As a software developer, I have been intrigued by TypeScript’s ability to introduce new features to the JavaScript language while also minimizing the potential for runtime errors. One of the best things about TypeScript is that it provides excellent support for large codebases, and one of the features that can help with this is Namespaces.
In this article, we will take a deep dive into TypeScript’s Namespaces, looking at how to create, nest, import, and export namespaces. We’ll also compare namespaces to ES6 modules, discuss best practices, and consider the possible limitations.
Introduction
Before we delve into Namespaces, it is essential to understand what TypeScript is and why it is valuable. TypeScript is an open-source programming language that is a superset of JavaScript. TypeScript provides optional static typing and is designed to make it easier to build large, complex applications.
TypeScript also provides a range of features beyond traditional JavaScript, including Enum, Generics, and Decorators. One major benefit of TypeScript is that it can provide better code assistance, such as syntax highlighting, code completion, and refactorings.
The goal of Namespaces in TypeScript is to organize related code in a logical and easy-to-understand way. Namespaces encapsulate a group of functions, variables, and classes into a single object, helping to reduce naming conflicts.
Creating a Namespace
To create a namespace in TypeScript, you need to use the ‘namespace’ keyword, followed by the name of the namespace, as shown below:
namespace MyNamespace {
export function myMethod() { }
export class myClass { }
export const myVariable = 42;
}
The namespace keyword is followed by the name of the namespace, which in this case is ‘MyNamespace.’ Within the curly braces {}, we define the elements that belong to the namespace. In this example, we have defined a function, a class, and a constant.
The ‘export’ keyword is used to make the elements within the namespace visible outside of the namespace. By default, elements within a namespace are not visible outside of the namespace.
To use the elements of the namespace, we simply need to include the namespace name, followed by the element name, as shown below:
MyNamespace.myMethod();
let myInstance = new MyNamespace.myClass();
let myValue = MyNamespace.myVariable;
Nesting Namespaces
Namespaces also support nesting, enabling us to create a hierarchy of namespaces. By nesting namespaces, we can organize our code into logical structures that make it easier to maintain and understand.
namespace FirstNamespace {
export namespace SecondNamespace {
export function secondMethod() { }
export class secondClass { }
export const secondVariable = 42;
}
}
In the example above, we have created a ‘FirstNamespace’ with a ‘SecondNamespace’ nested within it. Just like in the previous example, we have used the ‘export’ keyword to make the elements of the namespace visible outside the namespace.
To use the elements of the nested namespace, we can access them using dot notation, as shown in the example below:
FirstNamespace.SecondNamespace.secondMethod();
let myInstance = new FirstNamespace.SecondNamespace.secondClass();
let myValue = FirstNamespace.SecondNamespace.secondVariable;
Importing and Exporting Namespaces
Just like modules, we can also import and export namespaces in TypeScript. Suppose we have another file where we want to use our previously defined namespace, ‘MyNamespace.’ In that case, we need to import it into our file using the ‘import’ keyword, as shown below:
import { MyNamespace } from './MyNamespace';
In this example, we have imported the namespace ‘MyNamespace’ from the file ‘MyNamespace.ts.’ To import a namespace, we must use curly braces {} and the namespace name.
To export a namespace, we need to use the ‘export’ keyword before the ‘namespace’ keyword, as shown below:
export namespace MyNamespace {
export function myMethod() { }
export class myClass { }
export const myVariable = 42;
}
In this example, we have exported the namespace ‘MyNamespace’ so that it can be used in other files.
Limitations of Namespaces
While namespaces offer a valuable way of organizing code, there are some limitations to be aware of.
Scoping
One of the downsides of namespaces is that their scoping can become too broad, making it difficult for developers to manage dependencies. When using namespaces, the overall scope of the project can become blurred, making it challenging to determine where a piece of code should be implemented.
