Working With The FileSystem In Node.js
Node.js is a powerful platform for building web applications and backend services. One of the most common things that developers need to do is work with files and the file system. This involves creating, modifying, and deleting files, as well as reading from and writing to them.
In this article, I will explore how to work with the FileSystem module in Node.js. I will cover the basics of the FileSystem module, file system operations, synchronous and asynchronous file system operations, file streams, and watching files for changes. I will also provide real world examples of using the FileSystem module in Node.js.
Starting With The Basics
To get started with the FileSystem module, we need to import it into our Node.js application. We do this by requiring the module using the require function.
const fs = require('fs');
Once we have imported the FileSystem module, we can start using it to create, read, update and delete files.
Creating Files Using FileSystem Module
To create a new file, we use the fs.writeFile method. This method takes three arguments: the name of the file, the content of the file, and a callback function.
fs.writeFile('example.txt', 'Hello World!', function (err) {
if (err) throw err;
console.log('File created.');
});
In this example, we create a file called example.txt with the content ‘Hello World!’. When the file is successfully created, the callback function is executed and a message is logged to the console.
Reading Files Using FileSystem Module
To read the contents of a file, we use the fs.readFile method. This method takes two arguments: the name of the file and a callback function.
fs.readFile('example.txt', function (err, data) {
if (err) throw err;
console.log(data.toString());
});
In this example, we read the contents of the file example.txt. When the file is successfully read, the callback function is executed and the contents of the file are logged to the console.
Writing Files Using FileSystem Module
To write to an existing file, we use the fs.writeFile method. This method takes three arguments: the name of the file, the content to be written, and a callback function.
fs.writeFile('example.txt', 'New Content!', function (err) {
if (err) throw err;
console.log('File updated.');
});
In this example, we update the content of the file example.txt with ‘New Content!’. When the file is successfully updated, the callback function is executed and a message is logged to the console.
Deleting Files Using FileSystem Module
To delete a file, we use the fs.unlink method. This method takes the name of the file and a callback function.
fs.unlink('example.txt', function (err) {
if (err) throw err;
console.log('File deleted.');
});
In this example, we delete the file example.txt. When the file is successfully deleted, the callback function is executed and a message is logged to the console.
Filesystem Operations
The FileSystem module also provides methods for creating directories, renaming files, and updating files.
Creating a New Directory
To create a new directory, we use the fs.mkdir method. This method takes the name of the directory and a callback function.
fs.mkdir('exampleDir', function (err) {
if (err) throw err;
console.log('Directory created.');
});
In this example, we create a new directory called exampleDir. When the directory is successfully created, the callback function is executed and a message is logged to the console.
Renaming a File
To rename a file, we use the fs.rename method. This method takes two arguments: the name of the file to be renamed and the new name of the file, and a callback function.
fs.rename('example.txt', 'renamed.txt', function (err) {
if (err) throw err;
console.log('File renamed.');
});
In this example, we rename the file example.txt to renamed.txt. When the file is successfully renamed, the callback function is executed and a message is logged to the console.
Deleting a File
To delete a file, we use the fs.unlink method as described earlier.
Updating a File
To update the contents of a file, we use the fs.writeFile method as described earlier.
Synchronous Vs Asynchronous Filesystem Operations
Node.js supports both synchronous and asynchronous file system operations.
Synchronous filesystem operations block the main thread until the operation is complete, while asynchronous filesystem operations do not block the main thread and allow other code to execute while the operation is in progress.
Synchronous filesystem operations can make your application feel less responsive, so it is generally recommended to use asynchronous filesystem operations whenever possible.
Example of Writing a File Synchronously
To write a file synchronously, we use the fs.writeFileSync method. This method takes two arguments: the name of the file and the content to be written.
fs.writeFileSync('example.txt', 'Hello World Synchronously!');
console.log('File created synchronously.');
In this example, we create a file called example.txt and write the content ‘Hello World Synchronously!’ to it. The console.log statement is executed after the file has been successfully written.
Example of Writing a File Asynchronously
To write a file asynchronously, we use the fs.writeFile method as described earlier.
fs.writeFile('example.txt', 'Hello World Asynchronously!', function (err) {
if (err) throw err;
console.log('File created asynchronously.');
});
In this example, we create a file called example.txt and write the content ‘Hello World Asynchronously!’ to it. The callback function is executed when the file has been successfully written.
File Streams
Node.js also provides a mechanism called file streams that allow us to read and write large files efficiently.
