As a developer, I’ve always been interested in exploring different ways to improve the performance of my Node JS applications. One tool that has proven to be immensely beneficial is cluster module. In this article, we’ll dive deep into clustering for Node JS, how it works, how to implement it, and the benefits it provides.
Clustering in Node JS is a powerful concept that allows multiple Node processes to work together as a single system. By creating a cluster of nodes that can work together, you can easily increase the processing power of your applications, allowing them to handle more requests per second and perform more efficiently in general.
How Clustering Works in Node JS
Before diving into implementation, it’s important to understand how clustering works. At a high level, clustering in Node JS creates a group of child processes which each run a copy of a server. These processes work together to handle incoming requests and send back responses to the client.
Communication between the parent process (or “master”) and the child processes is accomplished using inter-process communication (IPC). IPC is a method of exchanging data between processes that offers higher performance than other methods like TCP.
The master process handles receiving requests from clients and creating worker processes to handle them. This is done through the “cluster” module built into Node JS. Once a process is created, it is assigned a unique ID, which the master uses to keep track of and communicate with that process.
Load balancing is another important aspect of clustering. Load balancing distributes incoming requests across the available worker processes. This ensures that no one worker process is overloaded with requests, leading to a bottleneck in processing.
Implementing Clustering in Node JS
Now that we understand how clustering works, let’s dive into how to implement it in Node JS. The first step is to include the cluster module in your application:
const cluster = require('cluster');
Next, you’ll need to set up the server to be created in each worker process. This is done by wrapping the server creation code in a function that can be called by each worker:
if (cluster.isMaster) {
// Code to create worker processes and manage the cluster
} else {
// Code to create the server in this worker process
}
Inside the “isMaster” block, you’ll want to create as many worker processes as you need for your application. This can be done using the “fork” method provided by the cluster module:
if (cluster.isMaster) {
// Creates four worker processes
for (let i = 0; i < 4; i++) {
cluster.fork();
}
} else {
// Code to create the server in this worker process
}
Once the workers are created, you’ll need to set up the load balancing. This is done by listening for incoming requests and sending them to the appropriate worker process:
if (cluster.isMaster) {
// Creates four worker processes
for (let i = 0; i < 4; i++) {
cluster.fork();
}
} else {
const http = require('http');
// Code to create the server in this worker process
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, world!');
}).listen(8000);
}
The code above shows how to set up a basic server in each worker process. Note that the servers are created using the “http” module built into Node JS. This should be familiar to most Node developers.
Best Practices and Common Mistakes
While clustering can provide great benefits to your application, it’s important to follow best practices to ensure it’s done correctly. Here are a few tips to keep in mind:
- Do not create too many worker processes. Each worker process requires a certain amount of memory, so creating too many can lead to a situation where your application runs out of memory. In general, it’s best to create a number of workers equal to the number of CPU cores on your machine.
- Properly handle errors in child processes. If one of your worker processes fails, it’s important to handle the error so that the process can be restarted. Otherwise, your application could be left with a broken worker process that is no longer responding to requests.
- Set up monitoring and health checks. When using clustering, it’s important to set up monitoring to ensure that your application is performing as expected. This can include things like logging, error reporting, and performance metrics.
Clustering for Scalability and Performance
So why should you use clustering in your Node JS applications? There are a number of benefits, including improved scalability and performance.
One of the key benefits of clustering is that it allows your application to handle more requests per second. By distributing incoming requests across multiple worker processes, you can effectively increase the processing power of your application. This is particularly useful for applications that have a large number of users or that need to handle a high volume of traffic.
Clustering can also help improve the responsiveness of your application. By distributing load across multiple processes, you can reduce the amount of time it takes for a single request to be processed. This means that your application will feel faster to users, even if it’s still processing the same number of requests.
Conclusion
Clustering in Node JS is a powerful tool that can help you improve the performance and scalability of your applications. By creating a cluster of child processes, you can distribute incoming requests across multiple processes, improving the processing power of your application. With proper implementation and monitoring, clustering can be a valuable addition to any Node JS application.
