Introduction:
If you’re a Node.js developer, you’ve probably heard the term “Async Hooks” thrown around in conversation. But do you know what they are or how they work? In this article, I’ll be diving into the world of Async Hooks, explaining what they are and how to use them effectively.
What are Async Hooks?
Async Hooks is a feature in Node.js that allows you to track and monitor asynchronous events when running applications. Essentially, it’s a way to tap into the low-level internals of Node.js and listen in on everything that’s going on.
Async Hooks can be a powerful tool for debugging, profiling, and tracing asynchronous code, but they can also be used for other purposes like monitoring resource usage and controlling application flow. The possibilities are virtually limitless.
Setting up Async Hooks:
To use Async Hooks, you need to have a Node.js version that supports this feature. You can check your version by running the command “node -v” in your terminal.
If you have the right version, you can enable Async Hooks by adding a flag when you start your application. The flag required is “–async_hooks”, and it needs to be added after “node” and before the name of the file you want to run.
For example, if I have a file called “my-app.js” and I want to enable Async Hooks, I would run: “node –async_hooks my-app.js”.
Understanding Async Resources:
Before we dive into creating and using Async Hooks, we need to understand what Async Resources are. Async Resources are objects that represent a particular type of asynchronous operation in Node.js.
There are five types of Async Resources in Node.js: timers, sockets, handles, requests, and custom. Each type of resource has unique properties and methods associated with it.
To access the Async Resource object, we can use the AsyncResource class in the “async_hooks” module. Here’s an example of how to create a new Async Resource for a timer:
const async_hooks = require('async_hooks');
const { Timeout } = require('timers');
const asyncContext = async_hooks.createHook({
init(asyncId, type, triggerAsyncId, resource) {
// Make the async context available in this function.
async_hooks.executionAsyncId(asyncId);
const context = async_hooks.executionAsyncId();
resource._asyncContext = context;
},
destroy(asyncId) {
// Destroy the async context.
// No code here for this example because no data is being used.
}
}).enable();
const timeout = new Timeout(() => {
console.log('Hello world!');
}, 1000);
const id = async_hooks.triggerAsyncId();
async_hooks.addHooks({
before() {
async_hooks.executionAsyncId(id);
}
});
timeout.refresh();
console.log(timeout._asyncContext);
setTimeout(() => {
console.log('Timeout async context:', timeout._asyncContext);
}, 500);
In this example, we’re using the createHook method to create a new hook that will be triggered when a new Async Resource is created. We’re then setting the executionAsyncId and storing it in the Async Resource object.
Using Async Hooks:
Now that we have a better understanding of Async Resources, we can start creating and using Async Hooks. Here’s an example of a simple Async Hook that will run every time a new Async Resource is created:
const async_hooks = require('async_hooks');
async_hooks.createHook({
init(asyncId, type, triggerAsyncId, resource) {
console.log(`Async Resource ${type} created with ID ${asyncId}`);
}
}).enable();
setTimeout(() => {
console.log('Hello world!');
}, 2000);
console.log('Async Hook was created');
In this example, we’re using the createHook method to create a new hook that will be triggered when a new Async Resource is created. Inside the hook, we’re logging the type of Async Resource and its ID.
When we run this code, we’ll see the message “Async Resource Timeout created with ID [number]” followed by “Async Hook was created”. Then, after two seconds, we’ll see “Hello world!” printed to the console.
Async Hook Examples:
Here are a few additional examples of how you can use Async Hooks in real-world scenarios:
Debugging with Async Hooks:
const async_hooks = require('async_hooks');
async_hooks.createHook({
init(asyncId, type, triggerAsyncId, resource) {
if (type === 'Timeout' || type === 'Immediate') {
console.log(`Debugging: ${type} created with ID ${asyncId}`);
}
}
}).enable();
setTimeout(() => {
console.log(addNumbers(1, 2));
}, 2000);
function addNumbers(a, b) {
return a + b;
}
In this example, we’re using an Async Hook to debug a simple function that adds two numbers. We’ve set up the hook to only trigger when a Timeout or Immediate Async Resource is created.
