As someone who has spent a lot of time working with Node JS, I have come to understand the importance of Asynchronous Context Tracking in the development of high-performance applications. In this article, I will explore the concept of Asynchronous Context Tracking with Node JS, its advantages, techniques, challenges and best practices.
Before we dive into Asynchronous Context Tracking, let’s first clarify what Node JS is. Node JS is a server-side JavaScript runtime environment that enables developers to build scalable, high-performance web applications. Unlike traditional web development technologies, Node JS is designed to be highly efficient at handling I/O operations and is inherently asynchronous. This means that developers can write code that executes asynchronously, allowing multiple concurrent operations to occur without blocking the main thread.
This brings us to the concept of Asynchronous Context Tracking. In simple terms, Asynchronous Context Tracking refers to the ability to track the execution context of an asynchronous operation, while it is being executed. In Node JS, this is particularly important because of its inherently asynchronous nature. The execution context in this case refers to everything that is required to execute a piece of code, including variables, functions, and the calling stack.
Without Asynchronous Context Tracking, it can be difficult to keep track of the state of concurrent operations, which can lead to bugs, errors, and poor performance. For example, let’s say we are running a Node JS application with multiple concurrent database queries. Without proper Asynchronous Context Tracking, we would not be able to determine which query is executing at a given time, leading to confusion and potential errors.
To understand how Asynchronous Context Tracking works in Node JS, let’s explore some techniques that can be used.
- Callbacks
One of the most common techniques for handling asynchronous operations in Node JS is through callbacks. A callback function is a function that is passed as a parameter to another function and is executed once the operation is complete. Here is an example of a callback function being used to handle an asynchronous database query:
function getDataFromDatabase(query, callback) {
const database = connectToDatabase();
database.query(query, (error, result) => {
if (error) {
callback(error, null);
} else {
callback(null, result);
}
});
}
In this example, the query function takes in a query parameter and a callback function as arguments. Once the database query is complete, the callback function is executed with an error and the query result. Using callbacks can help prevent blocking the main thread of the Node JS application, but can lead to “callback hell” and code that is difficult to read and maintain.
- Promises
Promises provide another way to handle asynchronous operations in Node JS. A promise is an object that represents the eventual completion or failure of an asynchronous operation. Promises allow developers to write code that is easier to read and maintain than code that uses callbacks. Here is an example of a Promise being used to handle an asynchronous database query:
function getDataFromDatabase(query) {
const database = connectToDatabase();
return new Promise((resolve, reject) => {
database.query(query, (error, result) => {
if (error) {
reject(error);
} else {
resolve(result);
}
});
});
}
In this example, the query function returns a Promise object that resolves with the query result or rejects with an error. Using Promises can lead to cleaner code that is easier to read and maintain.
- Async/Await
Async/Await is a relatively new feature of Node JS that allows developers to write asynchronous code in a way that looks and feels like synchronous code. Async/Await uses Promises under the hood, but simplifies the syntax and structure of the code. Here is an example of Async/Await being used to handle an asynchronous database query:
async function getDataFromDatabase(query) {
const database = connectToDatabase();
try {
const result = await database.query(query);
return result;
} catch (error) {
throw error;
}
}
In this example, the getDataFromDatabase function is declared as async, which allows us to use the await keyword to wait for the database query to complete. Using Async/Await can make asynchronous code more readable and maintainable.
Now that we understand how Asynchronous Context Tracking is achieved in Node JS, let’s explore some of its advantages.
- Reduced Code Complexity
Asynchronous Context Tracking reduces code complexity by allowing developers to write code that is easier to read and understand. Callbacks, Promises, and Async/Await all simplify the syntax of asynchronous code, making it easier to work with. - Improved Performance and Scalability
Asynchronous Context Tracking can greatly improve the performance and scalability of Node JS applications. By allowing multiple operations to execute concurrently without blocking the main thread, applications can handle more requests and perform more efficiently. - Enhanced Error Handling and Debugging
Asynchronous Context Tracking allows for better error handling and debugging in Node JS applications. By properly tracking the execution context of asynchronous operations, developers can pinpoint errors and debug more effectively.
