proven to be immensely beneficial is cluster module. In this article, we’ll dive deep into clustering for Node JS, how it works, how to implement it, and the benefits it provides. […]
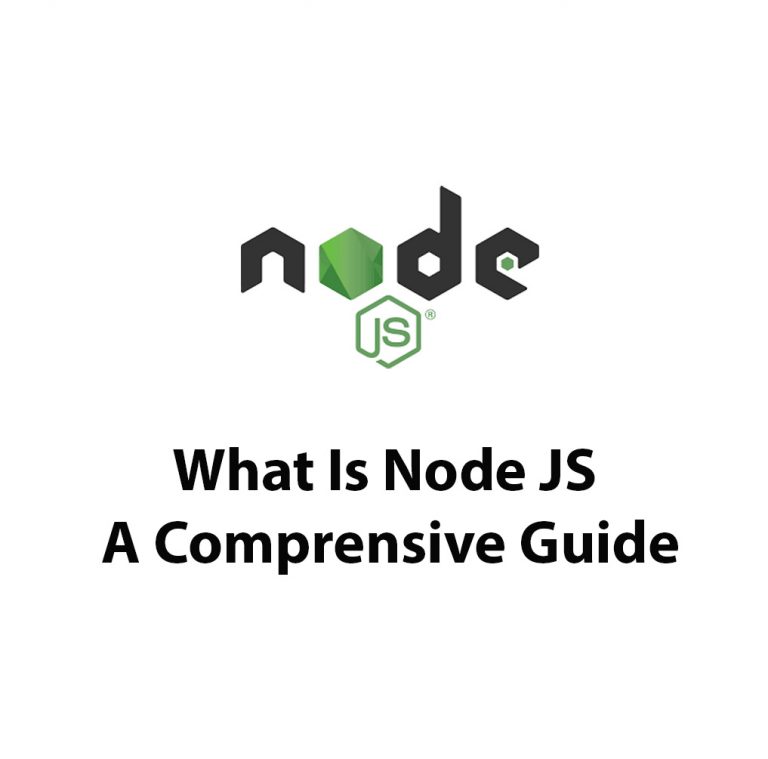
What Is Node JS: A Comprehensive Guide
Introduction As a full-stack developer, I have been working with various technologies over the past few years. But one technology that has caught my attention recently is NodeJS. With its event-driven and non-blocking I/O model, NodeJS has become an excellent choice for building real-time and highly-scalable applications. So, what is NodeJS, and how does it […]
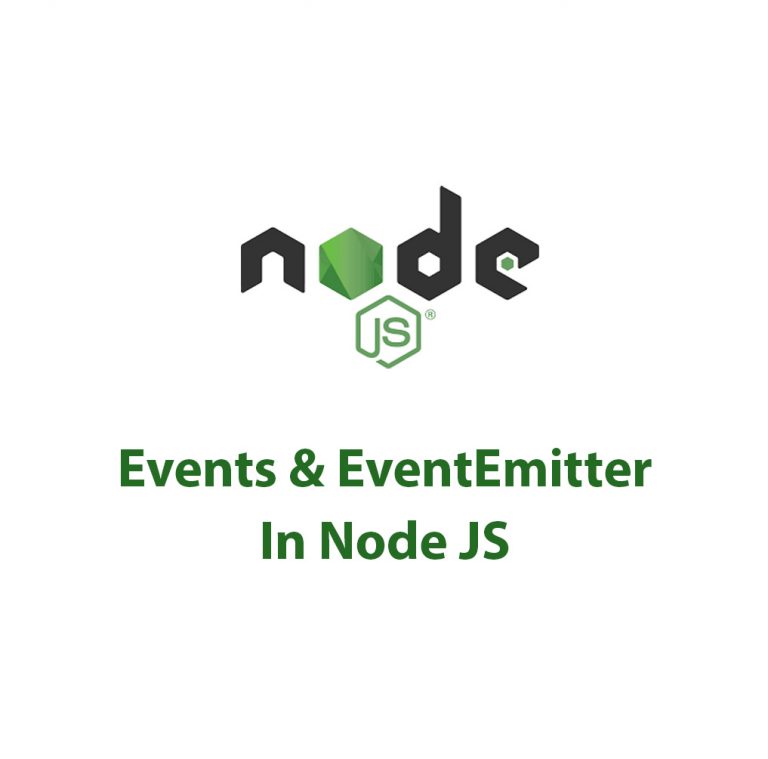
Events In Node JS
Introduction As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought. […]
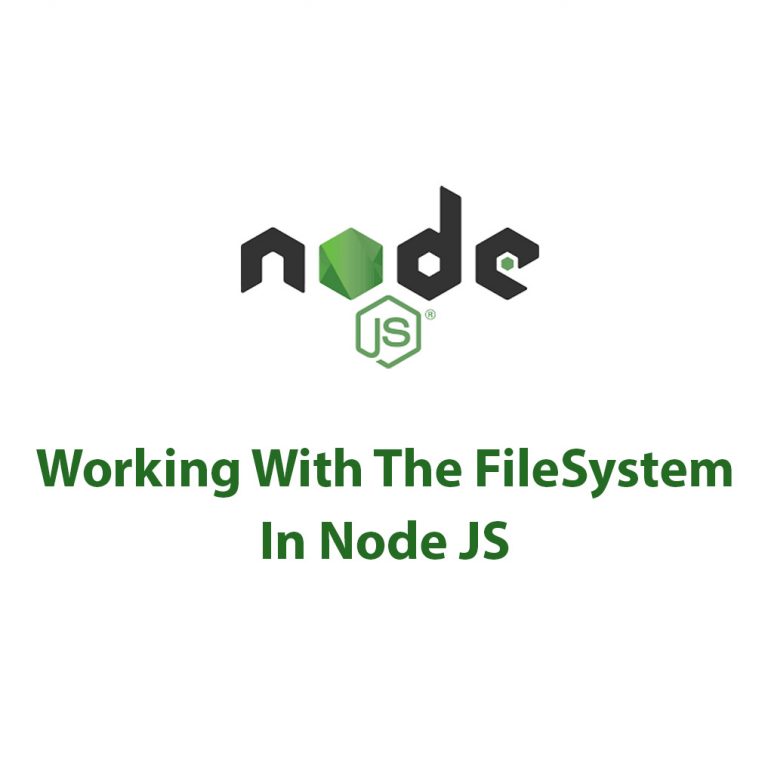