Overview of File Streams
A stream is a flow of data between two entities. In the case of file streams, data is read from or written to a file in chunks. This allows us to work with files that are too large to fit into memory all at once.
Reading Data From File Streams
To read data from a file stream, we create a read stream using the fs.createReadStream method. This method takes the name of the file as an argument.
const readStream = fs.createReadStream('example.txt');
readStream.on('data', function (chunk) {
console.log(chunk);
});
In this example, we create a read stream for the file example.txt. When we have data available, the ‘data’ event is emitted and we log the chunk of data to the console.
Writing Data To File Streams
To write data to a file stream, we create a write stream using the fs.createWriteStream method. This method takes the name of the file as an argument.
const writeStream = fs.createWriteStream('example.txt');
writeStream.write('Hello World!');
writeStream.end();
In this example, we create a write stream for the file example.txt. We write the content ‘Hello World!’ to the stream using the write method and then close the stream using the end method.
Piping Streams
Another powerful feature of file streams is the ability to pipe data between streams. This allows us to chain multiple streams together to perform complex operations on our data.
For example, we may have a large file that we want to compress and write to a new file. We can accomplish this with the following code:
const zlib = require('zlib');
const readStream = fs.createReadStream('example.txt');
const gzip = zlib.createGzip();
const writeStream = fs.createWriteStream('example.txt.gz');
readStream.pipe(gzip).pipe(writeStream);
In this example, we create a read stream for the file example.txt. We then create a gzip stream using the zlib module and a write stream for the compressed file. We pipe the data from the read stream to the gzip stream and then to the write stream, which compresses the data and writes it to the new file.
Watching Files For Changes
In some applications, we may need to watch a file for changes and perform an action when the file is modified. Node.js provides the fs.watch method for this purpose.
Overview of Watching Files For Changes
The fs.watch method allows us to watch a file or directory for changes. When a change is detected, the ‘change’ event is emitted and we can perform an action in response.
Using the FileSystemWatcher Module
To watch a file, we create a FileSystemWatcher object using the fs.watch method. This method takes the name of the file to watch as an argument.
const watcher = fs.watch('example.txt');
watcher.on('change', function (event, filename) {
console.log('File changed: ' + filename);
});
In this example, we create a FileSystemWatcher object for the file example.txt. When a change is detected, the ‘change’ event is emitted and we log a message to the console.
Handling Changes In Files
In our event listener for the ‘change’ event, we can perform any actions we need to in response to the change.
For example, we may want to read the contents of the file and send an email notification to a user.
const watcher = fs.watch('example.txt');
watcher.on('change', function (event, filename) {
console.log('File changed: ' + filename);
fs.readFile('example.txt', function (err, data) {
if (err) throw err;
sendEmail(data.toString());
});
});
In this example, we read the contents of the file example.txt and send an email notification to the user using the sendEmail function.
Real World Applications
The FileSystem module is an essential part of building backend services in Node.js. Let’s take a look at how we might use the module in a real world application.
Example of Creating a New File In An Application
Suppose we are building a web application that allows users to upload images. We want to save these images to a directory on our server.
const express = require('express');
const multer = require('multer');
const app = express();
const upload = multer({ dest: 'uploads/' });
app.post('/upload', upload.single('image'), function (req, res, next) {
const filename = req.file.filename;
const path = req.file.path;
fs.rename(path, 'uploads/' + filename, function (err) {
if (err) throw err;
res.send('File uploaded and saved.');
});
});
In this example, we use the multer middleware to handle the file upload. We save the file to a temporary directory and then rename the file and move it to our uploads directory using the fs.rename method.
Example of Reading From A File In An Application
Suppose we are building a web application that allows users to view their profile information. We want to store this information in a JSON file on our server.
const express = require('express');
const app = express();
app.get('/profile/:username', function (req, res, next) {
const username = req.params.username;
fs.readFile('profiles/' + username + '.json', function (err, data) {
if (err) throw err;
const profile = JSON.parse(data);
res.send(profile);
});
});
In this example, we read the contents of the JSON file for the specified user and send the contents to the client as a response.
Conclusion
The FileSystem module is an essential part of building backend services in Node.js. In this article, we explored the basics of the FileSystem module, file system operations, synchronous and asynchronous file system operations, file streams, and watching files for changes. We also provided real world examples of using the FileSystem module in Node.js.
Working with files and the file system can be a complex task, but Node.js provides powerful tools to make it easier. By using the FileSystem module effectively, we can build fast and reliable web applications and backend services that can handle large amounts of data.
