As a developer who loves working with Node JS, I’ve found myself using command line options in many of my projects. Command line options can make our Node JS programs more user-friendly and powerful. In this article, I’ll explain what command line options are, how to parse them in Node JS, how to use them in your programs, and best practices for using them.
What are command line options?
Command line options, sometimes called command line arguments, are parameters passed to an executable program when it’s run. In the context of Node JS, command line options are passed to our Node JS programs when we run them from the command line.
There are two types of command line options: flags and arguments. Flags are boolean options that trigger a certain behavior when passed to the program. Arguments are options that require a value to be passed to them, such as file names or port numbers.
Here’s an example of a Node JS program that accepts command line options:
const program = require('commander');
program
.version('1.0.0')
.option('-p, --port <port>', 'start the server on the specified port')
.option('-P, --production', 'start the server in production mode')
.parse(process.argv);
if (program.port) {
console.log(`Starting server on port ${program.port}`);
}
if (program.production) {
console.log('Starting server in production mode');
}
In this program, we’re using the commander
module to parse command line options. We have two options defined: -p, --port <port>
and -P, --production
. The first option takes an argument, which is the port number that our server should listen on. The second option is a flag that tells our server to run in production mode.
Parsing command line options
Now that we’ve seen an example of a program that uses command line options, let’s look at how we can parse those options in Node JS.
There are several modules available for parsing command line options in Node JS, including commander
, yargs
, and minimist
. In this article, we’ll focus on commander
.
To use commander
, we first need to install it:
npm install commander
Then, in our program, we can require it and define our options using its API:
const program = require('commander');
program
.version('1.0.0')
.option('-p, --port <port>', 'start the server on the specified port')
.option('-P, --production', 'start the server in production mode')
.parse(process.argv);
The version
method sets the version of our program, which will be displayed when the --version
flag is passed. The option
method defines our options. The first argument is the option definition, which consists of a short option (-p
) and a long option (--port
) separated by a comma. The second argument is the description of the option, which will be displayed when the --help
flag is passed. The <port>
part of the definition indicates that this option requires an argument.
Finally, we call parse
method, which parses the arguments passed to our program and populates the program
object with the parsed options.
Once we’ve parsed our options, we can access them using the properties of the program
object:
if (program.port) {
console.log(`Starting server on port ${program.port}`);
}
if (program.production) {
console.log('Starting server in production mode');
}
In this example, we’re checking whether the port
and production
options were passed, and logging messages to the console accordingly.
Using command line options in Node JS programs
Now that we know how to parse command line options, let’s look at how we can use them in our Node JS programs.
One common use case for command line options is to configure our programs. For example, we might want to allow the user to specify the port number that our server should listen on, or the file that our program should process.
Here’s an example of a simple program that reads a file and logs its contents to the console:
const fs = require('fs');
const program = require('commander');
program
.version('1.0.0')
.option('-f, --file <file>', 'read the contents of the specified file')
.parse(process.argv);
if (program.file) {
fs.readFile(program.file, 'utf8', (err, data) => {
if (err) {
console.error(err);
} else {
console.log(data);
}
});
}
In this program, we’re using the fs
module to read a file. We define an option -f, --file <file>
, which takes a file name as an argument. If the user passes the -f
or --file
option to our program, we read the contents of the file and log them to the console.
Best practices for using command line options
Now that we’ve seen how to parse and use command line options in Node JS, let’s look at some best practices for using them.
Firstly, we should make our command line options user-friendly and easy to understand. We should provide clear descriptions of each option and use short and long options that are easy to remember. We should also provide default values for our options where appropriate.
Secondly, we should validate the values of our options. We should ensure that the values are of the expected type and within reasonable bounds. For example, if we’re expecting a port number, we should ensure that it’s a valid port number and not a negative number.
Thirdly, we should handle errors gracefully. If the user passes an invalid option or value, we should provide a helpful error message instead of crashing our program.
Finally, we should test our programs with different combinations of options to ensure that they work as expected.
Conclusion
In this article, we’ve looked at what command line options are, how to parse them in Node JS, how to use them in our programs, and best practices for using them. Command line options can make our programs more user-friendly and powerful, so it’s important to know how to use them effectively.