While Asynchronous Context Tracking has many advantages, it also comes with its own set of challenges.
- Callback Hell
Callback Hell is a common problem in Node JS applications that use callbacks to handle asynchronous operations. As callbacks are nested within other callbacks, the code can become unreadable and difficult to debug. - Race Conditions
Race Conditions occur when two or more asynchronous operations are executed concurrently, and the results depend on the order in which those operations complete. Race Conditions can cause unpredictable behavior and can be difficult to track down. - Memory Leaks
Memory Leaks can occur when asynchronous operations are not properly cleaned up after they are completed. This can lead to a buildup of unused memory, which can result in performance issues and crashes.
To overcome these challenges, there are several best practices that developers can follow when implementing Asynchronous Context Tracking in Node JS applications.
- Avoid Nesting Callbacks
Developers can avoid Callback Hell by avoiding nesting callbacks. Instead of nesting callbacks, developers can use Promises or Async/Await, which provide cleaner and more readable code. - Use Promises or Async/Await
Promises and Async/Await are superior to callbacks when it comes to Asynchronous Context Tracking. They provide cleaner and more readable code, and help avoid Race Conditions and Memory Leaks. - Handle Errors Effectively
Properly handling errors is critical when it comes to Asynchronous Context Tracking. Developers should make sure to handle errors in a consistent and effective way, by using try/catch blocks, error objects and other best practices.
In conclusion, Asynchronous Context Tracking is
an essential technique for developing high-performance and scalable Node JS applications. By properly tracking the execution context of asynchronous operations, developers can greatly improve the performance, scalability, and error handling of Node JS applications. Despite the challenges that come with Asynchronous Context Tracking, such as Callback Hell, Race Conditions, or Memory Leaks, developers can follow best practices, such as avoiding nesting callbacks, using Promises or Async/Await, and handling errors effectively.
To illustrate the benefits of Asynchronous Context Tracking and how it works in practice, let’s take a look at some sample code.
Consider the following example, where we want to perform some database queries and aggregate the results.
const connectToDatabase = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve({
query: (query, callback) => {
setTimeout(() => {
callback(null, {
rows: [1, 2, 3]
});
}, 1000);
}
});
}, 1000);
});
}
const getResults = async () => {
const database = await connectToDatabase();
const [result1, result2, result3] = await Promise.all([
database.query('SELECT * FROM table1'),
database.query('SELECT * FROM table2'),
database.query('SELECT * FROM table3'),
]);
return [result1.rows, result2.rows, result3.rows]
}
getResults().then(console.log);
In this example, we are asynchronously connecting to a database and retrieving data from three tables. We are using the async/await
approach, which allows us to write asynchronous code that looks and behaves like synchronous code. By using this approach, our code is much easier to read and maintain than it would be if we had used callbacks.
Using Asynchronous Context Tracking in this way, we can easily handle multiple concurrent operations, and simplify our code, which makes it easier to maintain and debug.
In conclusion, Asynchronous Context Tracking is a crucial technique for developing high-performance and scalable Node JS applications. By following best practices and using techniques like Promises and Async/Await, developers can simplify their code and avoid common pitfalls like Callback Hell, Race Conditions, and Memory Leaks. With the help of Asynchronous Context Tracking, developers can build powerful and efficient Node JS applications that can handle multiple operations at once and are easy to maintain and debug.