When we run this code, we’ll see the message “Debugging: Timeout created with ID [number]” printed to the console, followed by the result of the addNumbers function.
Profiling with Async Hooks:
const async_hooks = require('async_hooks');
const { performance } = require('perf_hooks');
let requests = 0;
let startTime = 0;
async_hooks.createHook({
init(asyncId, type, triggerAsyncId, resource) {
if (type === 'HTTPINCOMINGMESSAGE') {
requests++;
startTime = performance.now();
}
},
destroy(asyncId) {
if (asyncId === requests) {
console.log(`Total requests: ${requests}`);
console.log(`Total runtime: ${performance.now() - startTime}ms`);
}
}
}).enable();
const http = require('http');
const server = http.createServer((req, res) => {
res.end('Hello world!');
});
server.listen(3000);
In this example, we’re using an Async Hook to profile the performance of an HTTP server in Node.js. We’ve set up the hook to trigger when an HTTPINCOMINGMESSAGE Async Resource is created and to log the total number of requests and runtime when that Async Resource is destroyed.
When we run this code and make requests to the server, we’ll see the total number of requests and runtime printed to the console.
Tracing with Async Hooks:
const async_hooks = require('async_hooks');
async_hooks.createHook({
init(asyncId, type, triggerAsyncId, resource) {
if (type === 'Timeout') {
console.log(`Tracing: Timeout created with ID ${asyncId}`);
console.trace();
}
}
}).enable();
setTimeout(() => {
console.log(addNumbers(1, 2));
}, 2000);
function addNumbers(a, b) {
return a + b;
}
In this example, we’re using an Async Hook to trace the call stack of a function that adds two numbers. We’ve set up the hook to trigger when a Timeout Async Resource is created and to log the call stack using console.trace().
When we run this code, we’ll see the message “Tracing: Timeout created with ID [number]” printed to the console, followed by the call stack of the addNumbers function.
Best Practices and Limitations:
As with any programming feature, there are some best practices and limitations to keep in mind when using Async Hooks.
One important thing to keep in mind is that Async Hooks can be performance-intensive, especially if you’re logging or tracking a large number of Async Resources. It’s crucial to use Async Hooks only when necessary and to be careful not to slow down your application.
Another thing to keep in mind is that Async Hooks are relatively new to Node.js, and some features may not be fully supported or may change in future versions. It’s essential to stay up-to-date with Node.js releases and to test your code thoroughly.
Conclusion:
Async Hooks are a powerful tool for Node.js developers, allowing you to track and monitor asynchronous events when running applications. With topics ranging from debugging to profiling and tracing, the possibilities of Async Hooks are virtually limitless.
Asynchronous programming has become a fundamental skill for any modern developer, and Async Hooks provide you with a set of tools to master it. Understanding Async Hooks is a vital step to unlocking the potential of Node.js and will make you a better developer overall.
