As a software developer, I am constantly looking for ways to improve the efficiency and effectiveness of my work. One of the most powerful tools in my arsenal has been typescript decorators. In this article, I will dive deep into the world of typescript decorators, exploring their history, various types, and usage in software development.
What Are Typescript Decorators?
Typescript decorators are a programming concept that allows developers to add metadata to a specific class, method, property or parameter. In other words, they are a way of adding custom attributes to certain features of your code. For example, you might use a decorator to specify the type of a particular element, the default value of a property, or the access level of a method.
Typescript decorators have been available since version 1.5 of the language, which was released in July of 2015. They are based on an older feature in JavaScript called “decorators,” which was introduced in the ES2015 (ES6) specification. However, typescript decorators go beyond the capabilities of their JavaScript counterparts, offering more advanced functionality for complex applications.
Why Are Typescript Decorators Important?
So why exactly are typescript decorators so important? There are a number of key benefits that come with using decorators in your code. First and foremost, they can help you write more readable and maintainable code. By adding metadata to different parts of your code, you can more easily understand what each element does and why it’s there.
Additionally, typescript decorators can help you enforce certain behaviors within your code. For example, you might use a decorator to ensure that a specific method can only be called by certain types of users or that a particular property can never be set to null. By adding these kinds of rules to your code, you can reduce the likelihood of bugs and improve overall code quality.
Another advantage of typescript decorators is that they can save you time and effort during development. Instead of having to manually add the same code to multiple elements in your codebase, you can simply apply a decorator to achieve the same effect. This can help to streamline your development workflow and allow you to focus on other, more pressing tasks.
How to start using Typescript Decorators
Now that we’ve discussed what Typescript Decorators are, why they are useful, and some of the risks and limitations associated with using them, let’s look at how you can start using decorators in your own projects.
- Update to the latest version of Typescript
To use Typescript Decorators, you will need to be using the latest version of Typescript. Be sure to check the Typescript website to make sure that you are running the latest version of Typescript.
- Enable the experimentalDecorators flag
To use Typescript Decorators, you will need to enable the experimentalDecorators flag in your Typescript compiler options. You can do this by adding the following line to your tsconfig.json file:
"compilerOptions": {
"experimentalDecorators": true
}
Types of Typescript Decorators
Now that we’ve explored the benefits of typescript decorators, let’s take a closer look at the different kinds of decorators available within the language.
Class Decorators
Class decorators are applied to the top-level class declaration and can be used to modify the behavior of the entire class. For example, you might use a class decorator to add some additional functionality to all instances of a particular class.
One common use case for class decorators is to implement mixins. A mixin is essentially a way of adding functionality to an existing class without having to modify its original source code. By using a class decorator, you can create a new class that “mixes in” the functionality of the original class, without actually modifying the original class itself.
Here’s an example of a class decorator that implements a simple mixin:
function MyMixin<T extends { new (...args: any[]): {} }>(constructor: T) {
return class extends constructor {
newProperty = "new property";
hello = "override";
};
}
@MyMixin
class MyClass {
hello = "world";
constructor() {}
}
console.log(new MyClass());
In this example, the MyMixin
decorator adds a new property and overrides the hello
property of the MyClass
class. When the MyClass
class is instantiated, the resulting object will contain both the original MyClass
properties and those added by the MyMixin
decorator.
Method Decorators
Method decorators are applied to individual method declarations and can be used to modify the behavior of those methods. For example, you might use a method decorator to log information about a method’s execution or to enforce certain preconditions before the method is called.
One common use case for method decorators is to implement caching. By adding a caching decorator to a particular method, you can cache the results of that method’s execution and return those cached results instead of re-executing the method every time it’s called.
Here’s an example of a caching method decorator:
function Memoize() {
const cache = {};
return (target: Object, key: string, descriptor: PropertyDescriptor) => {
const originalMethod = descriptor.value;
descriptor.value = function (...args) {
const cacheKey = JSON.stringify(args);
if (cache[cacheKey]) {
return cache[cacheKey];
}
const result = originalMethod.apply(this, args);
cache[cacheKey] = result;
return result;
};
return descriptor;
};
}
class MyClass {
@Memoize()
myMethod(param: string) {
// ...