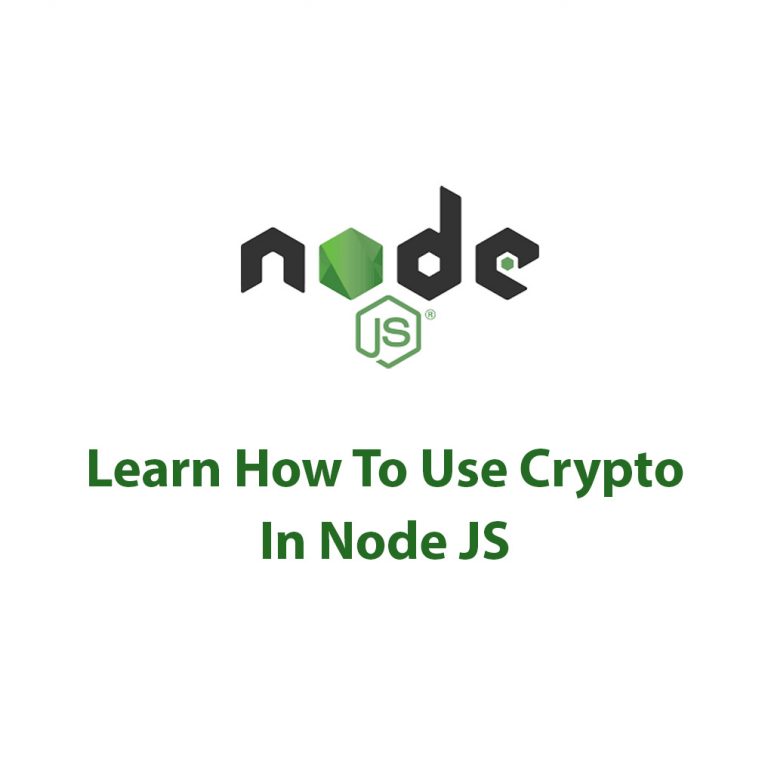
Using Crypto In Node JS
Have you ever wondered how some of the most secure websites and applications keep your data safe from malicious attacks? Well, one of the answers lies in the use of cryptography! Cryptography is the art of writing or solving codes and ciphers, and it has been around for centuries. In the world of computer science, […]
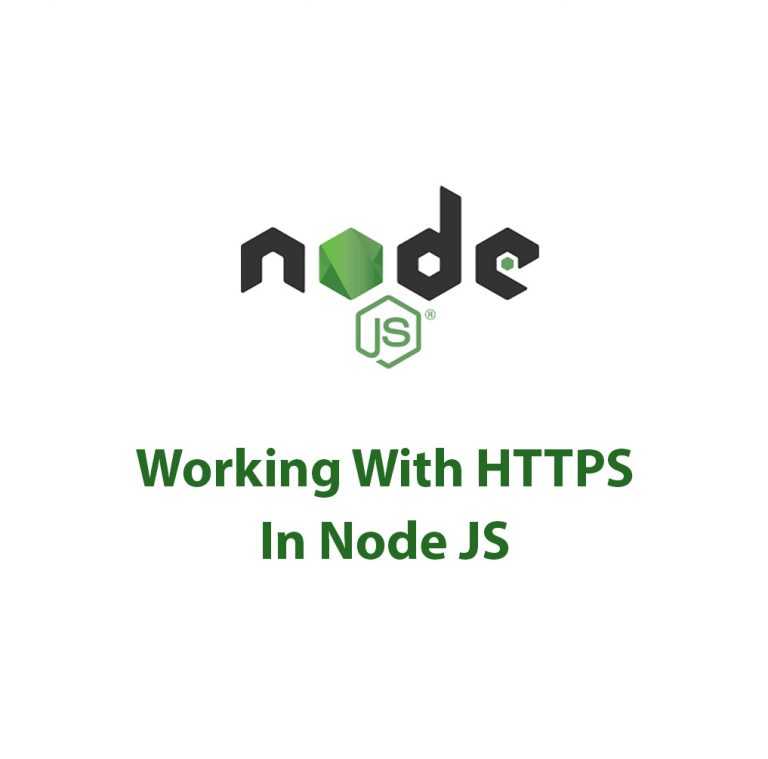
Working With HTTPS In Node JS
As a developer, I’m always on the lookout for security protocols that can help me protect users’ sensitive information. HTTP, while functional, lacks the