DNS Package in Node JS: A Complete Guide
As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of the internet that translates domain names into IP addresses. Without it, the internet, as we know it, would not exist.
In my quest for knowledge and improvement, I stumbled upon the DNS package in Node JS. At first, I was perplexed by what it was and how it worked. However, after some research, experiments, and trial-and-error, I now understand the package’s nuances and capabilities. In this article, I will share my discoveries and insights with you.
Installing the DNS Package
Before we dive deep into the DNS package in Node JS, you need to know how to install it first. Fortunately, installing the package is easy, and you can do it in two ways:
- Using NPM Command: You can use the following command to install the DNS package:
npm install dns
- Manually Downloading the Package: If you prefer to download the package manually, you can do so from the official Node JS GitHub repository.
Working With the DNS Package
Once you have installed the DNS package, you can start using its methods and functions. The package provides various methods that you can use to resolve a domain name and retrieve its IP address. Here are the methods and their explanations:
- resolve(): This method resolves the domain name using DNS servers and returns the IP address. You can use this method to resolve a domain name asynchronously, like this:
const dns = require('dns');
dns.resolve('example.com', function (err, addresses) {
if (err) throw err;
console.log('addresses:', addresses);
});
In the code example above, we used the resolve() method to resolve the domain name ‘example.com’ and retrieve its IP address. The function then logs the retrieved IP address to the console.
- reverse(): This method reverses the IP address and returns the domain name associated with it. You can use this method to reverse an IP address as shown below:
const dns = require('dns');
dns.reverse('8.8.8.8', (err, hostnames) => {
console.log('hostnames:', hostnames);
});
In the code snippet above, we used the reverse() method to reverse the IP address ‘8.8.8.8’ and retrieve its corresponding hostnames. The hostnames are then logged to the console.
- lookup(): This method performs a DNS lookup operation for the given hostname and returns both the IP address and the hostname. You can use this method like this:
const dns = require('dns');
dns.lookup('example.com', function onLookup(err, address, family) {
console.log('address:', address);
console.log('family:', family);
});
In the code above, we used the lookup() method to perform a DNS lookup for the ‘example.com’ hostname and retrieve its IP address and family.
DNS Caching
Caching is an essential feature of DNS. It stores data in memory to reduce the amount of time required to resolve a domain name. DNS caching can reduce DNS resolution time and improve website performance. In Node JS, DNS caching is handled automatically by the platform. When a domain name is resolved, the result is stored in memory and can be accessed quickly when needed.
However, DNS caching can cause issues, especially if the DNS cache becomes outdated or corrupted. This can lead to DNS errors and processing delays. To prevent this, you can clear the DNS cache in Node JS using the following method:
dns.flush();
Handling Errors and Failures
As with any programming task, errors and failures can occur when working with the DNS package in Node JS. Below are some common errors that you may encounter and ways to handle them:
- ENOTFOUND errors: This error occurs when the DNS package cannot resolve a domain name. You can handle it using the try-catch blocks, as shown here:
const dns = require('dns');
try {
dns.lookup('example.com', function onLookup(err, address, family) {
console.log('address:', address);
console.log('family:', family);
});
} catch (err) {
console.log('Error:', err);
}
In the code above, we used a try-catch block to handle the ENOTFOUND error when the lookup() method fails to resolve the ‘example.com’ hostname.
- EAI_AGAIN errors: This error occurs when the lookup operation times out while waiting for a response from the DNS server. You can fix it by increasing the DNS timeout setting like so:
const dns = require('dns');
dns.setServers([‘8.8.8.8’]); // use google’s DNS
dns.lookup('example.com', {hints: dns.ADDRCONFIG|dns.V4MAPPED, all: true, timeout: 2000}, function (err, addresses) {
console.log('addresses:', addresses);
});
Real-world Applications
Understanding the DNS package in Node JS is essential for web developers. DNS resolution can have a significant impact on website performance and speed. By utilizing the various methods and functions in the package, developers can optimize their web applications for faster load times and better user experiences.
One use case of the DNS package in Node JS is load balancing. Load balancing refers to the distribution of incoming traffic across multiple servers to avoid overload and improve website performance. By using the DNS package, developers can resolve multiple IP addresses for a domain name and distribute the traffic evenly among them.
Conclusion
In this article, we explored the DNS package in Node JS, its installation process, and the various methods and functions it provides. We also discussed DNS caching, error handling, and real-world applications of the package. As a web developer who is constantly seeking ways to improve website performance, understanding the DNS package in Node JS is a must. With its various capabilities, the DNS package can help you optimize your web applications for faster load times and better user experiences.