}
}
const instance = new MyClass();
instance.myMethod("hello");
In this example, the Memoize
decorator adds caching functionality to the myMethod
method of the MyClass
class. When the method is called with a particular set of arguments, the caching logic checks to see if there’s already a cached result for those arguments. If so, it returns that result; if not, it executes the method and caches the result for future use.
Property Decorators
Property decorators are applied to individual property declarations and can be used to modify the behavior of those properties. For example, you might use a property decorator to enforce certain constraints on a particular property, such as a minimum or maximum value range.
One common use case for property decorators is to implement dependency injection. Dependency injection is a design pattern that involves injecting dependencies into class instances at runtime, instead of hardcoding them into the class constructor. By using a property decorator, you can define the types of dependencies that a class instance should have and automatically inject those dependencies when the class is created.
Here’s an example of a simple dependency injection property decorator:
function Inject() {
return (target: any, key: string) => {
let type;
const getter = () => {
if (!type) {
type = Reflect.getMetadata("design:type", target, key);
}
const dependency = new type();
Reflect.defineProperty(target, key, {
get: () => dependency,
enumerable: true,
configurable: true,
});
return dependency;
};
Reflect.defineProperty(target, key, {
get: getter,
enumerable: true,
configurable: true,
});
};
}
class MyDependency {
// ...
}
class MyClass {
@Inject()
myDependency!: MyDependency;
myMethod() {
this.myDependency.doSomething();
// ...
}
}
const instance = new MyClass();
instance.myMethod();
In this example, the Inject
decorator is used to inject an instance of the MyDependency
class into the myDependency
property of the MyClass
class. When the myMethod
method is called, it can access and use the myDependency
instance without having to create it manually.
Parameter Decorators
Parameter decorators are applied to individual function or constructor parameters and can be used to modify the behavior of those parameters. For example, you might use a parameter decorator to validate or sanitize a particular parameter value before it’s used in the method or constructor.
One common use case for parameter decorators is to implement type checking. By using a parameter decorator to decorate a typed parameter, you can ensure that the value passed in as an argument matches the expected type.
Here’s an example of a simple type-checking parameter decorator:
function Validate(target: any, key: string, index: number, type: Function) {
const originalMethod = target[key];
target[key] = function (...args: any[]) {
const arg = args[index];
if (!(arg instanceof type)) {
throw new Error(`Parameter at index ${index} must be of type ${type.name}`);
}
return originalMethod.apply(this, args);
};
}
class MyClass {
myMethod(@Validate param1: string) {
// ...
}
}
const instance = new MyClass();
instance.myMethod("");
In this example, the Validate
decorator is used to check the type of the param1
parameter of the myMethod
method. If the parameter is not an instance of the string
type, an error is thrown.
Conclusion
Typescript decorators are a powerful tool for software developers, offering a wide range of benefits for creating efficient, maintainable, and high-quality code. By using decorators to add metadata and behavior to different parts of your codebase, you can streamline your development process, reduce the risk of bugs and errors, and create code that is easier to read and maintain.
In this article, we have explored the different types of typescript decorators, including class, method, property, and parameter decorators. We have seen how decorators can be used to implement mixins, caching, dependency injection, type checking, and more.
As you continue to expand your knowledge of typescript and its capabilities, I encourage you to experiment with different types of decorators in your own code. By using these powerful tools effectively, you can elevate your software development work to new heights.
