A Node JS developer may struggle with error handling on occasion. Although faults are occasionally unavoidable, you can ensure that your application continues to function flawlessly by implementing error handling correctly.
Let’s start by discussing the many kinds of problems you could run into when working with Node JS.
The Three Types of Errors in Node JS
Syntax Errors
When there is a problem in the code’s structure, syntax errors happen. For instance, using erroneous syntax or omitting a semicolon somewhere in your code. Your editor will instantly point up any syntax mistakes and provide you with thorough error messages that identify where the issue was made.
Runtime Errors
When your code suddenly breaks or when an undefined variable is referenced, runtime errors in Node JS occur. Runtime mistakes can be severe and take on a variety of shapes. Out of memory faults, network issues that interfere with program functionality, and other issues are examples of this.
Logic Errors
Logic mistakes in Node JS happen when your code doesn’t yield the desired outcomes. For instance, if your function returns a string when it should have returned a number. Logic mistakes can be challenging to spot and may require some investigation to find.
Implementing Error Handling in Node JS
There are several ways to implement error handling in Node JS, but I’m going to go over three of the most popular methods: try-catch blocks, error-first callbacks, and custom error objects.
Using Try-Catch Blocks
Try-catch blocks provide a simple way to catch errors that may occur in a block of code. The try block contains the code that may generate the exception, and the catch block handles the exception.
try {
// some code that may produce an error
} catch (error) {
// handle the error
}
When an error gets caught by the catch block, you can then handle the error and take necessary steps to ensure your code continues to run smoothly.
Using Error-First Callbacks
The “error-first callbacks” standard used by Node.js enables you to handle errors that could happen while running asynchronous code. The majority of Node.js’s features, such as reading and writing files and sending HTTP requests, are included in this.
A function that adheres to a particular callback pattern is said to be an error-first callback. An error object is always sent as the callback function’s first argument. Any further parameters are undefined if a function call error occurs, but the error object contains a value. If the function call is successful, any further arguments are returned together with the error object being null or undefined.
function doSomethingAsync(callback) {
asyncFunction((error, result) => {
if (error) {
callback(error)
} else {
callback(null, result)
}
})
}
The doSomethingAsync
function takes a callback function as an argument. The asyncFunction
is an asynchronous function that could potentially return an error, but if the function call is successful, it returns a result. By using the error-first callback pattern, we ensure that any error that may occur can be caught and handled without affecting the rest of the code.
Using Custom Error Objects
Sometimes you may want to create custom error objects that give more detail about what went wrong. When you create a custom error object, you can include more information about the error that occurred, which can be useful when using third-party libraries or debugging your application.
function CustomError(message) {
this.name = 'CustomError'
this.message = message || 'Default error message'
this.stack = (new Error()).stack
}
CustomError.prototype = Object.create(Error.prototype)
CustomError.prototype.constructor = CustomError
In this example, we are creating a custom error object called CustomError
, which includes a name, message, and stack trace. By doing this, we can easily identify where the error occurred, what it was, and how to fix it.
Best Practices for Error Handling in Node JS
While implementing error handling in Node JS, it’s important to keep some best practices in mind. Here are some best practices that I found to be useful:
Consistent Error Messages
Ensure that the error messages are clear and precise, and follow some conventions across the application. This makes it easier to locate the source of an error and resolve it quickly.
Logging Errors
Logging errors gives you useful information that can help you track down the root cause of the error. You can use Node.js built-in logger or any other third-party libraries to log errors stored in a file or send them to some third party logging service.
Propagating Errors
When an error occurs in a lower-level function, the higher-level function should have access to that error to take the right action based on the error. This is known as propagating the error. Propagating errors can be done using error-first callbacks or even custom error objects.
Conclusion
The management of errors is a crucial component of Node JS development. We discussed the three sorts of Node JS errors that are frequently made, namely syntax errors, runtime errors, and logic errors, in this article. Three well-known error-handling strategies were also covered: try-catch blocks, error-first callbacks, and custom error objects.
Last but not least, we discussed a few recommended practices to bear in mind when integrating error handling into your Node JS application, such as consistent error messages, logging errors, and propagating problems.
You may reduce errors and maintain the efficiency of your Node JS application by incorporating these strategies and best practices.