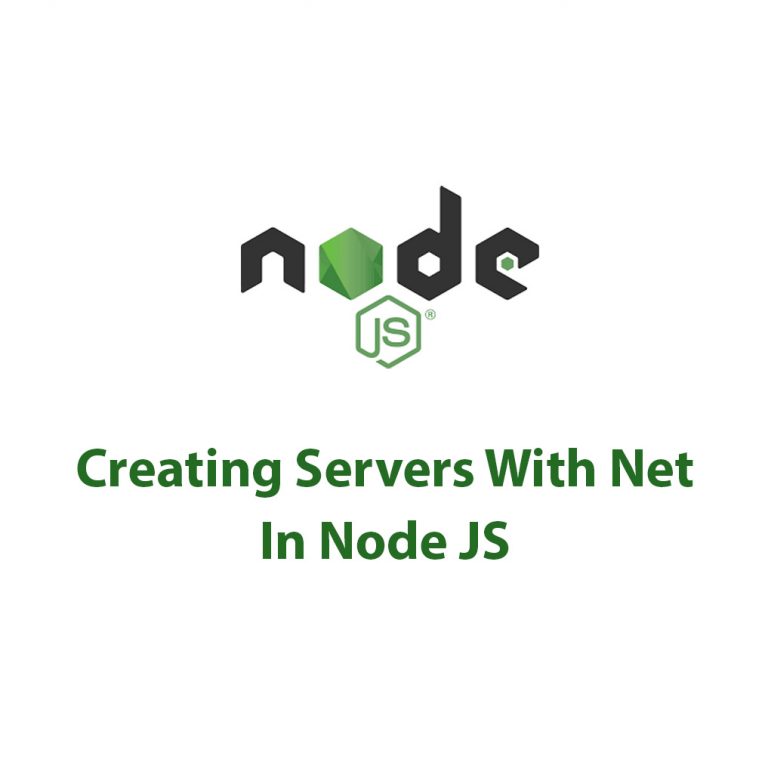
Working With The Net Package In Node JS
As a Node.js developer, I have always been fascinated by the vast array of modules and packages that can be used to simplify the development process. One such package that has long intrigued me is the Net package. In this article, I’ll delve deep into what the Net package is, how to set it up, […]
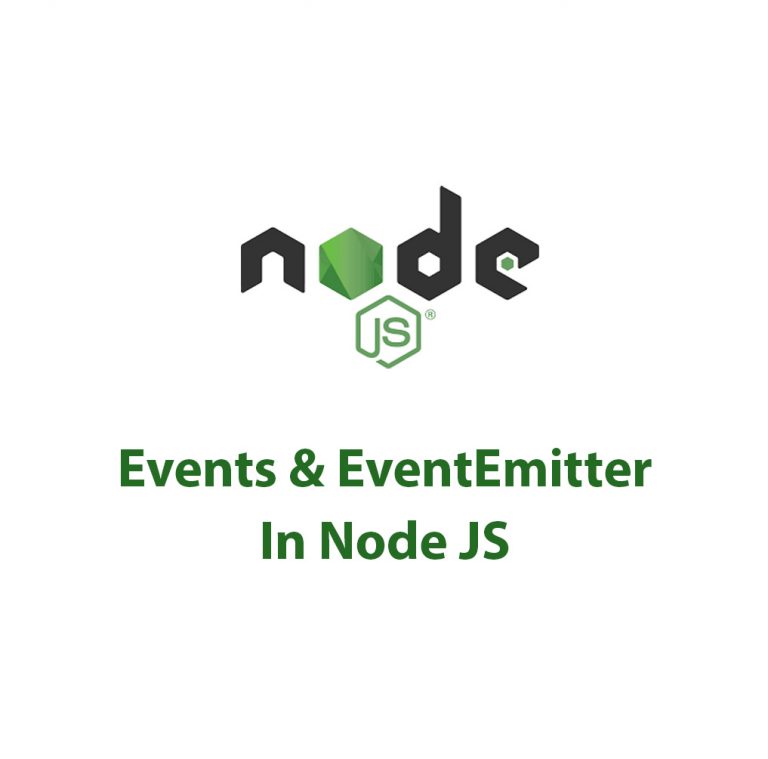
Events In Node JS
Introduction As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought. […]
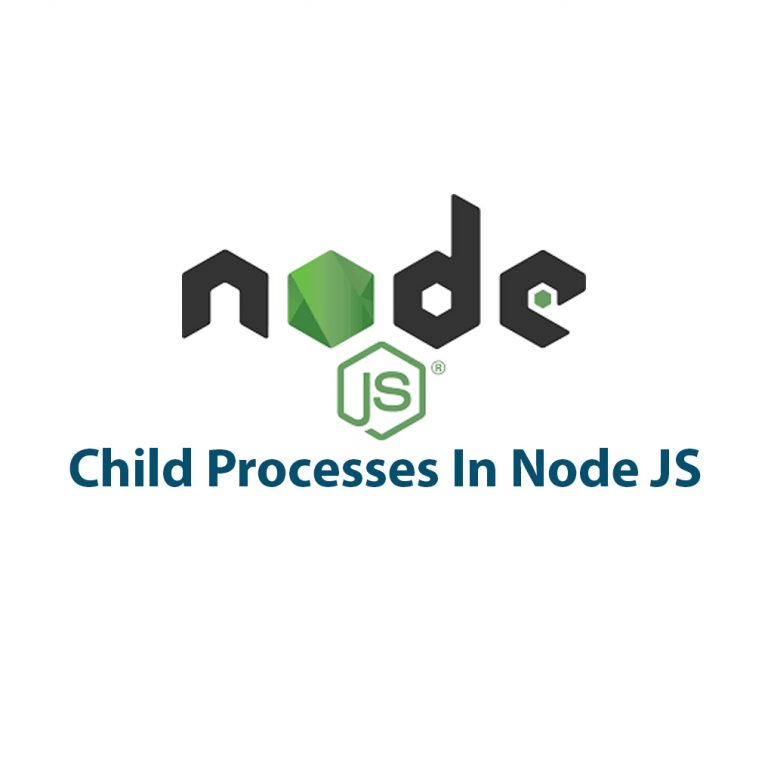
Child Processes In Node JS
Hey there! Today I’m going to talk about Child Processes in Node.js. As you may know, Node.js is a popular