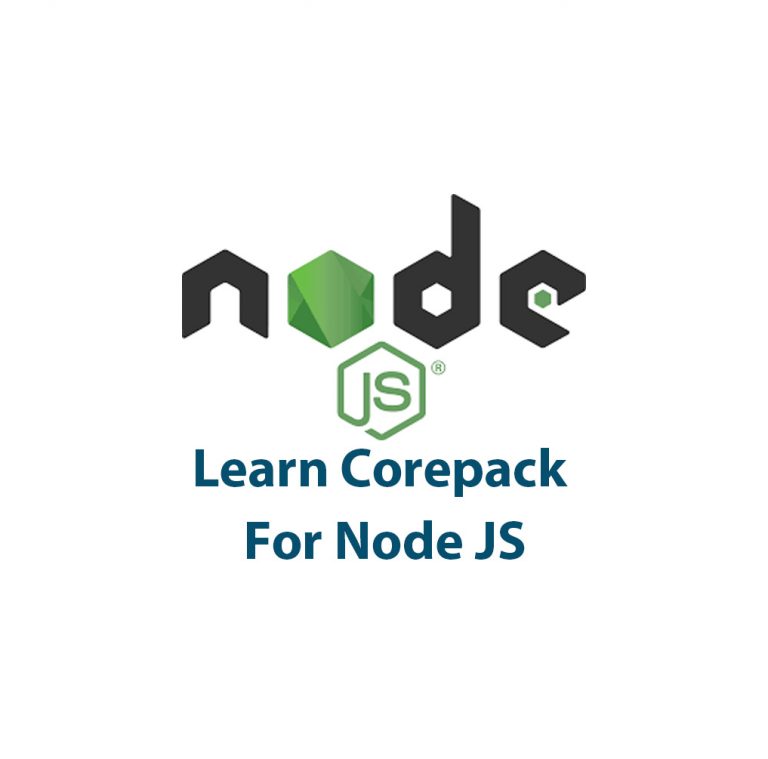
Corepack In Node JS
Have you ever worked on a Node JS project and struggled with managing dependencies? You’re not alone! Managing packages in Node JS can get confusing and messy quickly. That’s where Corepack comes in. In this article, we’ll be diving into Corepack in Node JS and how it can make dependency management a breeze. First of […]
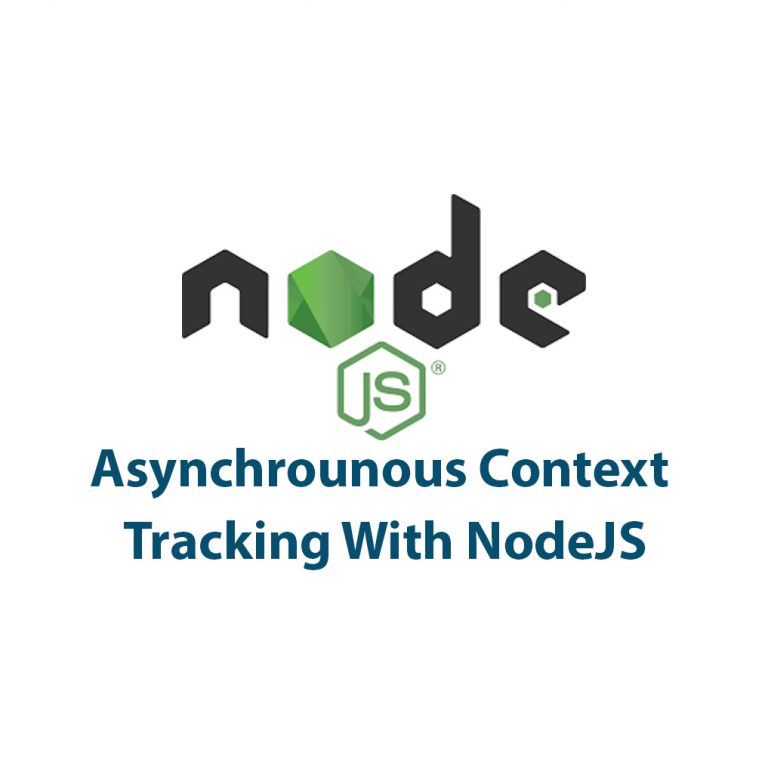
Asynchronous Context Tracking With Node JS
As someone who has spent a lot of time working with Node JS, I have come to understand the importance of Asynchronous Context Tracking in the development of high-performance applications. In this article, I will explore the concept of Asynchronous Context Tracking with Node JS, its advantages, techniques, challenges and best practices. Before we dive […]