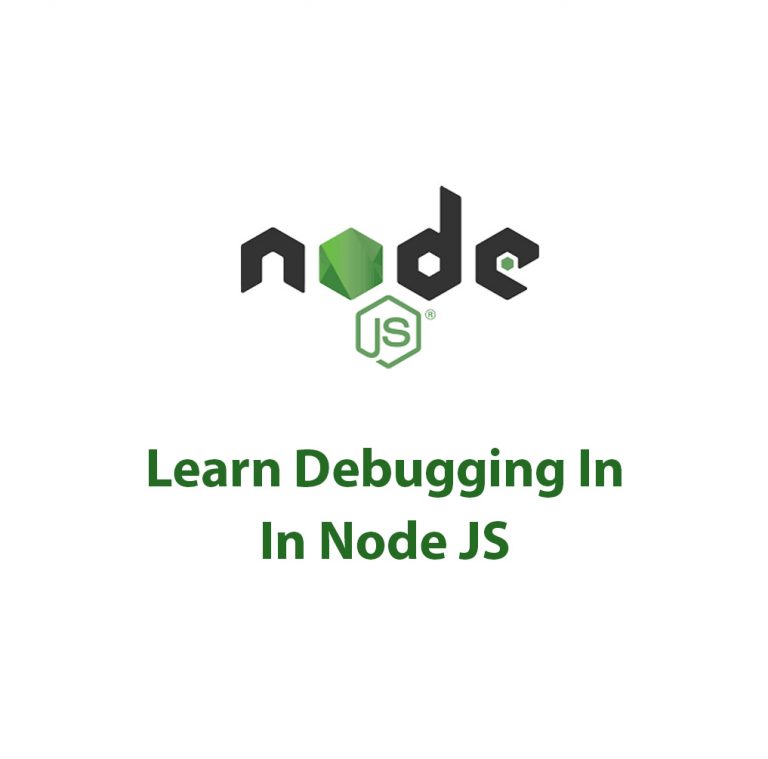
Debugger In Node JS
Debugger In Node JS: Getting a Deeper Understanding One thing we might all have in common as developers in the always changing tech industry is the ongoing need to come up with new and better approaches to debug our code. Since troubleshooting is a crucial step in the development process, we must be well-equipped with […]
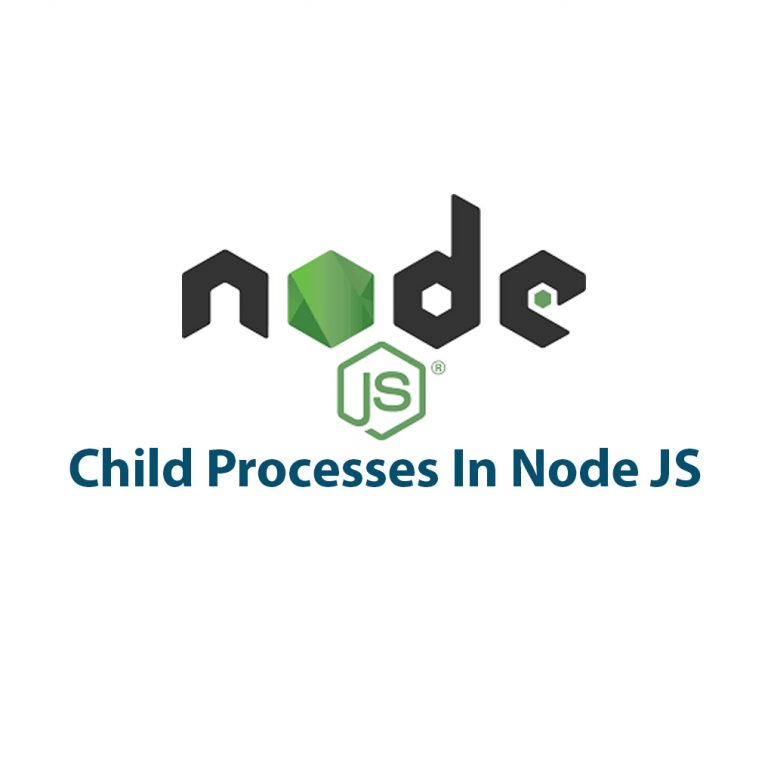
Child Processes In Node JS
Hey there! Today I’m going to talk about Child Processes in Node.js. As you may know, Node.js is a popular open-source, cross-platform, JavaScript