open-source, cross-platform, JavaScript runtime environment. It offers a lot of features out of the box, one of them being the ability to work with Child Processes. Child Processes allow you to run multiple processes simultaneously, […]
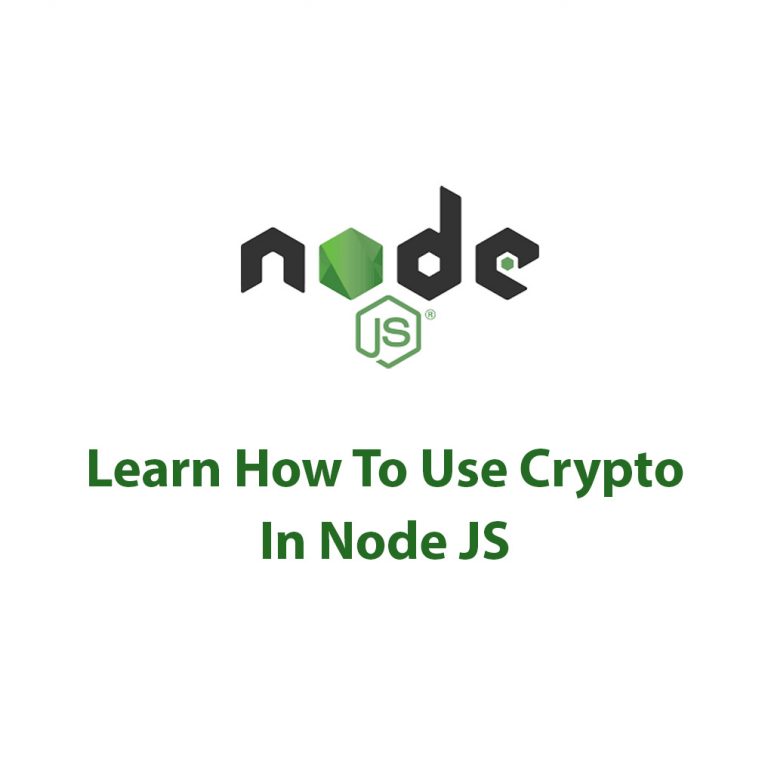
Using Crypto In Node JS
Have you ever wondered how some of the most secure websites and applications keep your data safe from malicious attacks? Well, one of the answers lies in the use of cryptography! Cryptography is the art of writing or solving codes and ciphers, and it has been around for centuries. In the world of computer science, […]
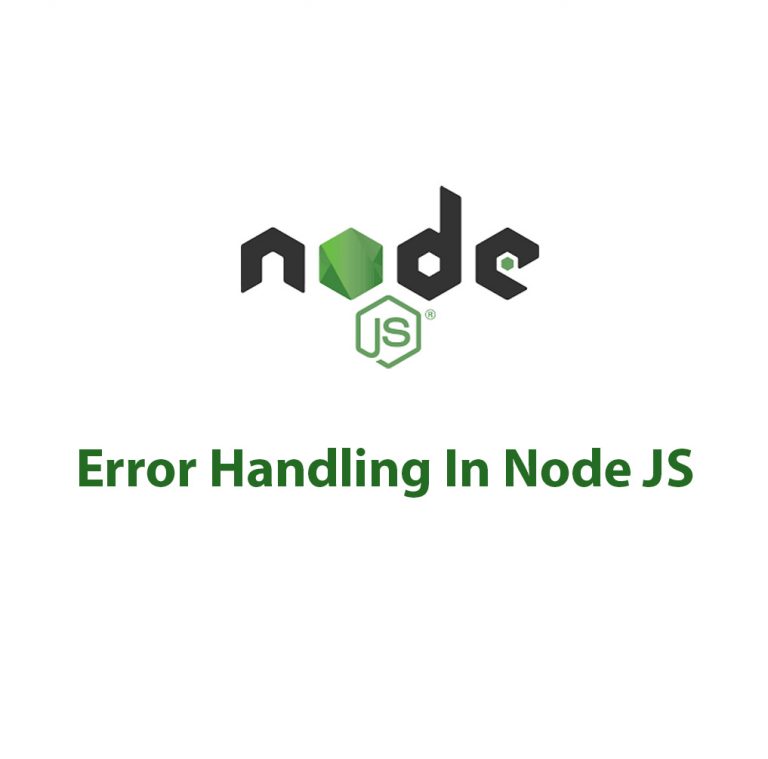
Error Handling in Node JS