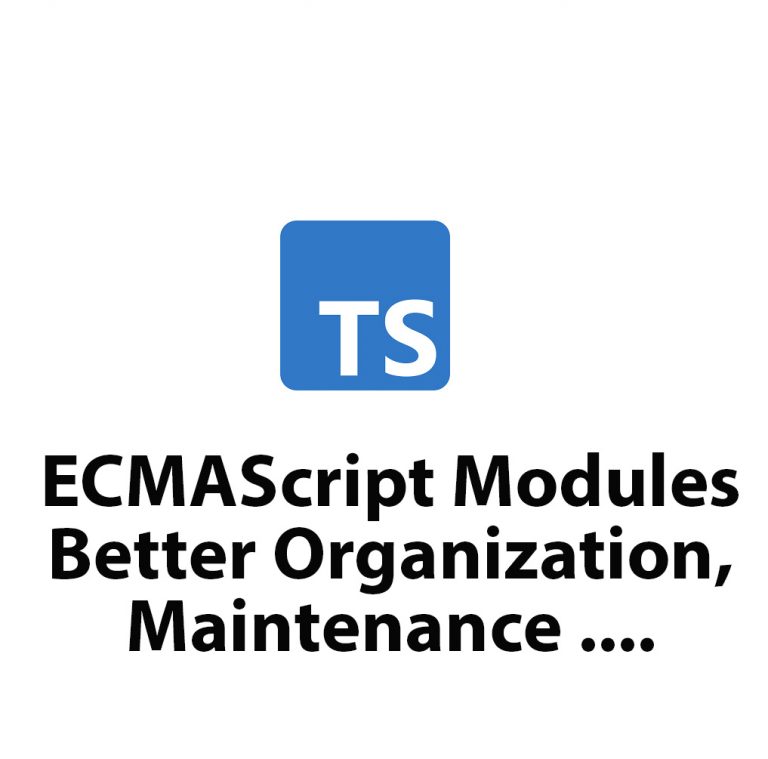
ECMAScript Modules in Node JS
As a software developer and avid Node JS user, I’ve always been on the lookout for ways to improve my workflow and simplify code maintenance. One of the most recent additions to Node JS that has greatly helped me achieve these goals is the implementation of ECMAScript (ES) Modules. ES Modules are a standard format […]
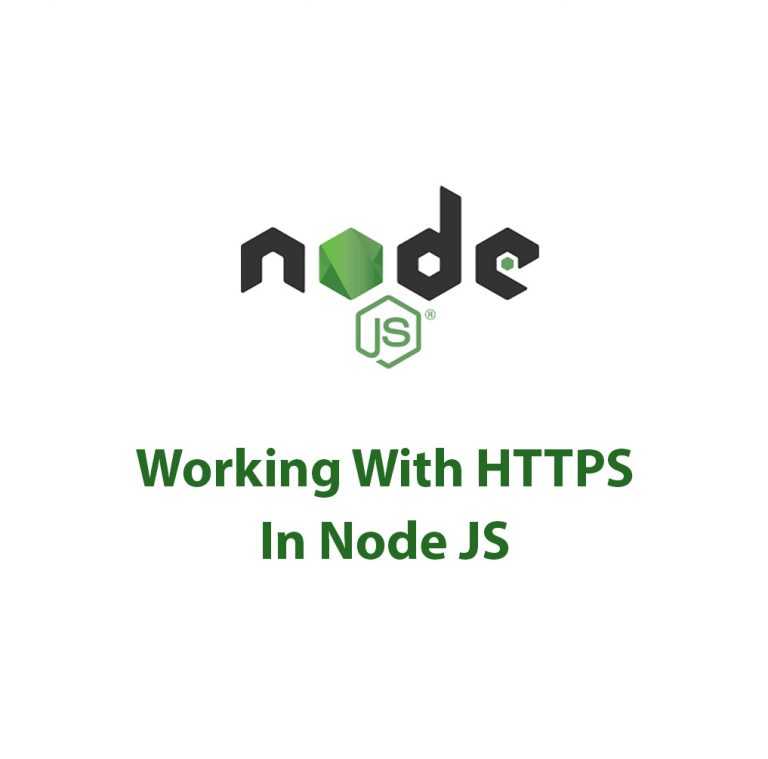
Working With HTTPS In Node JS
As a developer, I’m always on the lookout for security protocols that can help me protect users’ sensitive information. HTTP, while functional, lacks the encryption necessary to truly secure data transmission over the web. This