Naming Conflicts
Although namespaces aim to minimize naming conflicts, it is still possible to have clashes. When namespaces become too large or complex, it can be hard to ensure that all elements of the namespaces have unique names. This problem can lead to naming conflicts and errors in the code.
Accessibility
Namespaces also suffer from accessibility issues. Because namespaces are designed to work at a global level, there can be security concerns. Unauthorized parties may be able to access the namespace, leading to potential security breaches and privacy issues.
Comparison with Modules
Although namespaces and modules appear similar, they have some significant differences.
Namespaces provide a mechanism for organizing code logically, grouping related functions and classes together. They are best suited to large codebases with a well-defined structure.
In contrast, modules are designed for more modular systems that support small, easily testable components. Modules use the ‘export’ keyword to make functionality available outside of the module, only exposing what is necessary.
Modules are becoming increasingly popular due to their ability to reduce the size of code and improve security. However, for larger projects, namespaces are often more effective.
Best Practices
When working with Namespaces, there are several best practices to keep in mind:
Organization
It is essential to organize your namespaces into logical groupings. By organizing your namespaces in this way, you can ensure that your code remains comprehensible and maintainable.
Naming Conventions
Naming conventions are essential when developing with Namespaces. We recommend using a naming convention that includes a descriptive name that reflects the functionality defined within the Namespace.
Usage
NaVisually inspect your code to make sure it is well-organized and readable. Avoid creating overly complex namespaces, as this can make your code difficult to understand and maintain.
Conclusion
By using TypeScript Namespaces, you can better organize your code, minimize naming conflicts, and simplify your codebase. With Namespaces, you can group related functionality together, making it easier to maintain and understand.
Although there are some limitations to working with Namespaces, when used correctly, they can provide an effective and efficient solution for more significant codebases. By following our recommended best practices, you can ensure that your Namespaces are well-organized, maintainable, and secure.