A Node JS developer may struggle with error handling on occasion. Although faults are occasionally unavoidable, you can ensure that your application continues to function flawlessly by implementing error handling correctly. Let’s start by discussing the many kinds of problems you could run into when working with Node JS. The Three Types of Errors in […]
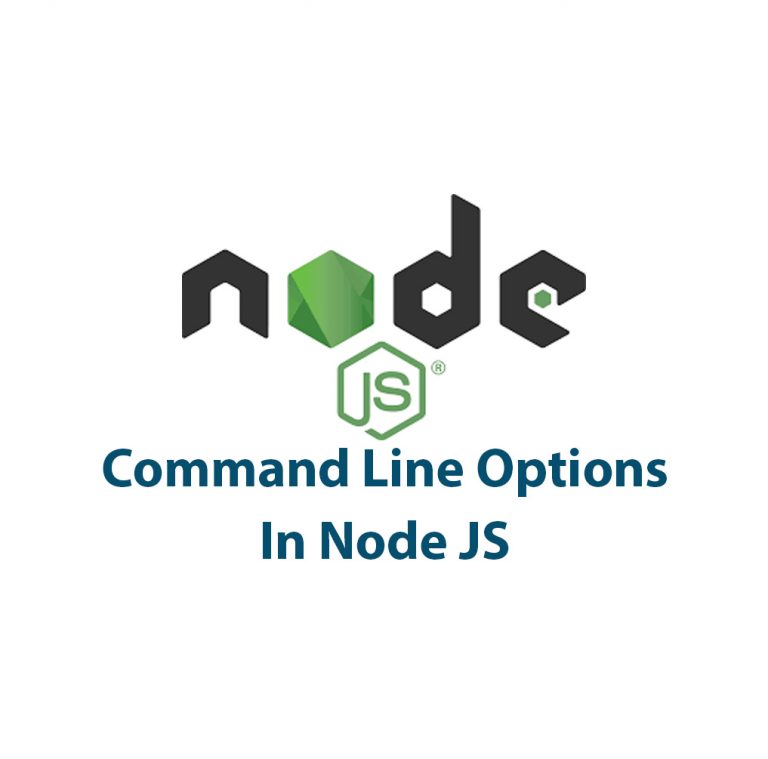
Command Line Options In Node JS
As a developer who loves working with Node JS, I’ve found myself using command line options in many of my projects. Command line options can make our Node JS programs more user-friendly and powerful. In this article, I’ll explain what command line options are, how to parse them in Node JS, how to use them […]