encryption necessary to truly secure data transmission over the web. This is where HTTPS comes in. But working with HTTPS can be a bit daunting, especially if you’re new to it. […]
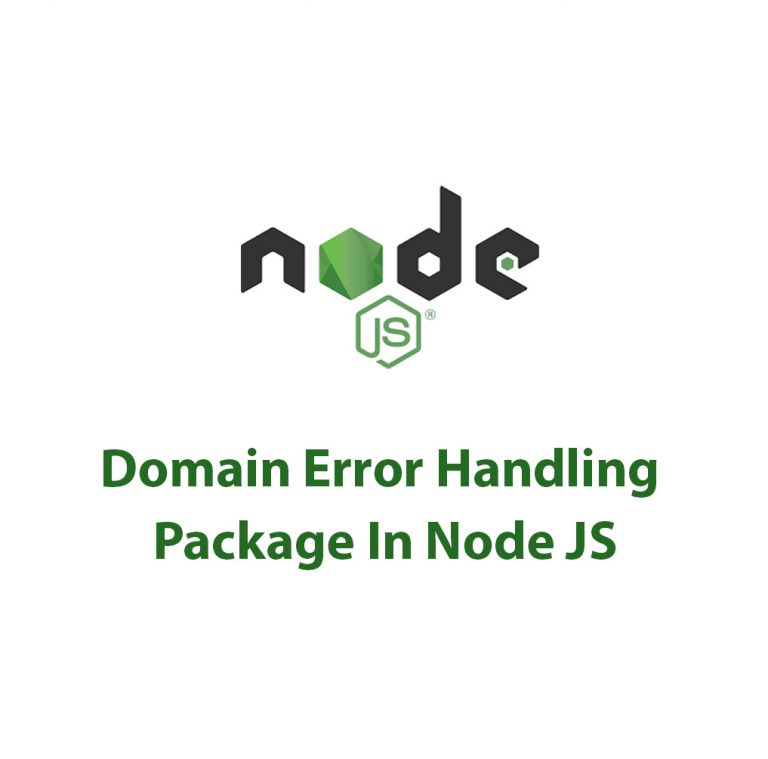
Domain Error Handling Package In Node JS
Domain Package In Node JS Have you ever been annoyed by dealing with mistakes in a Node.js application? It might be difficult for Node.js developers to handle problems when they happen. However, handling errors becomes much simpler with the Node.js Domain package. In this article, I’ll give a general overview of the Node.js Domain package, […]
