Introduction
As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic.
Both of these specifications are a departure from the traditional client-server model that HTTP/1.x relied heavily upon. HTTP/2 is a major revision of the HTTP protocol and WebSocket is an API that enables two-way communication channels between a client and a server.
In this article, we’ll explore the challenges and opportunities of working with HTTP/2 and WebSocket in Node JS and look at some code examples that demonstrate how to build and enhance web applications using these technologies.
What is Node JS?
Node JS is a JavaScript runtime built on top of the Chrome V8 engine. It’s a powerful way to create scalable and efficient network applications because it’s built with an event-driven, non-blocking I/O paradigm. Node JS runs on a single thread and uses non-blocking I/O operations to avoid blocking the execution of other requests.
Because Node JS uses JavaScript for coding, it has fostered an increasingly active ecosystem of open source modules, which developers can use to develop network applications quickly and easily.
Node JS is ideal for building real-time, scalable, and high-performance web applications because of its non-blocking I/O paradigm, which is a perfect fit for WebSocket’s two-way communication channels and HTTP/2’s multiple data streams.
HTTP/2 and its Advantages
HTTP/2 is a new version of the HTTP protocol, which has been in use in web applications since the early days of the web. The biggest advantage of HTTP/2 is that it enables multiple requests and responses to happen simultaneously on the same connection, which results in much faster page load times.
This new version of HTTP also brings some additional improvements in the areas of security, reliability, and scalability, as well as a number of new features, including the most exciting one: Server Push.
Server Push allows a server to send additional resources to the client before the client even knows it needs them. This feature makes it possible to optimize the performance of a web application significantly by pre-loading and pre-fetching assets. In short, HTTP/2 makes web applications significantly faster and more responsive.
WebSockets
WebSockets are another way to improve web application performance. WebSockets provide a way for a client and a server to maintain a continuous connection, allowing for real-time data transmission between the two endpoints.
WebSockets were introduced as an API in 2011, and they make it possible to create interactive web applications that are responsive and fast. A few examples of interactive web applications that use WebSockets include online games, chat apps, streaming services, and financial trading platforms.
WebSockets differ from traditional HTTP connections because they allow for bi-directional communication between a client and server without the need for the server to repeatedly handle requests. With WebSockets, the server can push data to the client in real-time without waiting for the client to request it.
Setting up a WebSocket server in Node JS
Before you can start using a WebSocket in your Node JS application, you need to create a WebSocket server. Creating a WebSocket server is easy in Node JS, and it only takes a few lines of code.
To get started, you’ll need to install the ws library. The ws library is easy to use and provides a complete WebSocket implementation.
const WebSocket = require('ws');
const websocketServer = new WebSocket.Server({ port: 8080 });
websocketServer.on('connection', (socket) => {
console.log('a client has connected');
socket.on('message', (message) => {
console.log(`received: ${message}`);
socket.send(`echo: ${message}`);
});
socket.on('close', () => {
console.log('client has disconnected');
});
});
This code creates a WebSocket server that listens on port 8080. When a client connects to the server, the code logs the connection to the console, and when the client sends a message, the server logs the message to the console and sends an echo message back to the client.
Sending and receiving data with WebSocket in Node JS
Sending and receiving data with WebSocket in Node JS is simple. The following code demonstrates basic message sending and receiving with WebSockets.
const WebSocket = require('ws');
const websocket = new WebSocket('ws://localhost:8080');
websocket.on('open', () => {
console.log('connected');
websocket.send('hello server');
});
websocket.on('message', (message) => {
console.log(`received: ${message}`);
});
In this code, we create a WebSocket client that connects to the WebSocket server on port 8080. When the connection is established, the code sends a “hello server” message to the server. When the server responds with the echo message, the client logs the message to the console.
Enriching WebSocket with HTTP/2 in Node JS
While WebSockets are great for real-time communication, they have some limitations. One of the biggest limits is that the WebSocket protocol itself doesn’t provide any built-in mechanisms for packet compression or retransmission.