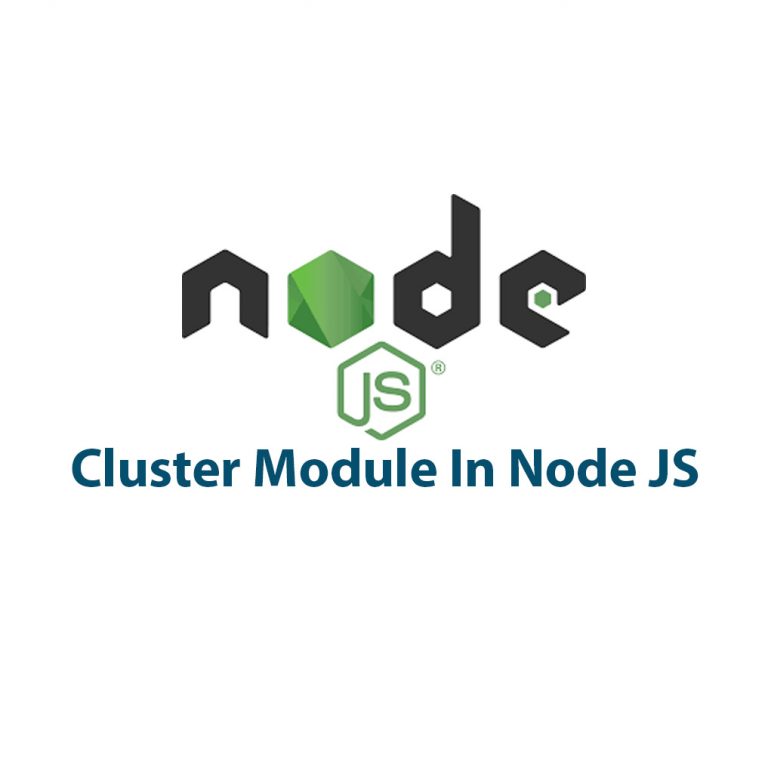
How To Cluster In Node JS
As a developer, I’ve always been interested in exploring different ways to improve the performance of my Node JS applications. One tool that has proven to be immensely beneficial is cluster module. In this article, we’ll dive deep into clustering for Node JS, how it works, how to implement it, and the benefits it provides. […]
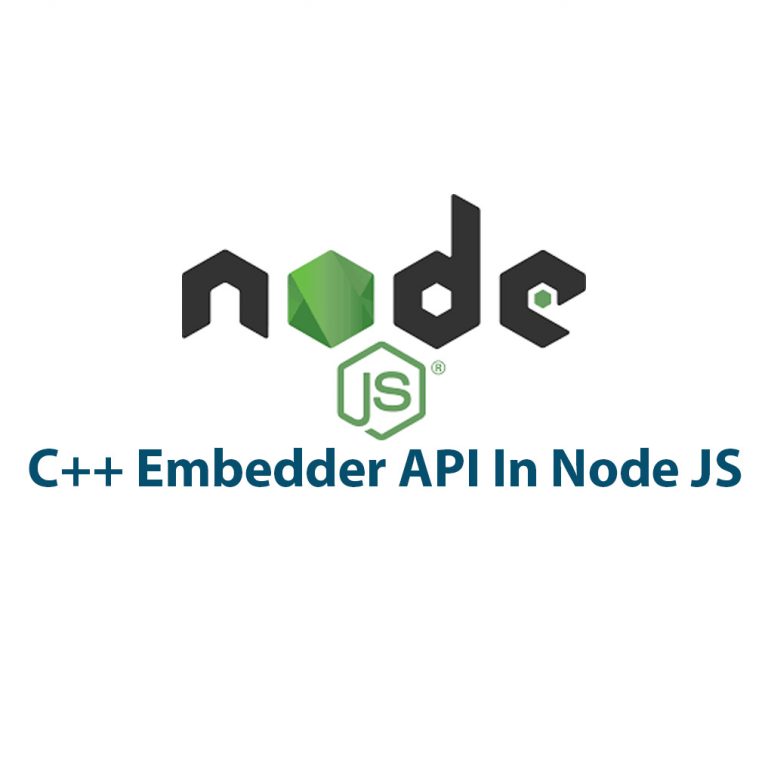
C++ Embedder API With Node JS
Introduction: As a programmer, I have always been fascinated with the power of NodeJS. It is a popular JavaScript runtime that can be used for server-side scripting. The beauty of NodeJS is that it allows for easy handling of I/O operations. However, sometimes the complexities of a project may go beyond just JavaScript coding, and […]
