Introduction
As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has had a huge impact on modular code is CommonJS, and in this article we’ll explore how CommonJS modules work within the Node.js platform.
What Are CommonJS Modules
Before we dive in too deep, let’s take a step back and define what exactly we mean when we say “CommonJS”. Essentially, CommonJS is a set of standards that define how modules should be written and used in JavaScript. The goal of CommonJS is to provide a standardized way for developers to create and share reusable code components in JavaScript.
Now, let’s move on to the specific implementation of CommonJS that we’re interested in: Node.js. Node.js is an environment for running JavaScript on the server side, and it’s become one of the most popular platforms for web development in recent years. One of the key features of Node.js is its module system, which is based on CommonJS conventions.
So, how does this module system work? At its core, CommonJS modules rely on two key concepts: defining a module, and exporting that module for use in other parts of the code. Let’s take a closer look at each of these concepts.
Creating CommonJS Modules In Node JS
Defining a module is pretty straightforward – essentially, a module is just a JavaScript file with some defined behavior. CommonJS modules are structured in a very specific way: they must have a defined “exports” object, which will contain all of the functions and variables that the module exposes to other parts of the code.
Here’s an example of a simple CommonJS module definition:
// math.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
module.exports = {
add,
subtract
};
In this example, we’re defining a module called “math.js” that contains two functions, “add” and “subtract”. We’re also defining an object that exports these two functions, using the “module.exports” syntax. We could then use this module in another part of our code like so:
// app.js
const math = require('./math');
console.log(math.add(2, 3)); // Output: 5
In this example, we’re using the “require” function to load the “math.js” module into our “app.js” file. The “math” variable then contains the exported object from the “math.js” module, which we can use to call the “add” function.
This is all well and good for simple modules like this one, but what about more complex situations? What if we have multiple modules that depend on each other, or if we need to dynamically load modules based on user input?
Luckily, the CommonJS module system has some built-in features that make these scenarios possible. One such feature is the ability to require modules dynamically, using the “require” function.
Here’s an example:
// app.js
const fs = require('fs');
const modulePath = './' + process.argv[2];
const myModule = require(modulePath);
console.log(myModule.myFunction());
In this example, we’re using the native Node.js “fs” module to read a file path from the command-line arguments. We then use that path to dynamically load a module at runtime, using the “require” function. This allows us to write code that is more flexible and adaptable – we can create new modules on the fly, or even load modules based on user input.
Circular Dependencies
Another feature of the CommonJS module system is the ability to handle circular dependencies. This is a situation where two or more modules depend on each other – for example, module A might use a function from module B, while module B uses a function from module A.
While circular dependencies can be tricky to manage in some systems, the CommonJS module system handles them elegantly. Essentially, each module is loaded into memory as-needed, and the “exports” object is only populated once all of the dependencies have been resolved. This means that circular dependencies can be resolved automatically, without the need for complex workarounds or hacks.
Of course, while the CommonJS module system is powerful and flexible, it’s not without its drawbacks. One of the biggest issues with CommonJS is that it’s a synchronous system – that is, modules are loaded one at a time, in the order that they’re required.
This can lead to performance issues in some situations, as the module system can become a bottleneck for large, complex programs. Additionally, the synchronous nature of the system can make it difficult to write code that is truly concurrent or asynchronous.
That being said, there are ways to work around these issues. For example, some developers have created tools and libraries that allow for asynchronous loading of modules, which can greatly improve performance in some scenarios.
Conclusion
At the end of the day, it’s clear that the CommonJS module system has had a significant impact on the world of JavaScript development. Whether you’re building a large-scale web application or just a simple script, understanding how CommonJS and Node.js work together can help you create more powerful and flexible code.
So go forth, experiment with CommonJS modules in Node.js, and don’t be afraid to get creative – you never know what kind of amazing things you might discover!