To address this issue, many developers now use the SPDY (pronounced “speedy”) protocol with WebSocket. SPDY is an application-layer protocol for transporting web content.
SPDY provides compression and multiplexing for HTTP/2 streams, which were developed to address the shortcomings of HTTP/1.x. By using SPDY with WebSocket, you can create a more reliable, efficient, and scalable web application.
To use SPDY with WebSocket, you’ll need to install the spdy and spdy-transport modules. Once you’ve installed these modules, you can modify the WebSocket server code to use SPDY.
const spdy = require('spdy');
const WebSocket = require('ws');
const options = {
key: fs.readFileSync(__dirname + '/server.key'),
cert: fs.readFileSync(__dirname + '/server.crt'),
};
const server = spdy.createServer(options, (req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello World!');
});
const websocketServer = new WebSocket.Server({ server });
websocketServer.on('connection', (socket) => {
console'tion', (socket) => {
console.log('a client has connected');
socket.on('message', (message) => {
console.log(`received: ${message}`);
socket.send(`${message}`);
});
socket.on('close', () => {
console.log('client has disconnected');
});
});
server.listen(8080, () => {
console.log(`Server running on port ${server.address().port}`);
});
In this code, we create an SPDY server and a WebSocket server. We add an SPDY server that listens for incoming client requests and returns a simple “Hello World!” response. We then modify the WebSocket server to use the SPDY server before listening on port 8080.
We also modify the WebSockets server code to send a message back to the client with the same data it received. We can verify that the WebSockets server is now using SPDY by connecting to it and then inspecting the network traffic. SPYD encrypts packets and uses binary framing (used in HTTP/2) instead of text parsing (as in HTTP/1.x).
Conclusion
In conclusion, Node JS has become popular among developers because of its non-blocking I/O paradigm and scalable network capabilities. HTTP/2 and WebSockets are two newer technologies that leverage Node JS’s potential.
HTTP/2 provides many benefits to web applications, including server push, multiple data streams, and significant performance enhancements. WebSockets enable real-time, bi-directional communication between clients and servers, like streaming services, chat apps, and online games.
With Node JS, developers can create efficient and scalable web applications infrastructure that can handle HTML/2 real-time data streams and WebSocket traffic.