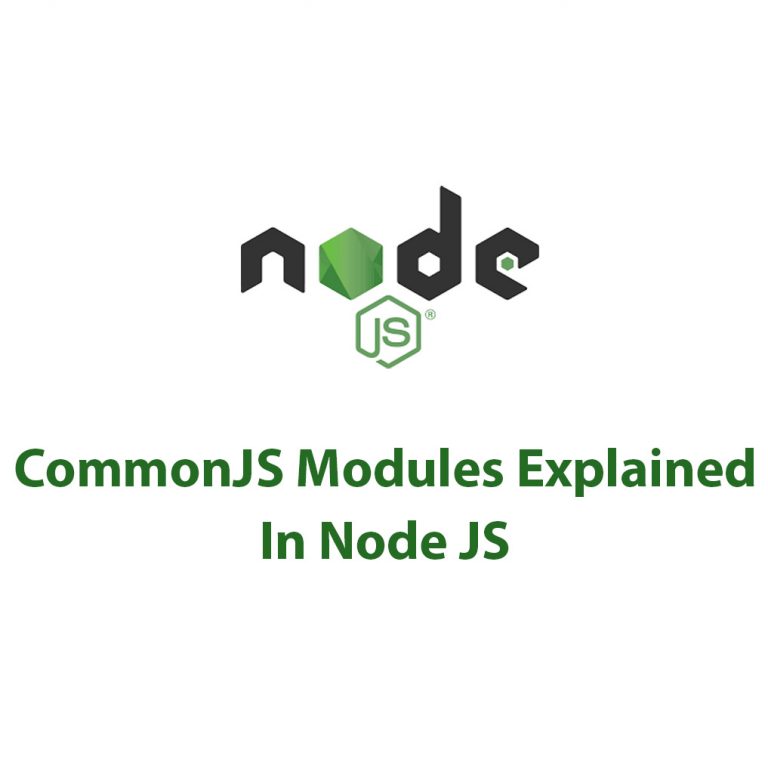
CommonJS Modules In Node JS
Introduction As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has […]
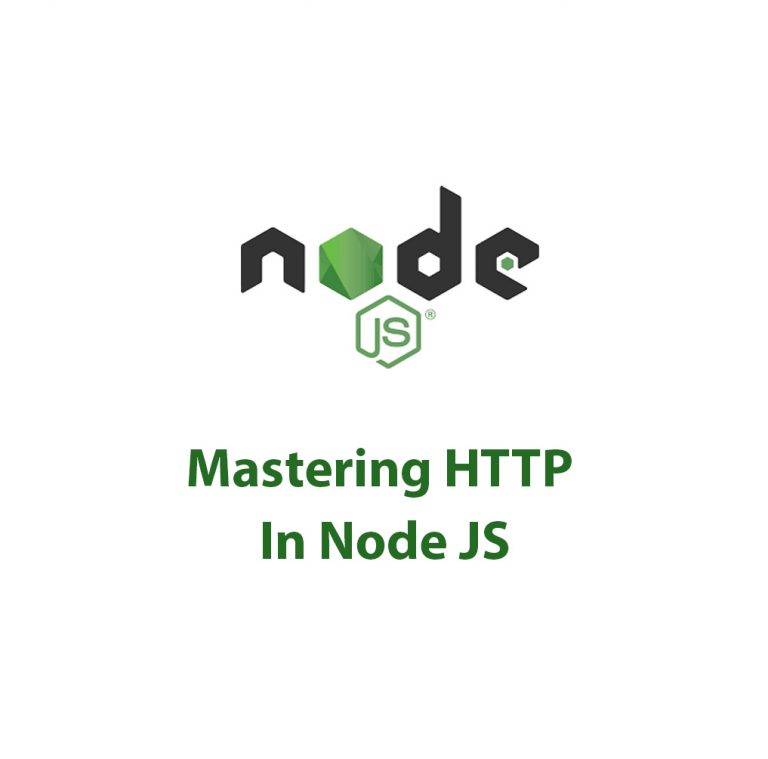
Working With HTTP In Node JS
Introduction As a developer, you may have come across the term HTTP quite a few times. It stands for Hypertext Transfer Protocol and is the backbone of how the internet works. It is the protocol you use when you visit a website, send emails, and watch videos online. In the world of programming, HTTP is […]
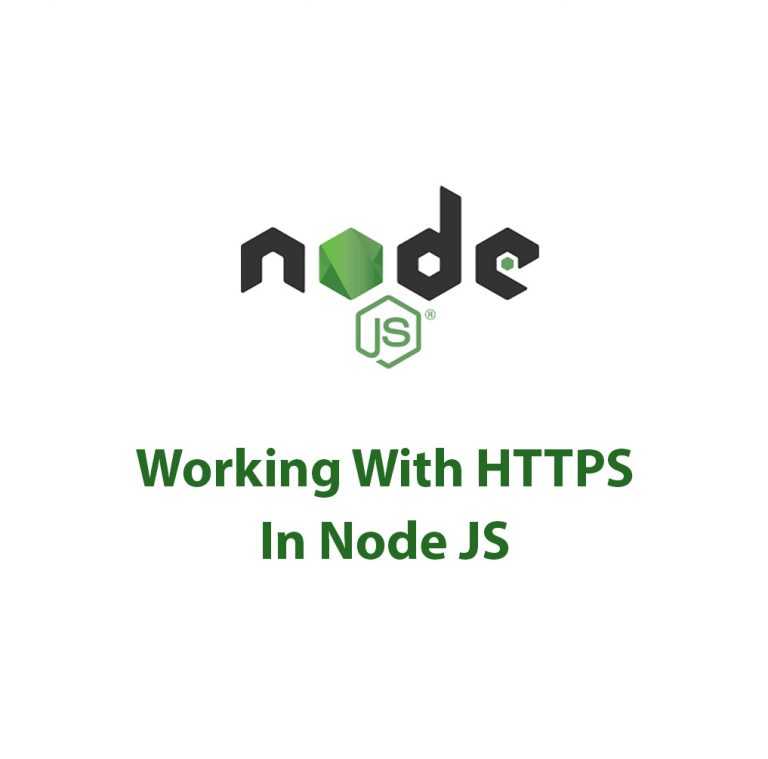
Working With HTTPS In Node JS