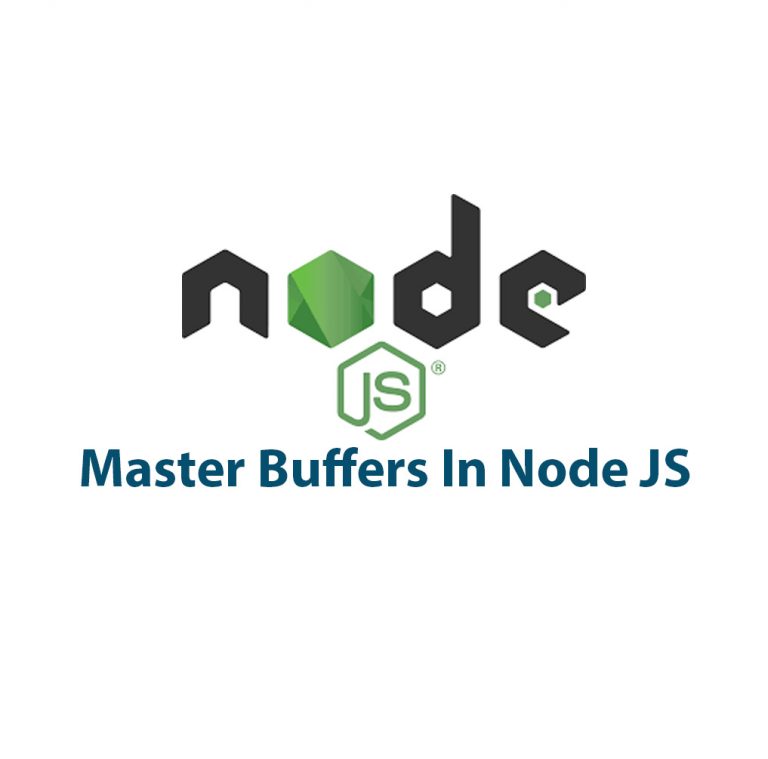
Mastering Buffers In Node JS
As someone who is just getting started with Node JS, hearing about buffers might be a bit confusing at first. What are they exactly, and why should you care about them? I know I was pretty perplexed by this concept when I first started working with Node JS. However, once I understood what buffers were […]
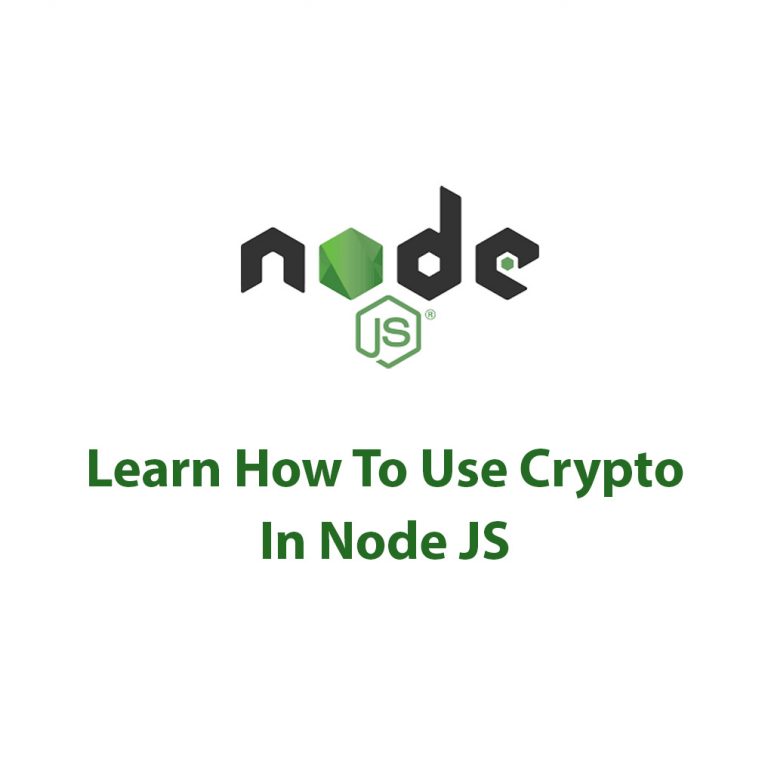
Using Crypto In Node JS
Have you ever wondered how some of the most secure websites and applications keep your data safe from malicious attacks? Well, one of the answers lies in the use of cryptography! Cryptography is the art of writing or solving codes and ciphers, and it has been around for centuries. In the world of computer science, […]