is where HTTPS comes in. But working with HTTPS can be a bit daunting, especially if you’re new to it. […]
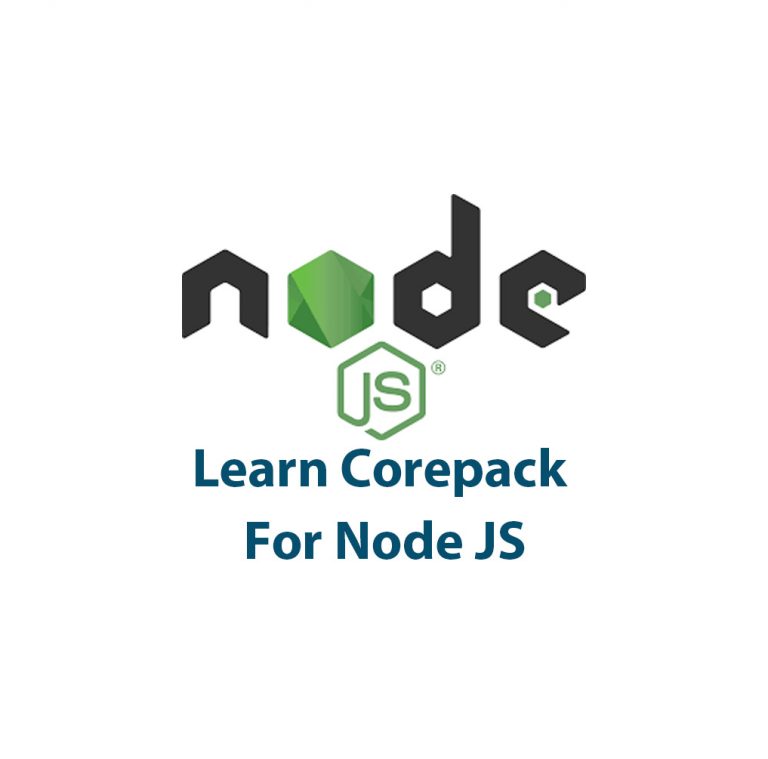
Corepack In Node JS
Have you ever worked on a Node JS project and struggled with managing dependencies? You’re not alone! Managing packages in Node JS can get confusing and messy quickly. That’s where Corepack comes in. In this article, we’ll be diving into Corepack in Node JS and how it can make dependency management a breeze. First of […]
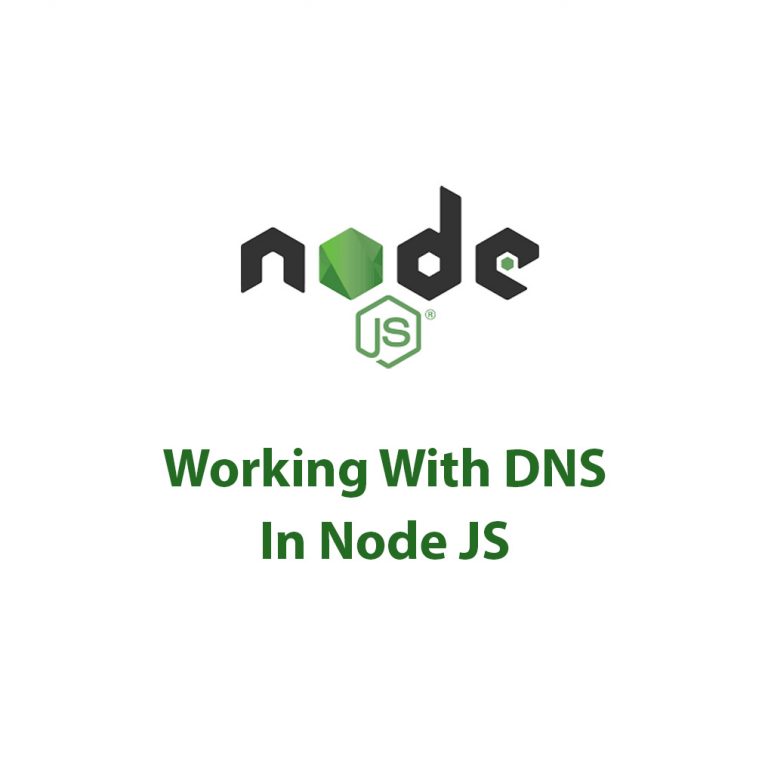
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]
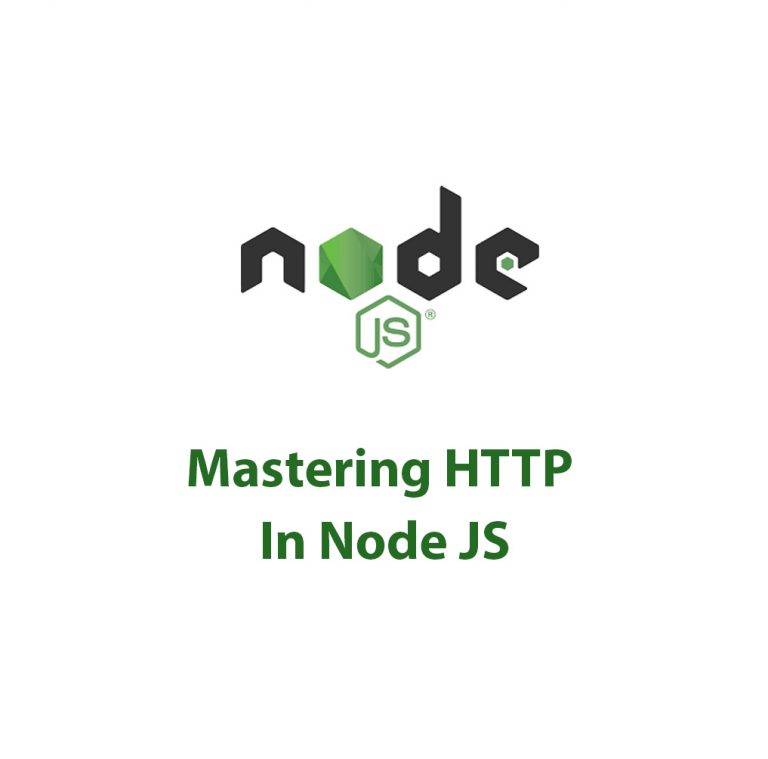
Working With HTTP In Node JS