If you are interested in learning more about Namespaces in TypeScript, we recommend exploring the official TypeScript documentation and trying out some examples of your own.
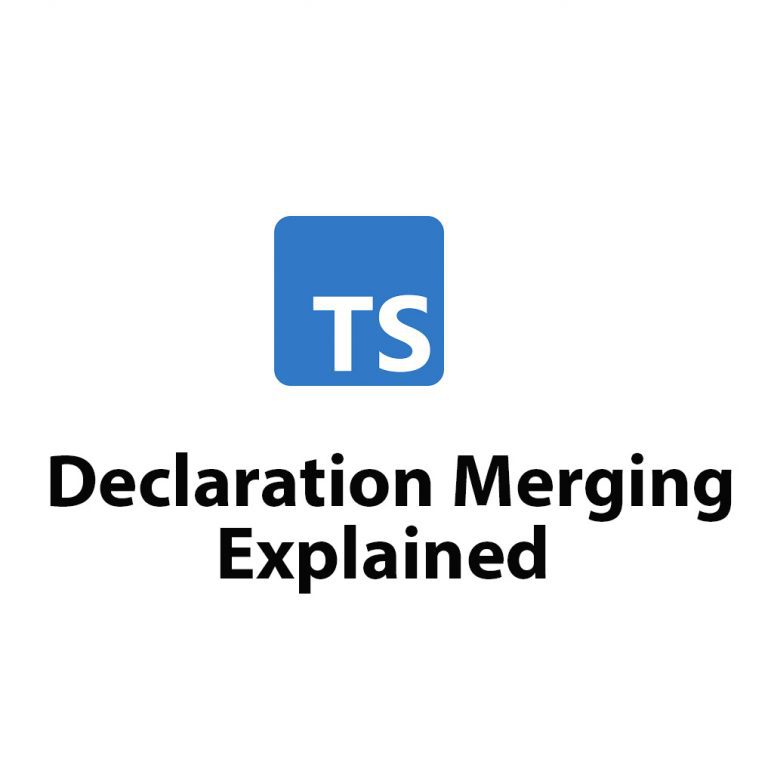
Typescript Declaration Merging
Introduction Typescript Declaration Merging is an essential feature of the Typescript language, which allows developers to extend existing code without modifying it. It can be a bit complex and overwhelming for beginners, but once you understand how it works, you’ll find it to be a powerful tool. As a developer, you will come across scenarios […]
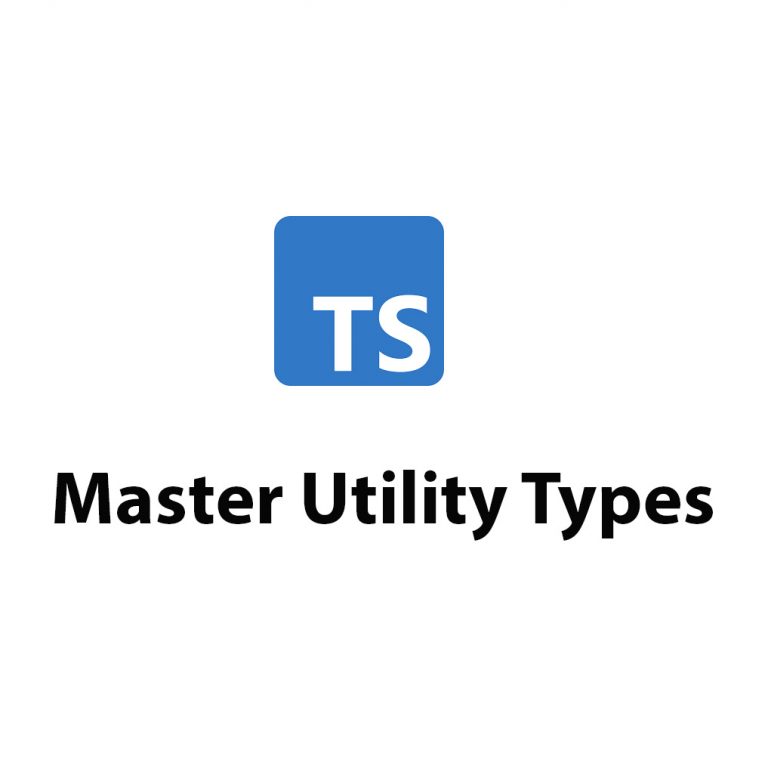
Typescript Utility Types
Introduction Hi there, I’m excited to talk to you today about Typescript Utility Types. First, let’s provide a brief overview of what Typescript is for those who may not be familiar with it. Typescript is an open-source programming language that builds on top of JavaScript by adding static types to code. This can help catch […]
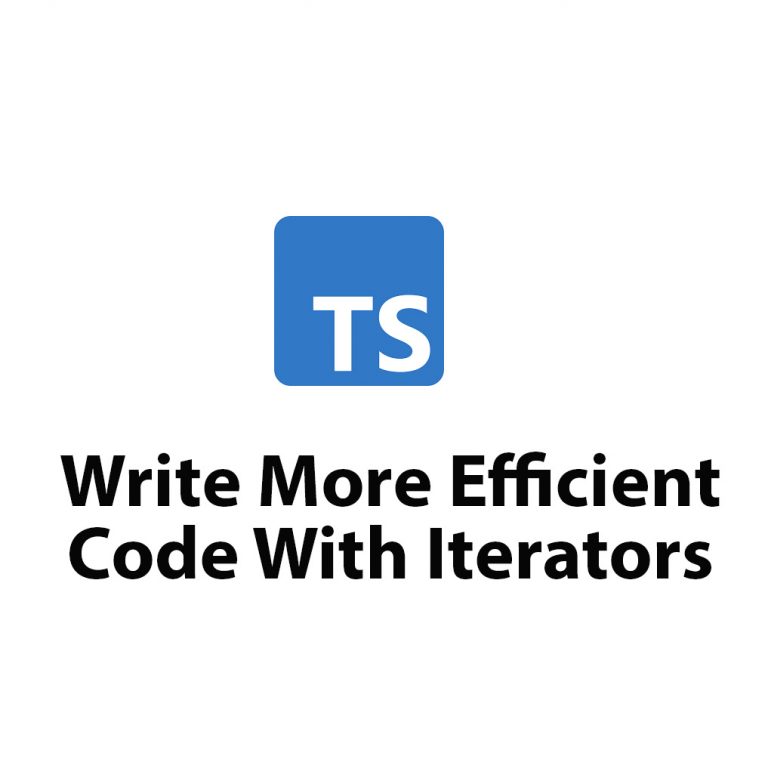
Learning Typescript Iterators
Introduction As a software developer who is always looking for efficient ways to write clean code, I discovered Typescript and the concept of iterators. Typescript is a JavaScript superset that enables the creation of more structured and maintainable code. In this article, I am introducing Typescript iterators and delving into their different types, how to […]