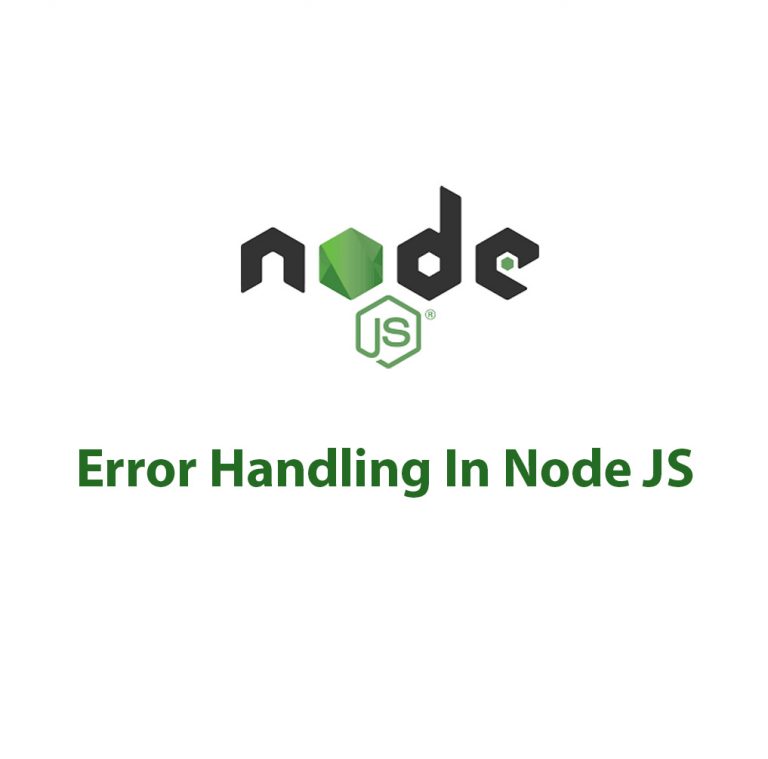
Error Handling in Node JS
A Node JS developer may struggle with error handling on occasion. Although faults are occasionally unavoidable, you can ensure that your application continues to function flawlessly by implementing error handling correctly. Let’s start by discussing the many kinds of problems you could run into when working with Node JS. The Three Types of Errors in […]
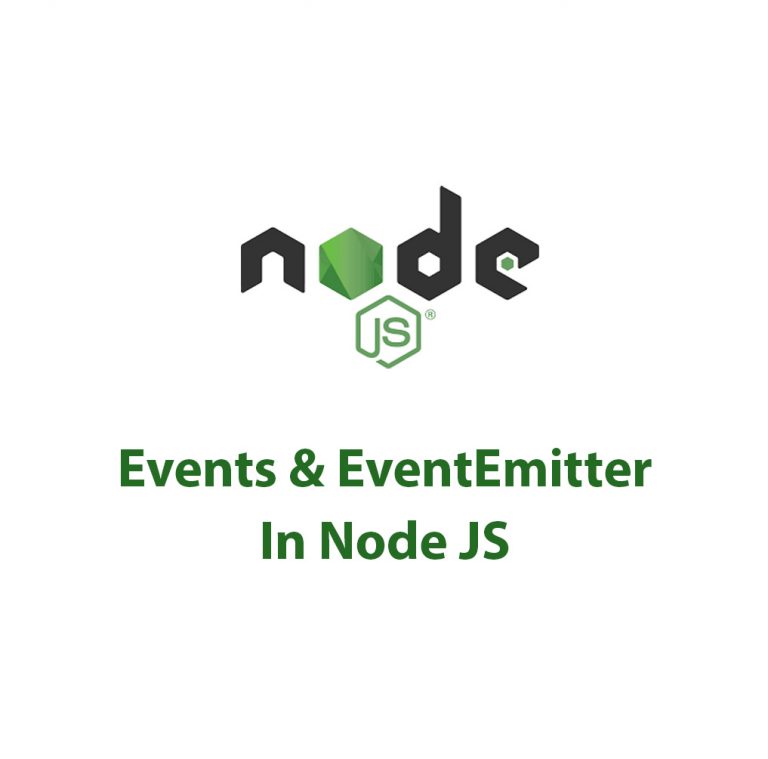
Events In Node JS
Introduction As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought. […]
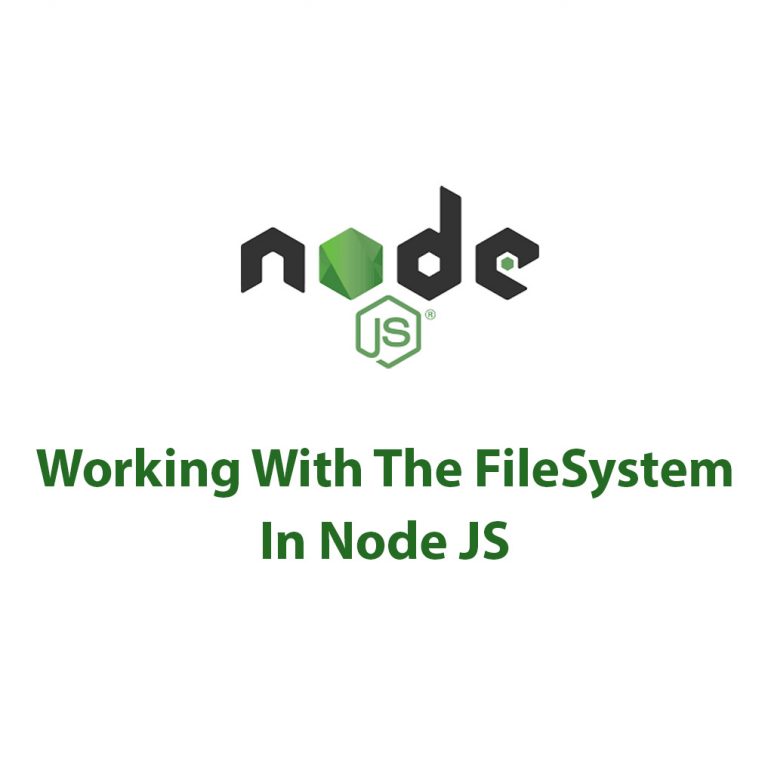
Working With The FileSystem In Node JS