Introduction As a developer, you may have come across the term HTTP quite a few times. It stands for Hypertext Transfer Protocol and is the backbone of how the internet works. It is the protocol you use when you visit a website, send emails, and watch videos online. In the world of programming, HTTP is […]
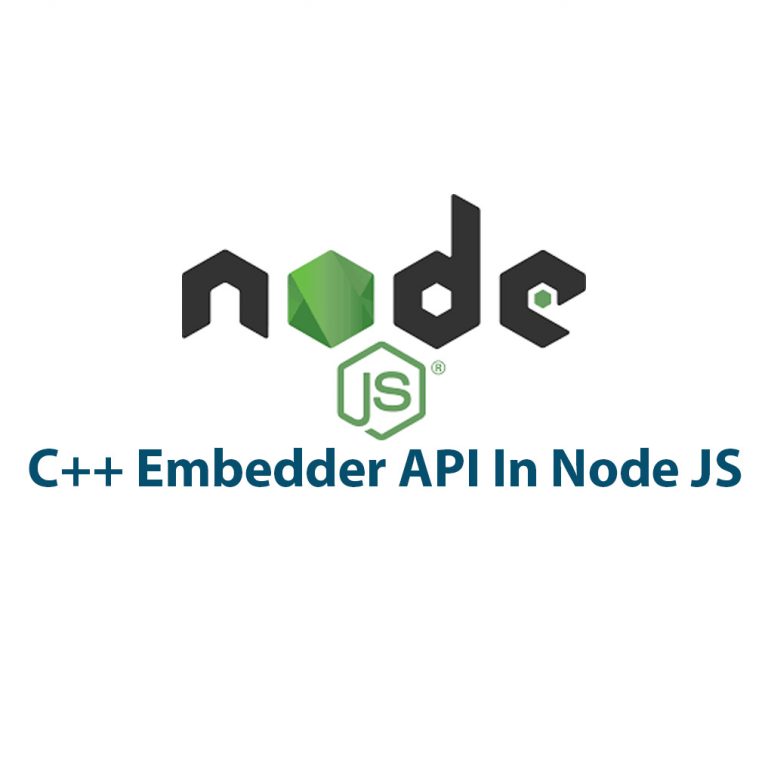
C++ Embedder API With Node JS
Introduction: As a programmer, I have always been fascinated with the power of NodeJS. It is a popular JavaScript runtime that can be used for server-side scripting. The beauty of NodeJS is that it allows for easy handling of I/O operations. However, sometimes the complexities of a project may go beyond just JavaScript coding, and […]