runtime environment. It offers a lot of features out of the box, one of them being the ability to work with Child Processes. Child Processes allow you to run multiple processes simultaneously, […]
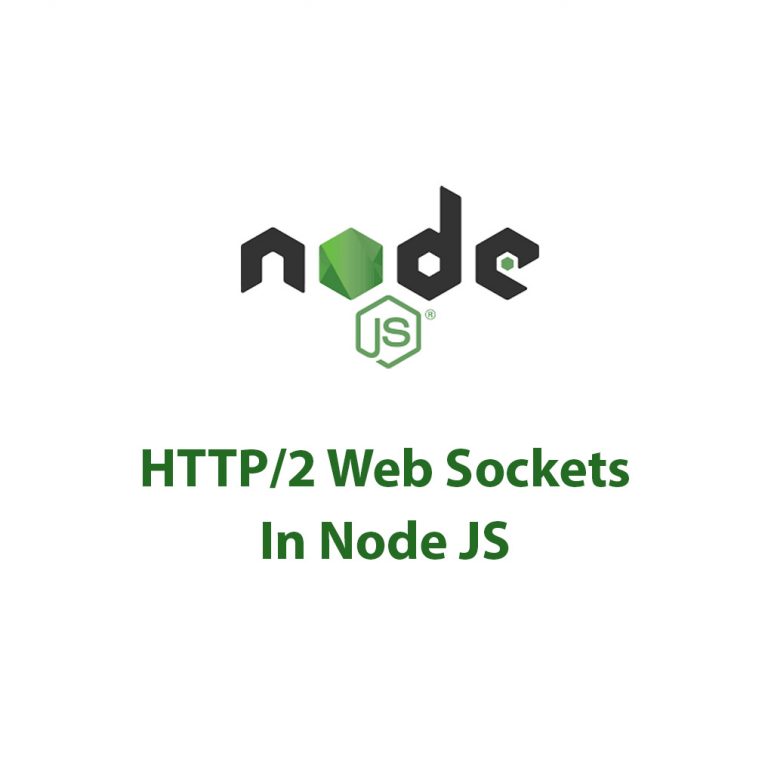
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
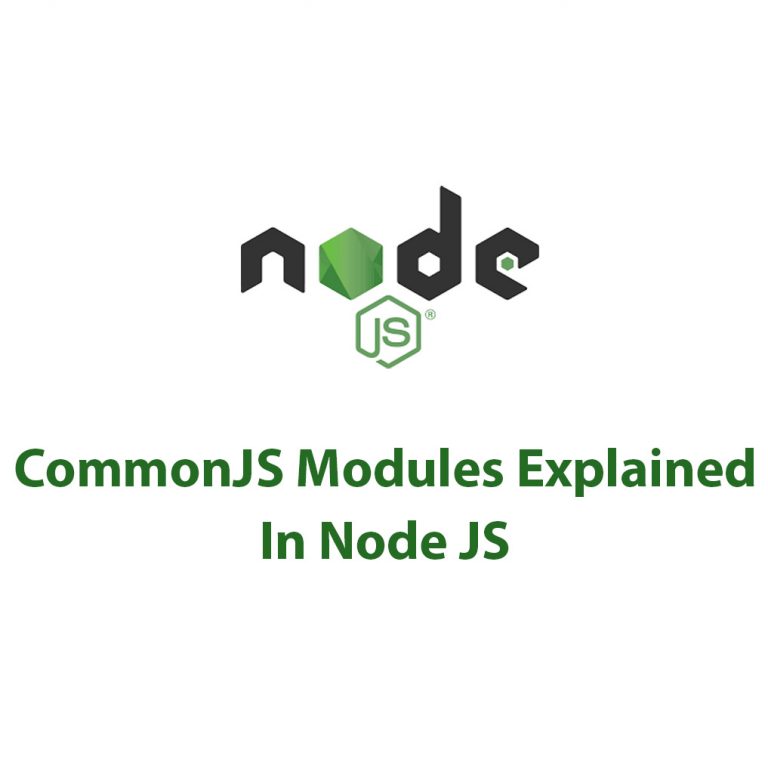
CommonJS Modules In Node JS
Introduction As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has […]
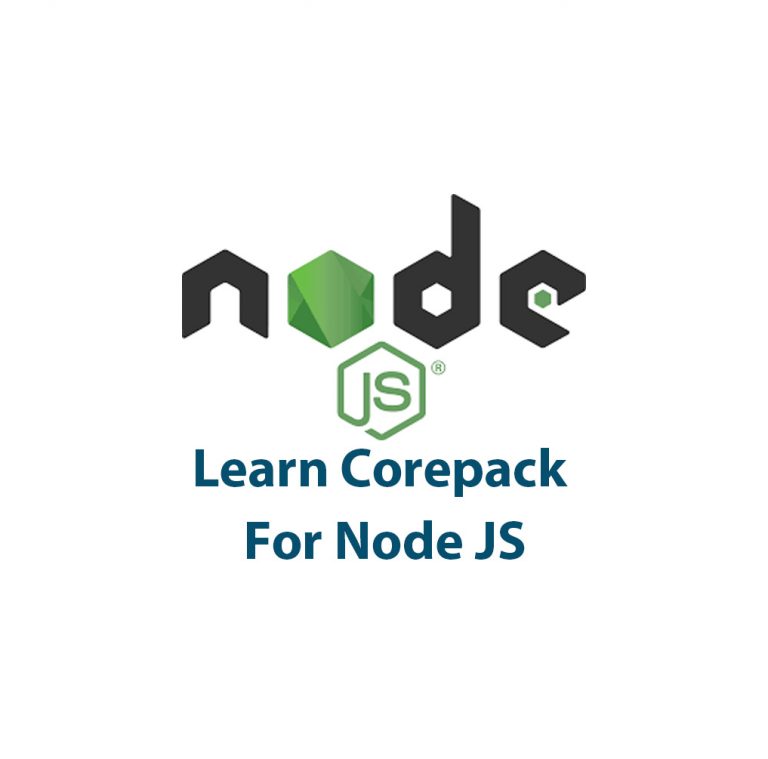
Corepack In Node JS