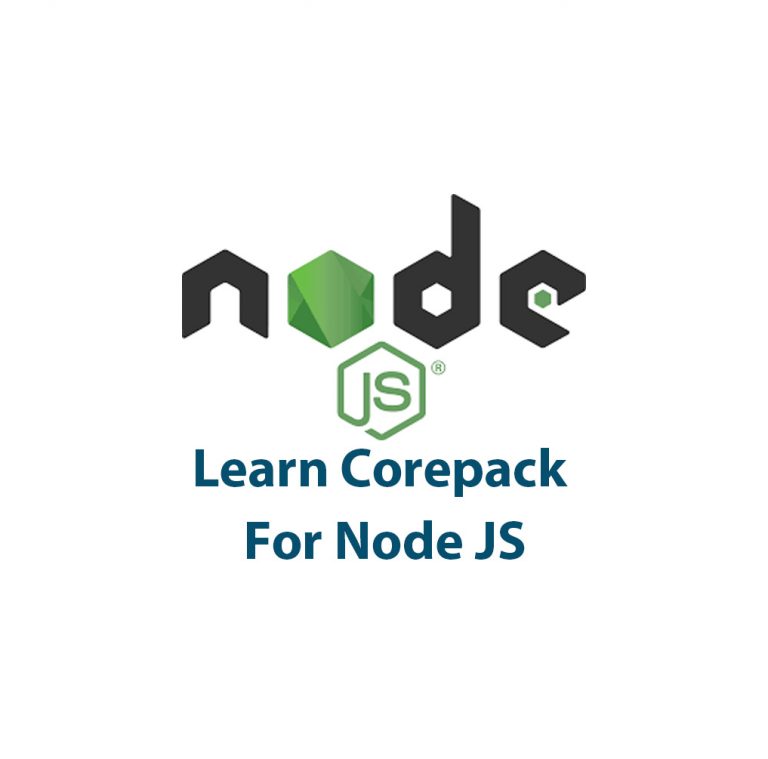
Corepack In Node JS
Have you ever worked on a Node JS project and struggled with managing dependencies? You’re not alone! Managing packages in Node JS can get confusing and messy quickly. That’s where Corepack comes in. In this article, we’ll be diving into Corepack in Node JS and how it can make dependency management a breeze. First of […]
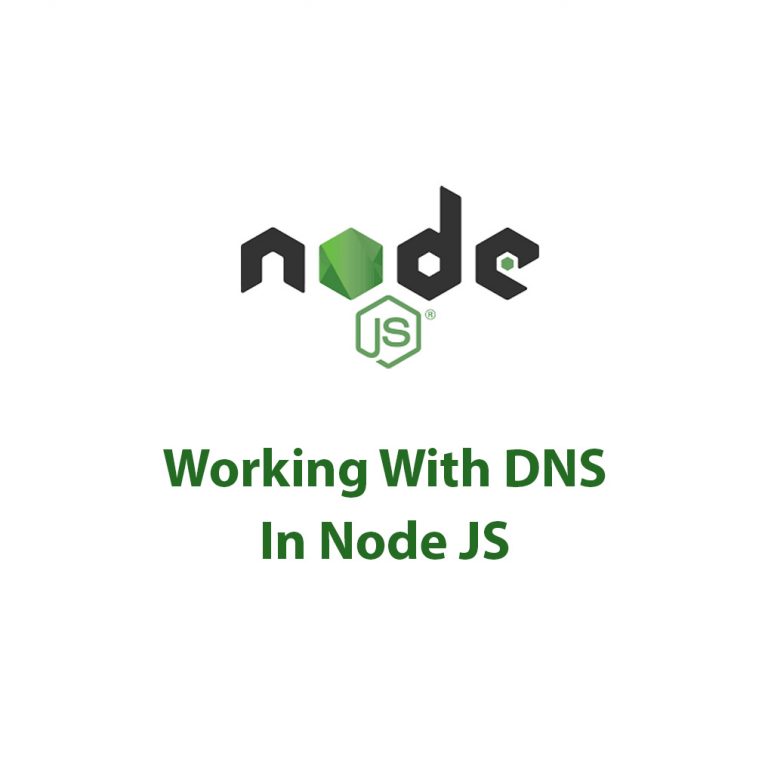
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]
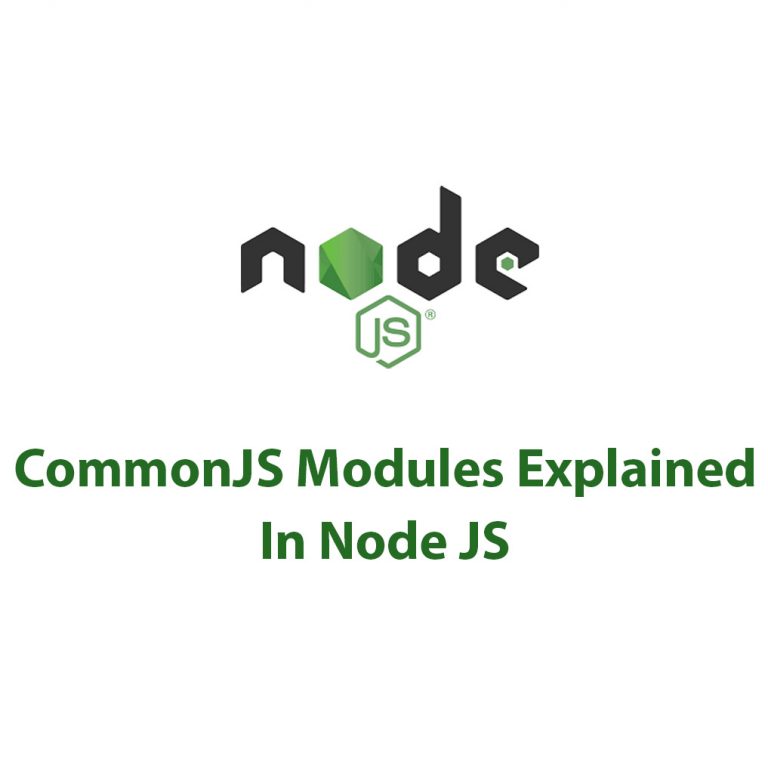
CommonJS Modules In Node JS
Introduction As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has […]