Working With The FileSystem In Node JS
Working With The FileSystem In Node.js Node.js is a powerful platform for building web applications and backend services. One of the most common things that developers need to do is work with files and the file system. This involves creating, modifying, and deleting files, as well as reading from and writing to them. In this […]
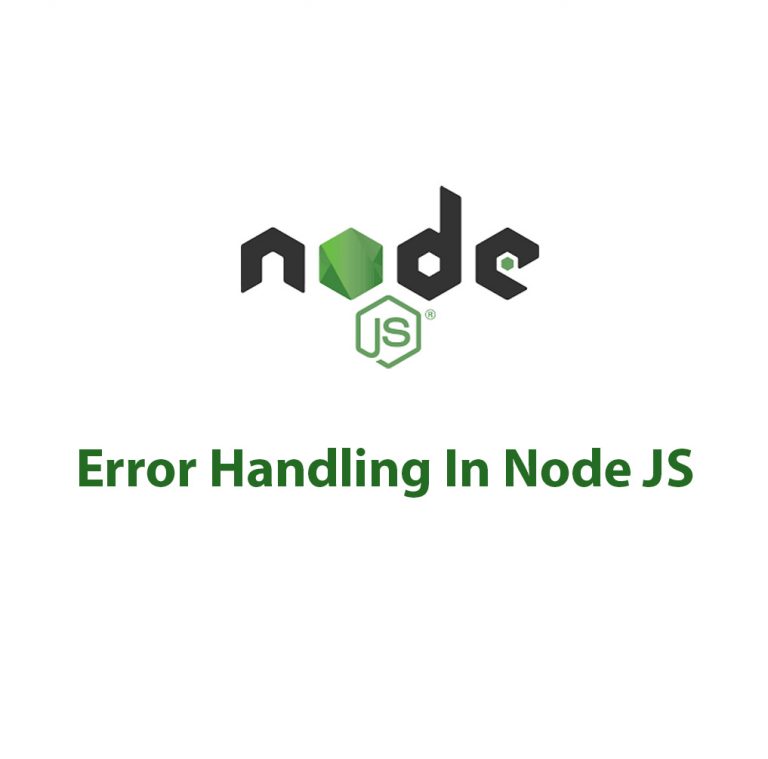
Error Handling in Node JS
A Node JS developer may struggle with error handling on occasion. Although faults are occasionally unavoidable, you can ensure that your application continues to function flawlessly by implementing error handling correctly. Let’s start by discussing the many kinds of problems you could run into when working with Node JS. The Three Types of Errors in […]