Whether you’re creating chat applications, online games or any other web applications, the combination of Node JS, HTTP/2, and WebSockets is an excellent option to streamline your development efforts.
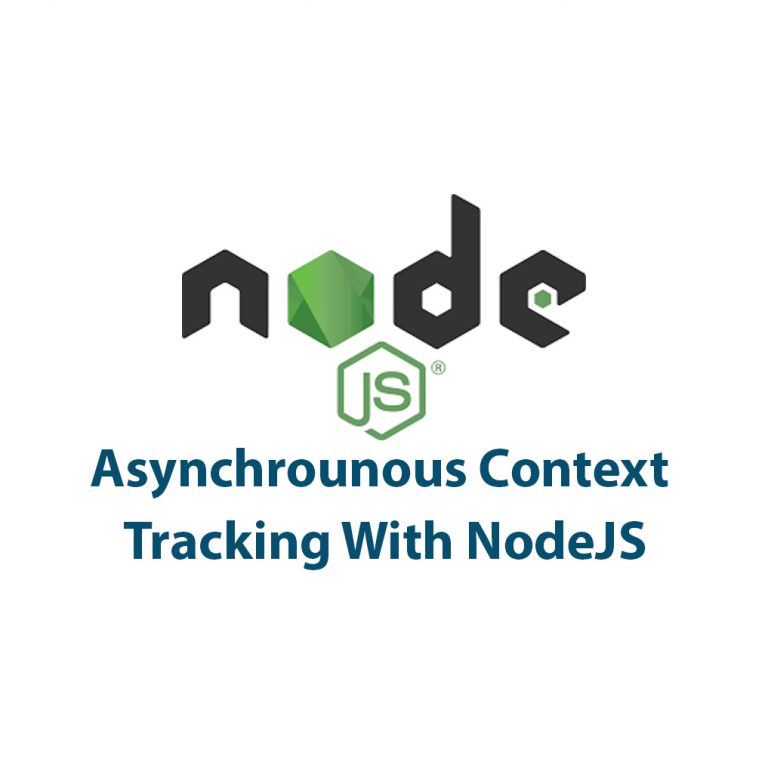
Asynchronous Context Tracking With Node JS
As someone who has spent a lot of time working with Node JS, I have come to understand the importance of Asynchronous Context Tracking in the development of high-performance applications. In this article, I will explore the concept of Asynchronous Context Tracking with Node JS, its advantages, techniques, challenges and best practices. Before we dive […]
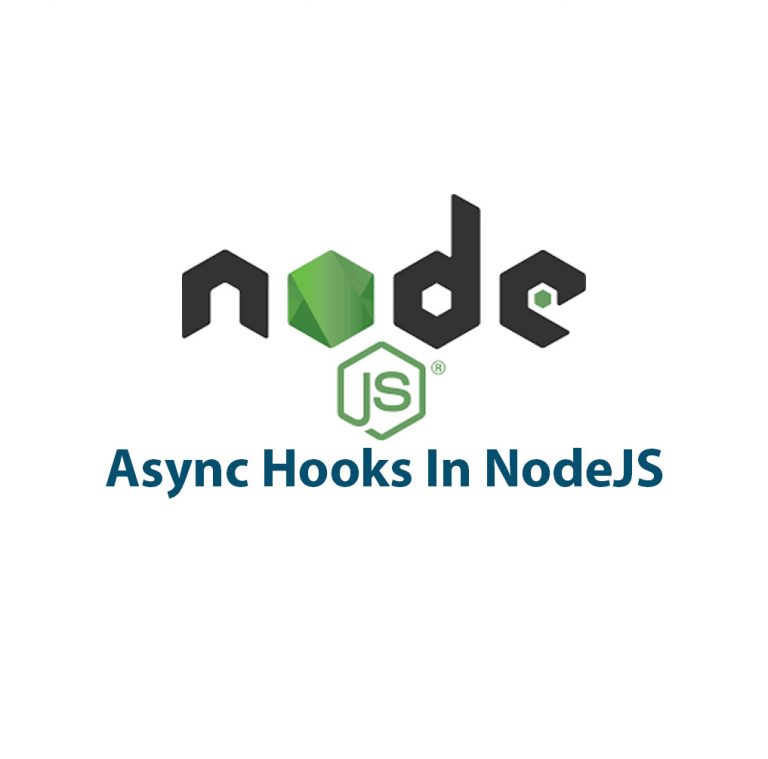
Async Hooks In Node JS
Introduction: If you’re a Node.js developer, you’ve probably heard the term “Async Hooks” thrown around in conversation. But do you know what they are or how they work? In this article, I’ll be diving into the world of Async Hooks, explaining what they are and how to use them effectively. What are Async Hooks? Async […]
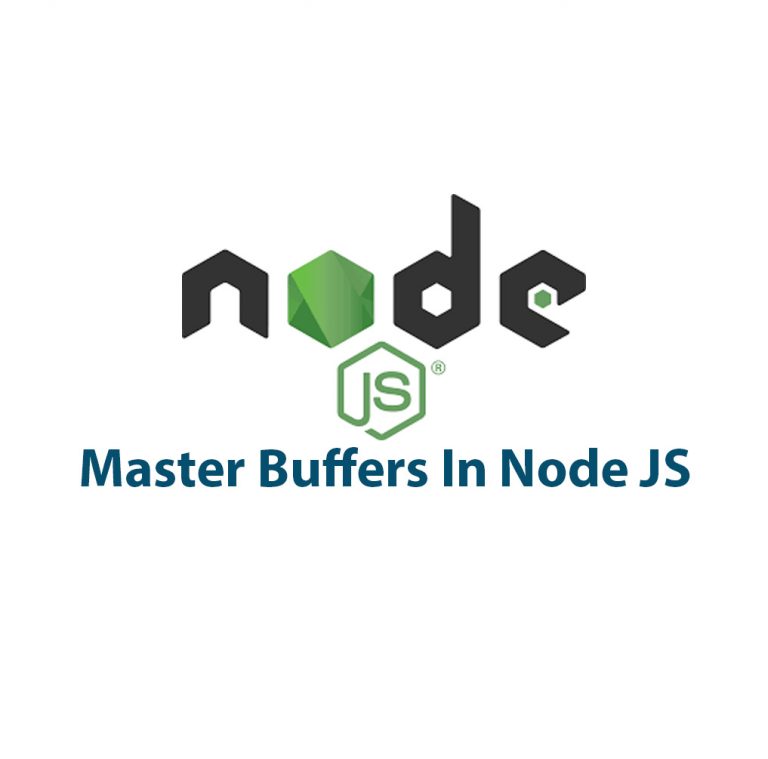
Mastering Buffers In Node JS
As someone who is just getting started with Node JS, hearing about buffers might be a bit confusing at first. What are they exactly, and why should you care about them? I know I was pretty perplexed by this concept when I first started working with Node JS. However, once I understood what buffers were […]