As a developer, I’m always on the lookout for security protocols that can help me protect users’ sensitive information. HTTP, while functional, lacks the encryption necessary to truly secure data transmission over the web. This is where HTTPS comes in. But working with HTTPS can be a bit daunting, especially if you’re new to it. […]
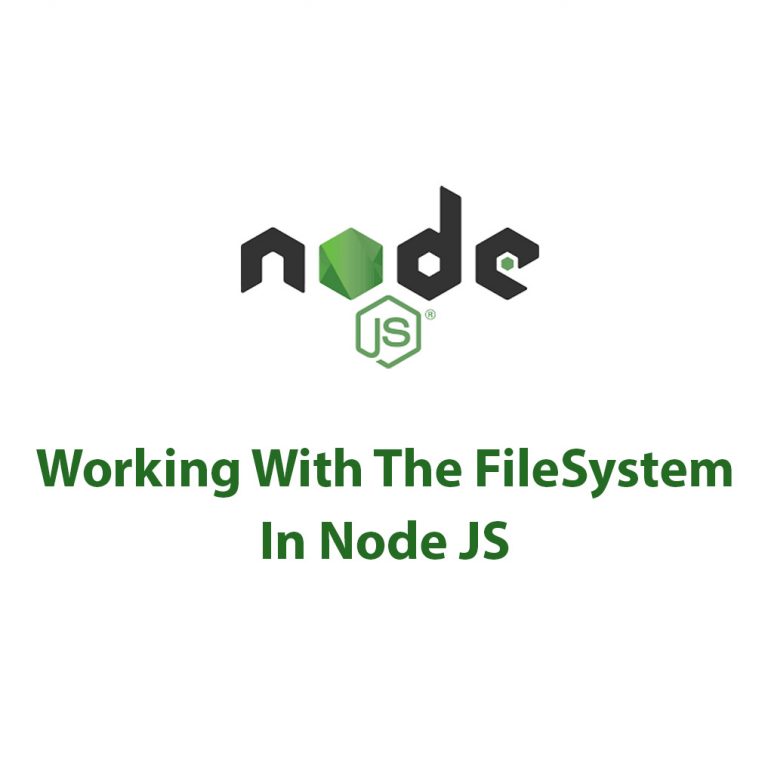
Working With The FileSystem In Node JS
Working With The FileSystem In Node.js Node.js is a powerful platform for building web applications and backend services. One of the most common things that developers need to do is work with files and the file system. This involves creating, modifying, and deleting files, as well as reading from and writing to them. In this […]