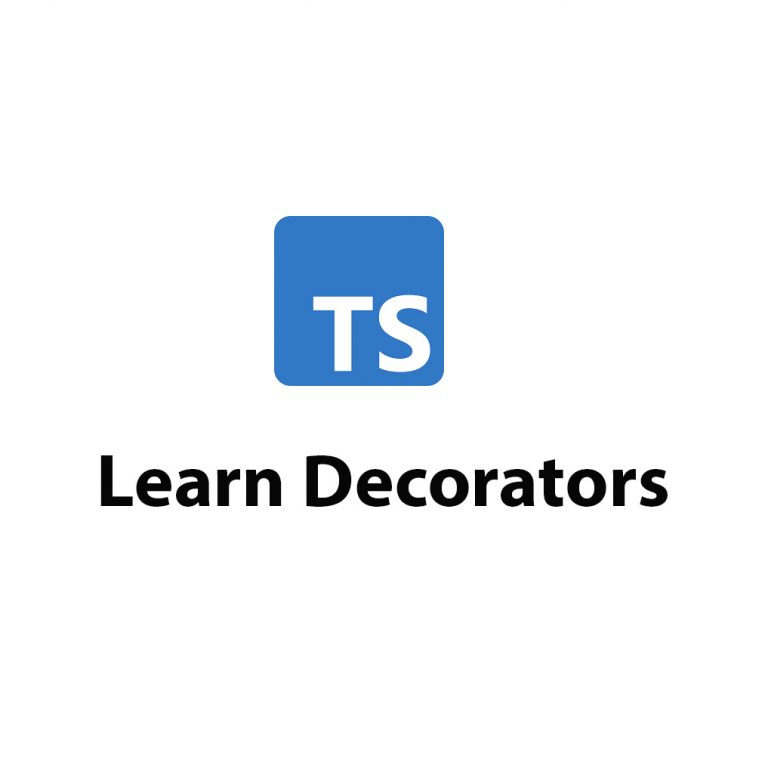
Mastering Typescript Decorators
As a software developer, I am constantly looking for ways to improve the efficiency and effectiveness of my work. One of the most powerful tools in my arsenal has been typescript decorators. In this article, I will dive deep into the world of typescript decorators, exploring their history, various types, and usage in software development. […]
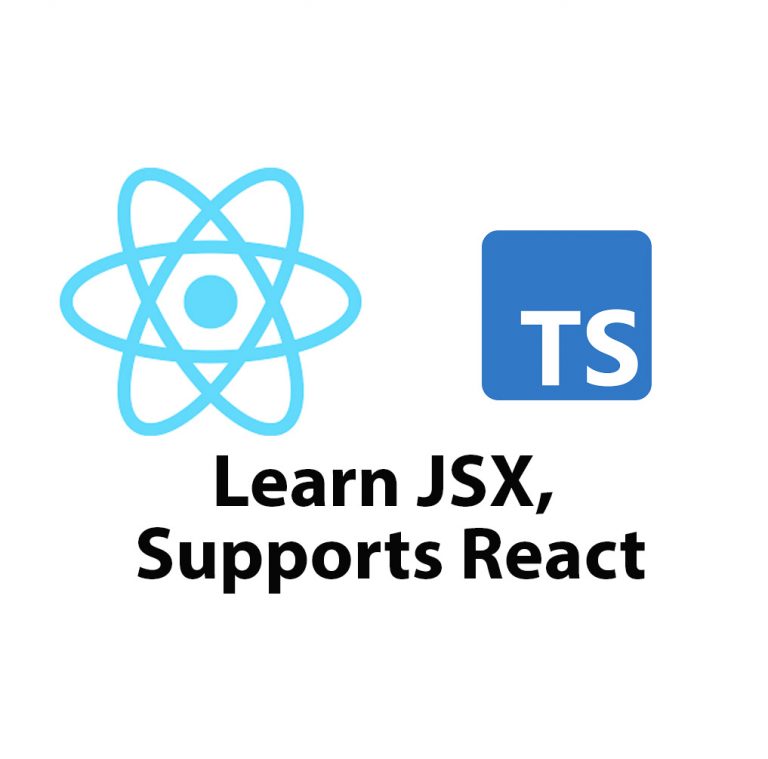
What Is JSX and how to use it.
Introduction As a web developer, I’m always on the lookout for new tools and technologies that can help me write better code faster. One tool that has caught my attention lately is Typescript JSX. In this article, I’m going to share my experience learning Typescript JSX and the benefits it has brought to my development […]
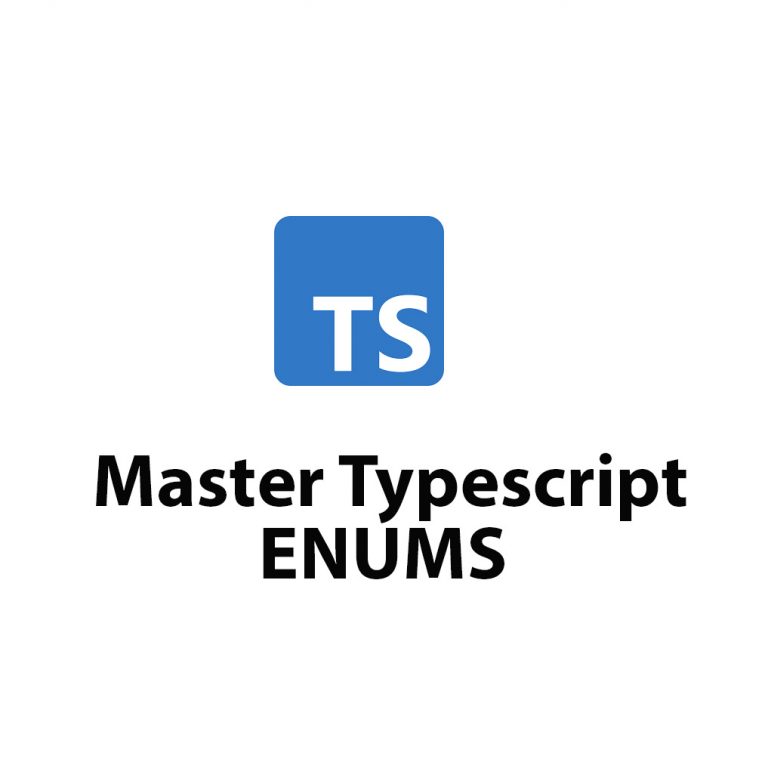
Mastering Typescript Enums
As a web developer, I’m constantly learning new ways to improve my coding practices. One of the more recent additions to my toolkit is TypeScript, a powerful superset of JavaScript that provides additional features to make coding easier and more efficient. One of my favorite features of TypeScript is enums, which allow me to better […]