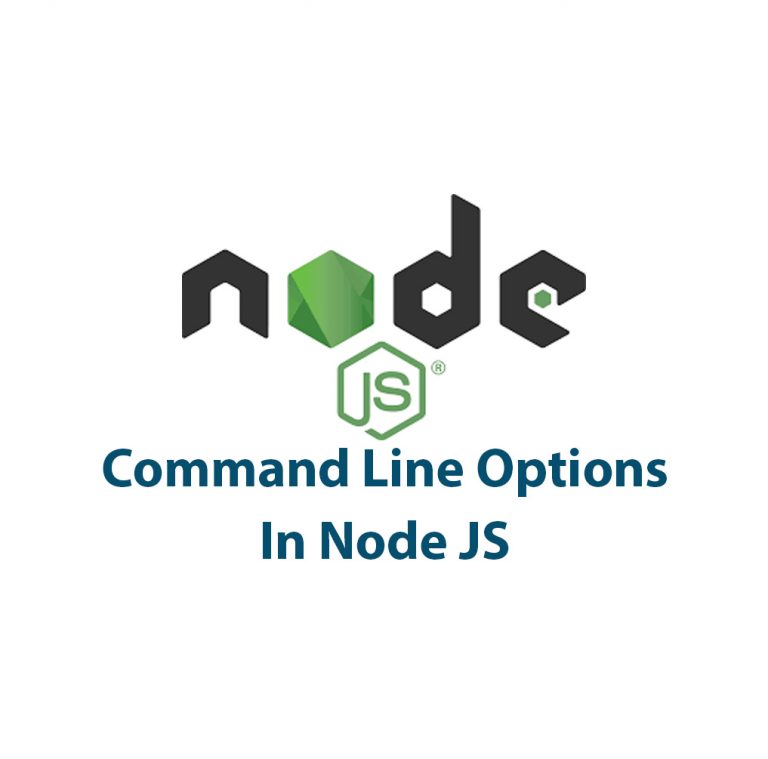
Command Line Options In Node JS
As a developer who loves working with Node JS, I’ve found myself using command line options in many of my projects. Command line options can make our Node JS programs more user-friendly and powerful. In this article, I’ll explain what command line options are, how to parse them in Node JS, how to use them […]
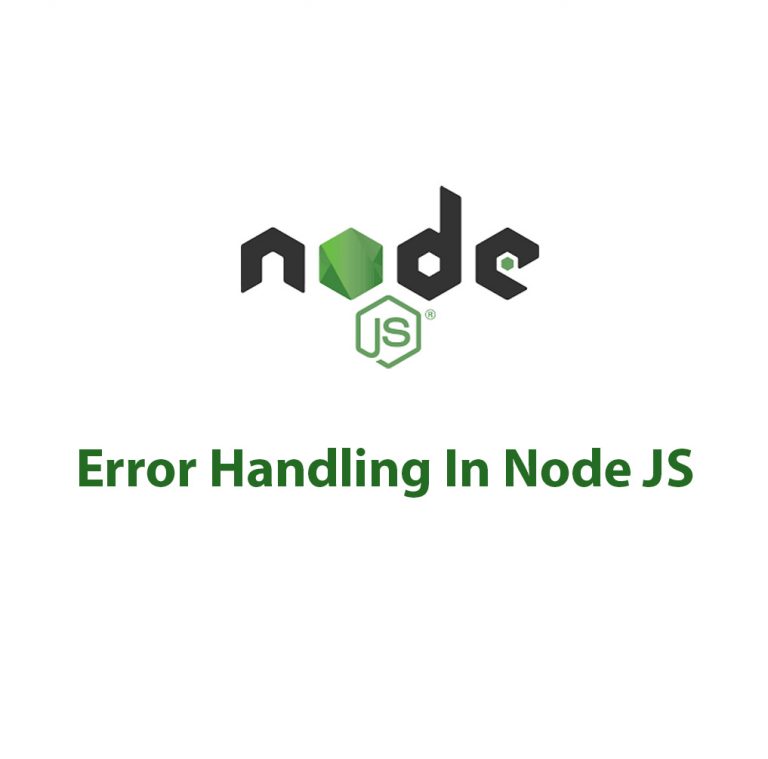
Error Handling in Node JS
A Node JS developer may struggle with error handling on occasion. Although faults are occasionally unavoidable, you can ensure that your application continues to function flawlessly by implementing error handling correctly. Let’s start by discussing the many kinds of problems you could run into when working with Node JS. The Three Types of Errors in […]
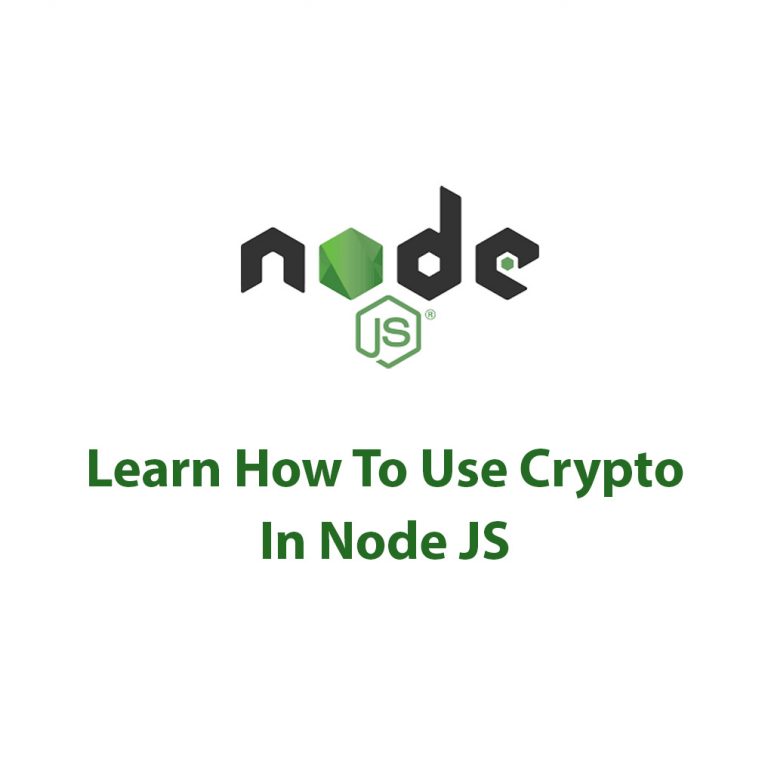
Using Crypto In Node JS
Have you ever wondered how some of the most secure websites and applications keep your data safe from malicious attacks? Well, one of the answers lies in the use of cryptography! Cryptography is the art of writing or solving codes and ciphers, and it has been around for centuries. In the world of computer science, […]