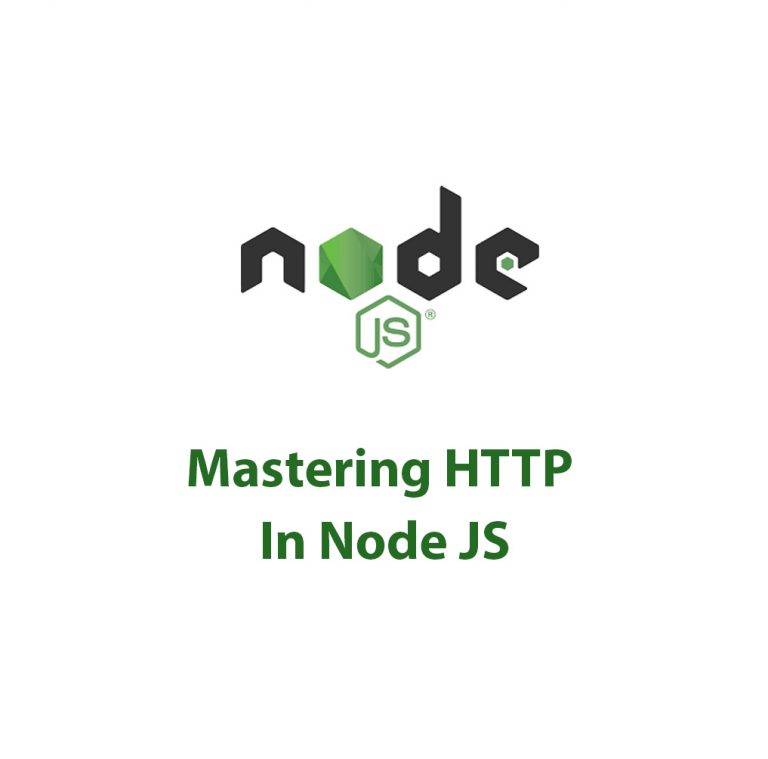
Working With HTTP In Node JS
Introduction As a developer, you may have come across the term HTTP quite a few times. It stands for Hypertext Transfer Protocol and is the backbone of how the internet works. It is the protocol you use when you visit a website, send emails, and watch videos online. In the world of programming, HTTP is […]
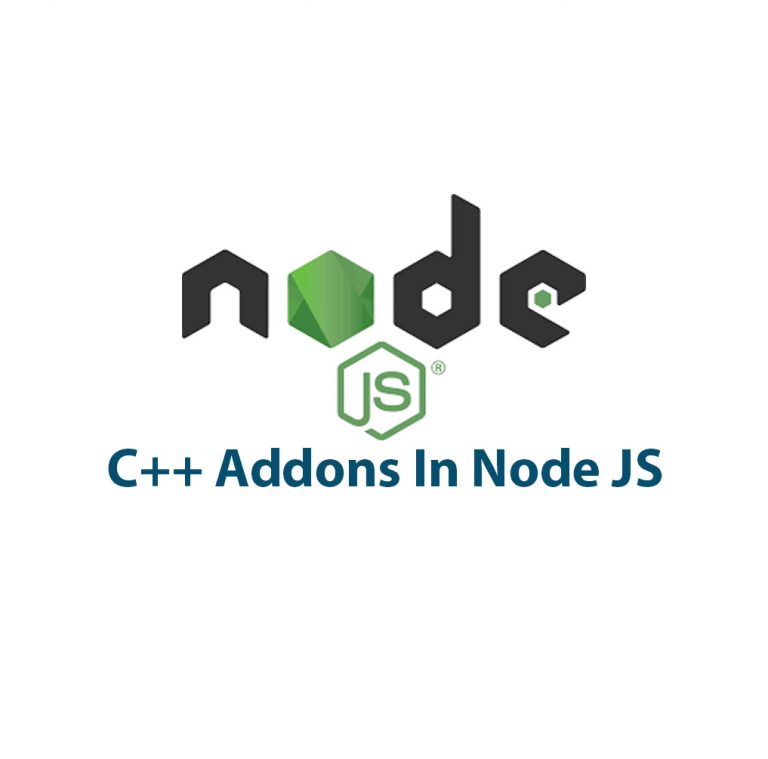
C++ Addons In Node JS
Introduction: As a software developer, I am always looking for ways to improve the performance of my applications. One way to achieve this is by using C++ Addons in Node JS. In this article, we will explore what C++ Addons are, why they are useful in Node JS, and how to create and use them. […]
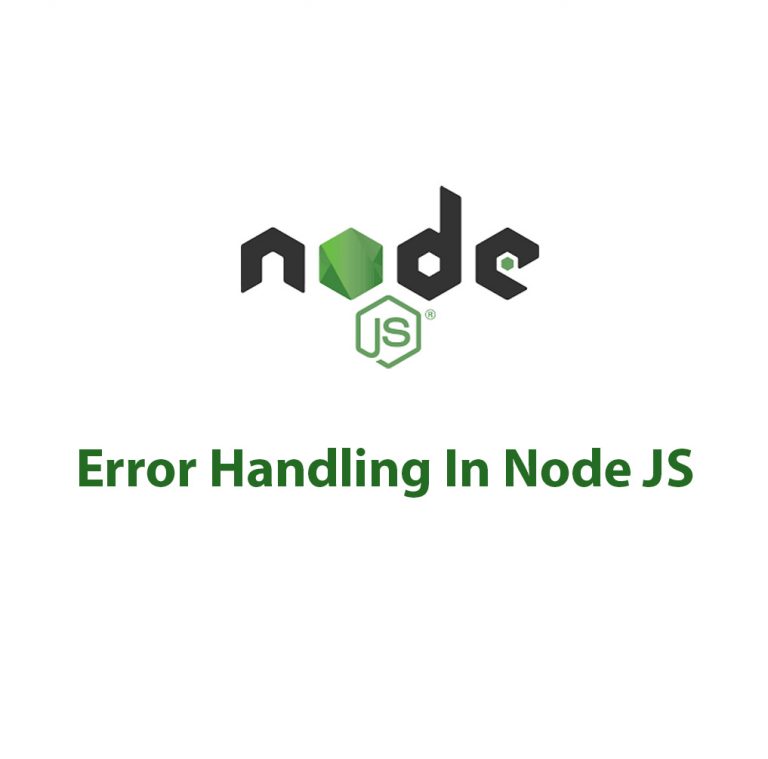