Have you ever worked on a Node JS project and struggled with managing dependencies? You’re not alone! Managing packages in Node JS can get confusing and messy quickly. That’s where Corepack comes in. In this article, we’ll be diving into Corepack in Node JS and how it can make dependency management a breeze. First of […]
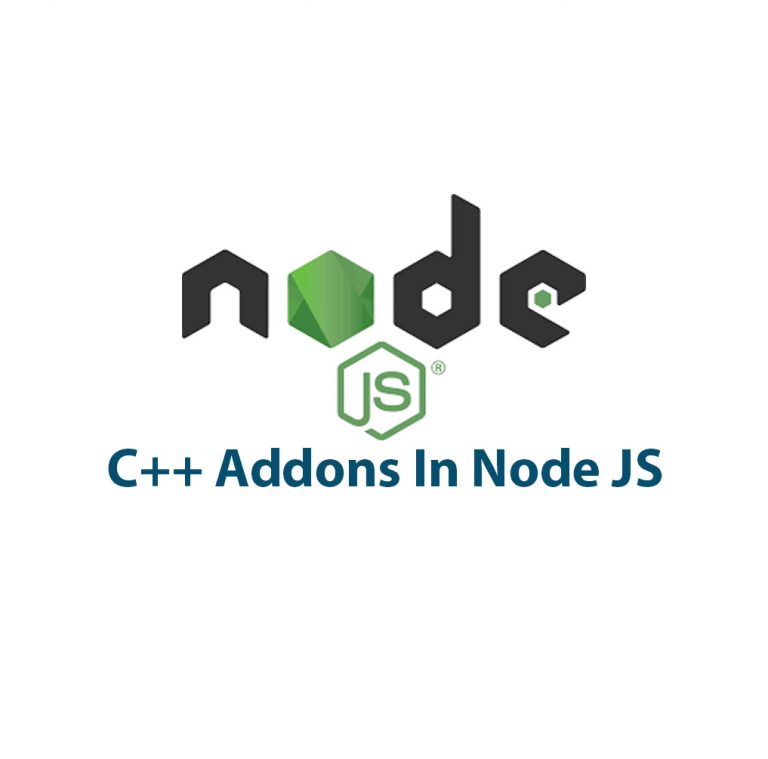
C++ Addons In Node JS
Introduction: As a software developer, I am always looking for ways to improve the performance of my applications. One way to achieve this is by using C++ Addons in Node JS. In this article, we will explore what C++ Addons are, why they are useful in Node JS, and how to create and use them. […]