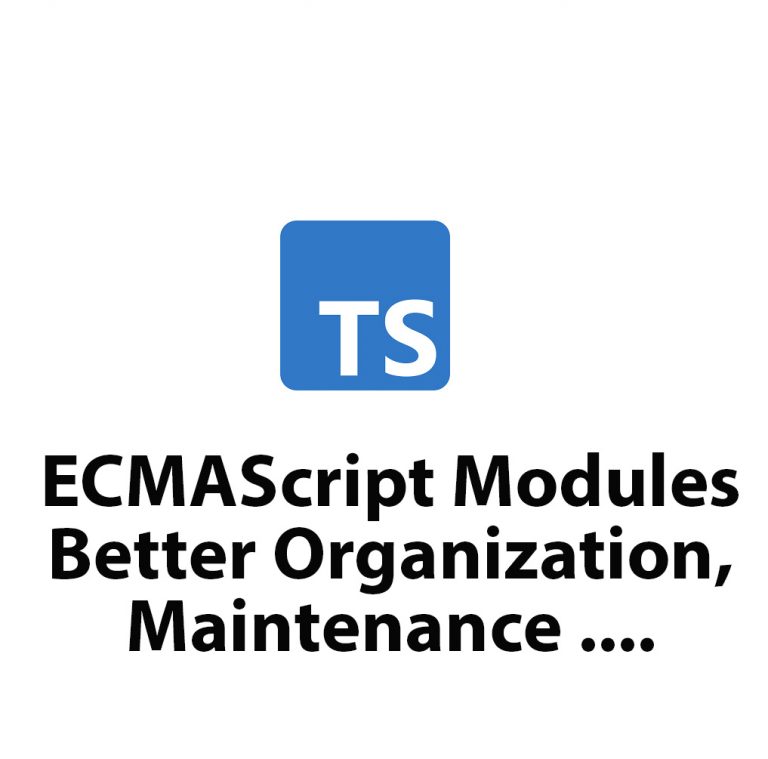
ECMAScript Modules in Node JS
As a software developer and avid Node JS user, I’ve always been on the lookout for ways to improve my workflow and simplify code maintenance. One of the most recent additions to Node JS that has greatly helped me achieve these goals is the implementation of ECMAScript (ES) Modules. ES Modules are a standard format […]
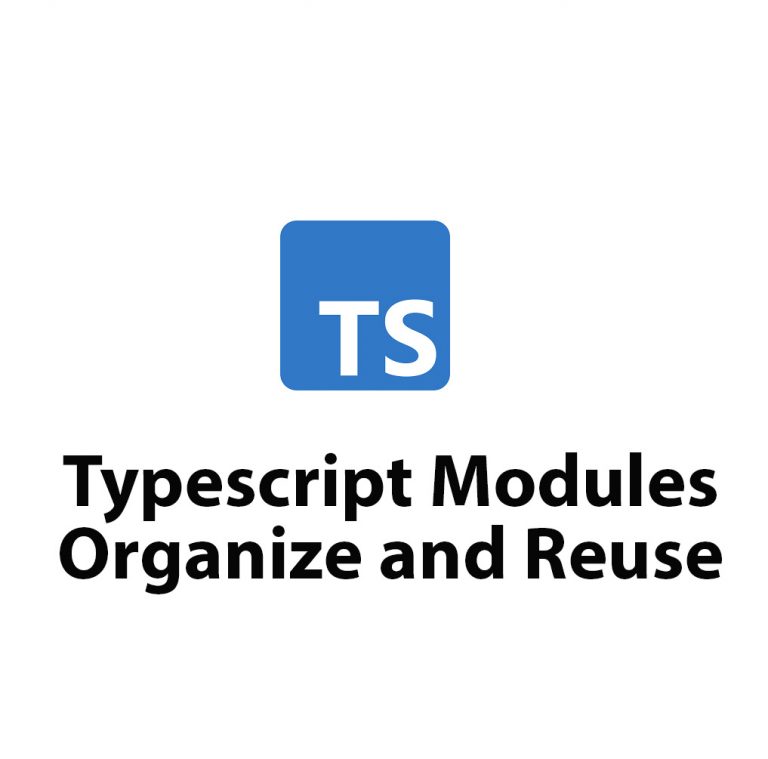
Learn Typescript Modules: Organization & Reusability
As a developer, I am always looking for ways to make my code more organized and efficient. One tool that has helped me achieve this goal is Typescript modules. In this article, I will be discussing Typescript modules in-depth, including how to create and import them, how to declare dependencies, and the benefits of using […]
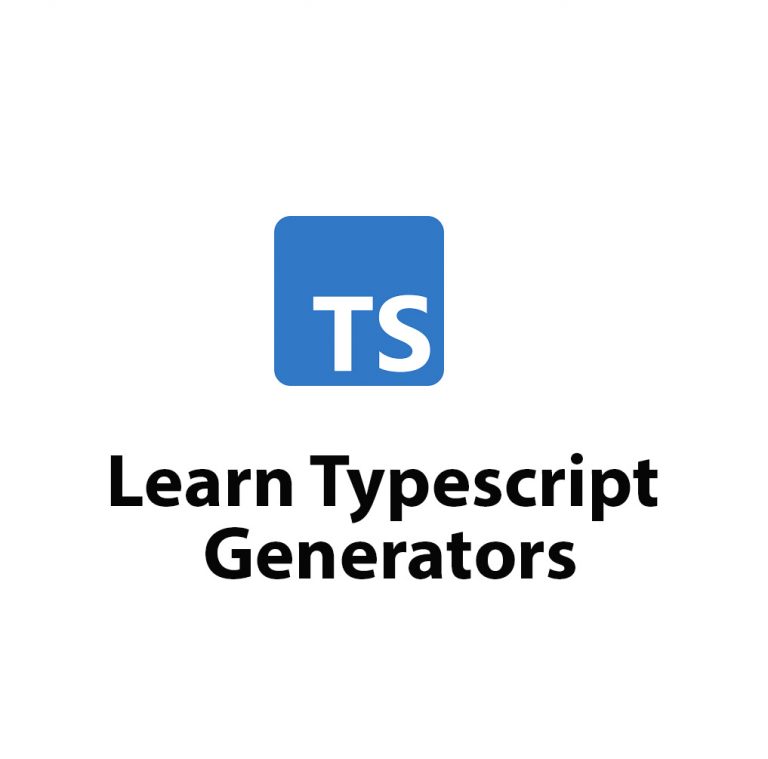