Working With The FileSystem In Node.js Node.js is a powerful platform for building web applications and backend services. One of the most common things that developers need to do is work with files and the file system. This involves creating, modifying, and deleting files, as well as reading from and writing to them. In this […]
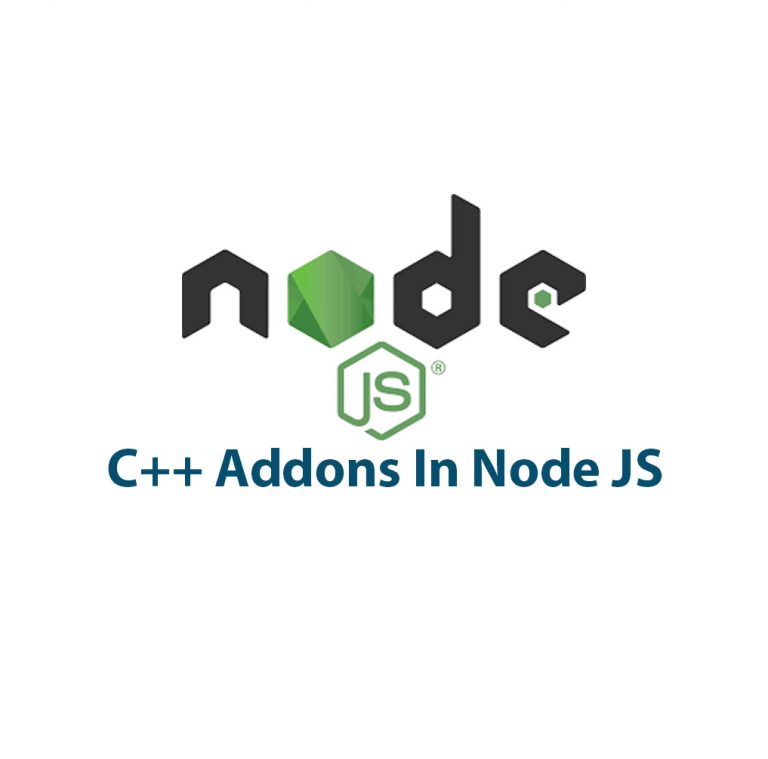
C++ Addons In Node JS
Introduction: As a software developer, I am always looking for ways to improve the performance of my applications. One way to achieve this is by using C++ Addons in Node JS. In this article, we will explore what C++ Addons are, why they are useful in Node JS, and how to create and use them. […]