Error Handling in Node JS
A Node JS developer may struggle with error handling on occasion. Although faults are occasionally unavoidable, you can ensure that your application continues to function flawlessly by implementing error handling correctly. Let’s start by discussing the many kinds of problems you could run into when working with Node JS. The Three Types of Errors in […]
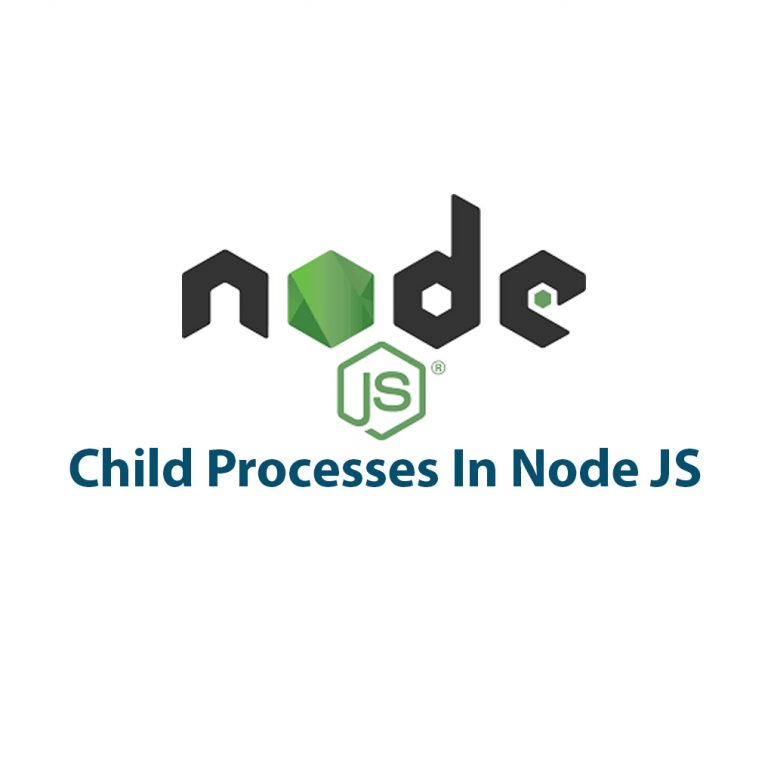
Child Processes In Node JS
Hey there! Today I’m going to talk about Child Processes in Node.js. As you may know, Node.js is a popular open-source, cross-platform, JavaScript runtime environment. It offers a lot of features out of the box, one of them being the ability to work with Child Processes. Child Processes allow you to run multiple processes simultaneously, […]