Learn Typescript Generators
Introduction TypeScript is a typed superset of JavaScript that brings static type checking to JavaScript development. TypeScript adds some much-needed structure and safety to JavaScript programming by providing tools for catching errors at compile time instead of run time. A generator is a feature that TypeScript borrows from Python. Generators are a special kind of […]
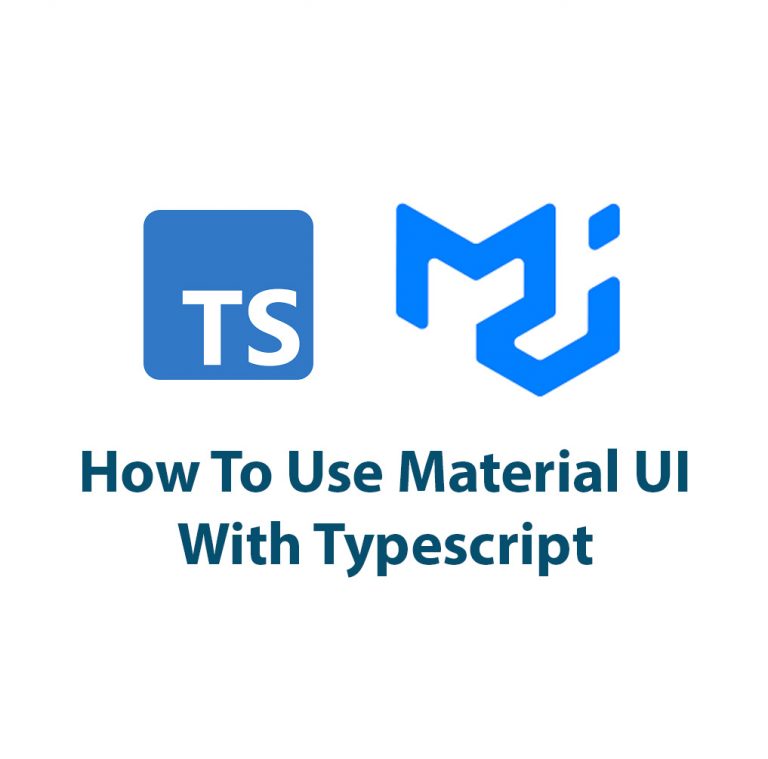
Use Material UI V5 With Typescript
Introduction Hello everyone! In this article, we are going to talk about Material UI V5 with Typescript. If you are a React developer, you must have heard of Material UI. It is one of the most popular React UI component libraries out there. On the other hand, Typescript is a superset of JavaScript that provides […]
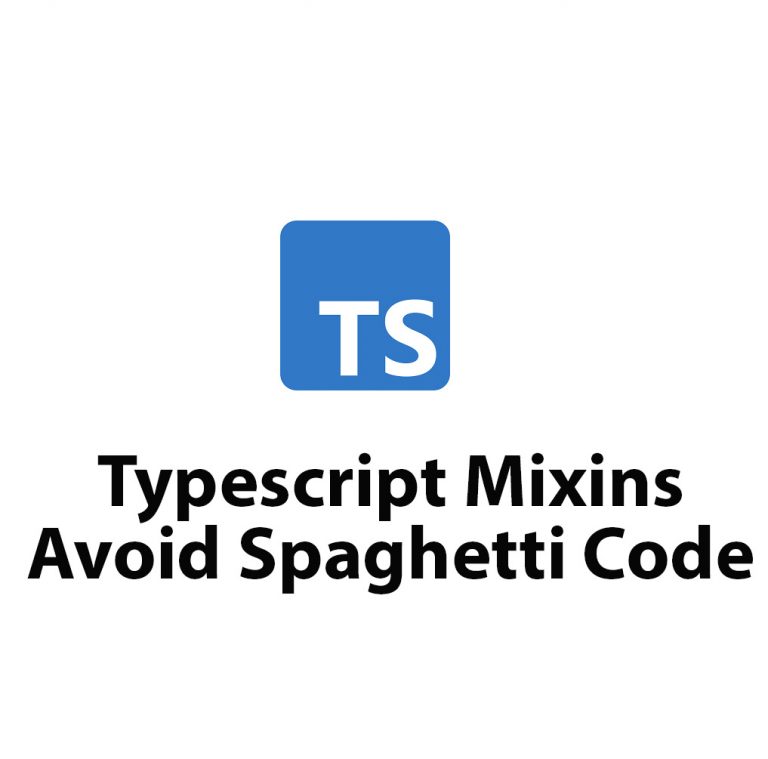