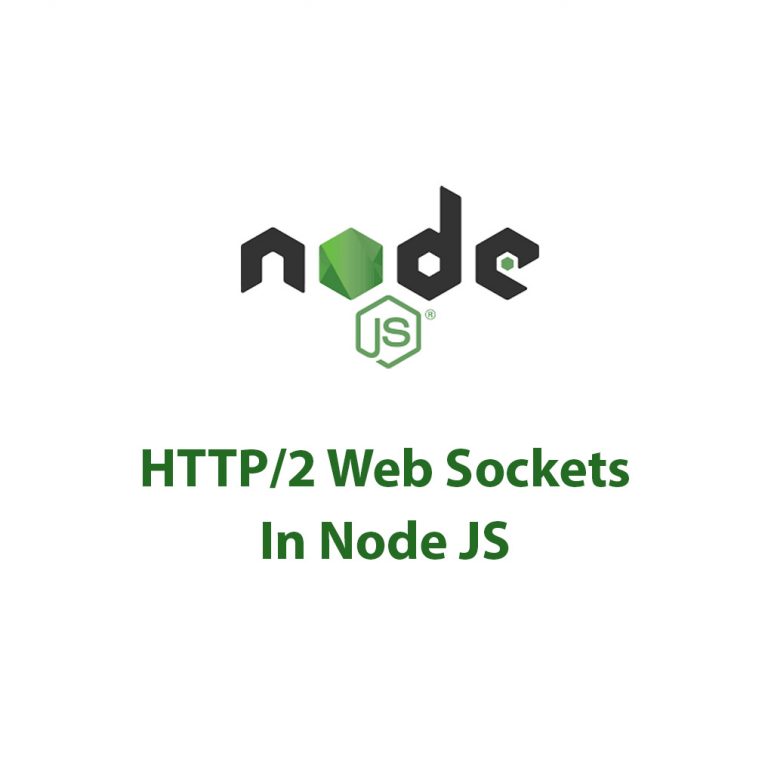
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
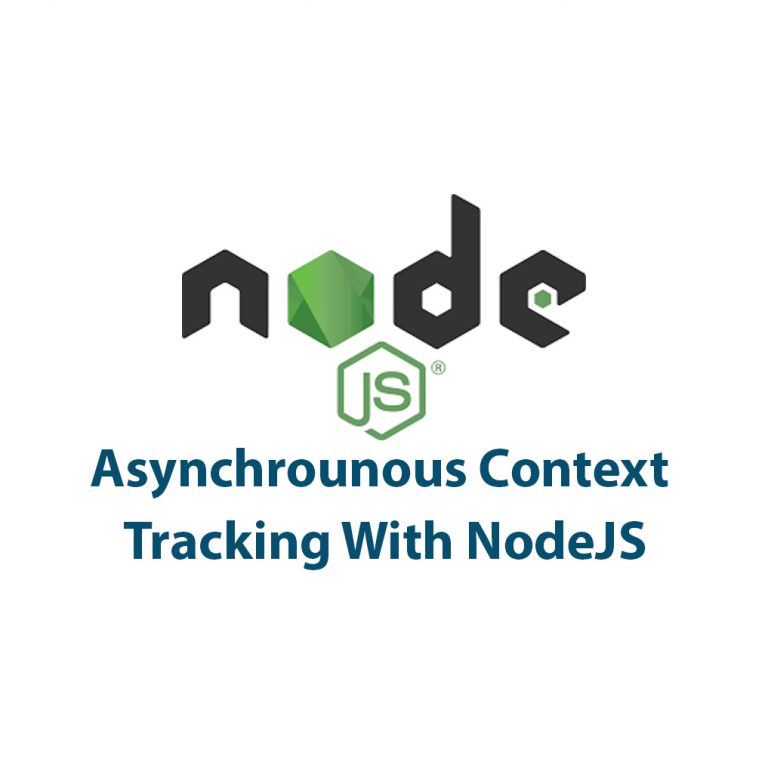
Asynchronous Context Tracking With Node JS
As someone who has spent a lot of time working with Node JS, I have come to understand the importance of Asynchronous Context Tracking in the development of high-performance applications. In this article, I will explore the concept of Asynchronous Context Tracking with Node JS, its advantages, techniques, challenges and best practices. Before we dive […]
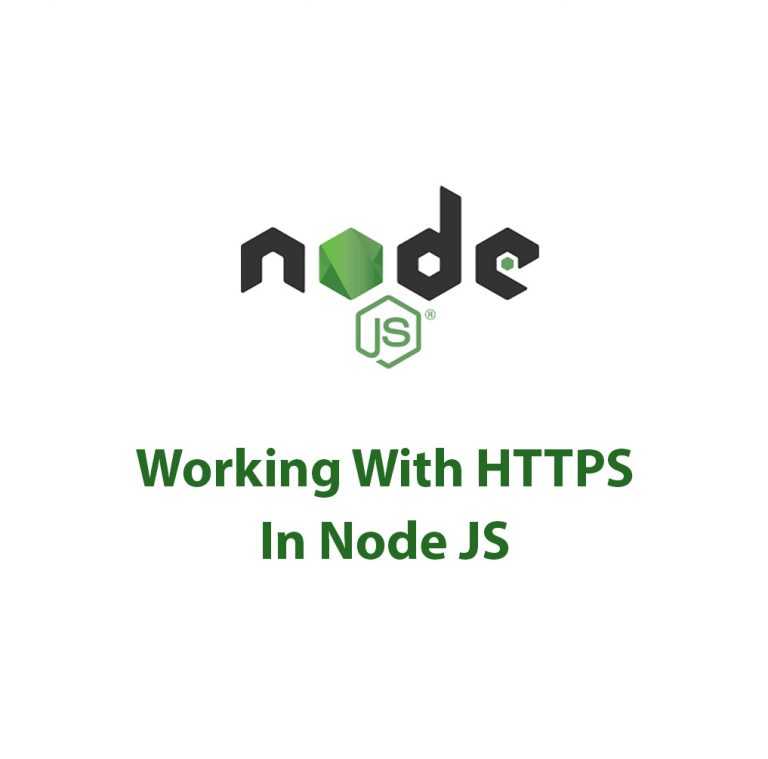
Working With HTTPS In Node JS