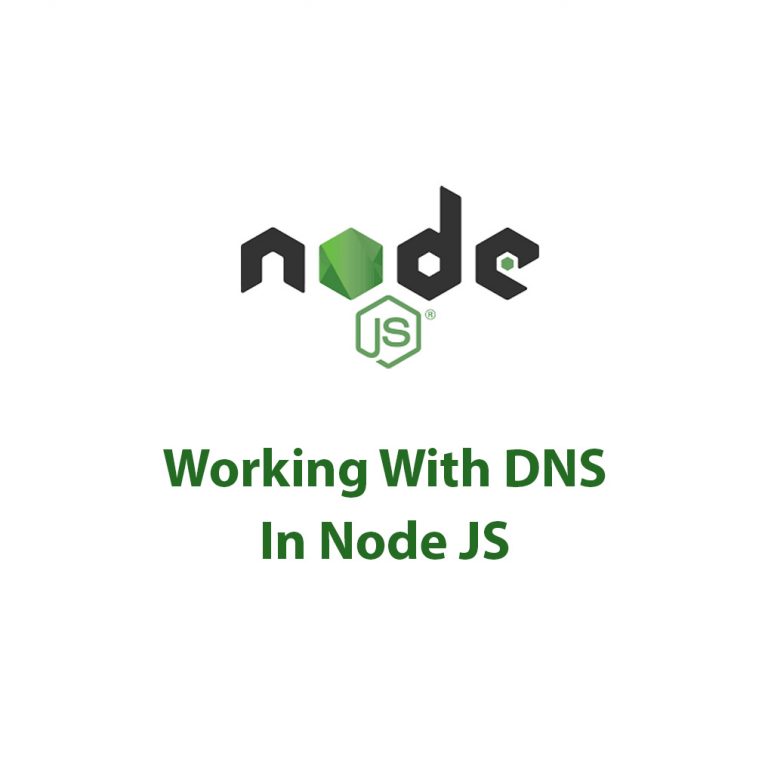
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]
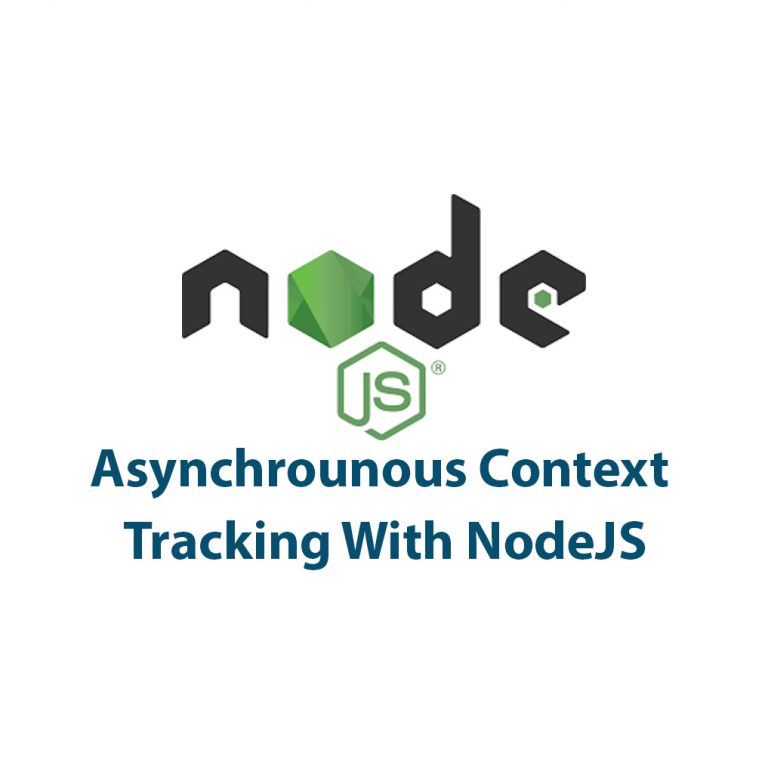
Asynchronous Context Tracking With Node JS
As someone who has spent a lot of time working with Node JS, I have come to understand the importance of Asynchronous Context Tracking in the development of high-performance applications. In this article, I will explore the concept of Asynchronous Context Tracking with Node JS, its advantages, techniques, challenges and best practices. Before we dive […]