Learn Typescript Mixins: Avoid Spaghetti Code
As a software developer, I’ve been using Typescript for most of my latest projects. One of the most interesting features I’ve come across is Typescript Mixins. These allow for simple composability of classes and functions, which can help to reduce code complexity and improve maintainability. In this article, I’m going to provide a comprehensive guide […]
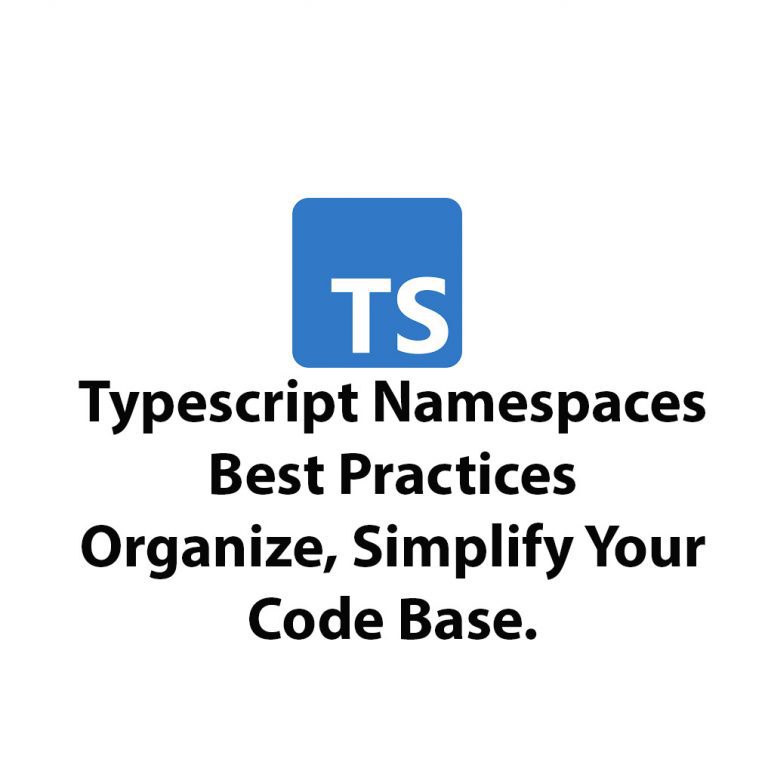
Typescript Namespaces: Best Practices
As a software developer, I have been intrigued by TypeScript’s ability to introduce new features to the JavaScript language while also minimizing the potential for runtime errors. One of the best things about TypeScript is that it provides excellent support for large codebases, and one of the features that can help with this is Namespaces. […]