As a developer, I’m always on the lookout for security protocols that can help me protect users’ sensitive information. HTTP, while functional, lacks the encryption necessary to truly secure data transmission over the web. This is where HTTPS comes in. But working with HTTPS can be a bit daunting, especially if you’re new to it. […]
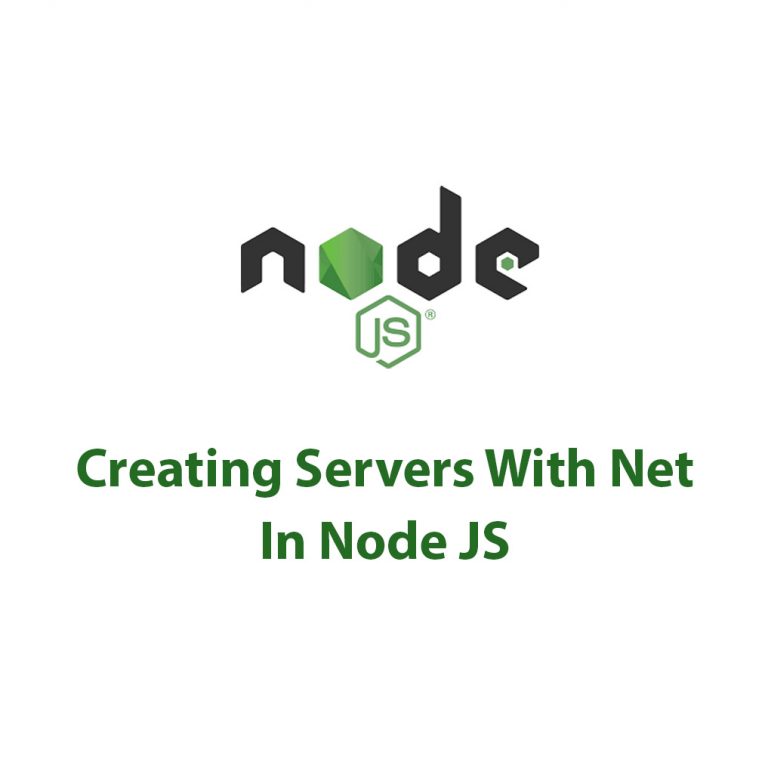
Working With The Net Package In Node JS
As a Node.js developer, I have always been fascinated by the vast array of modules and packages that can be used to simplify the development process. One such package that has long intrigued me is the Net package. In this article, I’ll delve deep into what the Net package is, how to set it up, […]